Week-5 React
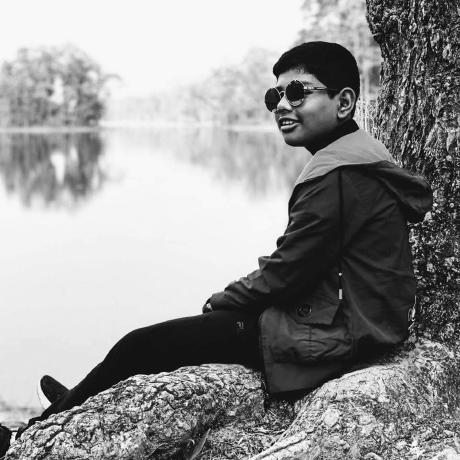
Hey everyone, I'm Binayak Shrestha, and I'm currently on a journey to learn full-stack web development through the 100xxdevs cohort. I've reached Week 5 and recently discovered Hashnode, where I've decided to share my weekly progress to stay consistent. Starting from Week 5, I'll be regularly updating this blog with my progress. Up to this point, I've learned the basics of backend development.
Course I am following
Week-5 Overview
Week 5 is the week of react basics. In this week we are going to learn the basics of react.
Why Do you need React?
React is used for efficient DOM manipulation. Under the hood, it converts React code into HTML, CSS, and JavaScript. Developed by Facebook, React is a powerful tool for creating dynamic websites.
State & Component
State is basically the current state of an element, and a component is a piece or part of the website.
As we can see in the example, the red boxes are the components, and the state is the documentation of the state of the component. For example, as the notification state changes from 10 to 11, the component re-renders to display 11.
Let's see an example of how react works using JS DOM
<!DOCTYPE html>
<html>
<body>
<div id="buttonParent">
</div>
<script>
let state = {
count: 0
}
function onButtonPress() {
state.count++;
buttonComponentReRender()
}
function buttonComponentReRender() {
document.getElementById("buttonParent").innerHTML = "";
const component = buttonComponent(state.count);
document.getElementById("buttonParent").appendChild(component);
}
function buttonComponent(count) {
const button = document.createElement("button");
button.innerHTML = `Counter ${count}`;
button.setAttribute("onclick", `onButtonPress()`);
return button;
}
buttonComponentReRender();
</script>
</body>
</html>
In this example, we can see that there is 3 primary function onButtonPress,buttonComponentReRender,buttonComponent.
-->Written by ChatGPT
In React terms, this code simulates a basic component where a button's state is managed locally. The state, state.count
, is equivalent to React's component state, holding the number of button clicks. The buttonComponentReRender()
function resembles React's component rendering method, triggered to update the UI whenever state.count
changes. Inside buttonComponentReRender()
, the DOM is manipulated directly (similar to how React would handle rendering with virtual DOM), where the old button is cleared and a new one with updated state (state.count
) is generated and appended. The onButtonPress()
function acts like an event handler in React, updating the state (state.count++
) and triggering a re-render (buttonComponentReRender()
) to reflect the updated count visually. This mirrors React's unidirectional data flow and state management, albeit without JSX and using plain JavaScript DOM manipulation methods.
Basic react commands
To create react app just use command npm create vite@latest
To run this app use npm run dev
To see the dist folder use npm run build
React Jargons
JSX=JavaScript XML (Let's you write HTML in JS). In JSX we have to use {} to run a js syntax.
Basic React code to create an counter
Assignment
After learning this much React, I created a business card assignment with the basic feature of filling out a form to create a business card. The data is stored in a MongoDB database and can be retrieved in the future using a card ID.
Github Link of the Assignment - Business Card Github
Adios!
Subscribe to my newsletter
Read articles from Binayak Shrestha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
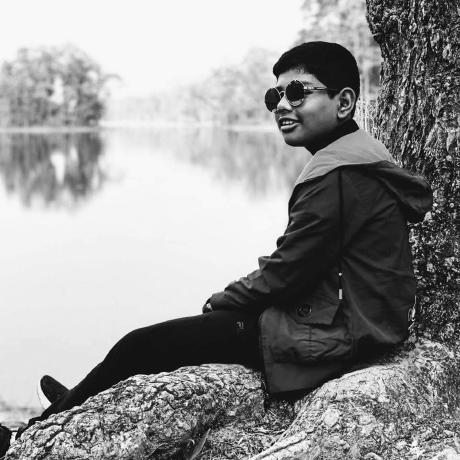