Managing CORS Problems in JavaScript Full-Stack Environments

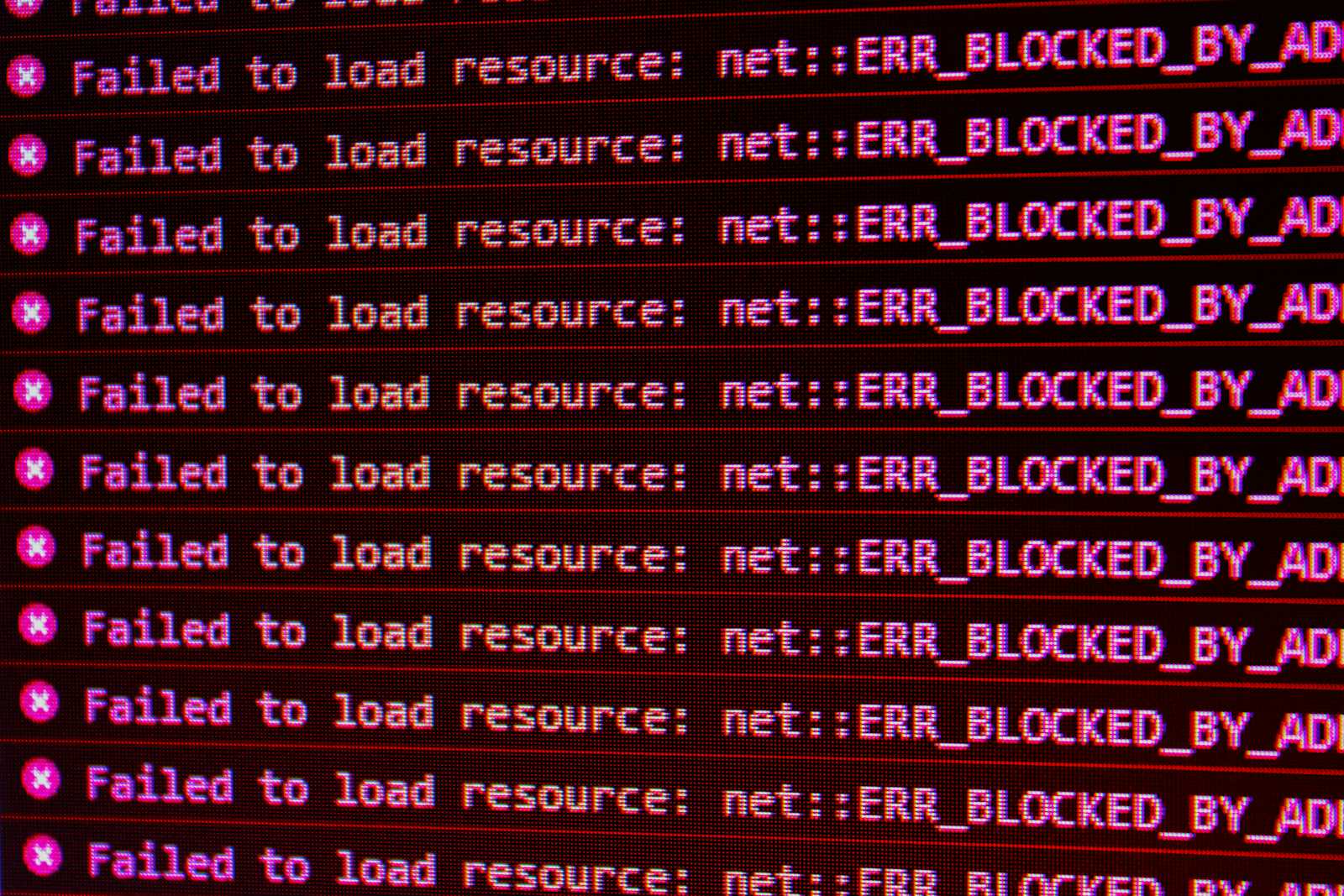
Solving CORS Issues in a Full-Stack JavaScript Application
When building a full-stack JavaScript application, it's common to encounter Cross-Origin Resource Sharing (CORS) issues. This is particularly true when your frontend and backend are served on different ports, such as 5173 for the frontend and 3000 for the backend. In this article, we'll explore what CORS is, why it occurs, and how to resolve it effectively.
What is CORS?
CORS is a security feature in web browsers that stops harmful websites from making requests to a different domain than the one that served the web page. While it's important for security, it can be challenging during development, especially when the frontend and backend are separate.
Scenario
In our case, the backend API is served on localhost:3000
and the frontend is served on localhost:5173
. When the frontend tries to make a request to the backend, the browser blocks the request due to CORS policy.
Example Request Flow
Backend API:
http://localhost:3000/api/joking
Frontend:
http://localhost:5173
The CORS Error
When the frontend tries to fetch data from the backend, the browser throws a CORS error similar to this:
Access to fetch at 'http://localhost:3000/api/joking' from origin 'http://localhost:5173'
How to Fix CORS Issues
To resolve this issue, you need to configure your backend to include the appropriate CORS headers. Here's a step-by-step guide to solving CORS issues in a Node.js and Express application:
Install CORS Middleware
First, you need to install the
cors
package in your backend project. This middleware will help you handle CORS easily.npm install cors
Configure CORS in Your Backend
In your Express app, require the
cors
package and use it as middleware. You can specify which origins are allowed to access your backend.const express = require('express'); const cors = require('cors'); const app = express(); const corsOptions = { origin: 'http://localhost:5173', // Allow only this origin optionsSuccessStatus: 200 }; app.use(cors(corsOptions)); app.get('/api/joking', (req, res) => { res.json({ jokes: [ { id: 1, joke: 'Why do programmers always mix up Christmas and Halloween? Because Oct 31 == Dec 25.' }, { id: 2, joke: 'Why do developers hate nature? It has too many bugs.' } ] }); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
Allow All Origins (Optional)
If you want to allow all origins (not recommended for production), you can configure the CORS middleware as follows:
app.use(cors());
Frontend Fetch Request
In your frontend code, you can now make a fetch request without worrying about CORS issues.
fetch('http://localhost:3000/api/jokes') .then(response => response.json()) .then(data => { console.log(data); }) .catch(error => { console.error('Error fetching jokes:', error); });
What I Used ?
I used the proxy method. I setup a proxy in my vite.config.js which easily resolved the CORS error.
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
// https://vitejs.dev/config/
export default defineConfig({
server: {
proxy: {
'/api': 'http://localhost:3000',
},
},
plugins: [react()],
})
Don't while you encounter CORS error
Don't Disable CORS in the Browser: Turning off CORS in the browser is risky and should never be done, especially in production.
Don't Use Wildcard (*) in Production: Using a wildcard (*) to allow all origins in CORS settings is not safe for production as it can expose your app to security risks.
Don't Ignore Preflight Requests: Preflight requests are a key part of CORS. Ignoring them can cause unexpected issues and errors.
Don't Hardcode Origins: Hardcoding origins in your CORS settings makes your app less flexible and harder to manage. Use environment variables or config files instead.
Don't Forget to Handle Credentials: If your app needs credentials (like cookies or HTTP authentication), make sure to set up CORS to allow credentials and specify the allowed origins.
Don't Assume CORS Issues are Always Backend Problems: Sometimes, CORS issues can come from frontend misconfigurations. Check that your frontend code is making the right requests to the backend.
Subscribe to my newsletter
Read articles from Anuj Kumar Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anuj Kumar Upadhyay
Anuj Kumar Upadhyay
I am a developer from India. I am passionate to contribute to the tech community through my writing. Currently i am in my Graduation in Computer Application.