The Evolution from Imperative to Declarative Programming: A Deep Dive into Modern Software Paradigms
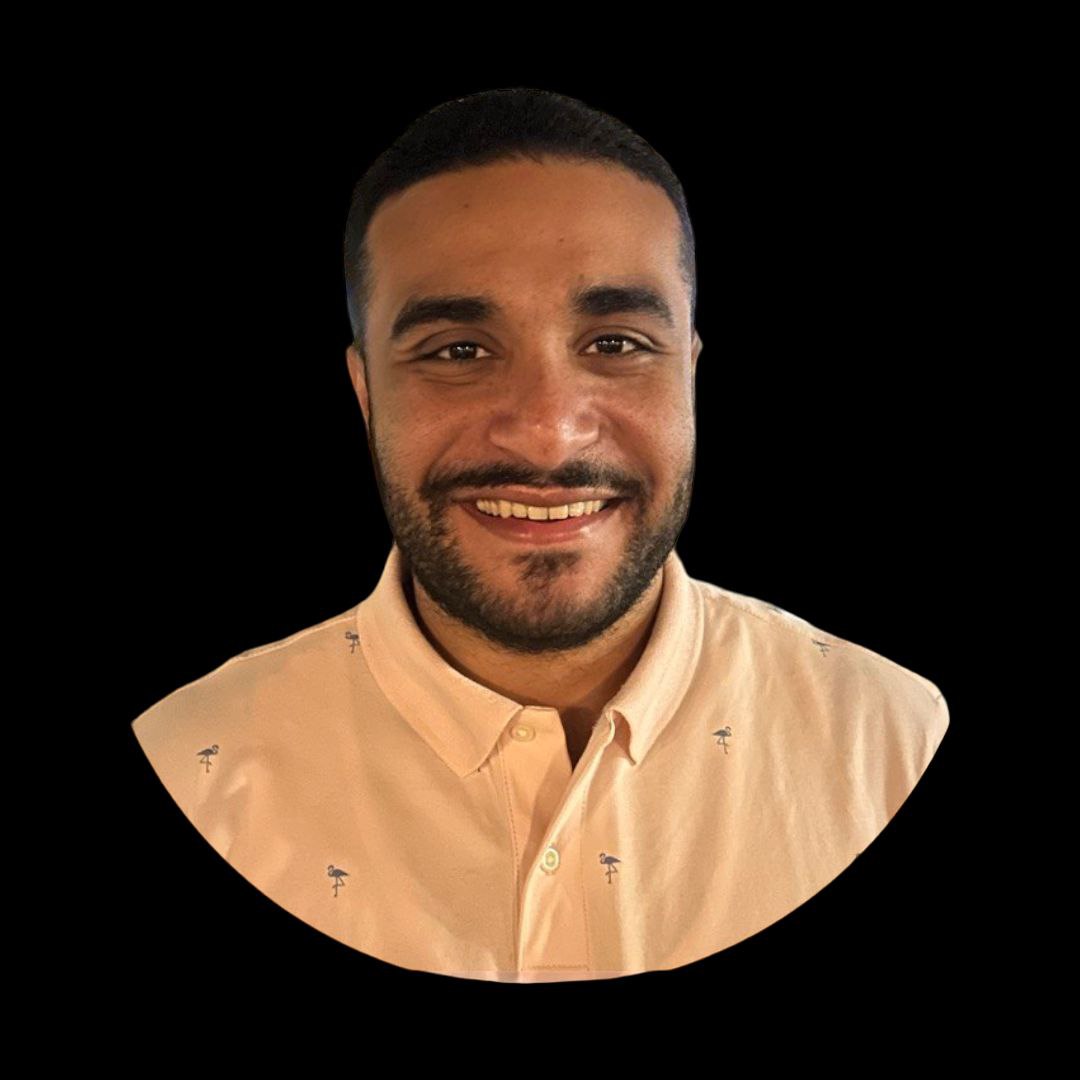
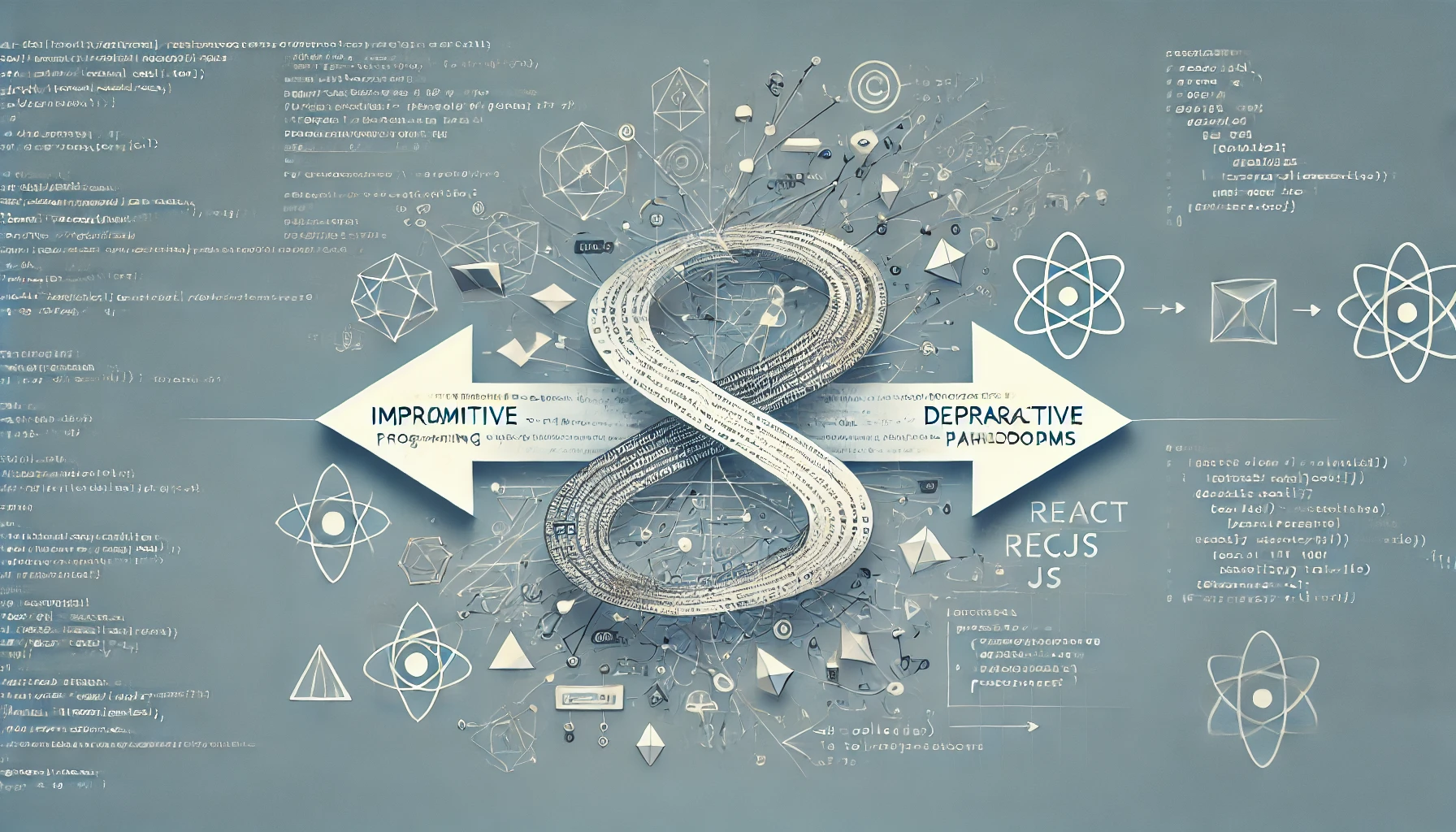
In the ever-evolving landscape of software development, the shift from imperative to declarative programming paradigms marks a significant milestone in how we approach problem-solving in code. This transition is not merely a change in coding style; it reflects a fundamental shift in how developers interact with technology, where the emphasis moves from describing how a program should accomplish tasks to specifying what the program should achieve. This article delves into the history, differences, and reasons behind this shift, highlighting ReactJS's role in popularizing the declarative paradigm.
Understanding Imperative and Declarative Paradigms
Imperative Programming
Imperative programming is characterized by code that explicitly instructs the machine on how to perform operations. It’s like giving step-by-step directions to reach a destination. This approach has its roots in procedural programming, which directly manipulates the machine’s state through detailed instructions. Languages like C, Java, and Python support this style, requiring developers to manage numerous details about program state, control flow, and more.
Declarative Programming
Conversely, declarative programming focuses on what the outcome should be rather than how to achieve it. This paradigm is akin to describing the characteristics of the destination without worrying about the specific path to get there. SQL is a prime example, where one describes the data they want without detailing the retrieval process. HTML and CSS are also declarative, specifying what should appear on a web page and its styling without dictating the step-by-step rendering process.
Historical Shift Towards Declarative Paradigms
The movement towards declarative programming paradigms can be traced back to the increasing complexity of systems and the need for higher abstraction levels. As applications grew more sophisticated, the imperative approach's limitations became apparent—more code to handle more scenarios led to higher maintenance and greater potential for errors.
Functional programming languages like Haskell and Erlang, which emphasize immutability and side-effect-free functions, have long championed the declarative approach. These languages allow developers to write expressive, concise code that is easier to reason about than typical imperative code.
ReactJS: A Standard-Bearer for Declarative UIs
ReactJS, developed by Facebook, stands out as a major proponent of declarative programming in the creation of user interfaces. React allows developers to describe their UIs in terms of a final state, like a function that describes the UI at any point in time for given inputs. This approach, known as reactive programming, abstracts away the complex behaviors associated with real-time user environments.
function App() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In this React example, the state (count
) and UI are tightly coupled in a way that abstracts the actual DOM manipulations. The developer is freed from directly handling the DOM or specifying how changes should occur, focusing instead on what the UI should look like at any given state.
Why the Shift Happened
The shift towards declarative programming has been driven by the need for more robust, scalable, and maintainable applications. Declarative paradigms reduce the cognitive load on developers, allowing them to define complex UI states and behaviors with less code, which is generally easier to debug and test.
React and Inversion of Control (IoC)
React's model, which could be seen as an inversion of control, delegates control of the UI rendering and state management to the framework itself. This raises an interesting question for developers experienced with Java Spring and its IoC container: Does React's approach stem from the same software engineering principles that advocate for reduced control by the developer and increased control by the framework?
IoC in Spring and declarative UIs in React both abstract away complex implementations, allowing developers to focus on higher-level business logic. This comparison invites further reflection on how modern development frameworks and libraries are increasingly handling more of the 'heavy lifting', letting developers specify higher-level intentions rather than lower-level operations.
Conclusion
The shift from imperative to declarative paradigms represents a fundamental change in how developers write software. ReactJS exemplifies how powerful the declarative approach can be in building interactive, stateful web applications. As the software industry continues to evolve, it's worth pondering how these paradigms influence the tools and frameworks we choose. For seasoned developers, especially those skilled in frameworks like Java Spring, how do you see these shifts influencing your approach to software architecture and development?
By considering these paradigms, we not only navigate the current landscape but also prepare for future innovations that might further abstract the complexities of software development.
Subscribe to my newsletter
Read articles from Mido Sayed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
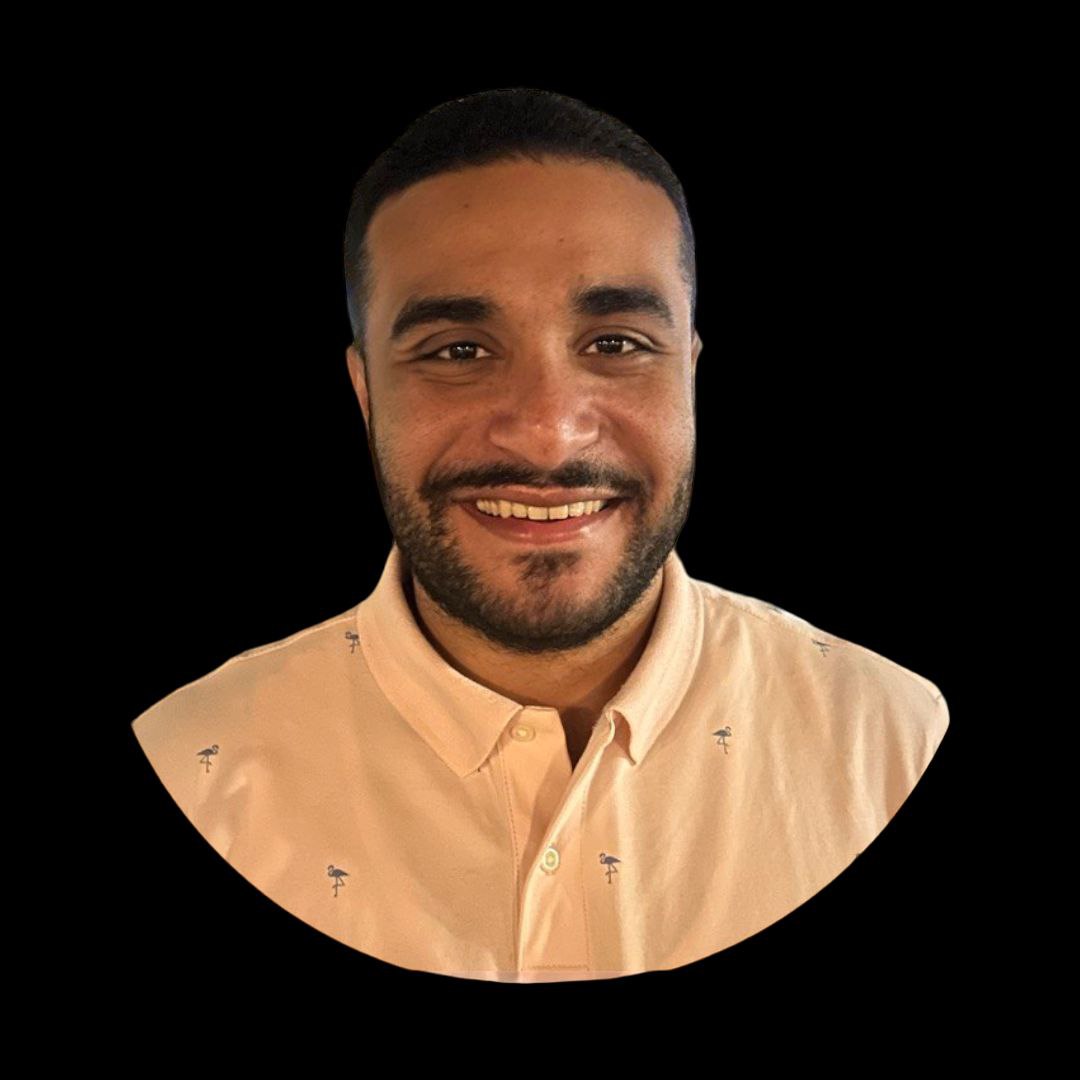
Mido Sayed
Mido Sayed
Certified AWS Solutions Architect, Software Developer, and Instructor with a passion for shaping the next-gen tech workforce. 10 years of dev experience, 6 years in curriculum development and teaching. MSc in Web Science, BSc in Computer Science. Experienced in web, data, and instructional design. Let's create tech wonders together!