How to Run JavaScript Code: A Complete Guide
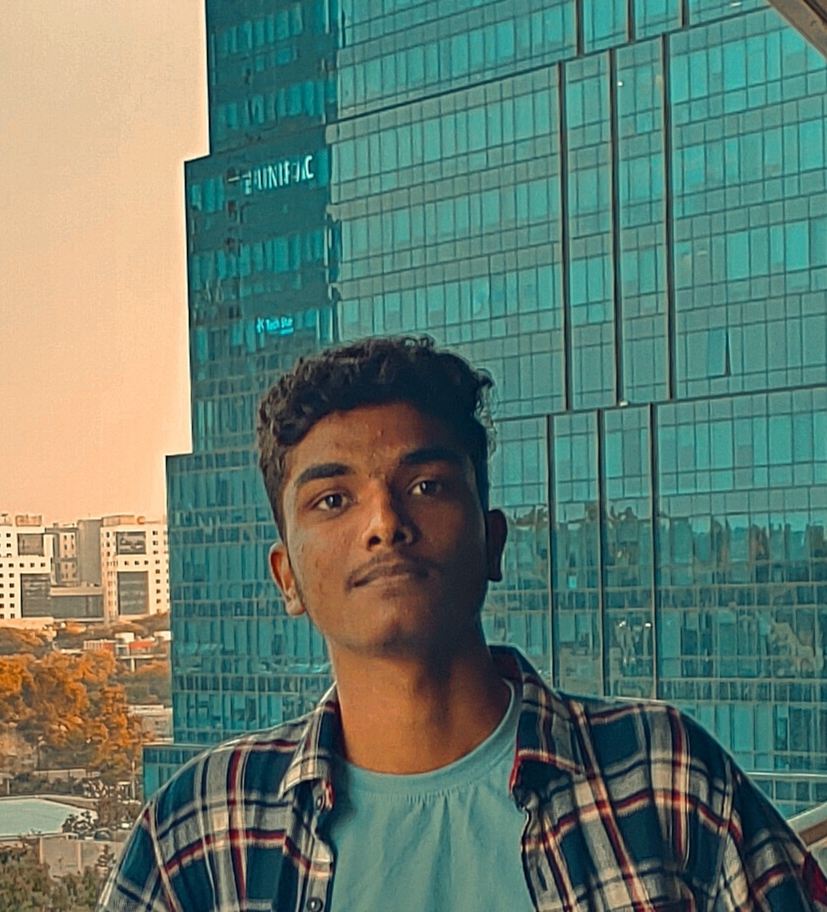

JavaScript is at the core of web development, enabling dynamic and engaging web interactions. Whether you're starting out or aiming to enhance your skills, understanding how to execute JavaScript code is crucial. This guide explores several methods for running JavaScript, from browsers to standalone environments like Node.js and online editors.
Running JavaScript in a Web Browser
One of the most common ways to execute JavaScript is directly within a web browser. Here’s how you can do it:
Using the Browser Console:
Open the Console: In most browsers like Chrome or Firefox, you can open the developer tools by pressing
Ctrl+Shift+J
(Windows/Linux) orCmd+Option+J
(Mac).Try It Out: Once the console is open, you can type JavaScript directly. For example, try typing
console.log("Hello, World!")
and press Enter to see the output.
Embedding JavaScript in HTML:
Create an HTML File: Start by creating a new file named
index.html
.Add JavaScript: Inside the
<body>
section of your HTML, use<script>
tags to include JavaScript. For example:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Running JavaScript in HTML</title> </head> <body> <script> alert("Welcome to JavaScript!"); // Example alert message </script> </body> </html>
View in Browser: Open
index.html
in your browser to see JavaScript in action.
Running JavaScript with Node.js
Node.js allows you to run JavaScript outside of the browser environment, often used for server-side applications. Here’s how to get started:
Install Node.js: Download and install Node.js from nodejs.org.
Create a JavaScript File: Open a text editor and create a file named
hello.js
.Write Your Script: In
hello.js
, write a simple JavaScript program, for example:// hello.js console.log("Hello, Node.js!");
Run Your Script: Open your terminal or command prompt, navigate to the directory containing
hello.js
, and type:node hello.js
You should see
Hello, Node.js!
printed in the terminal.
Using Online JavaScript Editors
If you prefer a hassle-free setup or want to experiment without local installations, online JavaScript editors are a great option:
Choose an Editor: Popular choices include CodePen, JSFiddle, and Replit.
Create a New Project: Start a new project and choose JavaScript as your language.
Write and Run: Write your JavaScript code directly in the editor and run it within the browser-like environment provided.
Best Practices and Tips
Debugging Tools: Use browser developer tools or Node.js debugger (
node inspect
) for troubleshooting.Code Organization: Organize your code into functions and modules for clarity and reusability.
Learning Resources: Explore websites like MDN Web Docs and books such as "Eloquent JavaScript" to deepen your knowledge.
Conclusion
JavaScript forms the backbone of web development, enabling essential functionalities for creating dynamic and interactive web experiences. Whether you're scripting interactions on a webpage, developing robust server-side applications with Node.js, or exploring coding possibilities in online editors, mastering these methods equips you to craft compelling digital interactions.
Call to Action
Try running a simple JavaScript program using one of the methods described. Share your experience or questions in the comments!
Happy coding!
Subscribe to my newsletter
Read articles from Gondi Bharadhwaj Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
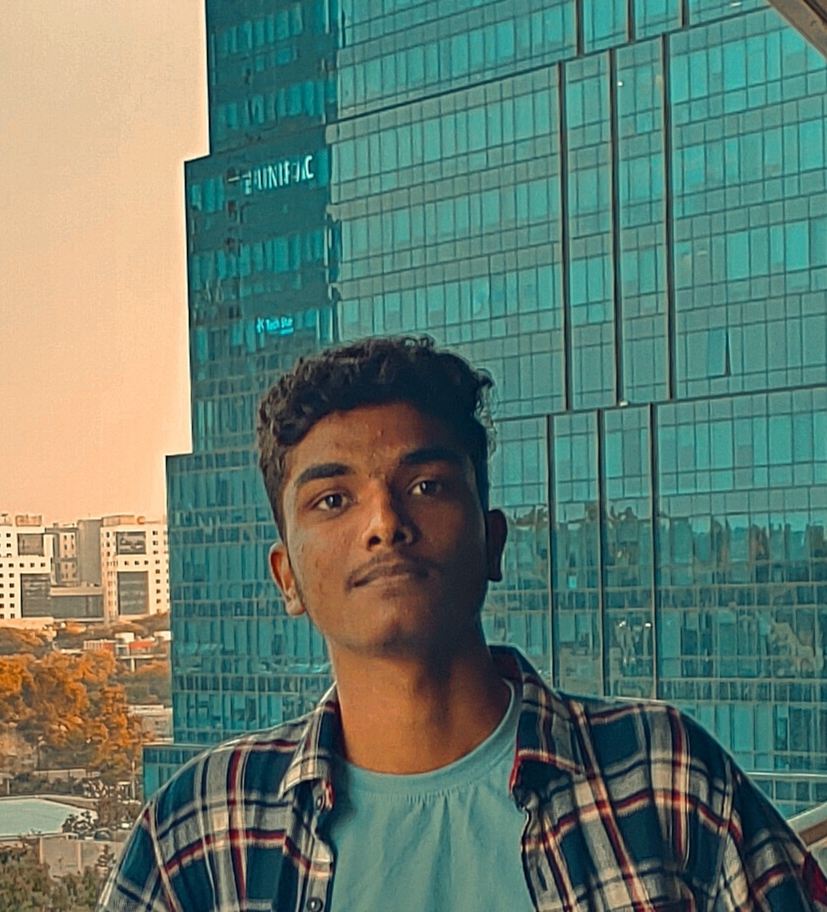
Gondi Bharadhwaj Reddy
Gondi Bharadhwaj Reddy
I am a dedicated undergraduate student passionate about exploring and learning new things. My interests span across various fields, from technology and web development. Currently focused on projects that enhance learning experiences and make meaningful contributions to communities.