Mocking APIs: How to Simulate Backend Responses for Testing
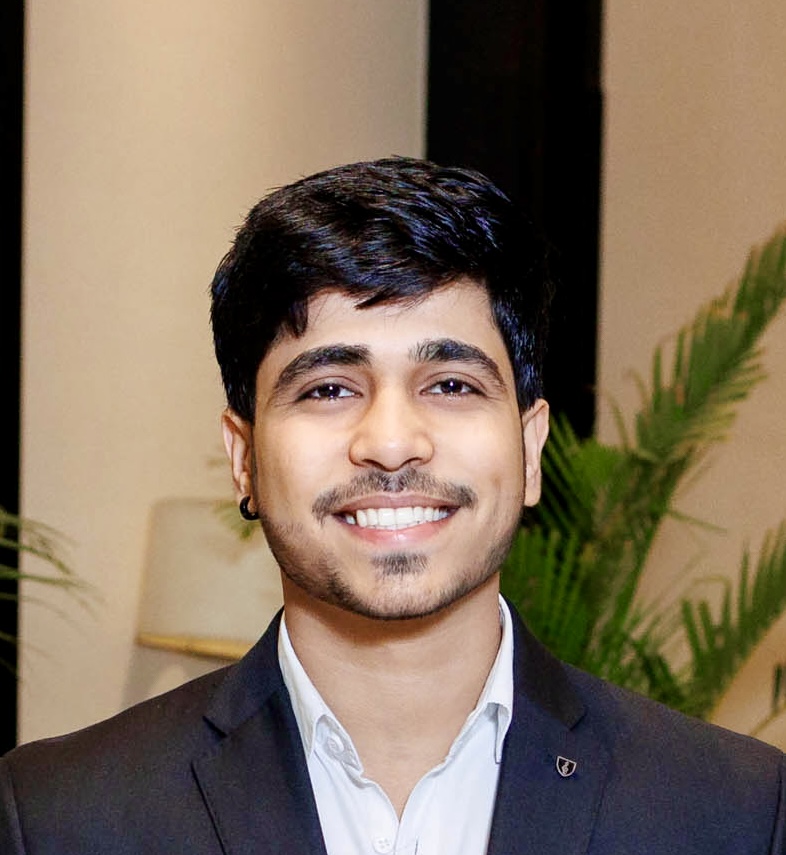
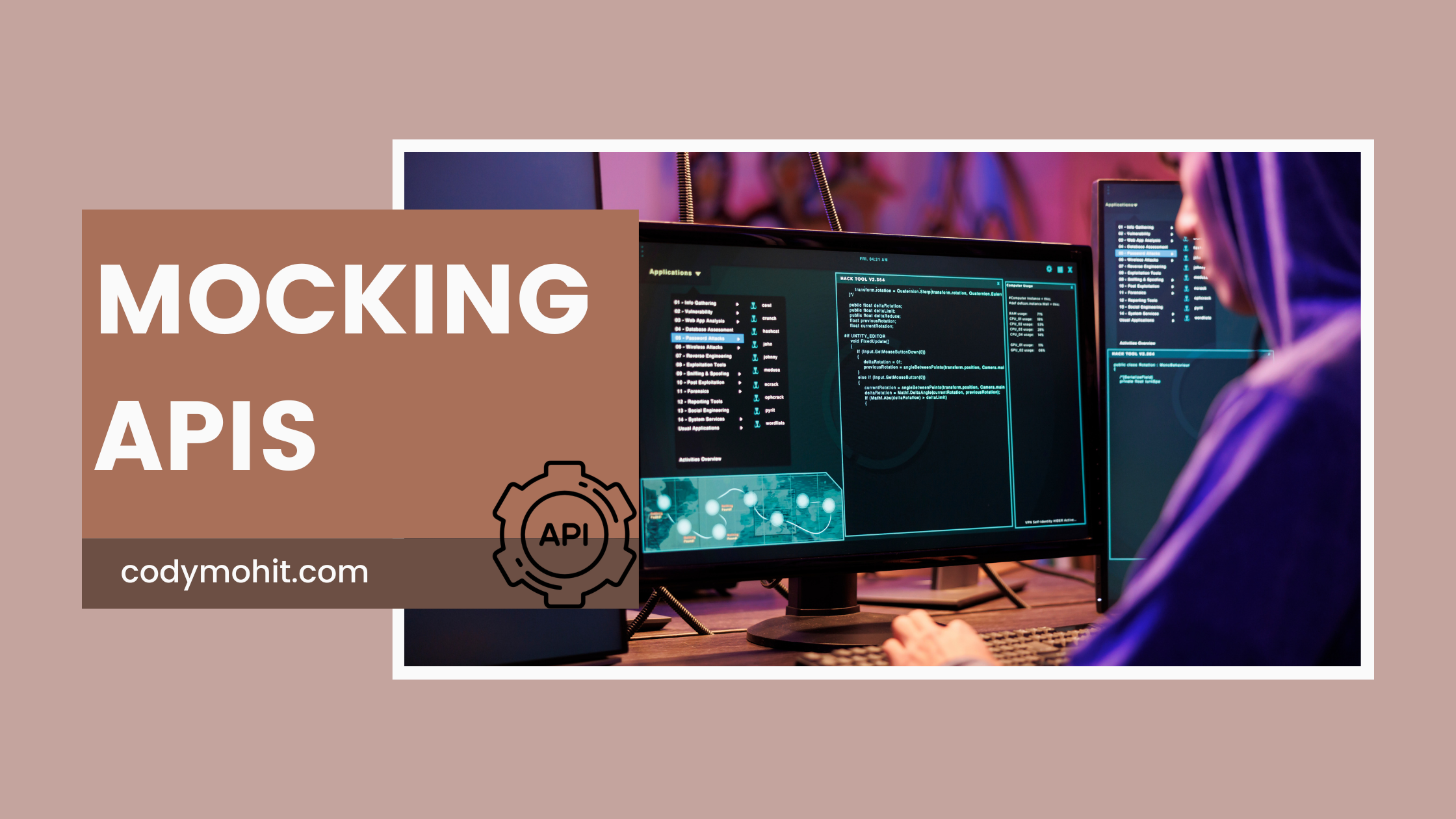
Ever got stuck trying to test your app coz the backend aint ready? Or maybe the API you're depending on keeps acting up? Trust me, we've all been there. In this blog, we're diving into the world of mocking APIs – yep, you heard it right! We’ll uncover the secrets of simulating backend responses for testing, making your life a whole lot easier. Mocking APIs isn't just a fancy term; it's a game changer for developers who want smooth, uninterrupted testing. So, buckle up and let’s explore how you can mock APIs like a pro and say goodbye to those pesky testing woes.
Ready to make your testing process super smooth? Let's jump right in!
Why Mock APIs?
Mock APIs are like practice runs before the real game. Imagine you’re building a new app that talks to a weather service to show the forecast. What if the weather service is not ready yet, or it's too expensive to keep calling it while you're still developing your app? That's where mock APIs come in. They act like stand-ins, giving you fake weather data so you can keep building and testing your app without any delays.
Benefits of API Mocking
Faster Development: You don’t have to wait for other teams to finish their APIs. You can keep working with the mock version and switch to the real one later.
Cost-Effective: Some real APIs charge you for each request. By using mocks, you save money because you’re not hitting the real service all the time.
Consistent Testing: Mock APIs give you the same response every time. This makes it easier to test your app and find bugs, because the data doesn't keep changing.
Isolation: When testing, you want to make sure your app works by itself. Mock APIs isolate your app from external factors, ensuring you’re testing only your code.
Common Use Cases for API Mocking
Early Development: When you’re just starting out and the real API isn’t ready yet, mock APIs let you move forward without waiting.
Automated Testing: In automated tests, you want predictable results. Mock APIs provide the same responses every time, which is crucial for reliable tests.
Integration Testing: To see how well your app integrates with others, you can use mocks to simulate the other apps’ behavior. This helps you catch issues early.
Performance Testing: When checking how well your app handles a lot of traffic, you can use mock APIs to simulate high loads without stressing the real services.
Tools and Frameworks for API Mocking
Overview of Popular API Mocking Tools
API mocking tools are essential for developers who want to test their applications without relying on real APIs. They simulate the behavior of a real API, allowing developers to work in isolation and test various scenarios, including edge cases and failures. Here’s a brief overview of some popular API mocking tools:
Postman
- Postman is a widely-used tool for API development. It offers a robust mocking service that allows developers to create mock servers and define custom responses. It's user-friendly and integrates well with the rest of the Postman suite.
WireMock
- WireMock is a flexible library for stubbing and mocking web services. It's highly configurable and can simulate various types of responses, including latency and error conditions. It's particularly popular in Java environments.
Mockoon
- Mockoon is an open-source tool that allows developers to create mock APIs quickly. It features a simple, intuitive interface and supports exporting and importing mock configurations. It's perfect for local development and testing.
Prism
- Prism is a powerful HTTP mock server from Stoplight that validates requests against an OpenAPI specification. It ensures that your mock responses adhere to the API schema, making it great for contract testing.
Beeceptor
- Beeceptor is a lightweight tool that allows you to mock REST APIs with minimal setup. You can create endpoints and configure responses easily through a web interface. It's excellent for quick prototyping and testing.
Comparison of Top API Mocking Frameworks
When it comes to choosing the right API mocking tool, it depends on your specific needs and the ecosystem you are working in. Here’s a comparison of some top frameworks based on key criteria:
Ease of Use
Postman: Very user-friendly, with a GUI that makes it easy to create and manage mocks. Ideal for developers who prefer a graphical interface.
WireMock: Requires more configuration and code, making it better suited for developers comfortable with scripting and command-line tools.
Mockoon: Extremely easy to use with its drag-and-drop interface. Perfect for quick setup and local testing.
Feature Set
WireMock: Offers advanced features like proxying, response templating, and request matching. It's very powerful but can be complex to set up.
Prism: Focuses on strict adherence to API contracts, ensuring that your mocks are always in sync with your OpenAPI specs.
Beeceptor: Simple and straightforward, with fewer advanced features but very easy to use for basic mocking needs.
Integration
Postman: Integrates seamlessly with other Postman features like testing, documentation, and monitoring. Great for an all-in-one solution.
WireMock: Can be integrated into various CI/CD pipelines and works well with other Java-based tools.
Mockoon: Limited integration options but can be exported and used in different environments.
Performance
WireMock: Highly performant and can handle a large number of requests, making it suitable for load testing.
Postman: Performance is decent for development and testing purposes but might not be the best choice for high-load scenarios.
Mockoon: Best for local development with moderate request volumes.
Community and Support
Postman: Large community and extensive documentation, plus active support from the developers.
WireMock: Good documentation and community support, with many resources available for troubleshooting.
Mockoon: Growing community and active development, with frequent updates and improvements.
In conclusion, choosing the right API mocking tool depends on your specific needs and preferences. For ease of use and integration, Postman is a great choice. If you need advanced features and are comfortable with more configuration, WireMock is ideal. For quick and simple mocking, Mockoon and Beeceptor are excellent options.
By understanding the strengths and limitations of each tool, you can make an informed decision that best fits your development workflow.
Using Mock Servers
Mock servers are a great way to simulate real servers, helping you test your applications without needing the actual backend. Let's dive into setting up a mock server and configuring mock responses.
Setting Up a Mock Server
Choose Your Tool: There are many tools available for setting up mock servers. Some popular ones are Postman, WireMock, and Mockoon. Pick one that fits your needs.
Install the Tool: Download and install the tool. For example, if you choose Postman:
Go to the Postman website.
Download the application for your operating system.
Install it following the instructions.
Create a New Mock Server:
Open your tool. In Postman, click on the "New" button and select "Mock Server".
Give your mock server a name. This helps you remember what the server is for.
Define the URL for your mock server. This can be anything, like
https://my-mock-server.com
.
Add Endpoints:
Define the endpoints your application will hit. For example,
GET /users
orPOST /login
.Make sure to list all the endpoints you need to mock.
Configuring Mock Responses
Create Mock Responses:
For each endpoint, you need to create responses that your mock server will return.
In Postman, select the endpoint and click on "Add Response".
Define the status code (like 200 for success or 404 for not found).
Write the body of the response. This is what your application will receive.
Set Headers:
Sometimes, you might need to set headers for your responses. Headers provide additional information about the response.
In Postman, you can add headers by going to the "Headers" tab and adding key-value pairs.
Save and Test:
Save your mock responses.
Test your mock server by sending requests to the endpoints. Make sure your application behaves as expected with the mock data.
Adjust as Needed:
Based on your tests, you might need to adjust the responses.
Update the response body, headers, or status codes to match what your application expects.
A Few Things to Remember:
Use Realistic Data: Make your mock responses as realistic as possible. This helps in finding potential issues early.
Keep it Updated: As your application evolves, update your mock server to reflect changes.
Test Different Scenarios: Create different responses for various scenarios, like success, error, and edge cases.
Using mock servers is an essential skill for developers. It helps in testing and developing applications more efficiently. So, go ahead and set up your mock server today! Happy coding!
Mocking with Postman
Mocking APIs in Postman is a great way to simulate endpoints and test your applications without relying on the actual backend. Let's dive into how to create and manage mock servers and responses in Postman.
Creating Mock Servers in Postman
Creating a mock server in Postman is pretty straightforward. Here’s how you can do it:
Open Postman: First, open the Postman application.
Create a Collection: Create a new collection if you don't already have one. You can do this by clicking on the "New" button and selecting "Collection".
Add a Request: Add a new request to your collection. You can do this by clicking on the "Add Request" button within your collection.
Set Up the Request: Configure your request by setting the method (GET, POST, etc.) and the endpoint URL.
Save the Request: Save your request with a meaningful name.
Mock the Server: Now, click on the three dots next to your request and select "Mock Request". Follow the prompts to set up your mock server. Postman will generate a unique mock server URL for you.
Managing Mock Responses in Postman
Once you have your mock server set up, you can start managing your mock responses. Here’s how you do it:
Open the Mock Server: Go to the "Mock Servers" tab on the left panel in Postman. You will see a list of all your mock servers.
Select Your Mock Server: Click on the mock server you want to manage.
Add Responses: Click on the request you want to manage responses for. You can add multiple responses with different status codes and bodies.
Edit Responses: Click on "Edit" to modify an existing response. You can change the status code, response body, headers, and more.
Save Changes: After making your changes, make sure to save them.
Quick Tips to Remember
Use Variables: Utilize variables in your mock responses to make them more dynamic and flexible.
Test Thoroughly: Always test your mock responses to ensure they behave as expected.
Documentation: Document your mock endpoints clearly so that your team members can easily understand them.
By mocking APIs in Postman, you can speed up your development process and ensure that your frontend can handle various responses. It’s a powerful feature that every developer should know how to use.
Mocking with WireMock
WireMock is a powerful tool for mocking HTTP services. It allows developers to create a fake server that mimics the behavior of real APIs. This can be extremely helpful for testing purposes, as it lets you simulate different scenarios and responses without relying on the actual external services.
Think of WireMock as a way to create a virtual API that you can control entirely. You can define how it should respond to different requests, making it perfect for testing how your application handles various API responses, errors, and delays. WireMock is widely used because it's easy to set up and provides a lot of flexibility.
Creating and Managing Mocks with WireMock
Using WireMock involves a few simple steps to get started:
Setup WireMock: First, you'll need to add WireMock to your project. If you're using Maven, you can include it as a dependency like this:
xmlCopy code<dependency> <groupId>com.github.tomakehurst</groupId> <artifactId>wiremock-jre8-standalone</artifactId> <version>2.31.0</version> </dependency>
This makes WireMock available in your project so you can start creating mocks.
Starting WireMock Server: Next, you'll start the WireMock server. This can be done with just a few lines of code:
javaCopy codeimport com.github.tomakehurst.wiremock.WireMockServer; import static com.github.tomakehurst.wiremock.core.WireMockConfiguration.wireMockConfig; WireMockServer wireMockServer = new WireMockServer(wireMockConfig().port(8080)); wireMockServer.start();
This code initializes a WireMock server running on port 8080.
Creating Mocks: Now comes the fun part—creating mocks. You can define what responses should be returned for specific requests. For example, to mock a GET request to
/api/data
, you can do:javaCopy codeimport static com.github.tomakehurst.wiremock.client.WireMock.*; wireMockServer.stubFor(get(urlEqualTo("/api/data")) .willReturn(aResponse() .withStatus(200) .withHeader("Content-Type", "application/json") .withBody("{ \"message\": \"Hello, world!\" }")));
This tells WireMock to return a JSON response with a "Hello, world!" message whenever a GET request is made to
/api/data
.Managing Mocks: WireMock also allows you to manage mocks dynamically. You can update, delete, or even verify that certain requests were made. For instance, to verify a request, you might use:
javaCopy codeverify(getRequestedFor(urlEqualTo("/api/data")));
This checks if a GET request to
/api/data
was made during your tests.
WireMock provides an extensive API to mock different HTTP methods, set response delays, and simulate faults. This flexibility makes it an invaluable tool for testing how your application interacts with external services.
By using WireMock, you can ensure that your tests are not dependent on external APIs' availability or behavior. This results in more reliable and faster test executions.
Remember, always double-check your mocks and ensure they represent realistic scenarios that your application might encounter. This will help you catch edge cases and improve your application's resilience.
Simulating Different Response Scenarios
When testing your backend APIs, it's crucial to simulate various response scenarios to ensure your application can handle different situations gracefully. Here are some key scenarios to consider:
Mocking Success and Error Responses
Mocking Success Responses
What it is: This involves creating fake (mock) responses that simulate successful API calls. This allows you to test how your application behaves when everything works as expected.
How to do it: You can use tools like Postman or frameworks like Mockito (for Java) or unittest.mock (for Python) to create these mock responses. For instance, in Postman, you can set up a mock server that returns predefined success responses for your API endpoints.
Why it's important: It ensures that your application can correctly handle and display the data it receives from successful API calls.
Mocking Error Responses
What it is: This involves creating fake error responses to test how your application handles different error scenarios, like 404 Not Found, 500 Internal Server Error, or network errors.
How to do it: Similar to mocking success responses, you can use tools like Postman to set up mock servers that return error responses. You can also use testing frameworks to simulate these errors in your code.
Why it's important: It helps you verify that your application can gracefully handle errors and provide useful feedback to the user, rather than crashing or showing confusing error messages.
Mocking Delays and Timeouts
Mocking Delays
What it is: This involves simulating a delay in the API response to test how your application handles slow network conditions.
How to do it: You can use tools like Postman to add a delay to your mock responses. In code, you can use functions like
time.sleep
in Python to simulate delays.Why it's important: It helps you ensure that your application remains responsive and user-friendly even when the network is slow. For example, you might want to show a loading spinner or a message indicating that the data is being fetched.
Mocking Timeouts
What it is: This involves simulating a situation where the API request times out due to a slow network or server issue.
How to do it: In testing frameworks, you can configure your mock server or API call to timeout after a certain period. For example, in Python, you can use the
requests
library's timeout parameter to simulate a timeout.Why it's important: It ensures that your application can handle timeouts appropriately, such as by retrying the request, showing an error message, or suggesting the user check their internet connection.
By mocking success and error responses, as well as delays and timeouts, you can create a more robust application that gracefully handles a variety of real-world scenarios. Remember, the goal is to ensure your application can handle the unexpected, keeping your users happy even when things go wrong. This will make your application more reliable and provide a better user experience overall.
Mocking for Different Environments
Mocking APIs is a crucial practice in software development, especially when working with backend services. It helps developers test their code without relying on actual API endpoints, which might not always be available. Here's a simple guide to understanding how to mock APIs for different environments: development, testing, and production.
Mocking APIs for Development, Testing, and Production
Development: During development, you might not have access to all the real APIs. Mocking allows you to simulate the API responses, so you can focus on building and testing your application's logic. It's like having a stand-in actor while the main actor is unavailable.
- Example: You are developing a weather app, but the weather API is down. By mocking, you can create fake weather data to continue building your app.
Testing: In testing, mocking is used to isolate the components being tested. This ensures that tests are reliable and not dependent on external services that may be slow or unstable. It also allows for testing edge cases that might be hard to reproduce with a real API.
- Example: You're testing an app's response to various error codes from an API. Mocking these responses helps you see how your app handles different scenarios without actually needing the API to fail.
Production: Mocking in production is less common but still useful. It can be used in A/B testing or for temporarily replacing a faulty service. This way, your app remains operational while the real service is being fixed.
- Example: If a payment service is down, you can mock the service to ensure users can still navigate your app while displaying a message that payments are temporarily unavailable.
Strategies for Environment-Specific Mocks
Configuration Files: Use different configuration files for each environment. These files can specify which mocks to use. For instance,
config.dev.json
for development andconfig.test.json
for testing. This way, switching environments is as simple as changing the configuration file.Environment Variables: Leverage environment variables to determine which mocks to use. This approach is dynamic and allows you to change the behavior of your app without modifying the code. For example,
process.env.NODE_ENV
can be set todevelopment
,testing
, orproduction
, and your app can load the appropriate mocks accordingly.Mocking Libraries: Use mocking libraries like MockServer, WireMock, or JSON Server to create and manage mocks. These tools can help you set up robust mocking solutions that can be easily configured for different environments.
Conditional Statements: Implement conditional statements in your code to check the environment and apply the correct mocks. Although this approach can be simple, it might make your codebase cluttered if not managed properly.
Example:
pythonCopy codeif environment == 'development':
mock_api_response = {'weather': 'sunny'}
elif environment == 'testing':
mock_api_response = {'weather': 'rainy'}
else:
mock_api_response = call_real_api()
Mocking APIs for different environments ensures that your development, testing, and production processes run smoothly. By using configuration files, environment variables, mocking libraries, and conditional statements, you can create a flexible and reliable mocking strategy tailored to each environment. Remember, the goal is to make your app resilient and ready for any situation, even if the real APIs aren't cooperating.
Integrating Mocking in CI/CD Pipelines
Importance of Mocking in Continuous Integration and Deployment
Mocking is like a secret weapon for developers working on Continuous Integration (CI) and Continuous Deployment (CD) pipelines. It allows you to create "fake" versions of components in your system so you can test your code without needing the actual services to be available. Imagine you have a microservice that depends on a payment gateway. When running your tests, you don't want to make real payment transactions (it could get expensive and messy!). Instead, you mock the payment gateway to simulate its behavior.
Here are a few reasons why mocking is so important:
Isolation: By mocking dependencies, you can isolate the part of the system you want to test. This means you can focus on testing one thing at a time without worrying about the rest of the system.
Speed: Mocking helps speed up tests because you don’t have to wait for real services to respond. This makes your CI/CD pipeline faster.
Reliability: Tests become more reliable since they don't depend on external services that might be down or slow. Your tests will give the same results every time.
Cost-Effective: Mocking external services means you avoid costs related to using third-party APIs during testing.
Security: You don't expose sensitive data or make potentially risky operations during tests.
Setting Up Mocking in CI/CD Workflows
Setting up mocking in CI/CD workflows can seem tricky, but it's pretty straightforward once you get the hang of it. Here’s a step-by-step guide to help you out:
Choose Your Mocking Tool: First, pick a mocking tool that suits your needs. There are plenty to choose from, like Mockito for Java, unittest.mock for Python, or WireMock for HTTP-based APIs.
Write Your Mock Services: Create mock versions of the services your application depends on. For example, if your app communicates with a REST API, you can use WireMock to create a mock server that returns predefined responses.
pythonCopy code# Example in Python using unittest.mock from unittest.mock import Mock mock_payment_gateway = Mock() mock_payment_gateway.process_payment.return_value = {"status": "success", "transaction_id": "12345"}
Integrate Mocks into Your Tests: Modify your test cases to use the mocks instead of the real services. This often involves dependency injection or configuration changes.
pythonCopy codedef test_payment(): payment_service = PaymentService(payment_gateway=mock_payment_gateway) response = payment_service.process_payment(amount=100) assert response["status"] == "success"
Configure Your CI/CD Pipeline: Ensure your CI/CD pipeline knows about your mocks. This might involve setting environment variables, adding setup scripts, or configuring your testing framework to use mocks.
yamlCopy code# Example in a CI/CD configuration file steps: - name: Run Tests run: | export MOCK_SERVER_URL=http://mock-server pytest tests/
Run Your Pipeline: Finally, run your CI/CD pipeline. Your tests should now use the mock services, making them faster, more reliable, and more cost-effective.
Mocking can make your CI/CD workflows more efficient and robust. It's like giving your tests a magic wand that makes dependencies behave just the way you want. So, give it a try and watch your testing process transform!
Note: In the real world, mocking isn't always perfect. Sometimes, you'll need to test with real services too, especially in staging environments, to catch any integration issues that might not be visible with mocks alone.
Designing Effective Mocks
Tips for Creating Realistic Mock Responses
Understand the Real API: Before creating a mock, understand the real API's responses. Look at the structure, data types, and common response patterns. This helps make your mocks realistic.
Include Various Scenarios: Don’t just mock successful responses. Include error cases, edge cases, and different status codes. This ensures your application handles all possible situations.
Use Realistic Data: Use data that looks real. Avoid placeholders like “test” or “123”. Use names, addresses, and values that mimic real-world data.
Keep Consistent with Actual Responses: Make sure your mock responses have the same fields and data types as the actual API. This prevents issues when you switch from mock to real API.
Automate Mock Generation: Use tools like Postman or Swagger to automatically generate mock responses based on the API schema. This saves time and ensures accuracy.
Avoiding Common Pitfalls in API Mocking
Ignoring Error Cases: One common mistake is only focusing on successful responses. Ignoring error cases can lead to untested paths in your application. Always include error responses in your mocks.
Inconsistent Data: Ensure your mock data is consistent. Inconsistent data can cause unexpected behaviors and make debugging difficult.
Over-Simplification: Don’t oversimplify your mocks. While it’s tempting to use basic responses, doing so can miss out on important edge cases. Strive for a balance between simplicity and realism.
Not Updating Mocks: As your API evolves, update your mocks. Using outdated mocks can lead to discrepancies and bugs when integrating with the real API.
Ignoring Performance: Mock servers can be fast, but real APIs might be slower. Include realistic delays in your mocks to mimic real-world performance.
By following these tips and avoiding common pitfalls, you can design effective mocks that help you build robust and reliable applications. Remember, the goal of mocking is to simulate the real API as closely as possible, without the dependency on the actual service.
Maintaining and Updating Mocks
Strategies for Keeping Mocks Up-to-Date
Keeping your mocks up-to-date is crucial for accurate testing. If your mocks are outdated, your tests might pass even when the real API is failing. Here are some simple strategies to ensure your mocks stay current:
Automate Mock Updates: Use tools that automatically update your mocks when the API changes. This reduces manual work and errors.
Regular Reviews: Schedule regular reviews of your mocks to make sure they match the current API specifications. This could be a part of your sprint reviews.
Version Control: Keep your mocks in version control systems like Git. This allows you to track changes and rollback if needed.
Sync with API Documentation: Always sync your mocks with the latest API documentation. If the docs change, your mocks should too.
Use Dynamic Mocks: Use tools that can create dynamic mocks based on API schemas. This way, mocks update automatically when the schema changes.
Handling Changes in API Specifications
API specifications can change frequently, and handling these changes properly is essential. Here's how you can manage it:
Notification Systems: Set up a notification system to alert your team whenever there’s a change in the API specs. This could be an email alert or a notification in your project management tool.
Automated Tests: Implement automated tests that check if your mocks align with the current API specs. These tests should run as part of your CI/CD pipeline.
Collaborate with API Developers: Maintain close communication with the API developers. If they make changes, they should inform you, so you can update your mocks accordingly.
Fallback Mechanisms: Have fallback mechanisms in place. If an API changes suddenly, your system should be able to handle it gracefully without breaking.
Detailed Documentation: Keep detailed documentation of the API changes and how your mocks should be updated in response. This helps new team members understand the process quickly.
Remember, keeping mocks up-to-date and handling API changes effectively ensures your tests remain reliable and your application functions correctly. Happy testing!
Frequently Asked Questions
What is API mocking and why is it important?
API mocking is the process of creating a fake version of an API that mimics the behavior of a real one. This mock API is used to simulate the responses and interactions you'd expect from the actual API without needing the real backend to be up and running. This is crucial for several reasons:
Development Speed: Developers can start building and testing their applications without waiting for the backend to be completed.
Isolation: It allows developers to isolate the frontend and backend development processes, making it easier to detect and fix issues.
Consistency: Mock APIs can provide consistent responses, which is helpful for automated testing and debugging.
Cost-Efficiency: Using a mock API can reduce the cost associated with accessing external APIs, especially when there are rate limits or usage charges.
What are the most popular tools for mocking APIs?
There are several popular tools for mocking APIs, each with its own set of features:
Postman: Widely known for API testing, Postman also provides powerful mocking capabilities.
WireMock: A flexible tool that can simulate HTTP-based APIs with customizable responses.
Mockoon: A desktop application for quickly creating mock servers.
JSON Server: A simple way to create a mock REST API using a JSON file as a data source.
Beeceptor: A web-based tool that allows for easy creation and management of mock APIs.
Prism: Part of the Stoplight suite, Prism can mock APIs based on OpenAPI specifications.
How do I set up a mock server?
Setting up a mock server can vary depending on the tool you choose, but here’s a general approach using Postman:
Create a Mock Server: In Postman, go to the 'Mock Server' option and create a new mock server.
Define Endpoints: Specify the endpoints and the expected responses. You can set different responses for different HTTP methods (GET, POST, etc.).
Save Examples: For each endpoint, save example responses that the mock server will return.
Integrate: Use the mock server URL in your application as you would use the real API endpoint.
What are the differences between static and dynamic mocking?
Static Mocking:
Fixed Responses: Static mocking involves pre-defined, unchanging responses to API requests.
Simpler Setup: It’s generally easier to set up and understand, making it ideal for basic testing.
Limited Flexibility: Since responses are fixed, it’s not suitable for scenarios requiring varied or conditional responses.
Dynamic Mocking:
Variable Responses: Dynamic mocking can generate different responses based on input parameters or state.
More Complex: It requires a more sophisticated setup and may involve scripting or more advanced configurations.
Greater Flexibility: Suitable for testing more complex interactions and behaviors, such as authentication flows or state-dependent responses.
Can I use Postman to mock APIs?
Yes, you can use Postman to mock APIs, and it’s quite straightforward:
Create Mock Server: In Postman, navigate to the 'Mock Server' section and create a new mock server.
Set Up Endpoints: Define the endpoints you want to mock and save example responses.
Configure Responses: For each endpoint, you can create multiple examples to cover different scenarios.
Access Mock Server URL: Postman provides a unique URL for your mock server, which you can use in your application for development and testing.
Using Postman for API mocking allows you to simulate different responses, test your application’s behavior, and ensure it handles various scenarios effectively.
Subscribe to my newsletter
Read articles from Mohit Bhatt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
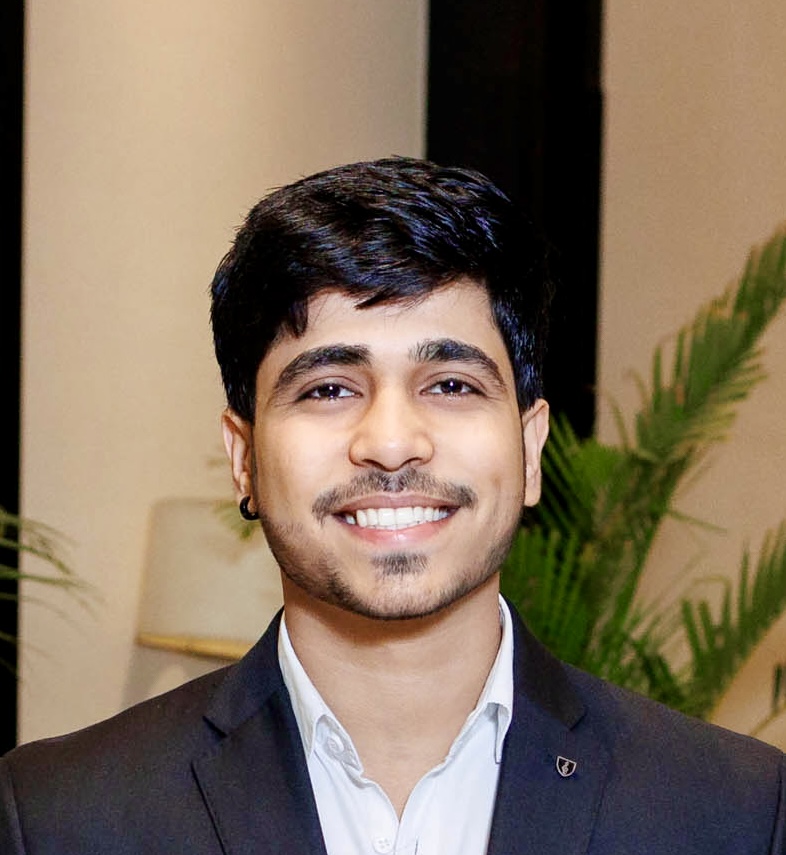
Mohit Bhatt
Mohit Bhatt
As a dedicated and skilled Python developer, I bring a robust background in software development and a passion for creating efficient, scalable, and maintainable code. With extensive experience in web development, Rest APIs., I have a proven track record of delivering high-quality solutions that meet client needs and drive business success. My expertise spans various frameworks and libraries, like Flask allowing me to tackle diverse challenges and contribute to innovative projects. Committed to continuous learning and staying current with industry trends, I thrive in collaborative environments where I can leverage my technical skills to build impactful software.