(lt.47)"Mastering Node.js: Creating a Server with HTTP Module, URL Handling, Express.js Frameworks, Port Numbers, and REST API Integration

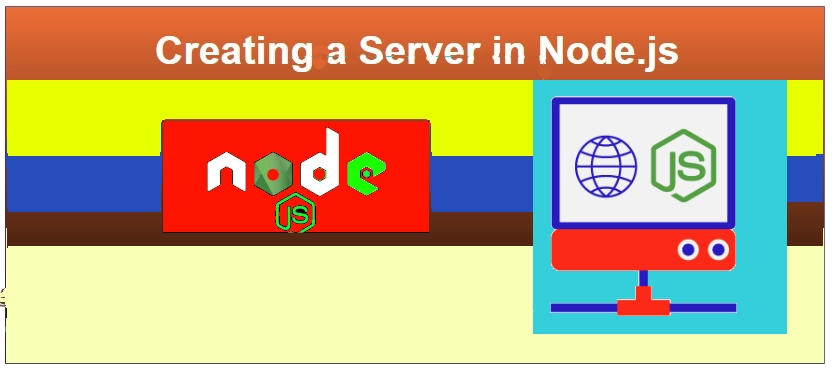
We can create a server using http module in NodeJS
In Nodejs, the HTTP (which stands for Hyper Transfer protocol) module is a core module that provides functionality for creating and interacting with HTTP servers and clients.
A HTTP request is a message sent by a client to a server, requesting for some information or action to be performed. It is the primary means by which web browsers, search engines, and other web clients communicate with web servers.
HTTP modules:
HTTP modules is the core modules of Nodejs
HTTP modules provide way to create server and handle HTTP request and response.
It exposes various classes, functions and properties to work with HTTP
To use the HTTP server and Client one must require(“node:http”)
Features
Create an HTTP server using http.createServer()
Listen to incoming request using the server.listen() method
Handle incoming requests and send responses using the request and response object passed to the server’s request event
Set headers on the response object using response.setHeader()
Write data to the response object using response.write()
End the response using response.end()
Send an HTTP request to a server using http.request()
Receive a response from a server using the response event of the http.clientRequest object returned by http.request()
URL
A URL, or Uniform Resource Locator, is a web address used to identify a specific resource on the Internet. It's what you type into your browser to visit a website or access a particular page or service online.
const PORT = 3022;
const HOST = "localhost";
const server = htttp.createServer((req, res) => {
if (req.url === "/") {
res.statusCode = 200;
res.setHeader("Content-Type", "plain/text");
res.end("Welcome to the server");
} else if (req.url === "/about") {
res.statusCode = 200;
res.setHeader("Content-Type", "text/plain");
res.end("About page");
} else if (req.url === "/contact") {
res.statusCode = 200;
res.setHeader("Content-Type", "text/plain");
res.end("Contact information");
}
else if (req.url == "/product") {
const options = {
hostname: "fakestoreapi.com",
path: "/products/1",
method: "GET",
}
const apireq = http.request(options, (apires) => {
apires.on("data", (data) => {
res.statusCode = 200;
res.setHeader("content-type", "application/json");
res.end(JSON.stringify(data.toString()));
});
});
apireq.on("error", () => {
console.log(e);
});
apireq.end();
}
// in the above if else part client is requesting product from node server
// and the node server is requesting it from the fakestoreapi server
// hence in the above if else we are requesting a service from server to server
else {
res.statusCode = 404;
res.setHeader("Content-Type", "text/plain");
res.end("Error: No page found");
}
});
server.listen(PORT, () => {
console.log(`Server running at http://${HOST}:${PORT}/`);
});
Frameworks
A framework is a collection of tools, libraries, and best practices that provide a structure for building applications. It helps developers by providing reusable code and a consistent way to organize and develop their projects, making it easier and faster to create software.
What Does a Framework Do?
Provides Structure: Offers a clear and organized way to build applications, so developers don't have to start from scratch.
Reuses Code: Contains pre-written code for common tasks, saving time and effort.
Simplifies Development: Handles common tasks (like routing, authentication, and database interactions) so developers can focus on the unique aspects of their application.
NODE frameworks
For Beginners:
Express.js: Flexible and popular choice for building basic web applications and APIs.
Sails.js: Makes building data-driven apps with real-time features easier, similar to Ruby on Rails.
For Modern Applications:
Koa.js: Smaller and more modular foundation for web applications, offering more control over how your app works.
NestJS: Builds complex and scalable applications with features like dependency injection and support for different data transfer methods. (Uses TypeScript)
Fastify: Prioritizes high performance and low overhead, making it ideal for fast and efficient applications.
For Enterprise-grade Applications:
Hapi.js: Powerful and feature-rich framework with a focus on configuration and reliability, good for complex business applications.
LoopBack: Highly focused on building APIs and connecting them to data sources (like databases). Maintained by IBM.
For Real-time Applications:
Meteor.js: Open-source platform for building real-time web, mobile, and desktop applications. Integrates well with MongoDB.
Feathers.js: Lightweight framework for building real-time functionality into web and mobile apps. Flexible and works with various databases.
Express.js
Express is a popular web framework for Node.js that makes it easier to build web applications and APIs. Think of it as a tool that helps you handle web requests and responses in a clean and organized way.
Express is a back-end component of popular development stacks like MEAN, MERN, or MEVN, together with the MongoDB database software and a JavaScript Front-end framework or library.
Why to choose express
Simplicity and Minimalism: Easy to learn and use.
Flexibility: Unopinionated and modular.
Large Ecosystem: Abundant middleware and active community.
Performance: Lightweight and efficient.
Compatibility and Integration: Seamless with Node.js and other tools.
Scalability: Suitable for microservices and APIs.
Robust Routing: Powerful and versatile routing capabilities.
Comprehensive Documentation: Well-documented with community support.
Coding demonstration to work with express
create a new folder u can name it any lets suppose we can name it express
cd express
npm init -y // installing package.json
npm i express --save // installing express we have to instll it to use it
/*+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/
const expr = require("express");
const app = expr();
const port = 4011;
const hostname = "localhost";
app.get("/", (req, res) => {
res.send("hello server ignite");
});
app.get("/about", (req, res) => {
res.send("about section");
});
app.get("/contact", (req, res) => {
res.send("contact info");
});
app.listen(port, () => {
console.log(`server running at ${hostname}: ${port}`);
});
PORT NUMBER
A port number is like an address for network communications. When you connect to a computer over the internet or a local network, you use an IP address to find the computer and a port number to connect to a specific service or application on that computer.
Address for Services:
- Think of an IP address as a street address and a port number as an apartment number. The IP address gets you to the building, and the port number gets you to the right apartment.
Identifying Services:
- Different services and applications on a computer listen on different port numbers. For example, web servers typically use port 80 (HTTP) or 443 (HTTPS), and email servers might use port 25 (SMTP).
Range of Port Numbers:
- Port numbers range from 0 to 65535. Some ports are reserved for specific services (like port 80 for HTTP), but many are available for general use.
The main types of ports:
1. Well-Known Ports
Range: 0 to 1023
Description: These ports are reserved for specific services and are standardized across different systems and applications.
Examples:
HTTP: Port 80
HTTPS: Port 443
FTP: Port 21
SMTP: Port 25
DNS: Port 53
SSH: Port 22
2. Registered Ports
Range: 1024 to 49151
Description: These ports are registered for specific services by software companies or organizations. They are not as standardized as well-known ports but are often used for specific applications.
Examples:
MySQL: Port 3306
PostgreSQL: Port 5432
Microsoft SQL Server: Port 1433
Apache Tomcat: Port 8080
3. Dynamic or Private Ports
Range: 49152 to 65535
Description: These ports are not assigned to any specific service and can be used for any general purpose. They are often used for temporary or private connections and are dynamically assigned by the operating system.
REST API
A REST API (Representational State Transfer Application Programming Interface) is a way for different software applications to communicate with each other over the internet using a set of rules. It allows one application to access and manipulate data from another application, typically using HTTP requests.
Data is usually sent to and received from a REST API in JSON format, which is easy to read and write for both humans and machines.
REST APIs use standard HTTP methods to perform operations on resources:
GET: Retrieve data from the server.
POST: Create new data on the server.
PUT: Update existing data on the server.
DELETE: Delete data from the serve
Stateful vs Stateless REST API
When talking about REST APIs, the terms "stateful" and "stateless" refer to how the server handles and manages the state of client interactions.
A stateless REST API does not store any client context (state) on the server between requests.
A stateful REST API stores some client context (state) on the server between requests. This means the server remembers previous interactions with the client.
Feature | Stateless API | Stateful API |
Session | No session state stored on the server | Session state stored on the server |
Request | Each request is independent and self-contained | Requests depend on previous interactions |
Scalability | Easier to scale | Harder to scale |
Complexity | Simpler to manage | More complex to manage |
Use Cases | Public APIs, Microservices | Web applications requiring user sessions |
Token and Cookie
A token is a piece of data created by a server and sent to a client (such as a web browser or mobile app) after successful authentication. The client stores this token and sends it with each subsequent request to the server to verify its identity.
A cookie is a small piece of data stored on the client’s browser by the server. Cookies can store user session information, which the browser sends with each request to the server, helping to maintain user sessions.
Feature | Tokens | Cookies |
Storage Location | Local storage or session storage | Browser-managed storage |
Automatic Handling | Not automatic; client code must include token | Automatic; browser includes cookies in requests |
Security | Can include additional security measures (e.g., signed tokens) | Subject to XSS and CSRF attacks |
Usage | Suitable for APIs and distributed systems | Traditional web applications |
Management | Stateless, no server-side session storage | Server-side session storage required |
Subscribe to my newsletter
Read articles from himanshu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
