(lt.46) Beyond Hello World: Explore Node.js Functionality with http, os, fs & path

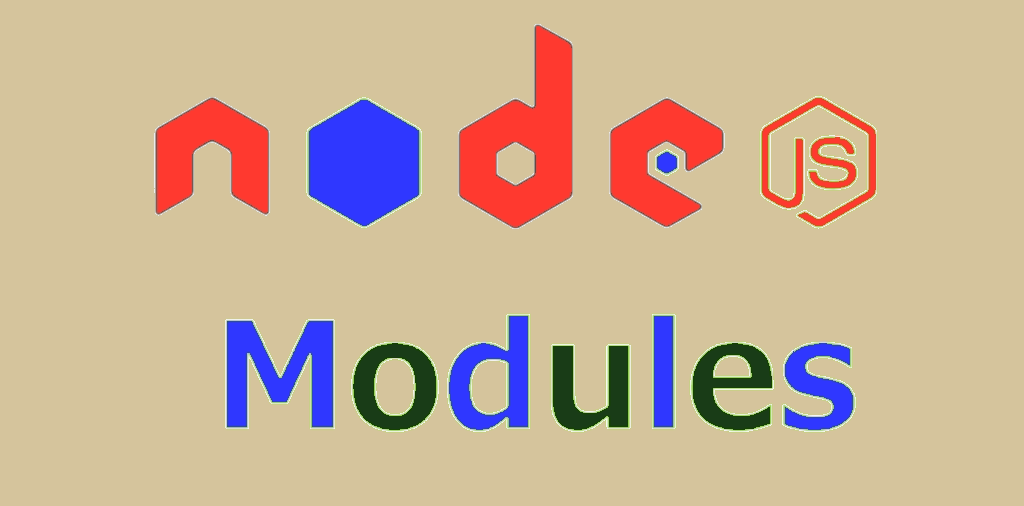
The path module in Node.js provides utilities for working with file and directory paths. It can be used to manipulate and handle file paths in a way that is cross-platform.
Here are some common methods(coding demonstration)
const path = require("path")
// console.log(path.sep)
// console.log(process.env.PATH)
const currrentPath = __filename
// console.log(currrentPath)
// console.log(__dirname)
// let a = path.basename(currrentPath)
// console.log(a)
let baseNamewithoutExt = path.basename(currrentPath , '.js')
console.log(baseNamewithoutExt)
let dirnames = path.dirname(currrentPath);
console.log(dirnames)
let findExt = path.extname(currrentPath)
console.log(findExt)
//
let findExt1 = path.extname('hwlo.html.js')
console.log(findExt1)
// no matter how many path you give node will print only the last extension
// how to add directory and base
let pathofFile = path.format({
dir :':new\home',
base : 'index.css'
});
console.log(pathofFile)
// to check whether my path is absolute or relative
console.log(path.isAbsolute(currrentPath))
console.log(path.isAbsolute('/index1.css'))
console.log(path.isAbsolute('./index1.css'))
// for joining differnet paths
let joinPath = path.join('/home' ,'js', 'home' ,'index.js')
console.log(joinPath)
// // to parse path of a file
console.log(path.parse(currrentPath))
// to find relative path
console.log(path.relative('/home/user/con' ,'/home/user/js'))
console.log("+++++++")
// to resolve
console.log(path.resolve())
/*++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/
const path = require('path');
const filePath = '/user/local/bin/file.txt';
console.log('Basename:', path.basename(filePath));
// Outputs: 'file.txt'
console.log('Directory:', path.dirname(filePath));
// Outputs: '/user/local/bin'
console.log('Extension:', path.extname(filePath));
// Outputs: '.txt'
console.log('Join:', path.join('/user', 'local', 'bin', 'file.txt'));
// Outputs: '/user/local/bin/file.txt'
console.log('Resolve:', path.resolve('file.txt'));
// Outputs the absolute path of 'file.txt' in the current directory
console.log('Normalize:', path.normalize('/user/local/../bin/file.txt'));
// Outputs: '/user/bin/file.txt'
console.log('Parse:', path.parse(filePath));
// Outputs the parsed path object
console.log('Format:', path.format({
root: '/',
dir: '/user/local/bin',
base: 'file.txt'
})); // Outputs: '/user/local/bin/file.txt'
FS module
The fs
module in Node.js is used for interacting with the file system. It allows you to read, write, update, delete, and perform many other operations on files and directories.
Key Features of the fs Module
Reading Files: You can read the contents of a file.
Writing Files: You can create a new file with specified content or overwrite an existing file.
Appending Files: You can add new content to the end of an existing file.
Deleting Files: You can delete a specified file.
Managing Directories: You can create, read, and remove directories (folders).
File Information: You can get information about a file or directory, such as its size, creation date, and permissions.
How to Use the fs Module
First, you need to include the fs module in your Node.js application:
const fs = require('fs');
const fs = require("fs");
const { buffer } = require("stream/consumers");
// async way to read file
// console.log("start")
// fs.readFile('fs/input.txt' , function(err,data)
// {
// if(err)
// {
// console.log('error' , err)
// return err
// }
// console.log('data : ', data.toString())
// console.log('read data')
// return data
// });
// console.log("finish")
// // synchronous way to read file
// console.log("synchronous way ie blocking operation")
// console.log("start");
// var data = fs.readFileSync("fs/input.txt");
// console.log("data : ", data.toString());
// console.log("read data");
// console.log("finish");
// another method to read a file using low level api
// const buf = new Buffer(1024)
// fs.open('fs/input.txt','r+',function(err, fd)
// {
// if(err)
// {
// console.log("error file" , err)
// }
// console.log("file opened")
// fs.read(fd , buf , 0 , buf.length ,0, function(errs, dataSize)
// {
// if (errs)
// {
// console.log("error in handling the file")
// }
// console.log("data size : ", dataSize)
// console.log("data is : ", buf.slice(0 , dataSize).toString())
// })
// });
// // to write in a file
// fs.writeFile('fs/input.txt' , 'hehe good' , function(err)
// {
// if(err)
// {
// console.log(" error in writing a file")
// }
// else
// {
// console.log("success in writing a file")
// }
// });
// when we have to append in a file
// fs.appendFile("fs/input.txt" , " appending new heading" ,"utf8", function(error )
// {
// if(error)
// {
// console.log("error present cant append")
// }
// console.log("appened successfully")
// })
// // for closing a file
// const buf = new Buffer(1024)
// fs.open('fs/input.txt','r+',function(err, fd)
// {
// if(err)
// {
// console.log("error file" , err)
// }
// console.log("file opened")
// fs.read(fd , buf , 0 , buf.length ,0, function(errs, dataSize)
// {
// if (errs)
// {
// console.log("error in handling the file")
// }
// console.log("data size : ", dataSize)
// console.log("data is : ", buf.slice(0 , dataSize).toString())
// })
// fs.close(fd, function(er)
// {
// if(er)
// {
// console.log("there is a error in closing of a file")
// }
// console.log("closed successfully")
// })
// });
// // deleting file
fs.unlink('fs/test.txt', function(er)
{
if(er)
{
console.log("there is a error ")
}
console.log("deleted successfully")
})
OS module
The os module in Node.js provides utility methods and properties for interacting with the operating system. It allows you to get information about the computer and operating system on which your Node.js application is running.
Key Features of the os Module
Getting System Information: Retrieve details about the system's architecture, platform, and release.
Getting User Information: Fetch information about the current user, such as the home directory.
System Uptime: Find out how long the system has been running.
Memory Information: Get details about the system's memory usage.
CPU Information: Access information about the system's CPUs.
Network Interfaces: Retrieve details about the network interfaces.
How to use os module
const os = require("os")
console.log("cpu " , +os.arch())
console.log("free memory " , os.freemem())
console.log("total memory " , os.totalmem())
console.log("network interface " + JSON.stringify(os.networkInterfaces()))
console.log("temp dir " + os.tmpdir())
console.log("temp dir " + os.hostname())
console.log("os type " + os.type())
console.log("os type " + os.platform())
console.log("os release " + os.release())
console.log("os endianess " + os.endianness())
HTTP module
The http module protocol is used for transmitting data over the internet, in Node.js it allows you to create HTTP servers and make HTTP requests. It's a core module, meaning it comes with Node.js and doesn't need to be installed separately. It is used for building web applications and handling HTTP interactions.
Key Features of the http Module
Creating HTTP Servers: Set up a server that listens for incoming HTTP requests.
Handling Requests and Responses: Manage the data sent to and received from the client.
Making HTTP Requests: Send HTTP requests to other servers and handle the responses.
How to Use the http Module
const http = require('http')
const srvr = http.createServer((requ , resp)=>
{
if(requ.url =='/')
{
resp.write("nice learning")
}
else if(requ.url =='/about')
{
resp.write('<h1>about page</h1>')
}
resp.end()
})
srvr.listen(5001)
console.log("port number 5001 running ")
Subscribe to my newsletter
Read articles from himanshu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
