Installing Dependencies for Running Java, Python, and JavaScript Applications: A Simple Guide
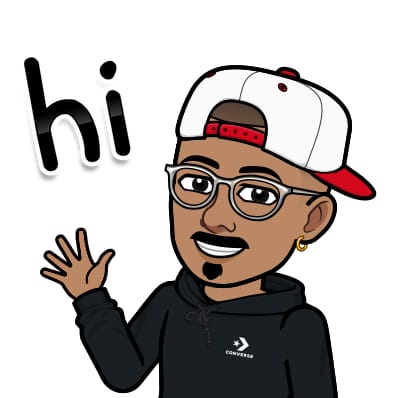
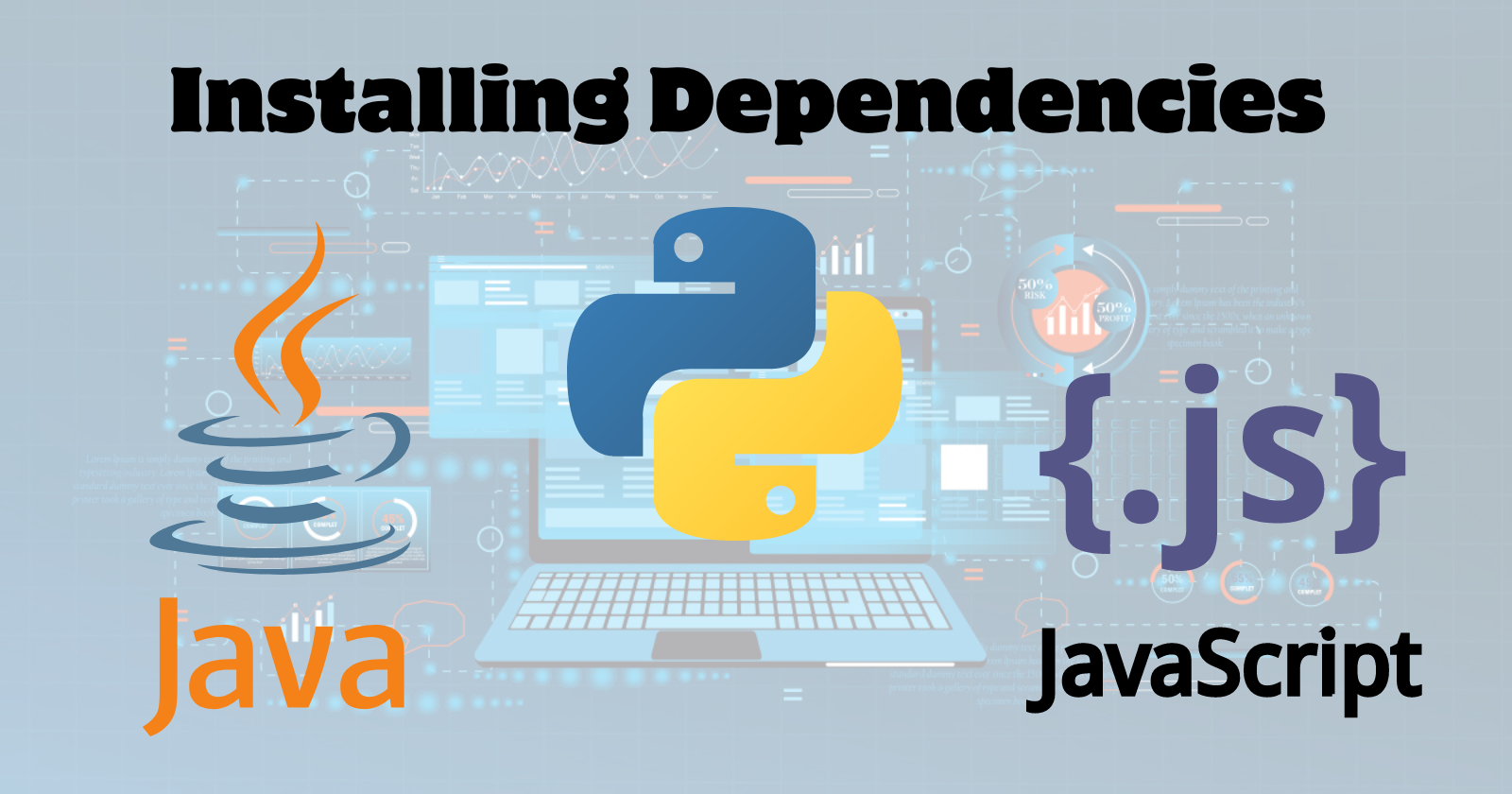
In software development, efficiently managing dependencies and setting up projects is crucial for seamless development workflows. This article provides a comprehensive guide on installing dependencies for running applications and running GitHub-cloned projects across Java, Python, and JavaScript environments..
You have cloned it, but how do you run it?
Sometimes, to run a cloned GitHub project on your system, you need to set it up properly first. This usually involves installing the necessary dependencies specified by the developer. These dependencies are often listed in specific files within the project.
For example, in a JavaScript project, you might find a package.json
file that lists all the required npm
packages.
In a Python project, there could be a requirements.txt
file containing the necessary libraries.
For Java projects, dependencies are typically managed through a pom.xml
file if using Maven, or a build.gradle
file if using Gradle.
By installing these dependencies, you ensure that your environment matches the one in which the project was originally developed, allowing the application to run smoothly on your system.
Installing Dependencies for Running Applications
Java Projects
Java projects commonly use build automation tools like Maven or Gradle for dependency management and project building.
Generate Dependency File:
Here’s how you can approach generating a list of dependencies for a Java project using Maven or Gradle:
Using Maven:
Navigate to Your Maven Project: Open a terminal or command prompt and change the directory (
cd
) to your Maven project's root directory.Generate
pom.xml
: If you don't already have apom.xml
file, you need to create one. Typically, Maven projects are initialized with apom.xml
that specifies project details and dependencies.List Dependencies: Maven provides a way to list all dependencies of your project using the following command:
mvn dependency:list
This command generates a list of dependencies and their versions used in your Maven project.
Using Gradle:
Navigate to Your Gradle Project: Change the directory (
cd
) to your Gradle project's root directory.Generate
build.gradle
: If you don't already have abuild.gradle
file, you need to create one. Gradle projects are initialized with abuild.gradle
file that specifies project details and dependencies.List Dependencies: Gradle provides a way to list all dependencies of your project using the following command:
gradle dependencies
This command generates a detailed report of dependencies used in your Gradle project.
Install Dependencies:
To install dependencies and run a Java project after cloning from Git, use build tools like Maven or Gradle. Here's a general guide:
Using Maven:
Navigate to Your Project Directory: Open a terminal or command prompt and change the directory (
cd
) to your Java project's root directory.Ensure
pom.xml
Exists: Maven projects require apom.xml
file that specifies dependencies and project configurations. If it's missing, you won't be able to proceed with Maven.Install Dependencies: Use Maven to download and install dependencies listed in
pom.xml
:mvn clean install
clean
: Cleans any existing build files.install
: Downloads dependencies and builds the project.
Run the Java Project: After dependencies are installed, you can run your Java application. If it's a web application using an embedded server like Tomcat, you might use Maven's
tomcat7-maven-plugin
or similar:mvn tomcat7:run
- This command starts the embedded Tomcat server and deploys your application.
Using Gradle:
Navigate to Your Project Directory: Change the directory (
cd
) to your Java project's root directory.Ensure
build.gradle
Exists: Gradle projects require abuild.gradle
file that specifies dependencies and project configurations. If it's missing, you won't be able to proceed with Gradle.Install Dependencies: Use Gradle to download and install dependencies listed in
build.gradle
:gradle build
build
: Downloads dependencies and builds the project.
Run the Java Project: After dependencies are installed, you can run your Java application. If it's a Spring Boot application, for example:
gradle bootRun
- This command starts the embedded Spring Boot server and runs your application.
Additional Notes:
Checking Project Requirements: Ensure that you have Java Development Kit (JDK) installed and configured properly on your system. Maven and Gradle rely on the JDK to compile and run Java projects.
Managing Dependencies: Maven and Gradle manage dependencies by downloading required libraries from remote repositories (like Maven Central) and caching them locally. This ensures that dependencies are consistent across different environments.
Build Output: After running
mvn clean install
orgradle build
, the compiled Java classes and packaged artifacts (like JAR files) will typically be located intarget/
(for Maven) orbuild/
(for Gradle) directory.
By following these steps, you can effectively install dependencies and run your Java project after cloning it from Git, leveraging the capabilities of Maven or Gradle to handle dependency management and project building.
Python Projects
Python projects use pip
for managing dependencies and requirements.txt
for listing them.
Generate Dependency File:
To generate arequirements.txt
file listing all the Python packages and their versions for your project, follow these steps:
Navigate to the Project Directory: Open a terminal or command prompt and change the directory (
cd
) to the root directory of your development project.Activate Your Virtual Environment (Optional but Recommended): If you're using a virtual environment (
venv
orvirtualenv
)(recommended for Python projects), activate it. For example, usingvenv
:On Windows:
venv\Scripts\activate
On Unix/macOS:
source venv/bin/activate
Generate
requirements.txt
: Once your environment is active, use thepip freeze
command to generate a list of installed packages and their exact versions:pip freeze > requirements.txt
This command should be used after running your application successfully so that you can share this requirements file along with your Python application.
This command saves the output of
pip freeze
, which lists all installed packages and their versions, into a file namedrequirements.txt
in the current directory.The
>
symbol redirects the output ofpip freeze
to a file.
Install Dependencies:
To run arequirements.txt
file after cloning a Python Project, you typically follow these steps:
Navigate to the Project Directory: Open a terminal or command prompt and change the directory (
cd
) to the root directory of your cloned project.Activate Your Virtual Environment: If you're using a virtual environment, activate it as mentioned above.
Install Dependencies: Use
pip
to install the dependencies listed inrequirements.txt
. Run the following command:pip install -r requirements.txt
This command will read
requirements.txt
and install all the Python packages listed there along with their specific versions.Verify Installation: After installation completes, you can verify that all dependencies were installed correctly. You might also want to check the versions of installed packages against what you expect.
Proceed with Project Setup: Depending on the project, there might be additional setup steps such as database migrations, environment variable configuration, or starting a development server. Refer to any README or documentation files provided with the repository for specific instructions.
Additional Notes:
Update
requirements.txt
Periodically: It's good practice to updaterequirements.txt
whenever you add or update packages in your project.Include Only Necessary Packages: Review
requirements.txt
to ensure it only includes packages essential for your project. Remove any that are no longer needed.
By following these steps, you can create and maintain a requirements.txt
file that accurately reflects the dependencies of your Python project, making it easier to manage and replicate your development environment.
JavaScript Projects
JavaScript projects dependencies are managed using npm
(Node Package Manager) or yarn
. These tools maintain a package.json
file that lists all dependencies along with their versions.
Generate Dependency File:
To generate apackage.json
file that lists all the required dependencies for your development project, you can follow these steps:
Using npm:
Navigate to Your Project Directory: Open a terminal or command prompt and change the directory (
cd
) to your development project's root directory.Generate
package.json
: Initialize it by running:npm init -y
This creates a basic
package.json
file with default settings.Install Dependencies: Install all the dependencies needed for your JavaScript project using
npm install
:npm install --save <package-name>
Replace
<package-name>
with the name of each package you want to install. For example:npm install --save react react-dom
This command installs React and ReactDOM and saves them as dependencies in
package.json
.
Using yarn:
If you're using yarn
instead of npm
, you can achieve a similar result:
Navigate to Your Project Directory: Change the directory (
cd
) to your JavaScript project's root directory.Initialize
package.json
: If you haven't already, create apackage.json
file by running:yarn init -y
Install Dependencies: Install your dependencies using
yarn add
:yarn add <package-name>
For example:
yarn add react react-dom
Install Dependencies:
To install dependencies after cloning a JavaScript Project, you typically follow these steps:
Using npm:
Navigate to Your Project Directory: Open a terminal or command prompt and change the directory (
cd
) to your root directory of your cloned project.Install Dependencies: Use
npm
to install dependencies listed inpackage.json
:npm install
- This command reads the
package.json
file in the current directory and installs all dependencies listed underdependencies
anddevDependencies
.
- This command reads the
Review Installation: After installation completes,
npm
will create anode_modules
directory in your project's root directory. This directory contains all the installed packages.
Using yarn:
If your project uses yarn
instead of npm
, the process is similar:
Navigate to Your Project Directory: Change the directory (
cd
) to your JavaScript project's root directory.Install Dependencies: Use
yarn
to install dependencies listed inpackage.json
:yarn install
- This command reads the
package.json
file in the current directory and installs all dependencies listed underdependencies
anddevDependencies
.
- This command reads the
Review Installation: After installation completes,
yarn
will also create anode_modules
directory in your project's root directory containing all installed packages.
Additional Notes:
Dependencies vs. devDependencies:
dependencies
: These are packages required for your application to function.devDependencies
: These are packages needed for development and testing purposes.
Lock Files: Both
npm
andyarn
generate lock files (package-lock.json
oryarn.lock
) to lock down the versions of dependencies installed, ensuring consistency across different environments.Sharing Projects: When sharing your project with others, it's common practice to include the
package.json
(andpackage-lock.json
oryarn.lock
) but not thenode_modules
directory. Others can then runnpm install
oryarn install
to install dependencies based on these files.
By following these steps, you can effectively manage and install dependencies for your JavaScript project using either npm
or yarn
, depending on your preferred package manager.
Conclusion
Efficiently managing project dependencies and setup is essential for productive software development. By leveraging tools like Maven, pip, npm, and GitHub, developers can streamline workflows, collaborate effectively, and ensure project consistency across teams.
Explore further customization options and tools specific to your project needs. Continuous learning and adaptation are key to mastering project setup and management.
Subscribe to my newsletter
Read articles from Jasai Hansda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
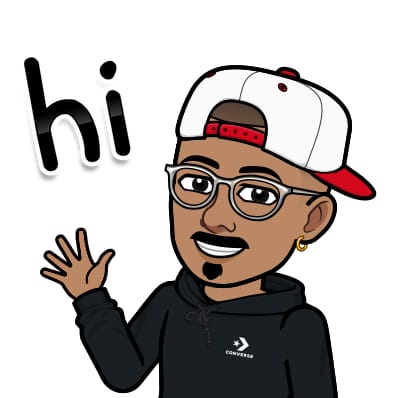
Jasai Hansda
Jasai Hansda
Software Engineer (2 years) | In-transition to DevOps. Passionate about building and deploying software efficiently. Eager to leverage my development background in the DevOps and cloud computing world. Open to new opportunities!