"π Securing Kubernetes Deployments: A Hands-On Guide to ConfigMaps, Secrets, and RBAC π‘οΈ"
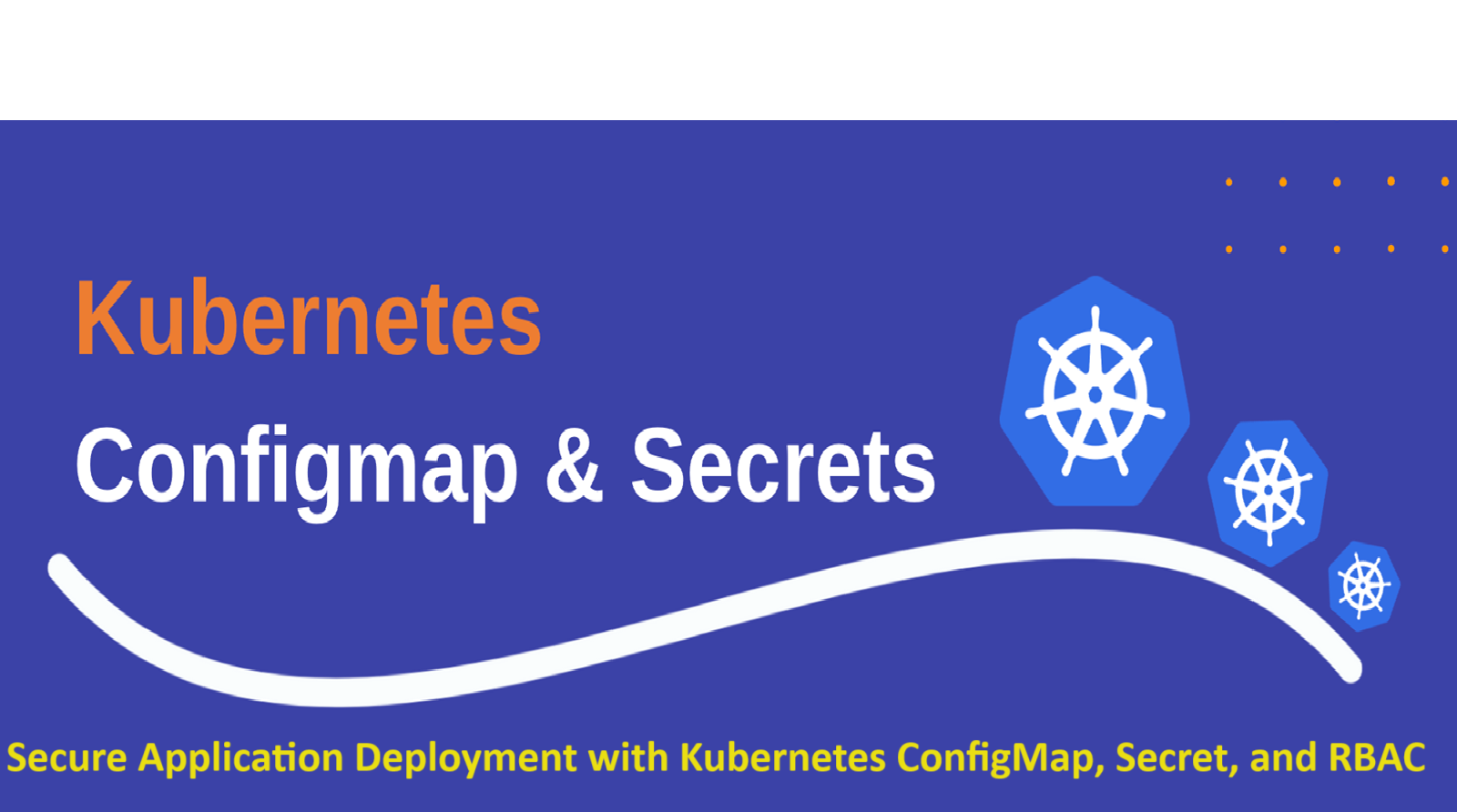
As a DevOps engineer, managing application configurations securely is crucial. Let's explore how Kubernetes helps us achieve this with ConfigMaps and Secrets.
π ConfigMaps: Your Configuration Companion
What are ConfigMaps?
Kubernetes objects for storing non-sensitive configuration data
Replace traditional .env files in containerized environments
Use Case: Imagine a backend app communicating with a database. You need to store: π Database hostname/IP π€ Username π’ Port π Network type
Why ConfigMaps?
Easily updatable without rebuilding containers
Centralized configuration management
Mountable into pods as environment variables or files
Creating a ConfigMap:
yamlCopyapiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
DB_HOST: "db.example.com"
DB_PORT: "5432"
APP_MODE: "production"
π Secrets: Safeguarding Sensitive Data
Why Secrets?
Enhanced security for sensitive information (passwords, API keys)
Data is encrypted before storage in etcd
Use Case: Storing database passwords, API tokens, etc.
Creating a Secret:
yamlCopyapiVersion: v1
kind: Secret
metadata:
name: app-secret
type: Opaque
data:
DB_PASSWORD: cGFzc3dvcmQxMjM= # base64 encoded
π‘οΈ RBAC: Controlling Access
Role-Based Access Control (RBAC):
Limits who can access and view Secrets
Implements the principle of least privilege
Setting up RBAC:
Create a ServiceAccount
Define a Role with specific permissions
Bind the Role to the ServiceAccount
Project: Secure Application Deployment with Kubernetes ConfigMap, Secret, and RBAC
This project will demonstrate how to use these Kubernetes features to manage configuration data, sensitive information, and access control in a cluster.
Objective: Deploy a web application that uses ConfigMap for configuration, Secret for sensitive data, and RBAC for access control.
Prerequisites:
A running Kubernetes cluster
kubectl CLI tool installed and configured
Basic understanding of Kubernetes concepts
Step 1: Create a Namespace
First, let's create a dedicated namespace for our project:
kubectl create namespace secure-app
kubectl config set-context --current --namespace=secure-app
Step 2: Create a ConfigMap
Create a file named app-config.yaml:
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
APP_COLOR: blue
APP_MODE: production
Apply the ConfigMap:
kubectl apply -f app-config.yaml
Step 3: Create a Secret
Create a file named app-secret.yaml:
apiVersion: v1
kind: Secret
metadata:
name: app-secret
type: Opaque
data:
DB_PASSWORD: cGFzc3dvcmQxMjM= # base64 encoded "password123"
Apply the Secret:
kubectl apply -f app-secret.yaml
Step 4: Create a Deployment
Create a file named app-deployment.yaml:
apiVersion: apps/v1
kind: Deployment
metadata:
name: secure-app
spec:
replicas: 1
selector:
matchLabels:
app: secure-app
template:
metadata:
labels:
app: secure-app
spec:
containers:
- name: secure-app
image: nginx:latest
ports:
- containerPort: 80
env:
- name: APP_COLOR
valueFrom:
configMapKeyRef:
name: app-config
key: APP_COLOR
- name: APP_MODE
valueFrom:
configMapKeyRef:
name: app-config
key: APP_MODE
- name: DB_PASSWORD
valueFrom:
secretKeyRef:
name: app-secret
key: DB_PASSWORD
Apply the Deployment:
kubectl apply -f app-deployment.yaml
Step 5: Create a Service
Create a file named app-service.yaml:
apiVersion: v1
kind: Service
metadata:
name: secure-app-service
spec:
selector:
app: secure-app
ports:
- protocol: TCP
port: 80
targetPort: 80
Apply the Service:
kubectl apply -f app-service.yaml
Step 6: Set up RBAC
First, create a ServiceAccount:
kubectl create serviceaccount app-sa
Now, create a Role for reading ConfigMaps and Secrets. Create a file named app-role.yaml:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
name: config-reader
rules:
- apiGroups: [""]
resources: ["configmaps", "secrets"]
verbs: ["get", "list"]
Apply the Role:
kubectl apply -f app-role.yaml
Bind the Role to the ServiceAccount. Create a file named app-rolebinding.yaml:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-config-binding
subjects:
- kind: ServiceAccount
name: app-sa
roleRef:
kind: Role
name: config-reader
apiGroup: rbac.authorization.k8s.io
Apply the RoleBinding:
kubectl apply -f app-rolebinding.yaml
Step 7: Update the Deployment to use the ServiceAccount
Edit the app-deployment.yaml file and add the serviceAccountName field under spec.template.spec:
spec:
template:
spec:
serviceAccountName: app-sa
containers:
...
Apply the updated Deployment:
kubectl apply -f app-deployment.yaml
Step 8: Verify the Setup
Check if the pod is running:
kubectl get pods
View the pod's logs:
kubectl logs Secure-app-*********
Describe the pod to see if the environment variables are set correctly:
kubectl describe pod Secure-app-*****
Test RBAC by trying to read the ConfigMap and Secret using the app-sa ServiceAccount:
kubectl auth can-i get configmaps --as=system:serviceaccount:secure-app:app-sa
kubectl auth can-i get secrets --as=system:serviceaccount:secure-app:app-sa
Both commands should return "yes".
AWS
This project demonstrates how to use Kubernetes ConfigMaps for configuration data, Secrets for sensitive information, and RBAC for access control. The application can now securely access its configuration and sensitive data, while the RBAC setup ensures that only authorized entities can read this information.
π Conclusion: Mastering Kubernetes Security
Congratulations! You've just walked through a comprehensive guide to securing your Kubernetes deployments. Let's recap the key takeaways:
π ConfigMaps provide a flexible way to manage non-sensitive configuration data, allowing for easy updates without rebuilding containers.
π Secrets offer enhanced security for sensitive information, encrypting data before storing it in etcd.
π‘οΈ RBAC enables fine-grained access control, ensuring that only authorized entities can access specific resources.
By implementing these features, you've significantly improved your application's security posture:
β Separated configuration from code β Protected sensitive data β Implemented principle of least privilege
Remember, security is an ongoing process. Regularly review and update your configurations, rotate secrets, and stay informed about Kubernetes best practices.
π Next Steps:
Explore more advanced RBAC configurations
Implement network policies for additional security
Consider using external secret management systems for enterprise-scale deployments
By mastering these Kubernetes features, you're well on your way to creating more secure, scalable, and maintainable applications in the cloud-native world.
Happy deploying, and stay secure! ππ³
Thank you for joining me on this journey through the world of cloud computing! Your interest and support mean a lot to me, and I'm excited to continue exploring this fascinating field together. Let's stay connected and keep learning and growing as we navigate the ever-evolving landscape of technology.
LinkedIn Profile: https://www.linkedin.com/in/prasad-g-743239154/
Github Url-https://github.com/sprasadpujari/Kubernative_Projects/tree/main/Kubernetes_ConfigMap_Secret_RBAC
Feel free to reach out to me directly at spujari.devops@gmail.com. I'm always open to hearing your thoughts and suggestions, as they help me improve and better cater to your needs. Let's keep moving forward and upward!
If you found this blog post helpful, please consider showing your support by giving it a round of applauseπππ. Your engagement not only boosts the visibility of the content, but it also lets other DevOps and Cloud Engineers know that it might be useful to them too. Thank you for your support! π
Thank you for reading and happy deploying! π
Best Regards,
Sprasad
Subscribe to my newsletter
Read articles from Sprasad Pujari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Sprasad Pujari
Sprasad Pujari
Greetings! I'm Sprasad P, a DevOps Engineer with a passion for optimizing development pipelines, automating processes, and enabling teams to deliver software faster and more reliably.