Key Notes on Computer Architecture and Assembly Language
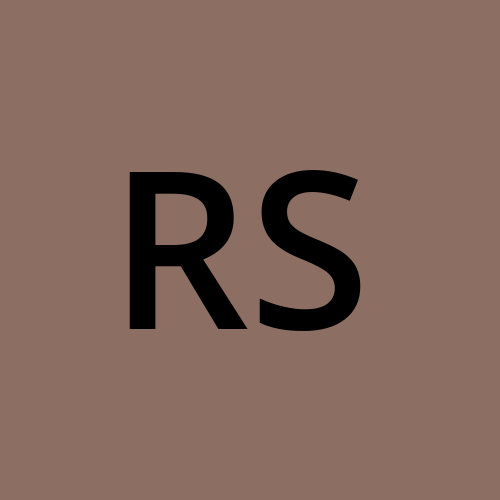
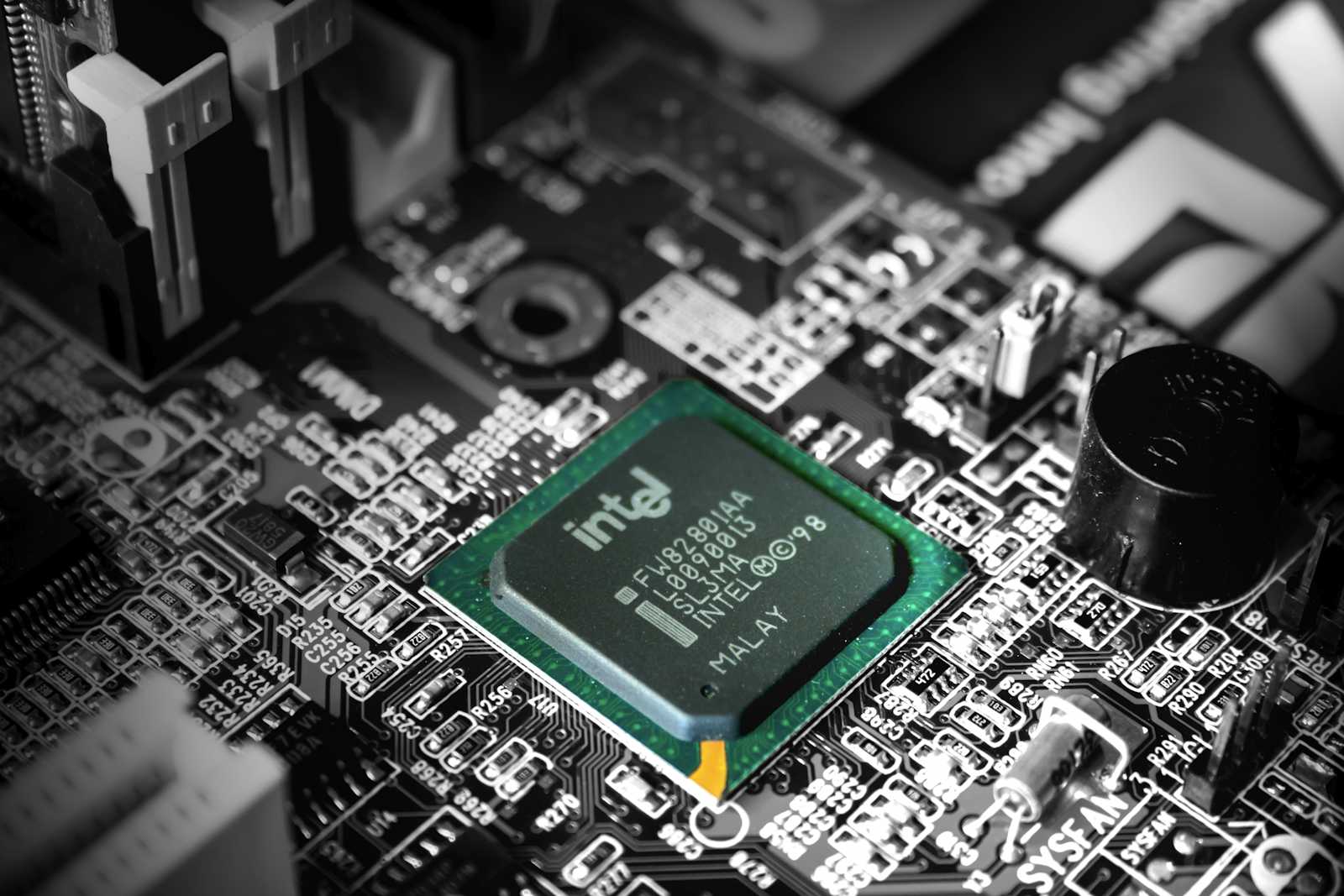
1. Basic Computer Architecture
Von Neumann Architecture:
Components: Central Processing Unit (CPU), Memory, Input/Output devices, and Storage.
Characteristics: Single bus for data and instructions, sequential instruction processing.
CPU Components:
ALU (Arithmetic Logic Unit): Performs arithmetic and logical operations.
Control Unit: Directs operations of the processor.
Registers: Small, fast storage locations for immediate data access.
Memory Hierarchy:
Registers: Fastest, smallest memory inside the CPU.
Cache: Small, fast memory between CPU and main memory.
Main Memory (RAM): Volatile memory for currently used data and instructions.
Secondary Storage: Non-volatile storage (e.g., HDD, SSD) for long-term data storage.
2. Instruction Set Architecture (ISA)
Definition: The part of the computer architecture related to programming, including the native commands, supported data types, registers, addressing modes, memory architecture, interrupt and exception handling, and external I/O.
Types of ISAs:
CISC (Complex Instruction Set Computer): Many complex instructions, fewer instructions per program.
RISC (Reduced Instruction Set Computer): Fewer, simpler instructions, more instructions per program.
Common Instructions:
Data Movement:
MOV
,LOAD
,STORE
.Arithmetic Operations:
ADD
,SUB
,MUL
,DIV
.Logical Operations:
AND
,OR
,NOT
,XOR
.Control Flow:
JMP
,CALL
,RET
,CMP
,JE
,JNE
.
3. Assembly Language
Definition: Low-level programming language that uses symbolic code and mnemonics to represent machine-level instructions.
Syntax:
Labels: Marks a location in code (e.g.,
START:
).Instructions: Operation codes (opcodes) like
MOV
,ADD
.Operands: Values or addresses used by the instructions.
Registers:
General-purpose:
EAX
,EBX
,ECX
,EDX
.Special-purpose:
ESP
(Stack Pointer),EBP
(Base Pointer),EIP
(Instruction Pointer).
Addressing Modes:
Immediate: Operand is a constant value (e.g.,
MOV AX, 5
).Direct: Operand is a memory address (e.g.,
MOV AX, [1234H]
).Indirect: Operand is a register containing the address of the data (e.g.,
MOV AX, [BX]
).Indexed: Combines base address and index (e.g.,
MOV AX, [BX+SI]
).
4. Microarchitecture
Pipeline: Technique to improve CPU performance by executing multiple instructions in different stages simultaneously.
- Stages: Fetch, Decode, Execute, Memory Access, Write Back.
Superscalar Architecture: Executes more than one instruction per clock cycle by having multiple execution units.
Out-of-Order Execution: Instructions are executed as resources are available rather than strictly in order.
5. Memory and Storage
Memory Management:
Virtual Memory: Uses disk space to simulate additional RAM.
Paging: Divides memory into fixed-size pages for efficient management.
Segmentation: Divides memory into variable-size segments based on logical divisions.
Cache Memory:
Levels: L1 (fastest, smallest), L2, L3 (larger, slower).
Cache Coherence: Mechanisms to maintain consistency between cache and main memory in multiprocessor systems.
6. Input/Output (I/O)
I/O Methods:
Polling: CPU continuously checks I/O device status.
Interrupts: I/O device sends an interrupt signal to CPU when it needs attention.
Direct Memory Access (DMA): Allows devices to transfer data directly to/from memory without CPU intervention.
7. Performance Metrics
Clock Speed: Measured in Hertz (Hz), indicates the speed of the CPU.
Instructions Per Cycle (IPC): Number of instructions the CPU can execute in one clock cycle.
Throughput: Number of instructions executed in a given period.
Latency: Time taken to complete a single task or instruction.
8. Assembly Programming Tips
Use Comments: Document code for readability.
Modular Code: Break programs into small, manageable procedures.
Debugging: Use tools like gdb or built-in debuggers to step through code.
Optimization: Focus on critical sections for performance improvement.
Conclusion
Understanding computer architecture and assembly language is crucial for developing efficient low-level software and optimizing performance. These key concepts form the foundation of how modern computers operate, enabling developers to write better, more optimized code.
Subscribe to my newsletter
Read articles from Riya Sinha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
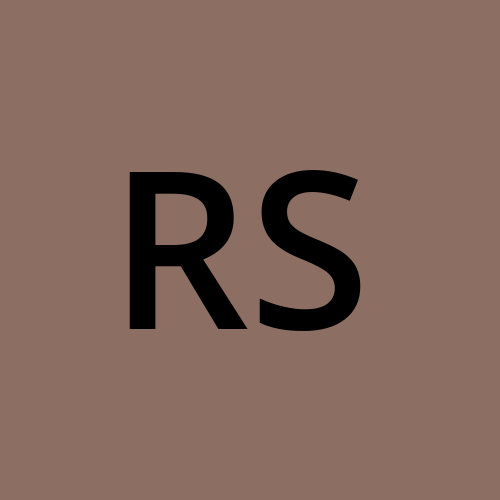
Riya Sinha
Riya Sinha
Web Developer | Data Structures and Algorithms | Hackerrank Certified developer | Amity University Patna