The Comprehensive Guide to Git: Tools and Commands
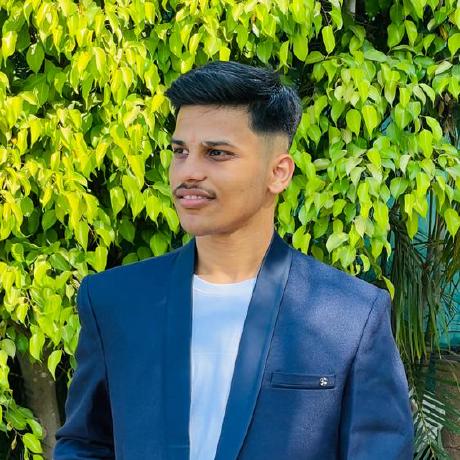
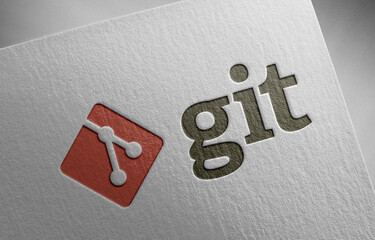
Introduction
Git is a powerful and widely used version control system that allows developers to track changes in their code, collaborate with others, and manage multiple versions of their projects efficiently.
Getting Started with Git
Installation
Before using Git, you need to install it on your system. You can download Git from git-scm.com. Installation instructions are available for different operating systems (Windows, macOS, Linux).
Configuration
After installing Git, you should configure your username and email address, which will be used for your commits.
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
You can verify your configuration with:
git config --list
Basic Git Commands
Initializing a Repository
To start tracking a project with Git, you need to initialize a repository.
git init
This command creates a .git
directory in your project folder, which contains all the metadata and history of your project.
Cloning a Repository
If you want to contribute to an existing project, you can clone its repository.
git clone <repository-url>
This command copies the repository from the URL to your local machine.
Working with Git
Checking the Status
To see the status of your working directory and staging area, use:
git status
This command shows which files are modified, staged, or untracked.
Adding Changes
To stage changes for the next commit, use:
git add <file>
You can add all changes with:
git add .
Committing Changes
To save your staged changes to the repository, use:
git commit -m "Your commit message"
This command records a snapshot of your project’s current state.
Viewing the Commit History
To see the commit history of your repository, use:
git log
This command shows a list of all commits with their messages, authors, and timestamps.
Branching and Merging
Creating a Branch
Branches allow you to work on different parts of a project simultaneously. To create a new branch, use:
git branch <branch-name>
Switching Branches
To switch to a different branch, use:
git checkout <branch-name>
Merging Branches
To merge changes from one branch into another, switch to the target branch and use:
git merge <branch-name>
Deleting a Branch
After merging a branch, you can delete it with:
git branch -d <branch-name>
Remote Repositories
Adding a Remote
To add a remote repository, use:
git remote add <remote-name> <repository-url>
Fetching Changes
To fetch changes from a remote repository without merging, use:
git fetch <remote-name>
Pulling Changes
To fetch and merge changes from a remote repository, use:
git pull <remote-name> <branch-name>
Pushing Changes
To push your local commits to a remote repository, use:
git push <remote-name> <branch-name>
Undoing Changes
Reverting a Commit
To create a new commit that undoes changes from a previous commit, use:
git revert <commit-id>
Resetting to a Previous Commit
To reset your repository to a previous state, use:
git reset --hard <commit-id>
Rebase
Understanding Rebase
The git rebase
command is a powerful tool that helps you integrate changes from one branch into another. Unlike git merge
, which creates a new commit that ties the histories of branches together, git rebase
moves or combines a sequence of commits to a new base commit. This can create a cleaner, more linear project history.
Basic Rebase Usage
To rebase the current branch onto another branch, use:
git rebase <branch-name>
For example, to rebase your feature branch onto the main branch:
git checkout main
git rebase feature
Interactive Rebase
Interactive rebase allows you to modify commits in various ways (edit, reword, squash) before applying them. Start an interactive rebase with:
git rebase -i <commit-id>
During the interactive rebase, you will see a list of commits that you can modify as needed.
Handling Rebase Conflicts
Rebasing can sometimes result in conflicts. If you encounter a conflict during a rebase:
Resolve the conflicts in the files.
Stage the resolved files with
git add
.Continue the rebase with
git rebase --continue
.
If you need to abort the rebase, use:
git rebase --abort
Important Considerations
Rewriting History: Rebasing rewrites commit history. Never rebase commits that have been pushed to a shared repository, as it can cause issues for other collaborators.
Cleaner History: Rebase can create a cleaner, more understandable project history compared to merge.
Additional Tools and Commands
Stashing Changes
To temporarily save changes without committing, use:
git stash
To apply stashed changes, use:
git stash apply
Viewing Differences
To see the differences between your working directory and the staging area, use:
git diff
To see the differences between commits, use:
git diff <commit-id> <commit-id>
Tagging Commits
To tag a commit with a specific version, use:
git tag <tag-name>
To push tags to a remote repository, use:
git push <remote-name> --tags
Conclusion
Git is an essential tool for modern software development. Understanding its commands and how they work allows you to manage your projects efficiently, collaborate with others, and maintain a clean and organized codebase.
Subscribe to my newsletter
Read articles from Darshan Atkari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
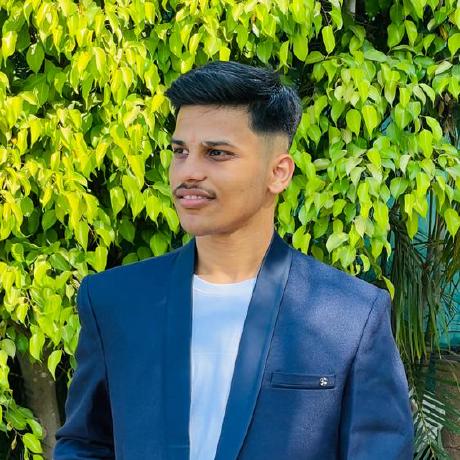
Darshan Atkari
Darshan Atkari
Hey there! I'm Darshan Atkari, a dedicated Computer Engineering student. I thoroughly enjoy documenting my learning experiences. Come along on my exciting journey through the world of technology!