Shell Scripting Challenge #day-9 Directory Backup with Rotation

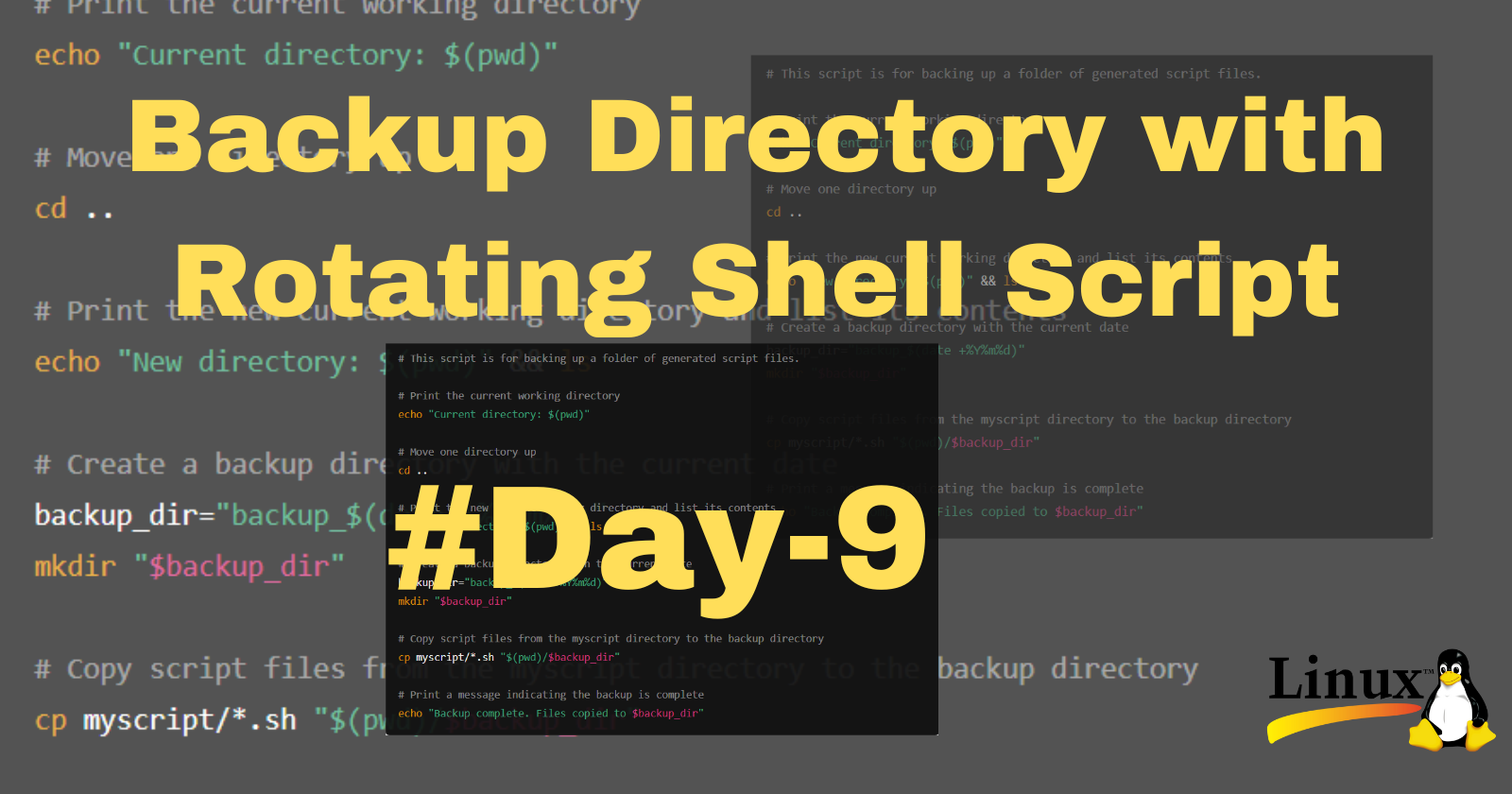
Your task is to create a bash script that takes a directory path as a command-line argument and performs a backup of the directory. The script should create timestamped backup folders and copy all the files from the specified directory into the backup folder.
Additionally, the script should implement a rotation mechanism to keep only the last 3 backups. This means that if there are more than 3 backup folders, the oldest backup folders should be removed to ensure only the most recent backups are retained.
#!/bin/bash
# Display usage information
function display_usage {
echo "Usage: $0 /path/to/source_directory"
}
# Check if a valid directory path is provided as a command-line argument
if [ $# -eq 0 ] || [ ! -d "$1" ]; then
echo "Error: Please provide a valid directory path as a command-line argument."
display_usage
exit 1
fi
# Assign source directory from command-line argument
source_dir="$1"
# Function to create a timestamped backup and zip it
function create_backup {
local timestamp=$(date '+%Y-%m-%d_%H-%M-%S')
local backup_dir="${source_dir}/backup_${timestamp}"
# Create backup directory and zip its contents
zip -r "${backup_dir}.zip" "$source_dir" >/dev/null
if [ $? -eq 0 ]; then
echo "Backup created successfully: ${backup_dir}.zip"
else
echo "Error: Failed to create backup."
fi
}
# Function to perform rotation and keep only the last 3 backups
function perform_rotation {
local backups=($(ls -t "${source_dir}/backup_"*.zip 2>/dev/null))
if [ "${#backups[@]}" -gt 3 ]; then
local backups_to_remove=("${backups[@]:3}")
for backup in "${backups_to_remove[@]}"; do
rm -f "$backup"
done
fi
}
# Main script logic
create_backup
perform_rotation
Result:
Conclusion
Creating a robust backup system is crucial for data integrity and security. By implementing a shell script that not only backs up directories but also manages backup rotation, you ensure that your system remains efficient and clutter-free. This script, which retains only the last three backups, provides a practical solution for maintaining up-to-date backups while conserving storage space. This challenge not only enhances your scripting skills but also underscores the importance of regular and managed backups in any computing environment.
Thanks for reading. Happy Learning !!
Connect and Follow Me On Socials :
Like👍 | Share📲 | Comment💭
Subscribe to my newsletter
Read articles from Nikunj Vaishnav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nikunj Vaishnav
Nikunj Vaishnav
👋 Hi there! I'm Nikunj Vaishnav, a passionate QA engineer Cloud, and DevOps. I thrive on exploring new technologies and sharing my journey through code. From designing cloud infrastructures to ensuring software quality, I'm deeply involved in CI/CD pipelines, automated testing, and containerization with Docker. I'm always eager to grow in the ever-evolving fields of Software Testing, Cloud and DevOps. My goal is to simplify complex concepts, offer practical tips on automation and testing, and inspire others in the tech community. Let's connect, learn, and build high-quality software together! 📝 Check out my blog for tutorials and insights on cloud infrastructure, QA best practices, and DevOps. Feel free to reach out – I’m always open to discussions, collaborations, and feedback!