Code Optimization Techniques: Balancing Readability and Performance in TypeScript
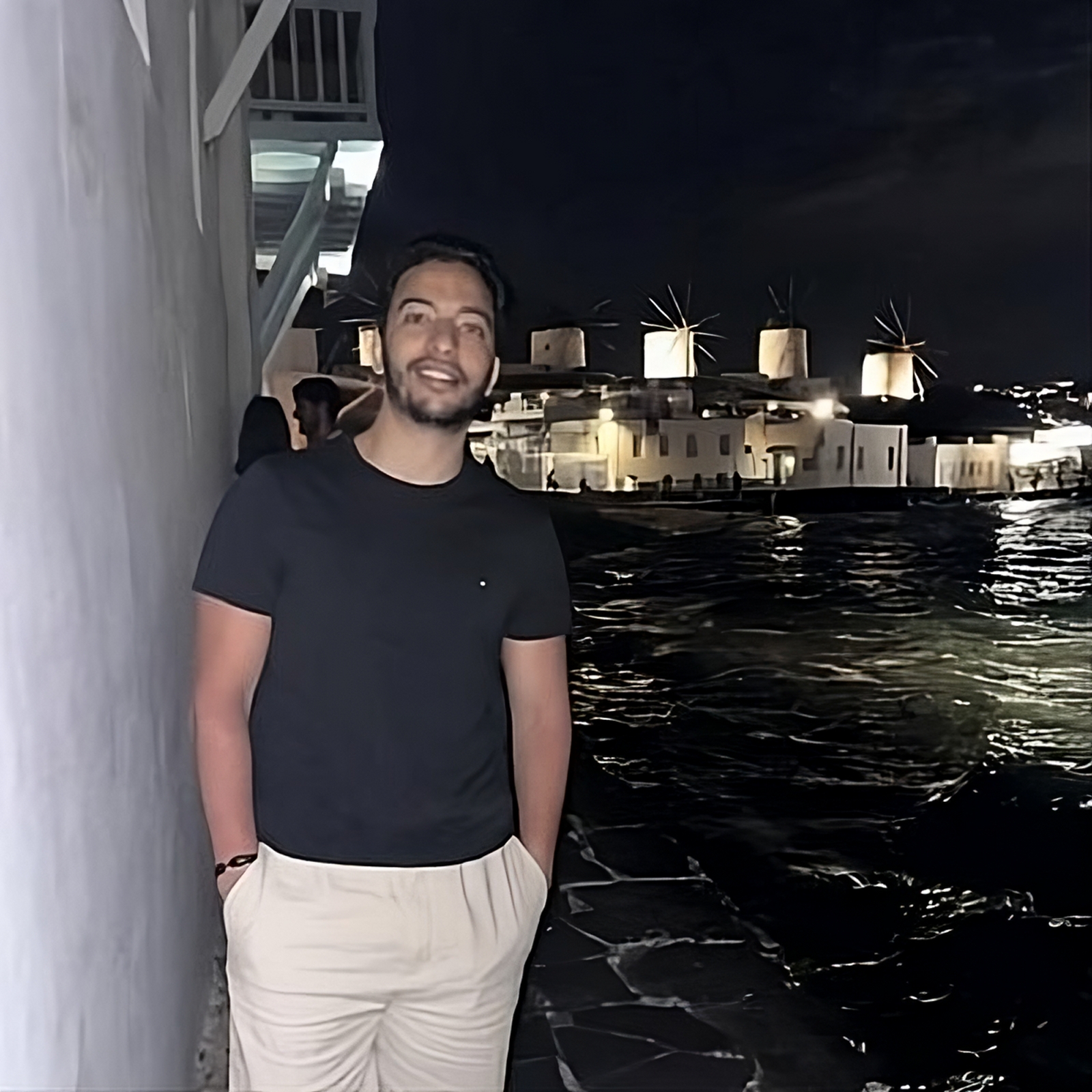
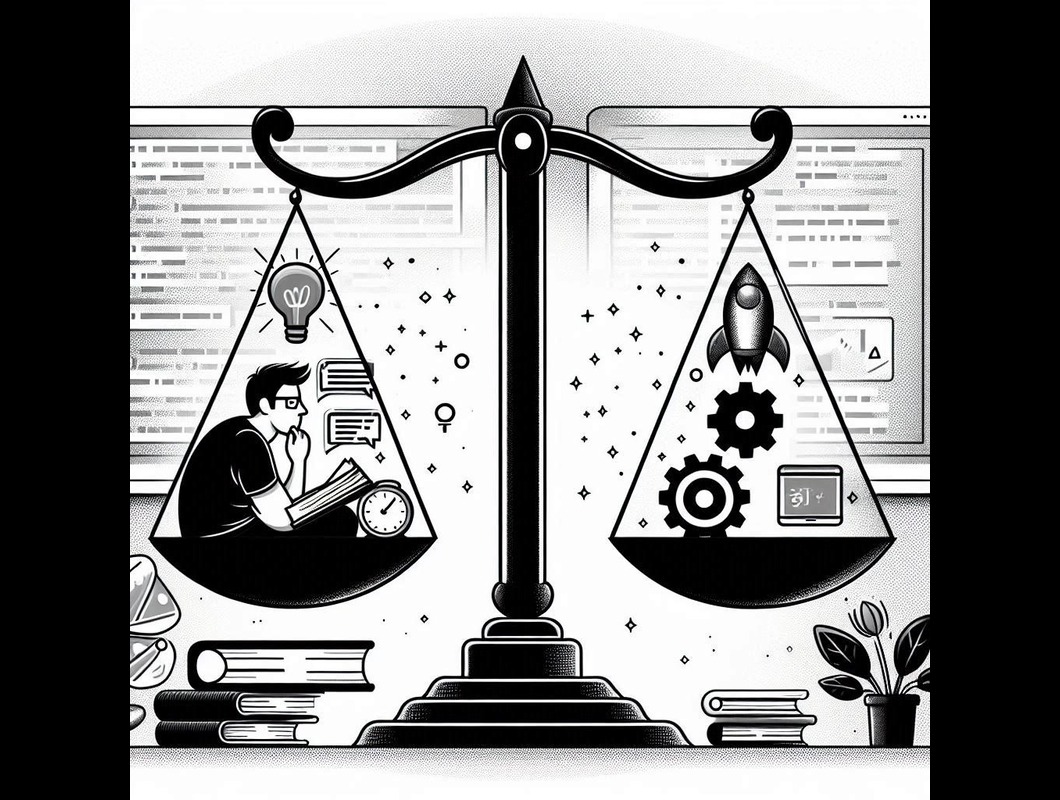
In the world of software development, writing code that is both efficient and easy to understand is a constant challenge. This is especially true when working with TypeScript, a statically typed superset of JavaScript that adds optional types to the language. While TypeScript offers many benefits in terms of code quality and maintainability, it's important to strike a balance between writing readable code and optimizing for performance.
In this article, we'll explore various code optimization techniques for TypeScript, discussing how to improve performance without sacrificing readability. We'll cover a range of topics, from basic optimizations to more advanced techniques, always keeping in mind the importance of maintaining clear and understandable code.
Understanding the Balance
Before diving into specific techniques, it's crucial to understand the balance between readability and performance. Readable code is easier to maintain, debug, and extend, which can save time and reduce errors in the long run. On the other hand, performant code ensures that your application runs efficiently, providing a better user experience and potentially reducing resource usage.
The key is to find the sweet spot where your code is both readable and performant. In many cases, you can achieve both goals simultaneously, but sometimes you may need to make trade-offs. When you do, it's important to document your decisions and explain the reasoning behind any optimizations that might make the code less immediately understandable.
Basic Optimization Techniques
Let's start with some basic optimization techniques that can improve performance without significantly impacting readability.
1. Use Appropriate Data Structures
Choosing the right data structure for your task can have a significant impact on performance. For example, using a Set instead of an Array for checking the existence of elements can be much faster for large collections:
// Less efficient for large collections
const array: number[] = [1, 2, 3, 4, 5];
const exists = array.includes(3); // O(n) time complexity
// More efficient for large collections
const set: Set<number> = new Set([1, 2, 3, 4, 5]);
const exists = set.has(3); // O(1) time complexity
2. Avoid Unnecessary Computations
Look for opportunities to avoid redundant calculations. For example, you can cache the length of an array if you're using it multiple times in a loop:
// Less efficient
for (let i = 0; i < array.length; i++) {
// Do something
}
// More efficient
const length = array.length;
for (let i = 0; i < length; i++) {
// Do something
}
3. Use TypeScript's Type System
Leverage TypeScript's type system to catch errors at compile-time and provide better editor support. This can lead to fewer runtime errors and more efficient code:
// Less safe and potentially less performant
function add(a: any, b: any) {
return a + b;
}
// More safe and potentially more performant
function add(a: number, b: number): number {
return a + b;
}
4. Use Lazy Evaluation
Lazy evaluation can improve performance by delaying the evaluation of an expression until its value is needed. This can be particularly useful for expensive computations:
// Eager evaluation
const expensiveValue = computeExpensiveValue();
if (condition) {
use(expensiveValue);
}
// Lazy evaluation
let expensiveValue: number | undefined;
if (condition) {
expensiveValue = computeExpensiveValue();
use(expensiveValue);
}
5. Optimize Loops
When working with loops, there are several optimizations you can apply:
a. Use for...of
for arrays and for...in
for object properties:
// Less efficient for arrays
for (let i = 0; i < array.length; i++) {
const item = array[i];
// Do something with item
}
// More efficient for arrays
for (const item of array) {
// Do something with item
}
b. Avoid modifying arrays while iterating over them. Instead, use filter
or reduce
:
// Less efficient and potentially error-prone
for (let i = 0; i < array.length; i++) {
if (someCondition(array[i])) {
array.splice(i, 1);
i--;
}
}
// More efficient and safer
array = array.filter(item => !someCondition(item));
6. Use Memoization for Expensive Functions
Memoization is a technique where you cache the results of expensive function calls and return the cached result when the same inputs occur again:
memoize<T extends (...args: any[]) => any>(fn: T): T {
const cache = new Map();
return ((...args: any[]) => {
const key = JSON.stringify(args);
if (cache.has(key)) {
return cache.get(key);
}
const result = fn(...args);
cache.set(key, result);
return result;
}) as T;
}
const expensiveFunction = memoize((a: number, b: number) => {
// Expensive computation here
return a + b;
});
7. Use TypeScript's Advanced Types
TypeScript's advanced types can help you write more performant code by catching errors at compile-time and providing better type inference:
// Using mapped types for efficient object transformation
type Getters<T> = {
[K in keyof T as `get${Capitalize<string & K>}`]: () => T[K]
};
interface Person {
name: string;
age: number;
}
type PersonGetters = Getters<Person>;
// Resulting type:
// {
// getName: () => string;
// getAge: () => number;
// }
8. Optimize Memory Usage
Be mindful of memory usage, especially when working with large data structures:
a. Use WeakMap
and WeakSet
for objects that should be garbage collected when no longer referenced:
const cache = new WeakMap();
function getExpensiveData(obj: object) {
if (cache.has(obj)) {
return cache.get(obj);
}
const expensiveData = computeExpensiveData(obj);
cache.set(obj, expensiveData);
return expensiveData;
}
b. Use generators for working with large sequences of data:
function* fibonacci(): Generator<number> {
let [a, b] = [0, 1];
while (true) {
yield a;
[a, b] = [b, a + b];
}
}
const fib = fibonacci();
console.log(fib.next().value); // 0
console.log(fib.next().value); // 1
console.log(fib.next().value); // 1
console.log(fib.next().value); // 2
In this example:
Generator Function: The
fibonacci
function is a generator that uses thefunction*
syntax.Yield: The
yield
keyword allows the function to pause execution and return a value, resuming where it left off whennext()
is called again.Memory Efficiency: By generating Fibonacci numbers on-demand, we avoid storing the entire sequence in memory, which is particularly useful if we only need a few numbers at a time.
Benefits of Using Generators:
Lazy Evaluation: Values are computed as needed, which saves memory and processing time.
Infinite Sequences: Generators can produce potentially infinite sequences without running out of memory.
9. Use Web Workers for Intensive Computations
For computationally intensive tasks that might block the main thread, consider using Web Workers:
// main.ts
const worker = new Worker('worker.js');
worker.postMessage({ data: 'some data' });
worker.onmessage = (event) => {
console.log('Received result:', event.data);
};
// worker.ts
self.onmessage = (event) => {
const result = performExpensiveComputation(event.data);
self.postMessage(result);
};
Balancing Optimization and Readability
While these optimization techniques can significantly improve your code's performance, it's crucial to maintain a balance with readability. Here are some tips to help you strike that balance:
Comment your optimizations: When you apply an optimization that might not be immediately obvious, add a comment explaining what you've done and why.
Use meaningful variable and function names: Even when optimizing, continue to use clear and descriptive names that explain the purpose of your code.
Extract complex optimizations into separate functions: If an optimization makes a piece of code harder to understand, consider extracting it into a well-named function.
Write tests: Comprehensive tests can help ensure that your optimizations don't introduce bugs and can serve as documentation for how the optimized code should behave.
Profile before optimizing: Use TypeScript's source maps with browser developer tools or Node.js profiling tools to identify actual bottlenecks before applying optimizations.
Consider the trade-offs: Always weigh the performance gain against the cost in terms of code complexity and maintainability.
Conclusion
Optimizing TypeScript code while maintaining readability is a balancing act that requires skill, experience, and careful consideration. By applying the techniques discussed in this article judiciously and always keeping code clarity in mind, you can write TypeScript code that is both performant and maintainable.
Remember that premature optimization can lead to unnecessary complexity, so always measure and profile your code to identify true bottlenecks. When you do optimize, strive to do so in a way that preserves or even enhances the readability of your code.
By following these principles and techniques, you'll be well on your way to writing TypeScript code that is not only fast and efficient but also clear, maintainable, and a joy to work with.
Subscribe to my newsletter
Read articles from Ayoub Touba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
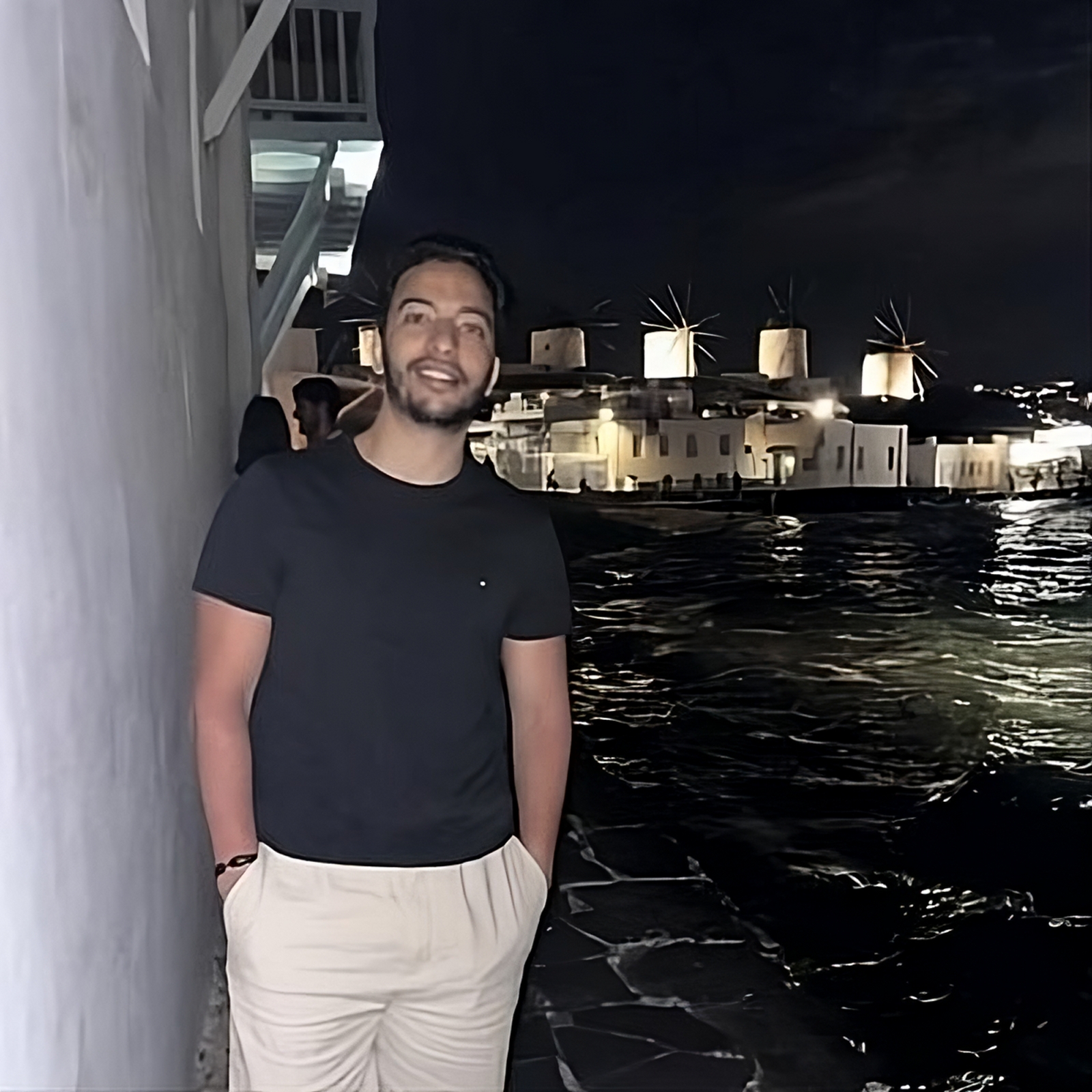
Ayoub Touba
Ayoub Touba
With over a decade of hands-on experience, I specialize in building robust web applications and scalable software solutions. My expertise spans across cutting-edge frameworks and technologies, including Node.js, React, Angular, Vue.js, and Laravel. I also delve into hardware integration with ESP32 and Arduino, creating IoT solutions.