Mastering Assembly Language
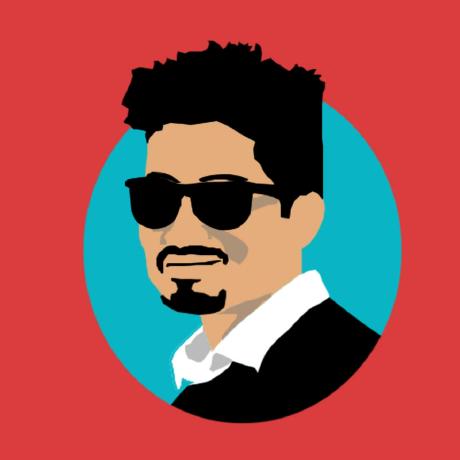
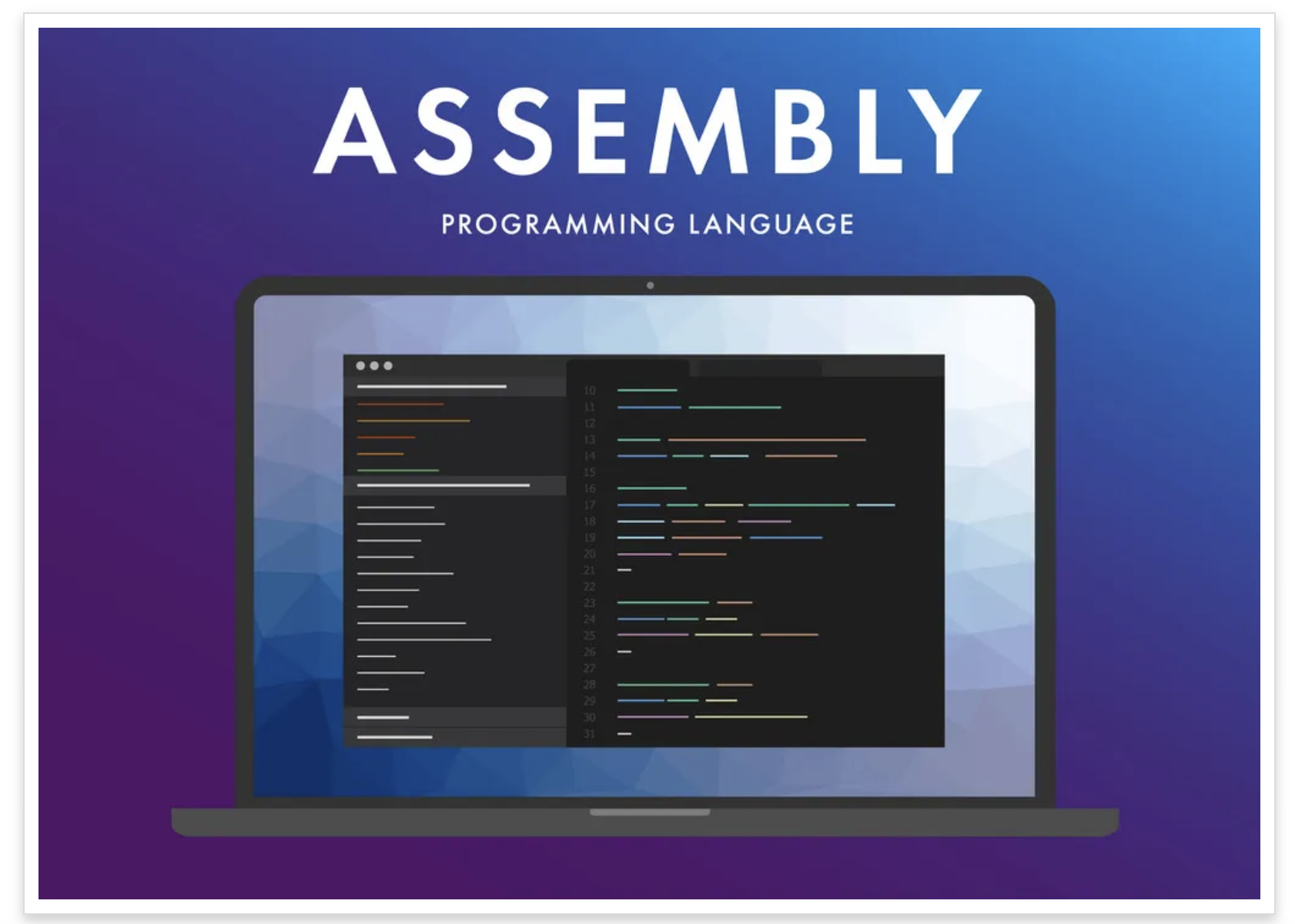
Assembly language, a low-level programming language, offers unparalleled control over computer hardware. This tutorial will guide you through the essentials of assembly language programming, culminating in the creation of a simple operating system kernel. By the end of this guide, you'll understand how to leverage assembly language for performance optimization, debugging, and system-level programming.
Brief History of Assembly Language
Origin and Evolution
Assembly language was developed to provide a more human-readable representation of machine code. Its origins date back to the early days of computing, with the creation of the first assemblers in the 1950s. Assembly language has evolved alongside computer architecture, adapting to new processors and system designs.
Importance in the History of Computing
Assembly language has played a crucial role in the development of software, enabling programmers to write code that interacts directly with hardware. It was essential for early operating systems, compilers, and performance-critical applications. Even today, understanding assembly language is vital for tasks like reverse engineering, systems programming, and performance optimization.
Why Learn Assembly Language Today?
Understanding Computer Architecture
Learning assembly language provides a deep understanding of computer architecture. It helps you grasp how processors execute instructions, manage memory, and handle I/O operations.
Optimizing Performance-Critical Code
Assembly language allows for fine-grained control over the hardware, enabling you to write highly optimized code. This is crucial for performance-critical applications like embedded systems, game development, and real-time computing.
Debugging and Reverse Engineering
Proficiency in assembly language is essential for debugging at the lowest level. It also aids in reverse engineering binaries, understanding malware, and developing security exploits.
Enhancing Problem-Solving Skills
Writing assembly code enhances your problem-solving skills by forcing you to think about resource management, instruction efficiency, and hardware constraints.
Setting Up Your Environment
Choosing an Assembler
NASM (Netwide Assembler): A popular, open-source assembler for x86 architecture, known for its simplicity and wide platform support.
MASM (Microsoft Macro Assembler): A powerful assembler for Windows, offering advanced features and integration with Visual Studio.
GAS (GNU Assembler): Part of the GNU Binutils package, widely used in Unix-like systems and GCC toolchain.
Installing Tools
Installation on Windows
NASM: Download from NASM Official Site and follow the installation instructions.
MASM: Install via Visual Studio by selecting the "Desktop development with C++" workload.
Installation on Mac
NASM: Use Homebrew:
brew install nasm
GAS: Pre-installed with Xcode Command Line Tools.
Installation on Linux
NASM: Use the package manager:
sudo apt-get install nasm
GAS: Pre-installed with GNU Binutils:
sudo apt-get install binutils
Setting Up an Integrated Development Environment (IDE)
Visual Studio Code: A versatile, free code editor with extensions for assembly language.
JetBrains CLion: A powerful IDE for C and assembly development.
Eclipse CDT: An open-source IDE with support for assembly language.
Basic Configuration and Testing
Install the chosen IDE.
Configure build tasks to compile and link assembly code.
Write a simple "Hello World" program to test the setup.
Basic Assembly Language Syntax and Concepts
Registers
Registers are small storage locations within the CPU used to hold data temporarily during execution. Common x86 registers include:
AX, BX, CX, DX: General-purpose registers.
SI, DI: Index registers used for string operations.
SP, BP: Stack pointer and base pointer for stack operations.
IP: Instruction pointer, holds the address of the next instruction.
Instructions
Instructions tell the CPU what operations to perform. Basic instructions include:
MOV: Move data from one location to another.
ADD, SUB: Perform addition and subtraction.
MUL, DIV: Perform multiplication and division.
JMP: Jump to a different part of the program.
CMP: Compare two values.
JE, JNE: Jump if equal, jump if not equal (conditional jumps).
Syntax
The general format of an assembly instruction is:
INSTRUCTION DESTINATION, SOURCE
For example:
MOV AX, 5 ; Move the value 5 into register AX
ADD AX, BX ; Add the value in BX to AX
Labels
Labels are used to mark locations in the code that can be jumped to:
START:
MOV AX, 5
JMP END
END:
HLT
Comments
Comments are denoted by ;
and are used to annotate code:
MOV AX, 5 ; Load 5 into AX
System Calls and Interrupts
System calls allow programs to request services from the operating system. In DOS, INT 0x21 is used for system calls, while in Linux, INT 0x80 is common.
Example of a DOS system call to print a character:
MOV AH, 0x0E ; BIOS teletype output
MOV AL, 'A' ; Character to print
INT 0x10 ; BIOS interrupt
Project: Simple Operating System Kernel
Objective
Develop a minimalistic operating system kernel to understand low-level system programming, boot processes, and hardware management.
Steps
Bootloader Creation
Bootloader.asm:
[BITS 16]
[ORG 0x7C00]
; Print a message
MOV AH, 0x0E
MOV SI, MSG
PRINT_LOOP:
LODSB
CMP AL, 0
JE DONE
INT 0x10
JMP PRINT_LOOP
DONE:
JMP $
MSG DB 'Booting the OS...', 0
TIMES 510 - ($ - $$) DB 0
DW 0xAA55
Assemble the bootloader:
nasm -f bin Bootloader.asm -o Bootloader.bin
Create a bootable image:
dd if=Bootloader.bin of=floppy.img bs=512 count=1
Setting Up the GDT (Global Descriptor Table)
GDT.asm:
[BITS 32]
GDT_START:
GDT_NULL: DD 0, 0
GDT_CODE: DW 0xFFFF, 0x0000, 0x9A00, 0x00CF
GDT_DATA: DW 0xFFFF, 0x0000, 0x9200, 0x00CF
GDT_DESCRIPTOR:
DW GDT_END - GDT_START - 1
DD GDT_START
GDT_END:
Implementing Basic Multitasking and Interrupt Handling
Kernel.asm:
[BITS 32]
[GLOBAL _start]
section .text
_start:
; Initialize GDT
LGDT [GDT_DESCRIPTOR]
; Setup segments
MOV AX, 0x10
MOV DS, AX
MOV ES, AX
MOV FS, AX
MOV GS, AX
MOV SS, AX
MOV ESP, 0x90000
; Enable interrupts
STI
; Infinite loop
JMP $
section .data
; Include GDT
GDT_DESCRIPTOR: TIMES 6 DB 0
GDT: TIMES 24 DB 0
Explanation
Understanding Boot Processes
The bootloader is a small program that loads the operating system kernel into memory. It sets up the initial environment and transitions the processor from real mode to protected mode.
Managing System Resources
Setting up the GDT is crucial for defining memory segments and enabling protected mode. The kernel code initializes the GDT, sets up segment registers, and prepares the stack.
Implementing Low-Level System Functions
The kernel code sets up basic multitasking and interrupt handling. Enabling interrupts allows the CPU to respond to hardware events, while multitasking involves context switching between different tasks.
Advanced Topics
Profiling and Benchmarking
Profiling and benchmarking are essential techniques for identifying performance bottlenecks and optimizing critical paths in your assembly code. Profiling involves measuring the time and resources used by different parts of your program, while benchmarking involves running tests to compare the performance of different code sections or algorithms.
Tools for Profiling and Benchmarking
gprof: A profiling tool for applications compiled with GCC.
perf: A powerful performance analysis tool for Linux.
Intel VTune: A performance analysis and profiling tool from Intel.
Example Project: Profiling and Optimizing a Matrix Multiplication Program
MatrixMultiplication.asm:
section .data
matrix1 dd 1, 2, 3, 4, 5, 6, 7, 8, 9
matrix2 dd 9, 8, 7, 6, 5, 4, 3, 2, 1
result dd 0, 0, 0, 0, 0, 0, 0, 0, 0
section .bss
tmp resd 1
section .text
global _start
_start:
; Initialize registers
mov esi, matrix1
mov edi, matrix2
mov ebx, result
mov ecx, 3 ; Matrix size (3x3)
multiply_matrices:
; Loop over rows of matrix1
mov edx, ecx
row_loop:
; Loop over columns of matrix2
push edx
mov edx, ecx
col_loop:
; Calculate dot product
push edx
push ecx
mov edx, ecx
dot_product_loop:
; Load elements and multiply
mov eax, [esi + edx*4]
imul eax, [edi + edx*4]
; Accumulate result
add [tmp], eax
dec edx
jnz dot_product_loop
pop ecx
; Store result
mov [ebx], eax
add ebx, 4
pop edx
dec edx
jnz col_loop
pop edx
add esi, 3*4 ; Move to the next row in matrix1
dec edx
jnz row_loop
; Exit program
mov eax, 1
xor ebx, ebx
int 0x80
Profiling Steps:
Compile the program:
nasm -f elf32 MatrixMultiplication.asm -o MatrixMultiplication.o
Link the program:
ld -m elf_i386 MatrixMultiplication.o -o MatrixMultiplication
Run the program with
perf
:perf record ./MatrixMultiplication
Analyze the results:
perf report
Writing Performance-Critical Code
Writing performance-critical code involves optimizing for speed and efficiency. This includes minimizing instruction count, reducing memory access latency, and leveraging CPU-specific features like SIMD (Single Instruction, Multiple Data) instructions.
Example Project: Optimizing a String Copy Function
StringCopy.asm:
section .data
source db 'Hello, Assembly!', 0
destination times 20 db 0
section .text
global _start
_start:
mov esi, source
mov edi, destination
; Copy string using SIMD
mov ecx, 4 ; Number of iterations (length / 4)
rep movsd
; Copy remaining bytes
mov ecx, 3 ; Remaining bytes (length % 4)
rep movsb
; Exit program
mov eax, 1
xor ebx, ebx
int 0x80
Optimizations:
SIMD Instructions: Using
movsd
to copy four bytes at a time, leveraging the CPU's ability to handle larger data blocks efficiently.Loop Unrolling: Reducing the overhead of loop control by copying multiple elements per iteration.
Reverse Engineering
Disassembling Binaries
Disassembling binaries involves converting machine code back into assembly code. This process helps analyze and understand the behavior of compiled programs, particularly useful in reverse engineering and security analysis.
Tools for Disassembling
IDA Pro: A commercial disassembler and debugger.
Ghidra: A free and open-source reverse engineering tool developed by the NSA.
Example: Disassembling a Simple Program
SimpleProgram.c:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Steps to Disassemble:
Compile the program:
gcc -o SimpleProgram SimpleProgram.c
Use Ghidra to analyze the binary:
Open Ghidra and create a new project.
Import the binary (
SimpleProgram
) into the project.Use Ghidra’s disassembly view to analyze the assembly code.
Analyzing Malware
Malware analysis involves reverse engineering malicious software to understand its behavior, identify vulnerabilities, and develop countermeasures.
Example: Analyzing a Simple Keylogger
Keylogger.asm:
section .data
log_file db 'keylog.txt', 0
section .bss
key_buffer resb 256
section .text
global _start
_start:
; Open log file
mov eax, 5
mov ebx, log_file
mov ecx, 2 ; O_RDWR
mov edx, 0600 ; Permissions
int 0x80
; Read keystrokes
mov ebx, eax ; File descriptor
mov eax, 3
mov ecx, key_buffer
mov edx, 1
read_loop:
int 0x80
; Write to log file
mov eax, 4
mov ecx, key_buffer
mov edx, 1
int 0x80
jmp read_loop
; Exit program
mov eax, 1
xor ebx, ebx
int 0x80
Analysis Steps:
Disassemble the binary using Ghidra.
Identify the system calls (e.g.,
int 0x80
) to understand file operations.Analyze the control flow to determine how keystrokes are logged.
Interfacing with High-Level Languages
Calling Assembly Code from C/C++
Integrating assembly code with C/C++ allows you to optimize performance-critical sections of your program while maintaining the benefits of high-level language constructs.
Example: Calling Assembly from C
assembly_function.asm:
section .text
global my_asm_function
my_asm_function:
; Example function: add two integers
mov eax, [esp + 4]
add eax, [esp + 8]
ret
main.c:
#include <stdio.h>
extern int my_asm_function(int a, int b);
int main() {
int result = my_asm_function(5, 3);
printf("Result: %d\n", result);
return 0;
}
Steps to Compile and Link:
Assemble the assembly file:
nasm -f elf32 assembly_function.asm -o assembly_function.o
Compile the C file:
gcc -m32 -c main.c -o main.o
Link the objects:
gcc -m32 main.o assembly_function.o -o program
Performance Benefits and Use Cases
Using assembly code can significantly boost performance in various scenarios, such as:
Compute-Intensive Applications: High-performance computing tasks that require efficient use of CPU resources.
Real-Time Systems: Applications with strict timing constraints, such as embedded systems and robotics.
Game Development: Performance-critical sections like graphics rendering and physics simulations.
Cryptography: Implementing cryptographic algorithms that require optimized, secure code execution.
Next Steps
Recommended Resources for Further Learning
- Books: "Programming from the Ground Up" by Jonathan Bartlett, "The Art of Assembly Language" by Randall Hyde
Engage with the community by contributing to open-source projects on GitHub, participating in coding challenges, and sharing your knowledge.
Final Thoughts
Understanding assembly language is invaluable for any software engineer, providing insights into computer architecture and enhancing problem-solving skills. Continuous learning and staying updated with the latest advancements will ensure you remain proficient in this foundational programming skill.
Appendices
Useful Resources
- Books: "Programming from the Ground Up," "The Art of Assembly Language"
Common Assembly Language Reference
Instructions: MOV, ADD, SUB, JMP, CALL, RET
Registers: AX, BX, CX, DX, SI, DI, SP, BP
System Calls: INT 0x80 (Linux), INT 0x21 (DOS)
Macros: EQU, %DEFINE
Glossary
Assembler: A program that converts assembly language into machine code.
Opcode: The portion of a machine language instruction that specifies the operation to be performed.
Register: A small amount of fast storage available to the CPU for temporary data.
Interrupt: A signal to the processor indicating an event that needs immediate attention.
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
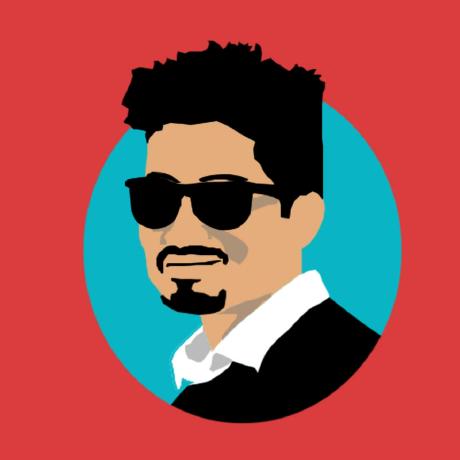