Understanding PHP Conditional Statements: if, else, and switch Explained

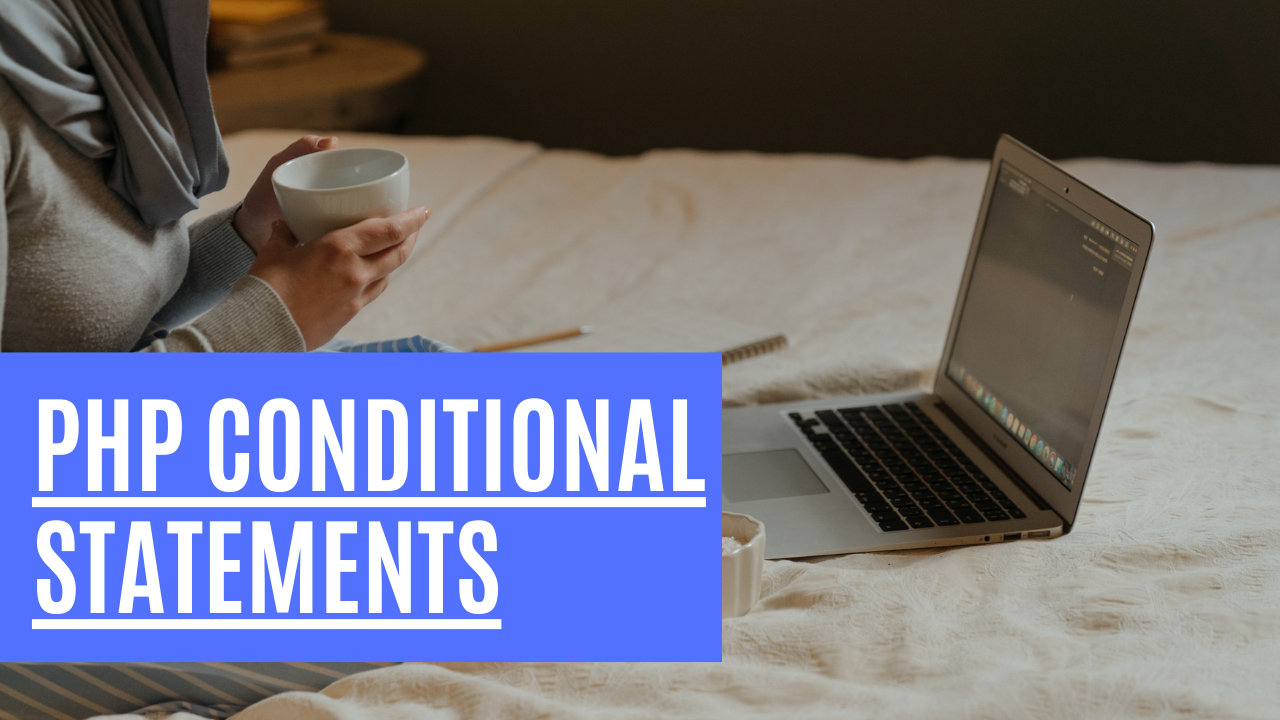
Today, we will explore PHP conditional statements, which allow you to execute different blocks of code based on certain conditions. Conditional statements are fundamental in programming as they enable decision-making in your code. Let's dive into the various conditional statements available in PHP.
Types of PHP Conditional Statements
if Statement
if...else Statement
if...elseif...else Statement
switch Statement
1. if Statement
The if
statement executes a block of code if a specified condition is true.
Syntax:
if (condition) {
// Code to be executed if the condition is true
}
Example:
<?php
$age = 20; // Assign the value 20 to the variable $age
if ($age >= 18) { // Check if $age is greater than or equal to 18
echo "You are an adult."; // If the condition is true, output "You are an adult."
}
// Outputs: You are an adult.
?>
2. if...else Statement
The if...else
statement executes one block of code if a condition is true and another block of code if the condition is false.
Syntax:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
Example:
<?php
$age = 16; // Assign the value 16 to the variable $age
if ($age >= 18) { // Check if $age is greater than or equal to 18
// $age >= 18 i.e. 16 >= 18 is false
echo "You are an adult."; // This line will not be executed since the condition is false
} else {
// If condition is false, the else block will run
echo "You are a minor."; // This line will be executed
}
// Outputs: You are a minor.
?>
3. if...elseif...else Statement
The if...elseif...else
statement allows you to test multiple conditions. If one of the conditions is true, the corresponding block of code is executed.
Syntax:
if (condition1) {
// Code to be executed if condition1 is true
} elseif (condition2) {
// Code to be executed if condition2 is true
} else {
// Code to be executed if neither condition1 nor condition2 is true
}
Example:
<?php
$score = 85; // Assign the value 85 to the variable $score
if ($score >= 90) {
// $score >= 90 i.e. 85 >= 90 is false
echo "Grade: A"; // This line will not be executed since the condition is false
} elseif ($score >= 80) {
// $score >= 80 i.e. 85 >= 80 is true
echo "Grade: B"; // This line will be executed since the condition is true
} elseif ($score >= 70) {
// $score >= 70 i.e. 85 >= 70 is true but the previous condition was met, so this block is not executed
echo "Grade: C";
} else {
// If all conditions are false, the else block will run
echo "Grade: F";
}
// Outputs: Grade: B
?>
4. switch Statement
The switch
statement is used to perform different actions based on different conditions. It is a more efficient way to compare a single variable against multiple values.
Syntax:
switch (n) {
case label1:
// Code to be executed if n == label1
break;
case label2:
// Code to be executed if n == label2
break;
// You can have any number of case statements
default:
// Code to be executed if n doesn't match any case
}
Example:
<?php
$day = "Tuesday"; // Assign the value "Tuesday" to the variable $day
switch ($day) { // Start switch statement to check the value of $day
case "Monday": // Check if $day is "Monday"
echo "Today is Monday."; // This line will not be executed since $day is not "Monday"
break; // Exit switch statement
case "Tuesday": // Check if $day is "Tuesday"
echo "Today is Tuesday."; // This line will be executed since $day is "Tuesday"
break; // Exit switch statement
case "Wednesday": // Check if $day is "Wednesday"
echo "Today is Wednesday."; // This line will not be executed since $day is not "Wednesday"
break; // Exit switch statement
default: // Default case if none of the above cases match
echo "Unknown day."; // This line will not be executed since one of the cases matched
}
// Outputs: Today is Tuesday.
?>
Best Practices for Conditional Statements
Use Braces for All Control Structures:
- Always use braces
{}
forif
,else
, andelseif
blocks, even for single-line statements. This improves readability and prevents errors.
- Always use braces
if ($condition) {
// Do something
}
Keep Conditions Simple:
- Break down complex conditions into smaller, understandable parts.
<?php
$isAdult = $age >= 18; // Assign true to $isAdult if $age is greater than or equal to 18, otherwise false
$hasPermission = $isAdmin || $isAdult; // Assign true to $hasPermission if $isAdmin or $isAdult is true
if ($hasPermission) {
// Do something if $hasPermission is true
}
?>
Avoid Deep Nesting:
Refactor deeply nested code into functions to improve readability and maintainability.
Note: The topic of functions will be covered in upcoming blogs.
<?php
function processOrder($order) {
if ($order->isValid()) { // Check if the order is valid
if ($order->isPaid()) { // Check if the order is paid
// Process the order if it is valid and paid
}
}
}
?>
Use
switch
for Multiple Conditions on a Single Variable:- When comparing a single variable against multiple values, use a
switch
statement for better readability and efficiency.
- When comparing a single variable against multiple values, use a
switch ($status) {
case "active":
// Code for active status
break;
case "inactive":
// Code for inactive status
break;
default:
// Code for unknown status
}
Conclusion
Today, we explored PHP conditional statements, including if
, if...else
, if...elseif...else
, and switch
statements. Conditional statements are crucial for making decisions in your code based on different conditions.
Subscribe to my newsletter
Read articles from Saurabh Damale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saurabh Damale
Saurabh Damale
Hello there! ๐ I'm Saurabh Kailas Damale, a passionate Full Stack Developer with a strong background in creating dynamic and responsive web applications. I thrive on turning complex problems into elegant solutions, and I'm always eager to learn new technologies and tools to enhance my development skills.