Printing a Diamond shape

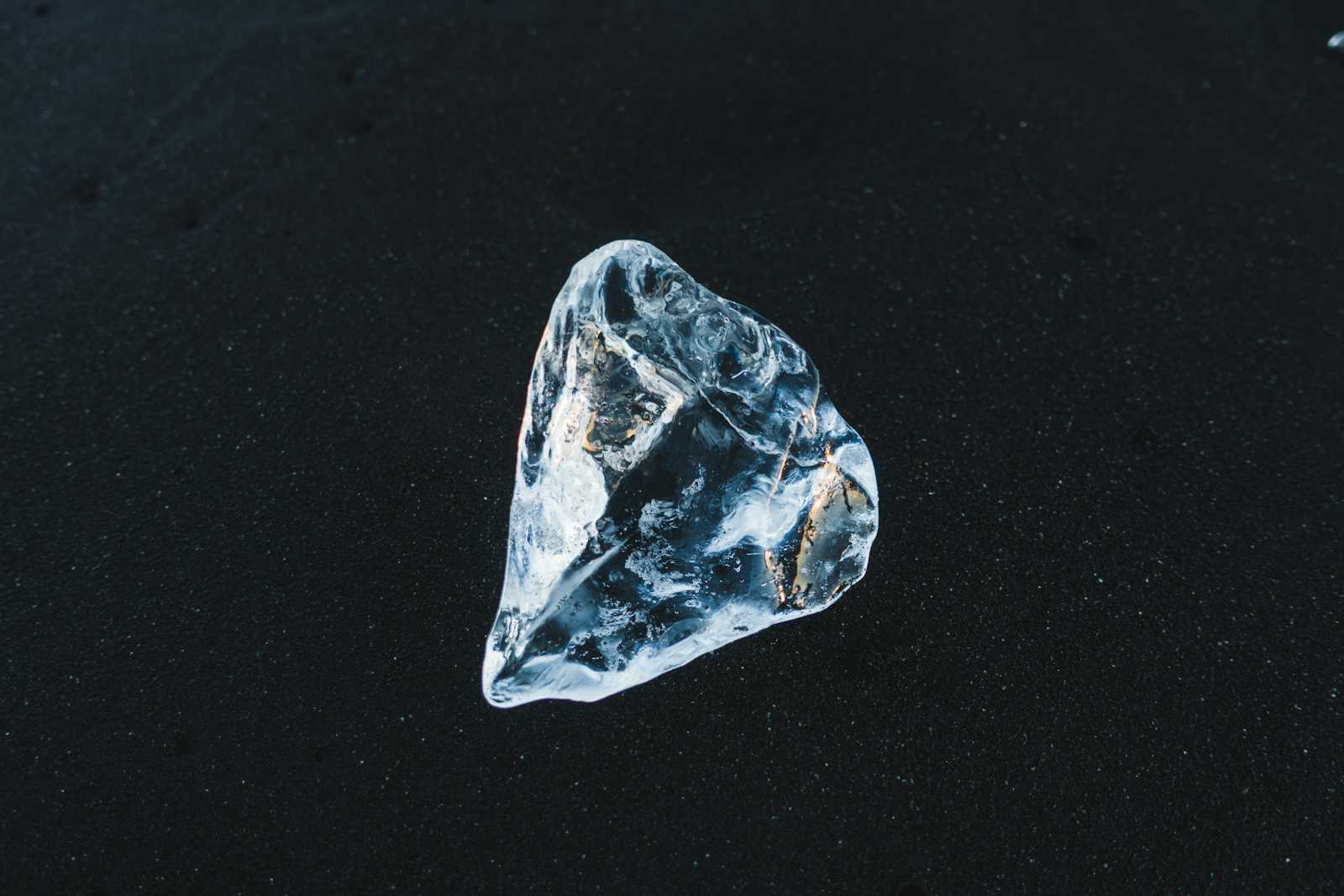
Introduction
Hey there! ๐ In this post, we will be discussing how to print a diamond shape using COBOL. We'll be using asterisk โณ symbol to form the shape.
Approach
Before coding the logic, I always sketch out the shape in an Excel sheet. The approach is to print the diamond shape spread across 6 lines with 9 characters in each line as shown in the picture below.
After printing the first line, it's almost similar to printing a reverse Triangle shape ๐ป
PERFORM loop and DISPLAY statement in COBOL will be used to form the shape.
Diamond shape in COBOL
Here is the COBOL code to print the Diamond shape.
IDENTIFICATION DIVISION.
PROGRAM-ID. DIAMOND.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-OUT PIC X(9) VALUE SPACES.
01 WS-I PIC 9(2) VALUE 0.
01 WS-N PIC 9(2) VALUE 6.
01 WS-LENGTH PIC 9(2) VALUE 9.
PROCEDURE DIVISION.
PERFORM VARYING WS-I FROM 1 BY 1 UNTIL WS-I > WS-N - 1
IF WS-I = 1
MOVE ALL '*' TO WS-OUT(2:7)
DISPLAY WS-OUT
END-IF
INITIALIZE WS-OUT
MOVE ALL '*' TO WS-OUT(WS-I:WS-LENGTH)
DISPLAY WS-OUT
COMPUTE WS-LENGTH = WS-LENGTH - 2
END-PERFORM.
STOP RUN.
What the above code does?
In the WORKING-STORAGE section, we define the following variables:
WS-OUT is alphanumeric data-item defined with a length of 9. This is used to display each line of the diamond shape.
WS-I is a counter variable for looping and is initialized with 0.
WS-N represents the total no. of lines in which the diamond shape will be spread out.
WS-LENGTH is an integer data-item initialized with the value of 9 and this will be used in the logic to control the no. of asterisks printed on each line.
In the PROCEDURE DIVISION, we have the logic to print shape and it goes like this:
The PERFORM loop uses WS-I as the counter variables and iterated from 1 until the value is greater than WS-N - 1 i.e., from 1 to 5.
Printing the first line needs a special treatment. Inside the PERFORM loop, we have an IF statement to identify if it's the first iteration. If it's, then we MOVE asterisks to positions 2 to 7.
For the rest of the lines:
WS-OUT is always initialized with spaces before displaying.
The MOVE statement moves asterisks to all the 9 positions of WS-OUT in the first iteration, but it reduces the number of asterisks in the further iterations based on the values in WS-I and WS-LENGTH. That's how we form the rest of the diamond's shape ๐ท
Output
*******
*********
*******
*****
***
*
That looks like a compressed diamond and I'm going to blame Hashnode's code editor for that ๐
Here's how ๐ the diamond looks like in JDoodle's Output window.
Nevertheless, diamonds are precious!
Click ๐ here to run the code on JDoodle's Online COBOL Compiler.
Conclusion
We've hit the bottom of the post.
See you in the post for the next shape.
Thx!
Subscribe to my newsletter
Read articles from Srinivasan JV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Srinivasan JV
Srinivasan JV
I have been a mainframe developer for the past 10 years. As part of work obligations, I am currently in pursuit of learning Java. You'll find me writing more about the mainframe.