Understanding Concurrency in Elixir: Key Practices and Examples
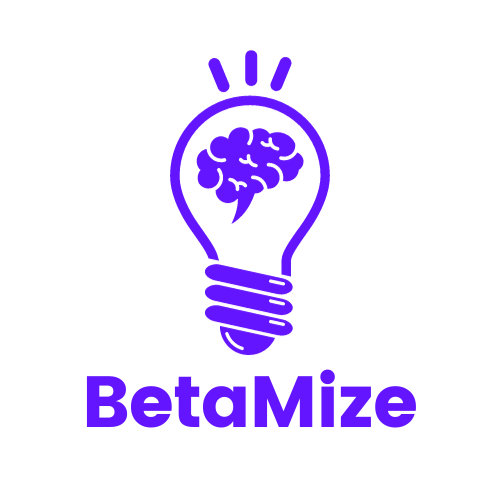
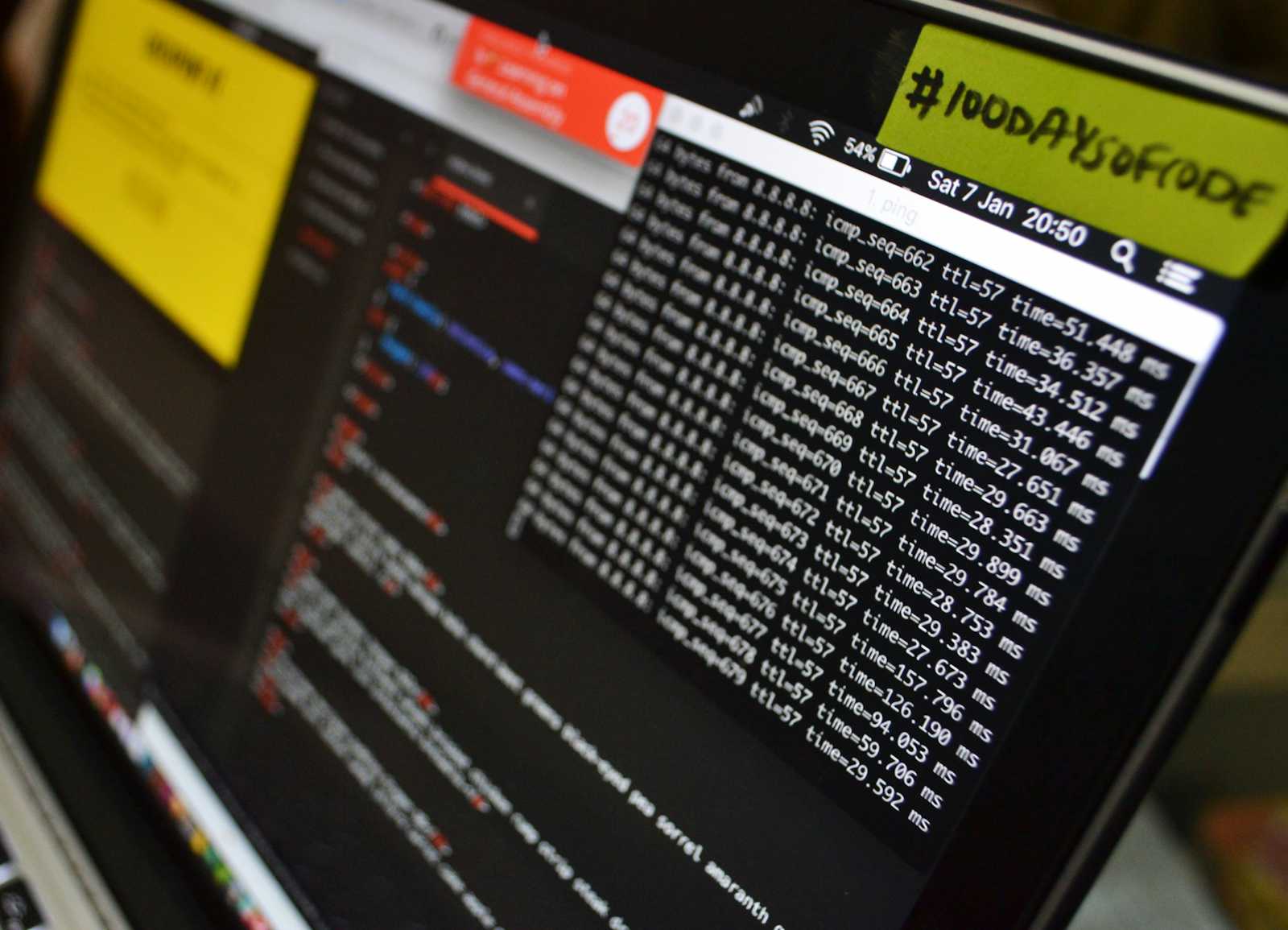
Concurrency is a core strength of Elixir, a language designed for building scalable and maintainable applications. Leveraging the Erlang VM, Elixir excels in handling numerous simultaneous connections, making it ideal for modern web applications, real-time systems, and distributed computing. In this blog post, we'll explore Elixir's concurrency model, discuss best practices, and provide practical examples to help you harness the power of concurrency in your applications.
Understanding Elixir's Concurrency Model
Elixir's concurrency model is based on the Actor model, where each actor (or process) runs independently and communicates with other actors via message passing. This model, inherited from Erlang, allows for:
Isolation: Each process has its own memory and state, preventing accidental interference from other processes.
Fault Tolerance: Processes can crash without affecting others, and supervisors can restart failed processes automatically.
Scalability: Lightweight processes can be created and managed efficiently, enabling the system to handle a large number of concurrent activities.
Key Concepts in Elixir Concurrency
1. Processes
Processes in Elixir are lightweight and managed by the BEAM virtual machine. They are not the same as operating system processes and can be created in large numbers without significant overhead.
spawn(fn ->
IO.puts("Hello from a new process!")
end)
2. Message Passing
Processes communicate by sending and receiving messages. This decouples them from each other, enhancing fault tolerance and scalability.
send(self(), :hello)
receive do
:hello -> IO.puts("Received a message!")
end
3. Task Module
The Task
module provides a simple way to perform concurrent operations. It abstracts the creation and management of processes.
task = Task.async(fn ->
:timer.sleep(1000)
42
end)
result = Task.await(task)
IO.puts("The result is #{result}")
4. GenServer
GenServer
is a generic server implementation that simplifies process creation and management. It's commonly used for building server-like processes that maintain state.
defmodule Counter do
use GenServer
def start_link(initial_value) do
GenServer.start_link(__MODULE__, initial_value, name: __MODULE__)
end
def increment do
GenServer.call(__MODULE__, :increment)
end
def handle_call(:increment, _from, state) do
{:reply, state + 1, state + 1}
end
end
{:ok, _pid} = Counter.start_link(0)
IO.puts("Counter value: #{Counter.increment()}")
Best Practices for Concurrency in Elixir
1. Keep Processes Lightweight
Elixir processes are designed to be lightweight, so use them liberally for concurrency. However, ensure that each process has a clear and focused responsibility to avoid unnecessary complexity.
2. Use Supervision Trees
Supervision trees provide a structured way to manage process lifecycles and handle failures gracefully. Use supervisors to restart failed processes and ensure system stability.
defmodule MyApp.Supervisor do
use Supervisor
def start_link(_) do
Supervisor.start_link(__MODULE__, :ok, name: __MODULE__)
end
def init(:ok) do
children = [
{Counter, 0}
]
Supervisor.init(children, strategy: :one_for_one)
end
end
{:ok, _supervisor} = MyApp.Supervisor.start_link([])
3. Avoid Blocking Operations
Avoid blocking operations inside processes, especially those managed by GenServer
. Use asynchronous tasks or delegate heavy computations to separate processes.
def handle_call(:heavy_task, _from, state) do
Task.start(fn -> perform_heavy_task() end)
{:reply, :ok, state}
end
4. Leverage OTP Behaviors
OTP (Open Telecom Platform) behaviors like GenServer
, Supervisor
, and Task
provide robust abstractions for building concurrent applications. Use them to simplify process management and ensure best practices.
Practical Examples of Concurrency in Elixir
1. Concurrent Web Requests
Imagine you need to fetch data from multiple external APIs concurrently. Elixir makes this task simple and efficient.
urls = ["https://api.example.com/1", "https://api.example.com/2", "https://api.example.com/3"]
tasks = for url <- urls do
Task.async(fn -> HTTPoison.get!(url).body end)
end
results = for task <- tasks do
Task.await(task)
end
IO.inspect(results)
2. Real-Time Chat Application
A real-time chat application benefits from Elixir's concurrency model by handling each user's connection as a separate process. Using Phoenix Channels, you can build scalable real-time features.
defmodule MyAppWeb.UserSocket do
use Phoenix.Socket
channel "room:*", MyAppWeb.RoomChannel
def connect(_params, socket, _connect_info) do
{:ok, socket}
end
def id(_socket), do: nil
end
defmodule MyAppWeb.RoomChannel do
use Phoenix.Channel
def join("room:lobby", _message, socket) do
{:ok, socket}
end
def handle_in("new_msg", %{"body" => body}, socket) do
broadcast!(socket, "new_msg", %{"body" => body})
{:noreply, socket}
end
end
3. Background Job Processing
Background job processing can be efficiently managed using Elixir's concurrency features. Libraries like Oban
provide robust solutions for job scheduling and execution.
defmodule MyApp.Worker do
use Oban.Worker, queue: :default
def perform(%Oban.Job{args: %{"task" => task}}) do
case task do
"send_email" -> send_email()
_ -> :ok
end
end
defp send_email do
# Email sending logic here
end
end
%{"task" => "send_email"}
|> MyApp.Worker.new()
|> Oban.insert()
Conclusion
Concurrency in Elixir, powered by the BEAM VM and OTP, provides a robust foundation for building scalable, fault-tolerant applications. By understanding and leveraging Elixir's concurrency model, you can create efficient and maintainable systems that handle numerous simultaneous tasks with ease.
Ready to build high-performance, concurrent applications with Elixir? Visit ElixirMasters for expert Elixir and Phoenix development services, developer hiring solutions, and consultancy. Elevate your project with the power of Elixir today!
Subscribe to my newsletter
Read articles from BetaMize directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
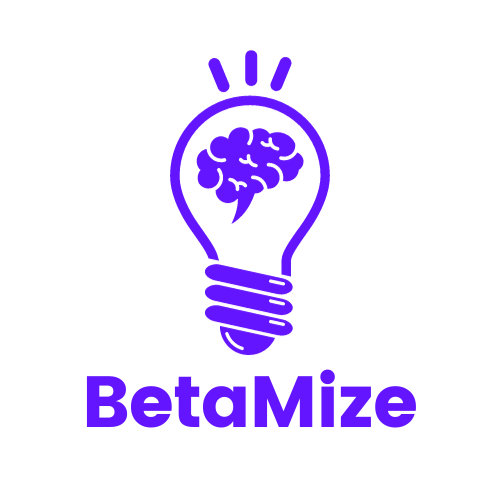