Setting Up an ASP.NET Core Application with Entity Framework: A Beginner's Guide
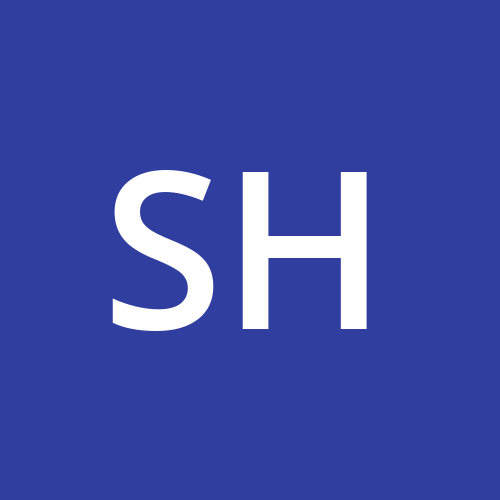
Introduction
This guide will take you through the process of setting up an ASP.NET Core application using Entity Framework (EF) for database management. We'll cover creating models, defining relationships, managing migrations, seeding data, and ensuring your API endpoints are accessible through Swagger UI.
Prerequisites
Basic knowledge of C# and ASP.NET Core.
.NET SDK installed on your machine.
SQL Server installed and running.
Step 1: Create a New ASP.NET Core Project
Open your terminal or command prompt.
Run the following command to create a new ASP.NET Core Web API project:
dotnet new webapi -n TaskManagementSystem cd TaskManagementSystem
Step 2: Add Entity Framework Core Packages
Run the following commands to add EF Core packages to your project:
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
dotnet add package Microsoft.EntityFrameworkCore.Design
Step 3: Define Your Data Models
Create a folder named Entities
and add the following classes to represent your data models.
User.cs
public class User
{
public int UserId { get; set; }
public string UserName { get; set; } = string.Empty;
public string UserEmail { get; set; } = string.Empty;
public string UserPassword { get; set; } = string.Empty;
// Navigation properties
public ICollection<UserTask>? UserTasks { get; set; }
public ICollection<Category>? Categories { get; set; }
}
Category.cs
public class Category
{
public int CategoryId { get; set; }
public int UserId { get; set; }
public string CategoryName { get; set; } = string.Empty;
// Navigation properties
public User? User { get; set; }
public ICollection<UserTask>? UserTasks { get; set; }
}
UserTask.cs
public class UserTask
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public string TaskDescription { get; set; } = string.Empty;
public int UserId { get; set; }
public int CategoryId { get; set; }
public DateTime DueDate { get; set; }
public string Status { get; set; } = string.Empty;
public string Priority { get; set; } = string.Empty;
public DateTime CreatedAt { get; set; }
// Navigation properties
public User? User { get; set; }
public Category? Category { get; set; }
}
Step 4: Configure Your DbContext
Create a folder named Data
and add the TaskDbContext
class.
TaskDbContext.cs
using Microsoft.EntityFrameworkCore;
namespace backend.Data
{
public class TaskDbContext : DbContext
{
public TaskDbContext(DbContextOptions<TaskDbContext> options) : base(options) { }
public DbSet<User> Users { get; set; }
public DbSet<Category> Categories { get; set; }
public DbSet<UserTask> UserTasks { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<User>()
.HasKey(u => u.UserId);
modelBuilder.Entity<UserTask>()
.HasKey(ut => ut.TaskId);
modelBuilder.Entity<Category>()
.HasKey(c => c.CategoryId);
modelBuilder.Entity<Category>()
.HasOne(c => c.User)
.WithMany(u => u.Categories)
.HasForeignKey(c => c.UserId);
modelBuilder.Entity<UserTask>()
.HasOne(ut => ut.User)
.WithMany(u => u.UserTasks)
.HasForeignKey(ut => ut.UserId)
.OnDelete(DeleteBehavior.ClientSetNull);
modelBuilder.Entity<UserTask>()
.HasOne(ut => ut.Category)
.WithMany(c => c.UserTasks)
.HasForeignKey(ut => ut.CategoryId)
.OnDelete(DeleteBehavior.ClientSetNull);
}
}
}
Step 5: Configure Your Connection String
In appsettings.json
, add your connection string:
"ConnectionStrings": {
"DefaultConnection": "Server=localhost;Database=TaskManagementSystem;Trusted_Connection=True;TrustServerCertificate=True;"
}
Step 6: Register Your DbContext
In Program.cs
, register your TaskDbContext
:
using backend.Data;
using Microsoft.EntityFrameworkCore;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
string connectionString = builder.Configuration.GetConnectionString("DefaultConnection")!;
builder.Services.AddDbContext<TaskDbContext>(options => options.UseSqlServer(connectionString));
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
Step 7: Create Migrations
Run the following command to create the initial migration and specify the output directory:
dotnet ef migrations add InitialCreate --output-dir Data/Migrations
Step 8: Apply Migrations
Apply the migrations to your database:
dotnet ef database update
Step 9: Seed Data
Add the following code in the OnModelCreating
method in TaskDbContext.cs
to seed initial data:
modelBuilder.Entity<User>().HasData(
new User { UserId = 1, UserName = "John Doe", UserEmail = "john.doe@example.com", UserPassword = "password" },
new User { UserId = 2, UserName = "Jane Smith", UserEmail = "jane.smith@example.com", UserPassword = "password" }
);
modelBuilder.Entity<Category>().HasData(
new Category { CategoryId = 1, UserId = 1, CategoryName = "Work" },
new Category { CategoryId = 2, UserId = 2, CategoryName = "Personal" }
);
modelBuilder.Entity<UserTask>().HasData(
new UserTask { TaskId = 1, TaskName = "Complete Project", TaskDescription = "Finish the ongoing project", UserId = 1, CategoryId = 1, DueDate = DateTime.Now.AddDays(5), Status = "Pending", Priority = "High", CreatedAt = DateTime.Now },
new UserTask { TaskId = 2, TaskName = "Buy Groceries", TaskDescription = "Buy weekly groceries", UserId = 2, CategoryId = 2, DueDate = DateTime.Now.AddDays(2), Status = "Pending", Priority = "Medium", CreatedAt = DateTime.Now }
);
Step 10: Verify API Endpoints
Run your application and verify that your API endpoints are accessible via Swagger UI by navigating to https://localhost:5001/swagger
(or the appropriate URL).
Conclusion
You've successfully set up an ASP.NET Core application with Entity Framework, created and applied migrations, defined model relationships, and seeded initial data. This guide should provide a strong foundation for building more complex applications with Entity Framework and ASP.NET Core.
Common Issues and Troubleshooting
Migration Errors: If you encounter errors during migrations, ensure your models and relationships are correctly defined. Check for circular dependencies and multiple cascade paths.
Connection String Issues: Ensure your connection string is correctly specified in
appsettings.json
.API Endpoints Not Showing: Ensure your controllers are correctly defined and registered in the services.
This comprehensive guide should help you understand the basics and get started with ASP.NET Core and Entity Framework. Happy coding!
Subscribe to my newsletter
Read articles from Shoyeab directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
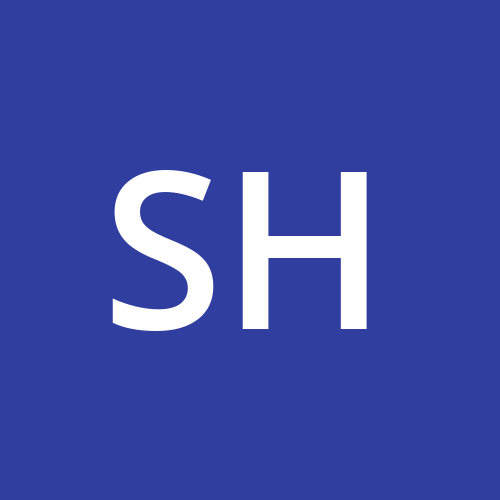
Shoyeab
Shoyeab
Passionate about building Web and mobile applications. Join me as we dive into the world of Tech!