The Ultimate Guide to Web Development with Elixir and Phoenix Framework
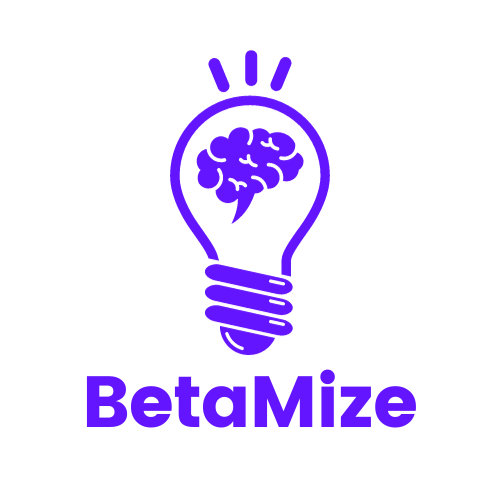
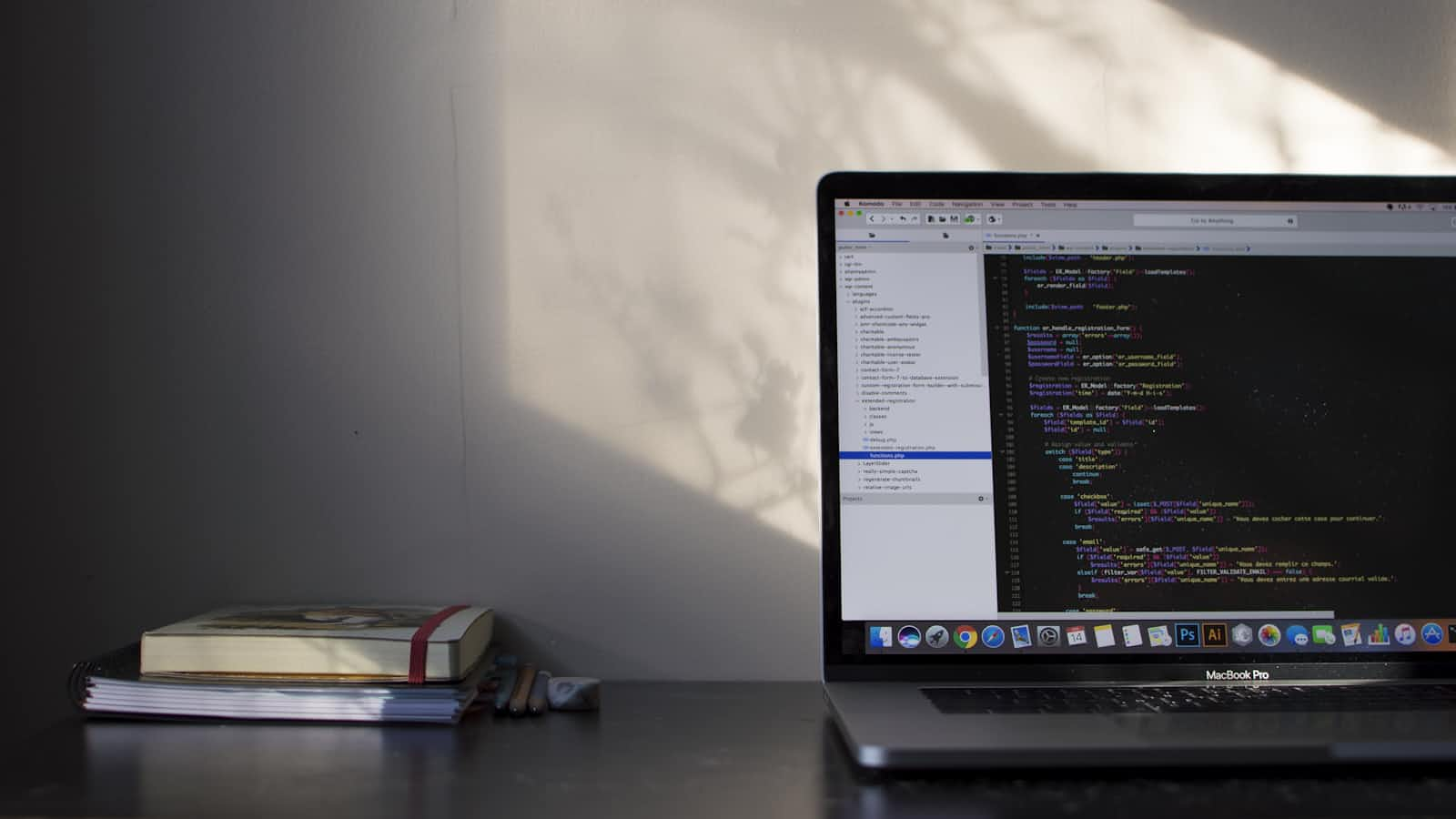
Elixir, a functional and concurrent programming language, has gained significant popularity for web development, thanks to its robust performance and scalability. At the heart of Elixir's web development capabilities is the Phoenix framework, which offers a modern, productive, and high-performance environment for building web applications. In this blog, we'll explore the key features and benefits of web development with Elixir and Phoenix, delve into practical examples, and highlight how ElixirMasters can help you succeed in your Elixir projects.
Why Choose Elixir for Web Development?
1. High Performance
Elixir's concurrency model, built on the Erlang VM, allows for efficient handling of many simultaneous connections. This makes Elixir an ideal choice for web applications that require real-time communication, such as chat applications, live updates, and online gaming platforms. The language's ability to handle numerous simultaneous connections with low latency ensures a smooth user experience even under high load.
2. Scalability
Elixir's lightweight processes and distributed nature enable applications to scale seamlessly. Whether you need to handle a spike in traffic or distribute your application across multiple nodes, Elixir provides the tools to scale efficiently. This scalability is crucial for growing applications that need to maintain performance and reliability as they expand.
3. Fault Tolerance
Inheriting Erlang's "let it crash" philosophy, Elixir applications are designed to be resilient. With robust supervision trees and process management, your web applications can recover gracefully from unexpected failures. This fault-tolerant approach ensures high availability and reliability, which is especially important for mission-critical applications.
4. Developer Productivity
Elixir's syntax is clean and expressive, promoting code readability and maintainability. Combined with the powerful features of the Phoenix framework, developers can build complex web applications quickly and efficiently. Elixir also encourages best practices such as immutability and functional programming, which reduce bugs and enhance code quality.
Introducing Phoenix: The Web Framework for Elixir
Phoenix is a web development framework that leverages Elixir's strengths to deliver a fast and productive environment for building web applications. Here are some of the key features that make Phoenix stand out:
1. Real-Time Communication with Channels
Phoenix Channels provide a simple and powerful way to handle real-time communication over WebSockets. This is particularly useful for building interactive applications like chat systems, notifications, and live updates. Channels enable seamless bi-directional communication between the server and clients.
defmodule MyAppWeb.UserSocket do
use Phoenix.Socket
channel "room:*", MyAppWeb.RoomChannel
def connect(%{"token" => token}, socket, _connect_info) do
{:ok, assign(socket, :user_id, token)}
end
def id(_socket), do: nil
end
2. MVC Architecture
Phoenix follows the Model-View-Controller (MVC) pattern, making it easy to organize and manage your codebase. This separation of concerns enhances maintainability and scalability. Models handle data and business logic, views manage the presentation layer, and controllers act as intermediaries between models and views.
3. Ecto: The Database Wrapper
Ecto is a powerful database wrapper and query generator that integrates seamlessly with Phoenix. It provides a robust and flexible way to interact with databases, perform migrations, and validate data. Ecto's composable queries and changeset validations ensure data integrity and consistency.
defmodule MyApp.Accounts.User do
use Ecto.Schema
import Ecto.Changeset
schema "users" do
field :name, :string
field :email, :string
timestamps()
end
def changeset(user, attrs) do
user
|> cast(attrs, [:name, :email])
|> validate_required([:name, :email])
|> unique_constraint(:email)
end
end
4. Built-in Testing Tools
Phoenix comes with built-in support for ExUnit, Elixir's testing framework, making it easy to write and run tests for your web application. This ensures that your code is reliable and maintainable. Writing comprehensive tests for your modules, functions, and processes helps catch bugs early and improves overall code quality.
5. LiveView: Interactive, Real-Time UI
Phoenix LiveView enables rich, real-time user experiences without the need for JavaScript. LiveView allows you to build interactive, real-time user interfaces directly in Elixir, making it easy to create dynamic applications with minimal client-side code. This significantly reduces complexity and improves maintainability.
defmodule MyAppWeb.CounterLive do
use Phoenix.LiveView
def render(assigns) do
~L"""
<div>
<h1>Count: <%= @count %></h1>
<button phx-click="increment">Increment</button>
</div>
"""
end
def mount(_params, _session, socket) do
{:ok, assign(socket, :count, 0)}
end
def handle_event("increment", _value, socket) do
{:noreply, update(socket, :count, &(&1 + 1))}
end
end
Getting Started with Phoenix
1. Install Elixir and Phoenix
First, ensure you have Elixir installed on your machine. Then, install the Phoenix framework by following the official installation guide here.
2. Create a New Phoenix Project
Run the following command to create a new Phoenix project:
mix phx.new my_app
cd my_app
mix ecto.create
This sets up a new Phoenix application with a default directory structure and dependencies.
3. Start the Phoenix Server
Start the Phoenix server using the following command:
mix phx.server
Your new Phoenix application is now running, and you can access it by navigating to http://localhost:4000
in your browser.
Conclusion
Web development with Elixir and Phoenix offers a powerful, scalable, and efficient environment for building modern web applications. With features like real-time communication, robust performance, and a productive development experience, Elixir and Phoenix are an excellent choice for your next web project.
If you're ready to harness the power of Elixir and Phoenix for your web development needs, visit ElixirMasters. ElixirMasters provides expert Elixir and Phoenix development services, developer hiring solutions, and consultancy to help you build and scale your web applications effectively.
Subscribe to my newsletter
Read articles from BetaMize directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
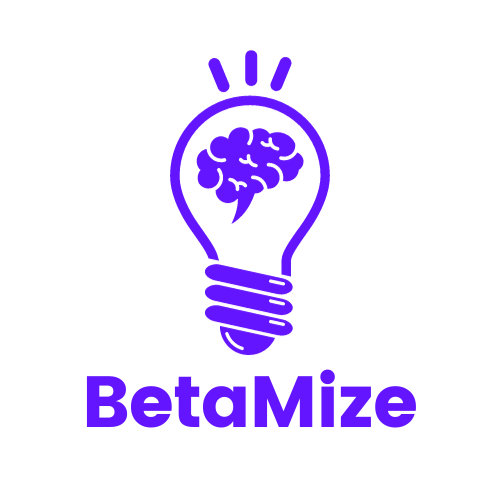