Understanding JavaScript Engines and Their Execution
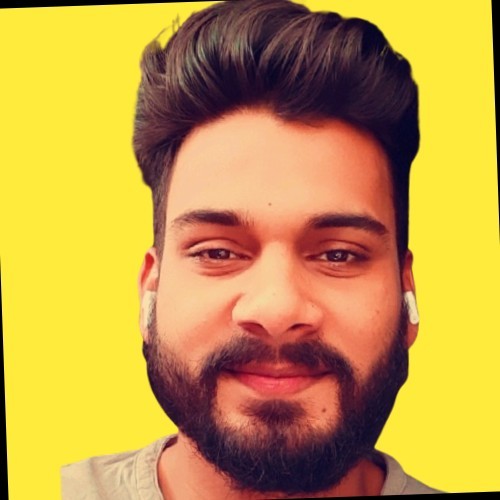
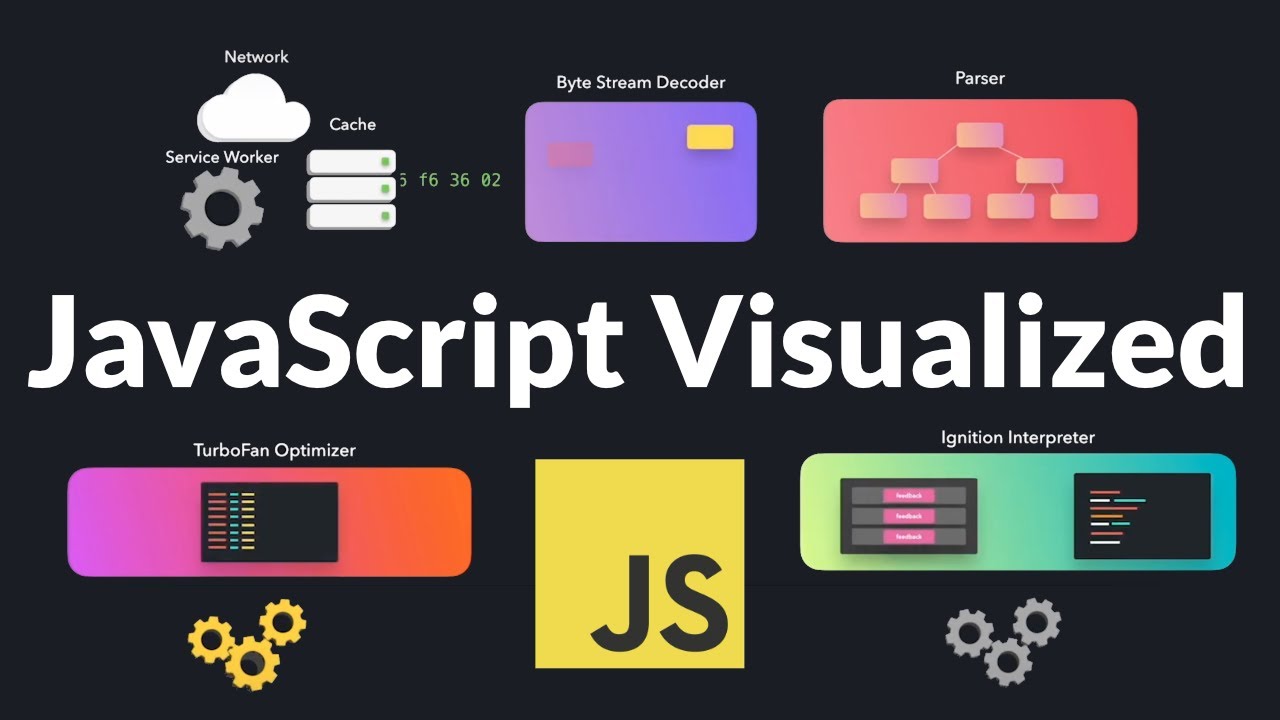
What is a JavaScript Engine?
A JavaScript engine is a virtual machine within a web browser or server that executes JavaScript code. Each browser typically has its own engine:
Google Chrome and Edge: V8
Mozilla Firefox: SpiderMonkey
Safari: JavaScriptCore (also known as Nitro)
Microsoft Edge (Legacy): Chakra
These engines interpret, compile, and execute JavaScript code, transforming it from high-level language to machine-readable instructions.
Architecture of a JavaScript Engine
While specific implementations vary, most modern JavaScript engines share a common architecture comprising several key components:
Parser: The parser reads the JavaScript code and converts it into an Abstract Syntax Tree (AST). This step involves lexical analysis (tokenization) and syntactical analysis.
Interpreter: The interpreter generates bytecode from the AST. Bytecode is an intermediate, low-level representation of the code that is more efficient for execution than raw source code.
JIT Compiler: Just-In-Time (JIT) compilation is a crucial optimization technique. The JIT compiler converts frequently executed bytecode into machine code at runtime, allowing the engine to execute hot code paths much faster.
Garbage Collector: JavaScript engines include a garbage collector to manage memory allocation and deallocation. It automatically frees up memory occupied by objects that are no longer in use, preventing memory leaks.
Runtime: The runtime provides essential built-in functions and APIs, such as handling data types, executing mathematical operations, and managing input/output operations.
Execution Process
The execution of JavaScript code involves several steps, from loading the script to running it within the engine:
Loading: The browser fetches the JavaScript file from the server.
Parsing: The parser converts the script into an AST.
Bytecode Generation: The interpreter generates bytecode from the AST.
Execution: The engine begins executing the bytecode. During this phase, the JIT compiler identifies hot code paths and compiles them into machine code for optimized execution.
Optimization: The engine continually profiles the running code, making optimizations and deoptimizations as needed. This adaptive process ensures that the most frequently executed code runs as efficiently as possible.
Optimization Techniques
Modern JavaScript engines employ several optimization techniques to enhance performance:
Inline Caching: This technique speeds up property access on objects by caching the location of properties after their first access.
Hidden Classes: Engines create hidden classes to optimize object property access. Instead of looking up properties by name, the engine can access them directly via these hidden classes.
Dead Code Elimination: The engine removes code that is never executed, reducing the amount of work during execution.
Type Specialization: By specializing functions for specific data types, the engine can avoid type checks and conversions, leading to faster execution.
Garbage Collection: Advanced garbage collection algorithms, such as generational and incremental garbage collection, improve memory management efficiency.
Challenges and Future Directions
JavaScript engines face several challenges, including:
Complexity of Optimization: Balancing the trade-offs between compilation time and execution speed is complex. Over-optimization can lead to increased memory usage and compilation overhead.
Security: Ensuring the security of the execution environment is paramount, as vulnerabilities in the engine can lead to exploits.
Cross-Browser Compatibility: Differences in engine implementations can lead to inconsistencies in how JavaScript behaves across browsers.
Future directions for JavaScript engines include further optimization of JIT compilation, enhanced memory management techniques, and improved support for WebAssembly, a binary instruction format for faster web applications.
Conclusion
JavaScript engines are sophisticated pieces of software that play a vital role in the execution of JavaScript code. Through a combination of parsing, interpreting, JIT compilation, and various optimization techniques, they ensure that JavaScript runs efficiently and securely. As web applications continue to grow in complexity, the evolution of JavaScript engines will remain crucial for maintaining performance and user experience.
Subscribe to my newsletter
Read articles from Pallav Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
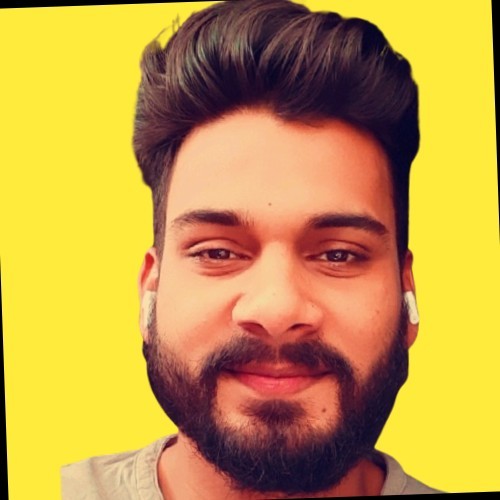
Pallav Singh
Pallav Singh
I am a software developer from India. I'm currently working full-time at an IT Service Company. I'm here to share my journey and my learnings with the community.