React JS - Props and Events
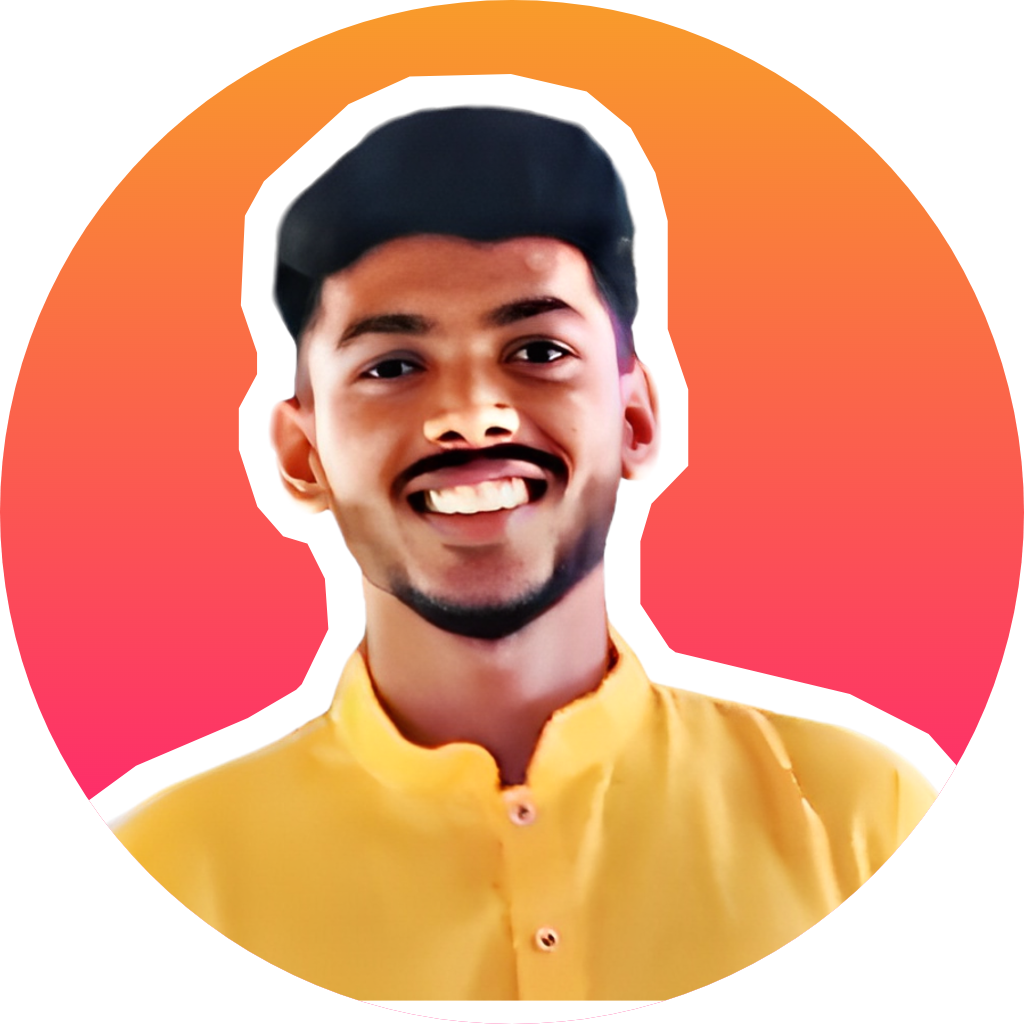
Table of contents
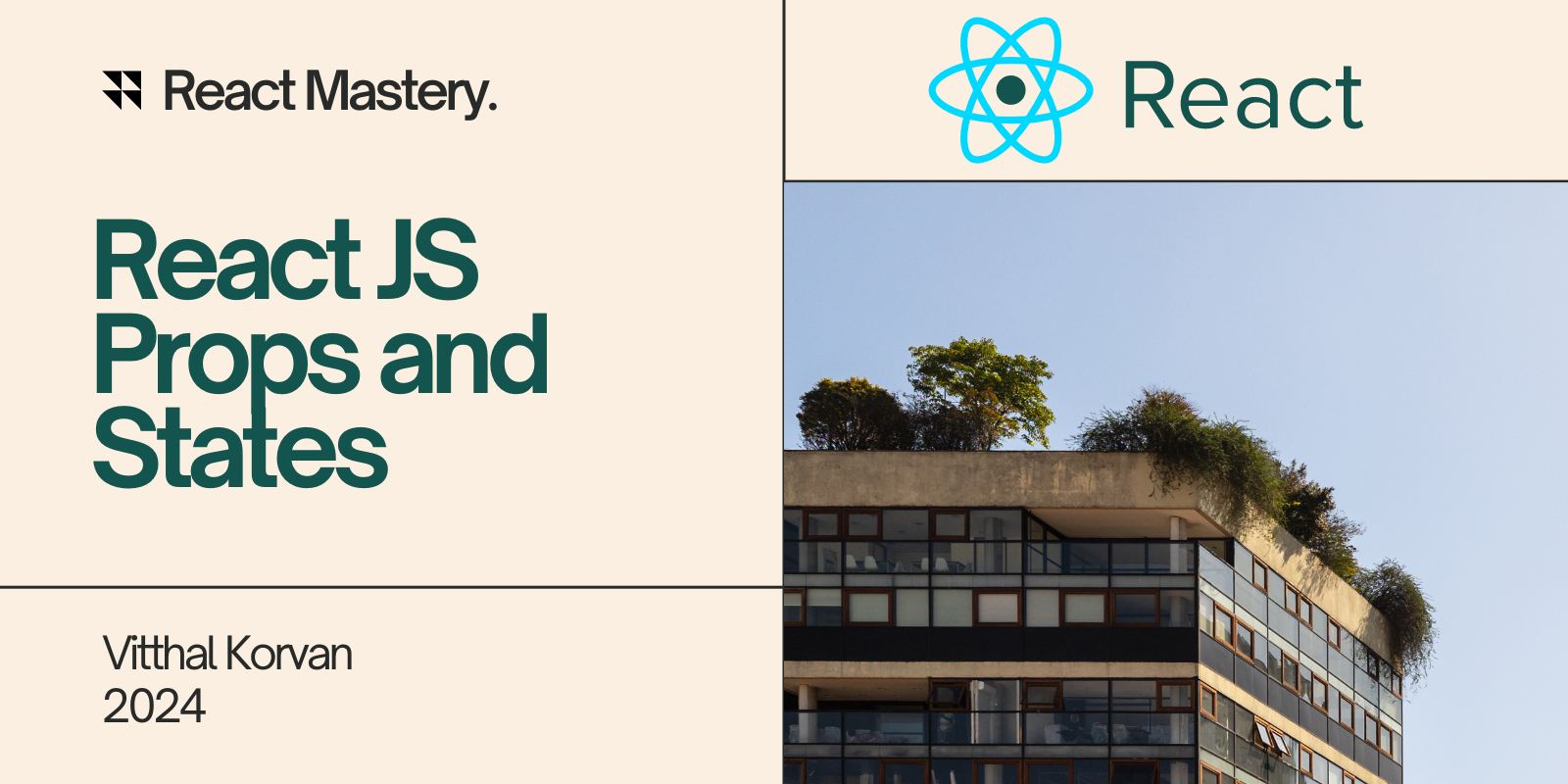
Props
Props (short for properties) are used in React to pass data from one component to another, usually from a parent component to a child component. Props are immutable, meaning they cannot be changed by the receiving component. Instead, they are meant to provide a way to configure or customize a component.
Props are used to pass data from parent to child components.
Props are immutable, meaning they cannot be modified by the receiving component.
They help create dynamic and reusable components.
You can define default values for props and validate them using
defaultProps
andPropTypes
.The
children
prop allows you to pass components as nested elements.
Passing Props to a Component
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
return (
<ChildComponent name="Vitthal" age={24} />
);
}
export default ParentComponent;
// ChildComponent.js
import React from 'react';
function ChildComponent(props) {
return (
<div>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
}
export default ChildComponent;
Destructuring Props
To make the code cleaner and more readable, you can use object destructuring to extract the properties from the props object. Here’s how you can do it:
// ChildComponent.js
import React from 'react';
function ChildComponent({ name, age }) {
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
</div>
);
}
export default ChildComponent;
we can pass anything String, number, array and objects to the props.
Default Props
You can define default values for props in case they are not provided by the parent component. This can be done using the defaultProps
property:
// ChildComponent.js
import React from 'react';
function ChildComponent({ name, age }) {
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
</div>
);
}
ChildComponent.defaultProps = {
name: 'Anonymous',
age: 0
};
export default ChildComponent;
Children Props
The children
prop is automatically passed to components without needing to explicitly define it. Any content placed between the opening and closing tags of a component will be available via the children
prop.
import { Greetings } from "./components/Greetings";
function App() {
return (
<>
<Greetings firstName="Vitthal" lastName="korvan">
<h2> Hey! User Welcome </h2>
</Greetings>
</>
);
}
export default App;
export function Greetings({children, firstName, lastName}) {
return <>
<h2>Hey! {firstName} {lastName}</h2>
{children}
</>;
}
Render Lists
In React, rendering a list of items is a common pattern, usually achieved by mapping over an array of data and returning JSX elements for each item. This is particularly useful for rendering dynamic data, such as arrays retrieved from an API.
Steps to Render a List in React
Prepare the Data:
- Typically, the data comes from an array. Each item in the array will be mapped to a JSX element.
Use
map()
to Iterate Over the Array:- The
map()
function in JavaScript is used to iterate over the array and return JSX for each item.
- The
Add a Unique
key
Prop:- React requires each element in a list to have a unique
key
prop. This helps React identify which items have changed, added, or removed. Thekey
should ideally be a unique identifier like anid
from the data.
- React requires each element in a list to have a unique
import React from "react";
function User({lastName, name}) {
return (
<>
<h1>
{name} {lastName}
</h1>
</>
);
}
export default User;
import User from "./component/User"
function App() {
const user = [
{id:'1', name:'vitthal', lastName:'korvan'},
{id:'2', name:'anand', lastName:'korvan'},
{id:'3', name:'lingesh', lastName:'korvan'}
]
return (
<>
{/* <User name={user[0].name} lastName={user[0].lastName} />
<User name={user[1].name} lastName={user[1].lastName} />
<User name={user[2].name} lastName={user[2].lastName} /> */}
{
// user.map((user)=>{
// return <User name={user.name} lastName={user.lastName} />;
// })
}
{/* {
user.map((user)=>(
<User name={user.name} lastName={user.lastName} />
))
} */}
{/*user.map((user) => (
<User {...user} />
))*/}
{user.map((user) => (
<User {...user} key={user.id} />
))}
</>
);
}
export default App
Key Prop
The key
prop in React is crucial for efficiently updating lists by uniquely identifying elements. It ensures stable identity, preserves state, and prevents UI bugs. Use unique, consistent keys (not array indices) for each item to optimize rendering and maintain predictable behavior during re-renders.
if you're using static list then using index as key is good, but when you have dynamic list then using index as key will not work. when we delete or update the list then index element will change.
const items = [
{ id: 1, name: 'Apple' },
{ id: 2, name: 'Banana' },
{ id: 3, name: 'Cherry' }
];
const ItemList = () => {
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
};
Events
events are actions or occurrences that happen in the browser, such as clicking a button, typing into a text box, or submitting a form. React provides a way to handle these events using event handlers, which are functions that execute in response to these events.
Key Concepts About Events in React
React Synthetic Events:
React uses its own event system called Synthetic Events, which is a cross-browser wrapper around the browser’s native events. This ensures consistent behavior across different browsers.
These synthetic events are very similar to native DOM events but provide additional capabilities, such as automatic event delegation and performance optimizations.
Event Naming in CamelCase:
React events use camelCase instead of lowercase as in HTML.
Example: In HTML, you would use
onclick
, but in React, you would useonClick
.
Passing Event Handlers:
Event handlers are passed as props to JSX elements in React.
Example: Passing a function to handle a
click
event:function handleClick() { alert('Button clicked!'); } function App() { return <button onClick={handleClick}>Click Me</button>; }
Event Objects:
In React, event handlers receive an event object as an argument. This object contains information about the event, such as the target element, mouse position, key pressed, etc.
Example: Using the event object to access the clicked element:
function handleClick(event) { console.log('Element clicked:', event.target); } function App() { return <button onClick={handleClick}>Click Me</button>; }
Common Events in React
Mouse Events:
onClick
: Triggered when an element is clicked.onDoubleClick
: Triggered when an element is double-clicked.onMouseEnter
: Triggered when the mouse enters an element.onMouseLeave
: Triggered when the mouse leaves an element.Example:
function handleMouseEnter() { console.log('Mouse entered'); } function App() { return <div onMouseEnter={handleMouseEnter}>Hover over me!</div>; }
Form Events:
onChange
: Triggered when the value of an input field changes (useful for input elements).onSubmit
: Triggered when a form is submitted.Example:
function handleInputChange(event) { console.log('Input value:', event.target.value); } function App() { return <input type="text" onChange={handleInputChange} />; }
Example of form submission:
function handleSubmit(event) { event.preventDefault(); // Prevents page refresh console.log('Form submitted'); } function App() { return ( <form onSubmit={handleSubmit}> <input type="text" /> <button type="submit">Submit</button> </form> ); }
Keyboard Events:
onKeyDown
: Triggered when a key is pressed.onKeyUp
: Triggered when a key is released.onKeyPress
: Triggered when a key is pressed, but is generally less preferred since it doesn't handle non-character keys likeonKeyDown
andonKeyUp
.Example:
function handleKeyPress(event) { console.log('Key pressed:', event.key); } function App() { return <input type="text" onKeyDown={handleKeyPress} />; }
Focus Events:
onFocus
: Triggered when an element gains focus.onBlur
: Triggered when an element loses focus.Example:
function handleFocus() { console.log('Input focused'); } function App() { return <input type="text" onFocus={handleFocus} />; }
Touch Events (for mobile devices):
onTouchStart
: Triggered when the user starts touching the screen.onTouchMove
: Triggered when the user moves their finger on the screen.onTouchEnd
: Triggered when the user stops touching the screen.Example:
function handleTouchStart() { console.log('Touch started'); } function App() { return <div onTouchStart={handleTouchStart}>Touch me!</div>; }
Clipboard Events:
onCopy
: Triggered when the user copies content.onPaste
: Triggered when the user pastes content.Example:
function handleCopy() { console.log('Text copied'); } function App() { return <input type="text" onCopy={handleCopy} />; }
Passing Arguments to Event Handlers
Sometimes you may want to pass additional arguments to your event handlers. You can achieve this by using arrow functions or wrapping the handler in another function.
Example with arrow function:
function handleClick(name) { alert(`Hello, ${name}`); } function App() { return <button onClick={() => handleClick('Vitthal')}> Click Me </button>; }
Example with function wrapping:
function handleClick(name) { alert(`Hello, ${name}`); } function App() { return <button onClick={handleClick.bind(null, 'Vitthal')}> Click Me </button>; }
Preventing Default Behavior
React allows you to prevent the default behavior of certain elements, such as form submissions or anchor tags, using event.preventDefault()
.
Example with form:
function handleSubmit(event) { event.preventDefault(); // Prevent page refresh console.log('Form submitted'); } function App() { return ( <form onSubmit={handleSubmit}> <input type="text" /> <button type="submit">Submit</button> </form> ); }
Example with anchor tag:
function handleClick(event) { event.preventDefault(); // Prevent link navigation console.log('Link clicked'); } function App() { return <a href="https://www.example.com" onClick={handleClick}> Click Here</a>; }
Event Propagation and stopPropagation()
By default, events propagate (or "bubble") up the DOM tree, triggering event handlers on parent elements. You can stop this propagation using event.stopPropagation()
.
Example:
function handleParentClick() { console.log('Parent clicked'); } function handleChildClick(event) { event.stopPropagation(); // Prevents the parent event handler from running console.log('Child clicked'); } function App() { return ( <div onClick={handleParentClick}> <button onClick={handleChildClick}>Click Me</button> </div> ); }
GitHub Code - Props and Events
Subscribe to my newsletter
Read articles from Vitthal Korvan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
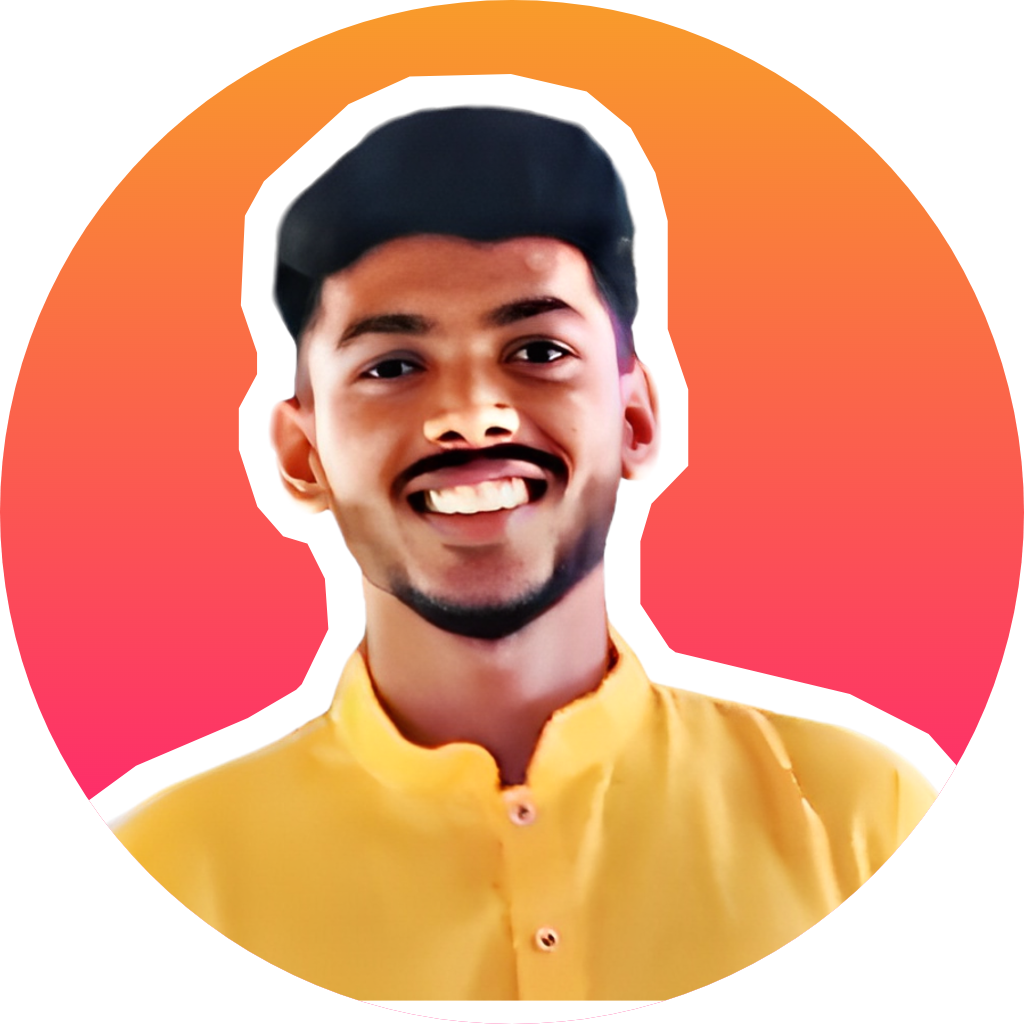
Vitthal Korvan
Vitthal Korvan
🚀 Hello, World! I'm Vitthal Korvan 🚀 As a passionate front-end web developer, I transform digital landscapes into captivating experiences. you'll find me exploring the intersection of technology and art, sipping on a cup of coffee, or contributing to the open-source community. Life is an adventure, and I bring that spirit to everything I do.