Understanding Context API in React: Managing State Efficiently
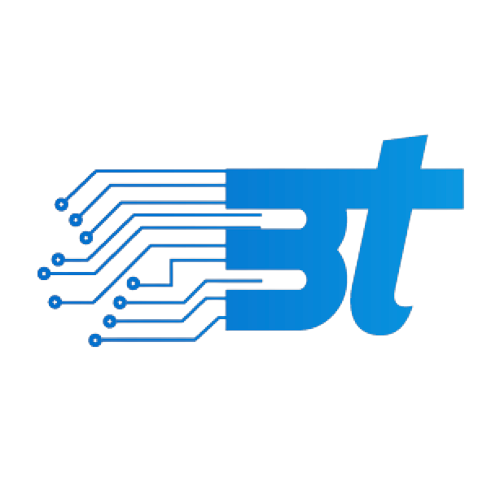
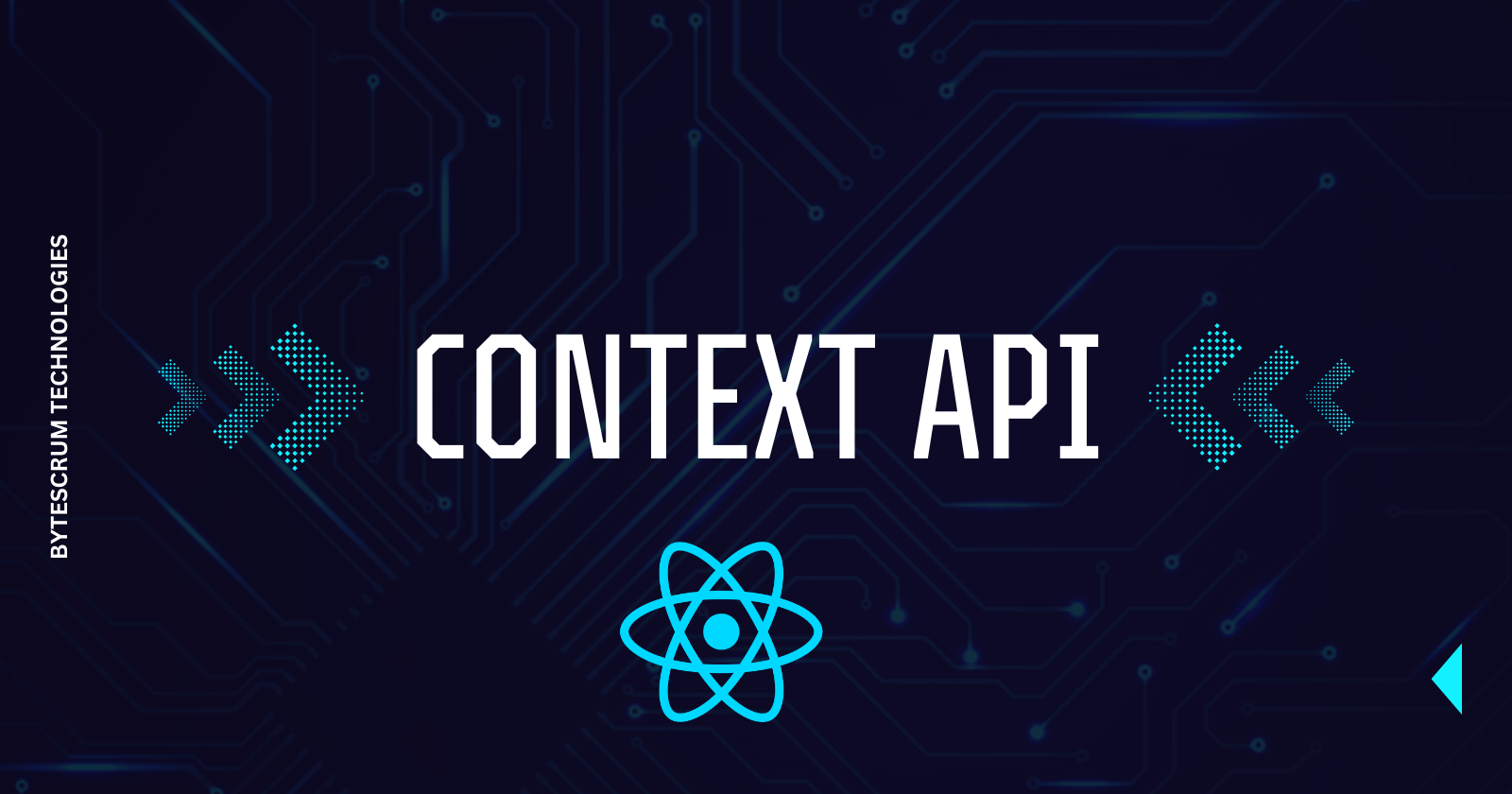
State management is a fundamental aspect of building React applications. As your application grows, passing props through multiple levels of components can become cumbersome and lead to what's known as "prop drilling." The Context API, introduced in React 16.3, offers a more efficient way to share state across your component tree without prop drilling. In this blog post, we'll dive into the Context API, explore its benefits, and demonstrate how to use it effectively.
What is Context API?
The Context API is a React feature that allows you to share state and functions across your component tree without passing props manually at every level. It provides a way to create global variables that can be passed around and accessed by any component in your application.
Why Use Context API?
Avoid Prop Drilling:
The Context API eliminates the need to pass props through intermediate components, simplifying the component tree and making your code cleaner.
Centralized State Management:
It allows you to centralize state management for related components, making it easier to manage and update state.
Improved Code Readability:
By reducing the number of props passed around, your code becomes more readable and maintainable
Creating and Using Context API
Let's walk through an example to illustrate how to use the Context API in a React application.
Step 1: Creating a Context
First, create a new context using the createContext
method:
import React, { createContext, useState } from 'react';
// Create a new context
const MyContext = createContext();
export const MyProvider = ({ children }) => {
const [state, setState] = useState('Hello, World!');
return (
<MyContext.Provider value={{ state, setState }}>
{children}
</MyContext.Provider>
);
};
Step 2: Providing Context
Wrap your component tree with the MyProvider
component to make the context available to all child components:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { MyProvider } from './MyContext';
ReactDOM.render(
<MyProvider>
<App />
</MyProvider>,
document.getElementById('root')
);
Step 3: Consuming Context
Use the useContext
hook to access the context values in any component:
import React, { useContext } from 'react';
import { MyContext } from './MyContext';
const MyComponent = () => {
const { state, setState } = useContext(MyContext);
return (
<div>
<p>{state}</p>
<button onClick={() => setState('Hello, React!')}>Change Text</button>
</div>
);
};
export default MyComponent;
Advanced Usage: Context API with Reducer
For more complex state management, you can combine the Context API with the useReducer
hook. This approach is useful for managing state transitions in a predictable way:
import React, { createContext, useReducer } from 'react';
// Create a new context
const MyContext = createContext();
// Define an initial state
const initialState = { count: 0 };
// Define a reducer function
const reducer = (state, action) => {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
};
export const MyProvider = ({ children }) => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<MyContext.Provider value={{ state, dispatch }}>
{children}
</MyContext.Provider>
);
};
To consume the context in a component:
import React, { useContext } from 'react';
import { MyContext } from './MyContext';
const MyComponent = () => {
const { state, dispatch } = useContext(MyContext);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
};
export default MyComponent;
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
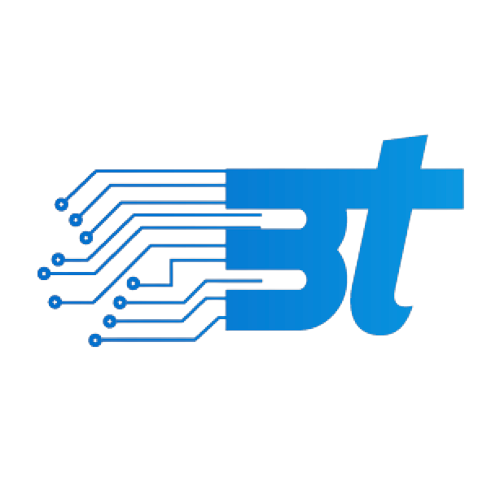
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.