How Java is made a safe programming language.

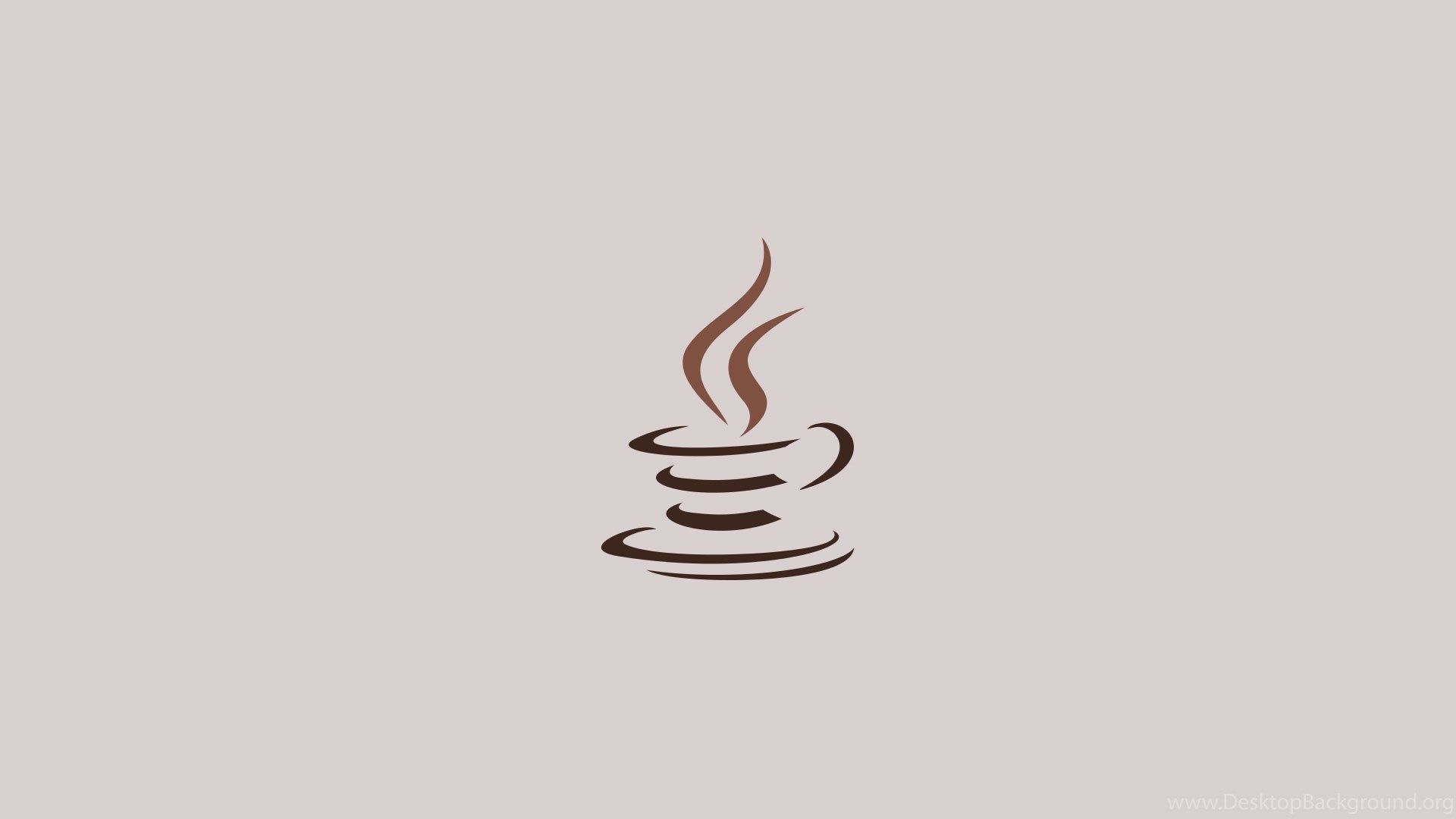
Java, developed by Sun Microsystems in the mid-1990s,Now its a product of Oracle has become one of the most popular programming languages in the world. Its success can be attributed to many factors, including its platform independence, robustness, and simplicity. However, one of the standout features that makes Java particularly appealing is its focus on security. In this blog post, we will explore how Java is designed to be a safe programming language, and the mechanisms it employs to protect applications from various security threats. We will see why in Java garbage collection is automatic and removed the Destructors, why Java doesn't support pointer for primitive data types, No support for multiple inheritance and much more ...
1. Java Virtual Machine (JVM)
Java Development kit is used where it contains the compiler, interpreter, predefined classes and utilities.
JVM has compiler interpreter and classess
Java programs are compiled into bytecode, an intermediate code, which is then executed by the Java Virtual Machine (JVM). This type of compilation gives us such benefits:
Platform Independence: Since bytecode is the same regardless of the underlying platform, it can run on any device with a compatible JVM, reducing the risk of platform-specific vulnerabilities. where in C++, the code won't compile on other machine, if it has compiled to other machine. Before execution, the JVM checks the bytecode to ensure Java’s security constraints . This verifies that the code does not perform illegal casts, access out-of-bounds arrays, or perform unauthorized operations which includes accessing the variable without assigning a value to it.
2. Why Garbage Collection is Automatic
One of the most common sources of security vulnerabilities in programming languages is improper memory management that if we can see in C++ the memory release is difficult while interacting with layered application. Java addresses this through automatic garbage collection, which helps prevent:
Memory Leaks: By automatically releasing the memory that is no longer in use, Internally it maintains some DS to manage this and the JVM always looks for some data which is not used. So this is how Java reduces the risk of memory leaks, which can lead to system crashes and unpredictable behavior.
Destructor : Garbage collection is automatic so James Gosling removed the feature of Destructor also.
Arrays as Objects : In Java Arrays are treated as an Object where Java performs automatic bounds checking on arrays, preventing buffer overflow as the Object contains the length named property to do this, which are common in languages like C and C++.
3. Inheritance
In Java we can inherit a class properties using "extends" keyword, and we don't have to provide the visibility mode while inheritance.
Java has removed the multiple inheritance because of the inheritance through multiple path which create an issue in C++, so we have to declare the n-1 path to be declared as virtual.
But Java introduced a feature called Interface to impose guidelines. where we can inherit multiple interfaces in a class by "implements" keyword, and interface can extends multiple interfaces.
interface extends interface
whereas,
class implements interface.
We cannot create an object of interface. Interface is only to impose guidelines.
In new versions, we can define the method in Interface by using a "default" keyword.
5. Class Loaders
Class Loaders in Java are responsible for dynamically loading classes at runtime. This help us to access the class which will be created in future. This is a very powerful feature if it used properly. They provide a namespace for the classes they load and help isolate classes from one another, which is vital for maintaining security:
Static "Class" named class is already created to assess the functionalities of other classes and it we want to know about some other class, so we can get the details about all the classes with "Class" named static class.
Namespace Separation: By loading classes into separate namespaces, Class Loaders prevent unauthorized interactions between classes from different sources.
Bytecode Verification: Class Loaders also play a role in bytecode verification, ensuring that loaded classes adhere to Java’s security constraints.
6. Access Specifier and Encapsulation
Java also does not support the access specifier which Inheritance.
Java’s access control mechanisms allow developers to define the visibility and accessibility of classes, methods, and variables:
Encapsulation: By encapsulating data and providing controlled access through methods, Java promotes secure coding practices and reduces the risk of unintended data exposure.
Modifiers: Keywords like
private
,protected
, andpublic
help restrict access to class members, preventing unauthorized access and modification.public can be accessed any where in package.
private cannot be accessed anywhere.
No Specifier is as good as public for inside the package, cannot accessed outside the package.
protected is as good as public derived classes and public inside the package.
7. Strong Typed Language
Java is a strongly-typed language, meaning that types are strictly enforced at both compile-time and runtime. This reduces the risk of type confusion errors, which can lead to loss of data.
Java internally does not typecast the from larger bytes values to small byte value. But can be done explicitly by the programmer that the programmer know about the type conversion.
But in some case such as Long(64 bit) type value is converted and kept to float type values(32 bit). bcoz internally the bit conversion from long to float value is different.
Final variable cannot be redeclared, otherwise code not compiles.
Final method can't be overridden, and Final class cannot be inherited.
8. Exception Handling
Additionally, Java’s robust exception handling mechanisms allow developers to write code that can gracefully handle unexpected conditions and errors, improving the overall reliability and security of applications.
Java has divided the exception handling into various patterns.
Object is the super-most class in Java. If we don't write then JVM itself provides that.
Java provides a great feature that :
In Java if we use Exception Handling where no exception will be raised then the code won't compile and if something throws an exception then we have to handle it properly with a catch block or
we can pass the exception to other with "throws" keyword followed by type of exception is being raised.
The Exception Handling chart is given below to understand better.
Conclusion
In conclusion, I need to say that java is created safer that any average programmer can't make any such silly mistakes while creating an application.
So all these feature which create problematic situation or which can't be handled by an average programmers are removed and encapsulated. if any how the programmer makes a mistake them it will be tested while compilation or in testing.
Thanks for your patience and time :)
Hope you will understand the concept of creating Java programming language.
Subscribe to my newsletter
Read articles from Shubham Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
