Introduction to Variables in Cairo
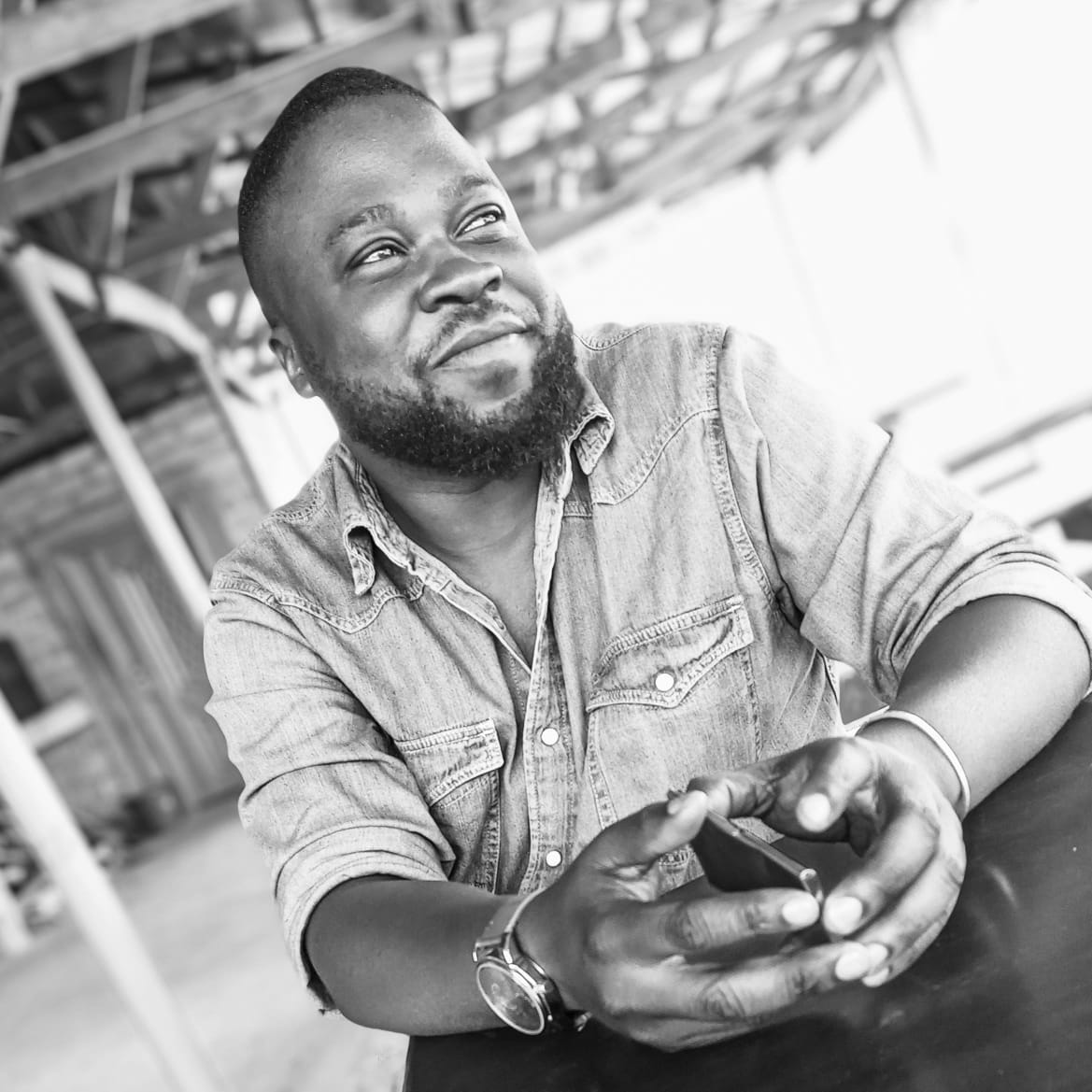
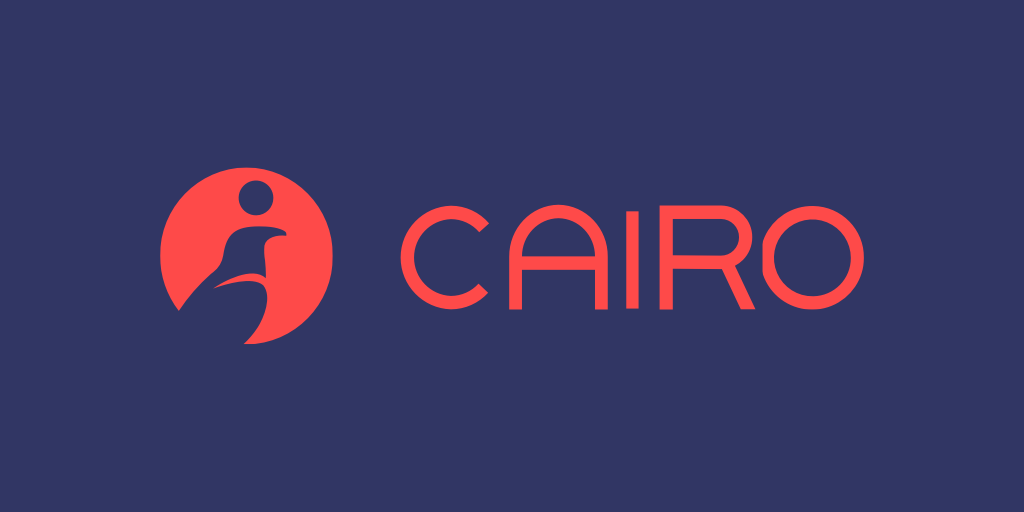
Cairo is a Rust-inspired language for creating provable programs for general computation. Cairo is also the native smart contract language for Starknet.
Sharing similarities with Rust, Starknet developers are assured of a safe and terrific developer experience.
In this article, we will be talking about variables one of the building blocks in any programming language.
Let’s get on with it 🚀
Think of variables like your wallet if you use one. Your wallet keeps your money and cards safe. Anytime you require cash or your card all you have to do is reach for your wallet and you can access its contents.
A variable works in the same way. It is a memory location that stores information and can be called at any time to access whatever is stored in it.
You can declare a variable in Cairo by using the let
keyword followed by the variable name and the data type.
let variable:u256;
It is important to note that the default behavior of variables in Cairo uses a concept called immutable memory model. This means once a value is bound to a name(i.e. a memory location is written to) the value cannot be changed it can only be read from.
Let’s illustrate this with the below code.
fn main() {
let x = 34;
println!("The value of x is:{}", x)
x = 45;
println!("The value of x is :{}", x)
}
Try running the code using scarb cairo-run
and you will be greeted by the below error. The below is a compile error telling you what you are trying to do is not allowed making it easy to catch bugs during compile time.
At this point, you might be scratching your head saying what if I want to be able to change the value? Cairo has a nice keyword you can use to make that happen.
That keyword is the mut
keyword. This allows you to change the value associated with the variable without getting a compile error.
Let’s see this in action
fn main() {
let mut x = 45;
println!("The value of x is: {}", x);
x = 35;
println!("The value of x is: {}", x);
}
Run with scarb cairo-run
.
You can see you did not encounter a compile error. This is because the mut keyword allows you to change the value from 45 to 35 without any hassles.
Another concept worth mentioning since we are talking about variables in constants. As the name implies constants are values bound to a name and cannot be changed.
To declare a constant the const
keyword is used followed by the name of the constant variable which must be in capital letters and the underscore is used for longer words.
const ONE_HOUR_IN_SECONDS: u32 = 3600:
fn main() {
println!("This is the value of the constant:{}", ONE_HOUR_IN_SECONDS);
}
Not so fast, the mut keyword does not work on constants. Let’s try this and see what happens.
const mut ONE_HOUR_IN_SECONDS: u32 = 3600:
fn main() {
println!("This is the value of the constant:{}", ONE_HOUR_IN_SECONDS);
}
Run with scarb cairo-run
.
You get the above error and it says "mut is a reserved Identifier". Meaning it cannot be used with constants.
That's all for variables in Cairo. Other than an advanced concept called shadowing all we have learned is more than enough to get you going with Cairo and writing smart contracts for Starknet.
Any questions you can drop them in the comments I promise to respond to all.
Subscribe to my newsletter
Read articles from Emmanuel Soetan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
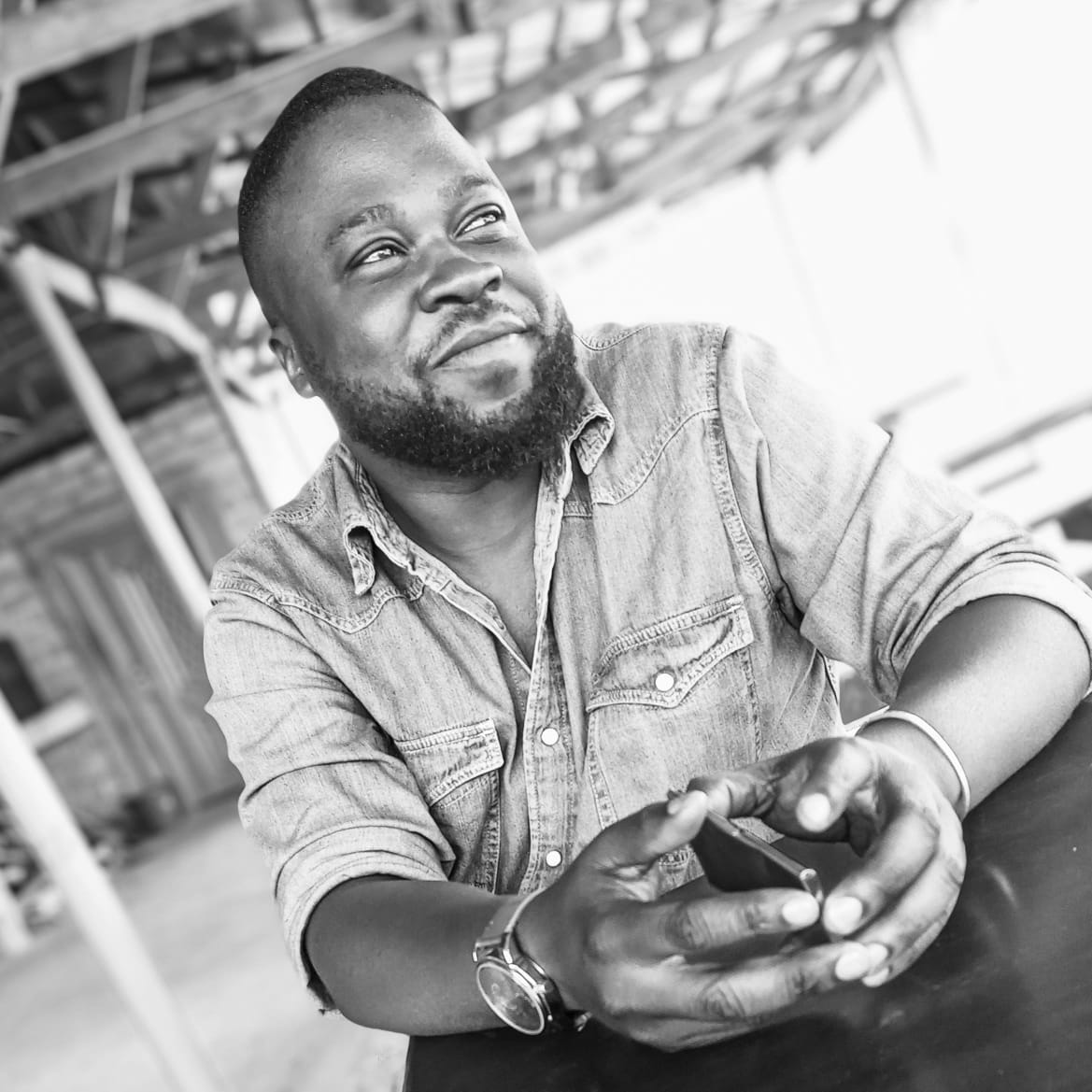
Emmanuel Soetan
Emmanuel Soetan
Maximizing user experience with elegant web solutions. Passionate software engineer with a demonstrated history of solving complex problems using HTML, CSS, JavaScript, React, nextJs, Redux, Firebase, MongoDB, node js, express js, tailwindCss, Material UI Committed to being a valuable team player and always willing to lend a hand to teammates when needed. I thrive in a collaborative and fast-paced environment and am always looking for new challenges to help me grow and learn. Let's connect and discuss how I can make a significant contribution to your team.