Step-by-Step Guide to Getting Started with GraphQL | Part- 1

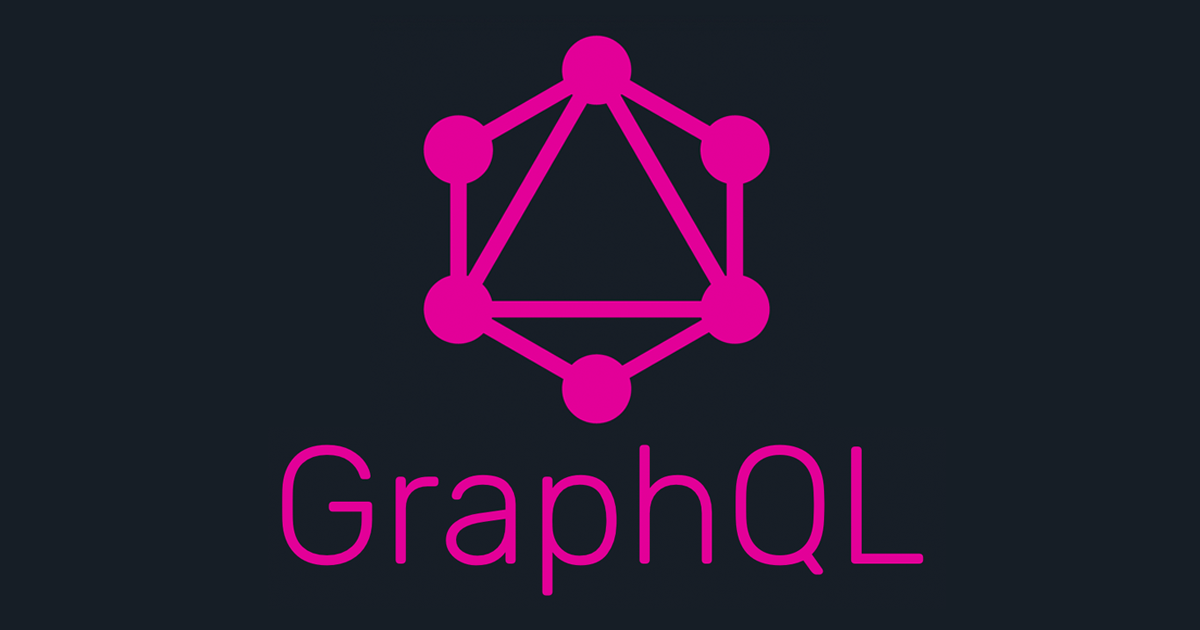
Course Introduction
GraphQL is becoming the new way to use APIs in modern web and mobile apps. However learning new things always takes time, and without getting hands dirty, it's hard to understand nuances of technology.
What is GraphQL?
GraphQL is a way to interact with an API. It's usually used over HTTP, where you POST
a "query" to one HTTP endpoint instead of accessing different endpoints for various resources.
GraphQL is designed for developers of web/mobile apps (HTTP clients) to be able to make API calls to fetch exactly the data they need from their backend APIs.
What are the similarities between GraphQL and REST?
Both GraphQL and REST are popular API architecture styles that enable the exchange of data between different services or applications in a client-server model.
REST and GraphQL allow you to create, modify, update, and delete data on a separate application, service, or module via API. APIs developed with REST are known as RESTful APIs or REST APIs. Those developed with GraphQL are simply GraphQL APIs.
Frontend and backend teams use these API architectures to create modular and accessible applications. Using an API architecture helps keep systems secure, modular, and scalable. It also makes systems more performant and easier to integrate with other systems.
Architecture
Both REST and GraphQL implement several common API architectural principles. For example, here are principles they share:
Both are stateless, so the server does not save response history between requests
Both use a client-server model, so requests from a single client result in replies from a single server
Both are HTTP-based, as HTTP is the underlying communication protocol
GraphQL Core Concepts
The content of a GraphQL request string is called the GraphQL document. The following is an example of a document:
{
author {
id
name
}
}
The string follows a syntax like the above which a GraphQL server or client can parse and validate. The above syntax uses a shorthand notation for a query operation.
GraphQL Operation
Just like in REST we perform operation like (GET, POST, PUT, DELETE). In GraphQL we have concept of Query of Mutations.
Query used (a read-only fetch)
Mutations used (to create/update/delete)
subscription (a long‐lived request that fetches data in response to source events.)
Note: A GraphQL document can contain one or more of these operations (i.e multiple queries/mutations/subscriptions).
Let's look an example of GraphQL document with an operation.
query {
author {
id
name
}
}
In the above example, the query operation selects information about the author
, including their id
and name
.
Arguments
Imagine fields as functions that return values. Now let's assume the function also accepts arguments that behave differently. author(limit: 5)
The author field accepts an argument limit
to limit the number of results returned. These arguments can be optional or mandatory and can appear on any field in the document.
query {
author(limit: 5) {
id
name
articles {
id
title
content
}
}
}
Variables
GraphQL queries can be parameterized with variables for reuse and easy construction of queries on the client-side.
In the above example, assume the limit parameter is configurable by the user viewing the page, then it would be easier to pass a variable to the field argument.
query ($limit: Int) {
author(limit: $limit) {
id,
name
}
}
The variable(s) is defined at the top of the query operation and the value for the variable can be sent by the client in a format that the server understands. Typically variables are represented in JSON like below.
{
limit: 5
}
Operation Name
When the document contains multiple operations, the server has to know which ones to execute and map the results back in the same order. For example
query fetchAuthor {
author(id: 1) {
name
profile_pic
}
}
query fetchAuthors {
author(limit: 5, order_by: { name: asc }) {
id
name
profile_pic
}
}
The above example have two operations- one for fetching a single author and second one for fetching multiple authors by ascending order.
Aliases
When you are fetching information about an author, let's say you have two images, different sizes and you have a field with an argument to do that. In this case, you cannot use the same field twice under the same selection set and hence an Alias
would be helpful to distinguish the two fields. look at the following example.
query fetchAuthor {
author(id: 1) {
name
profile_pic_large: profile_pic(size: "large")
profile_pic_small: profile_pic(size: "small")
}
}
Fragments
Fragments make GraphQL even more reusable. If there are some parts of your document that reuses the same set of fields on a given type, then fragment can be powerful.
fragment authorFields on author {
id
name
profile_pic
created_at
}
query fetchAuthor {
author(id: 1) {
...authorFields
}
}
query fetchAuthors {
author(limit: 5) {
...authorFields
}
}
Notice the usage of fragment here - ...authorFields
. This type of usage is called a spread fragment. There are also inline fragments where you don't explicitly declare fragment separately but use it inline in a query.
Directives
Directives are identifiers which add additional functionality without affecting the value of the response but can affect what response comes back to the client.
The identifier @
is optionally followed by a list of named arguments.
Some default server directives supported by GraphQL spec are:
@deprecated(reason: String) - marks the field as deprecated
@skip (if: Boolean) - Skips GraphQL execution for this field
@include (if: Boolean) - Calls resolver for an annotated field, if true.
For example:
query ($showFullname: Boolean!) {
author {
id
name
fullname @include(if: $showFullname)
}
}
In the above query, we include the field fullname, only if the condition is true (the condition can have its own logic depending on the app).
You can also use custom directives to handle other use cases.
Subscribe to my newsletter
Read articles from Shivam Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shivam Sharma
Shivam Sharma
I’m an open source enthusiast and I am passionate Web Development, DevOps & I enjoy learning new things.