Day 9 Task: Shell Scripting Challenge Directory Backup with Rotation

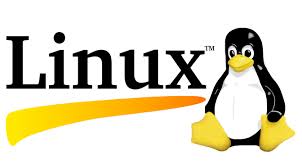
Bash Scripting Challenge: Backup with Rotation
Welcome to this exciting Bash scripting challenge! Today, we'll create a script that performs a backup of a specified directory, includes timestamped backup folders, and implements a rotation mechanism to retain only the last 3 backups. Let's dive in!
Challenge Description
Your task is to create a bash script that takes a directory path as a command-line argument and performs a backup of the directory. The script will:
Create timestamped backup folders.
Copy all files from the specified directory into the backup folder.
Implement a rotation mechanism to keep only the last 3 backups, removing the oldest backups.
Example Usage
Assume the script is named backup_with_
rotation.sh
. Below is an example of how it will look when executed on different dates.
First Execution (2023-07-30):
$ ./backup_with_rotation.sh /home/user/documents
Output:
Backup created: /home/user/documents/backup_2023-07-30_12-30-45
Backup created: /home/user/documents/backup_2023-07-30_15-20-10
Backup created: /home/user/documents/backup_2023-07-30_18-40-55
Directory Contents After Execution:
backup_2023-07-30_12-30-45
backup_2023-07-30_15-20-10
backup_2023-07-30_18-40-55
file1.txt
file2.txt
...
Second Execution (2023-08-01):
$ ./backup_with_rotation.sh /home/user/documents
Output:
Backup created: /home/user/documents/backup_2023-08-01_09-15-30
Directory Contents After Execution:
backup_2023-07-30_15-20-10
backup_2023-07-30_18-40-55
backup_2023-08-01_09-15-30
file1.txt
file2.txt
...
The Script
Here's the complete backup_with_
rotation.sh
script that incorporates all the tasks mentioned above.
#!/bin/bash
# Check if the directory path is provided as a command-line argument
if [ -z "$1" ]; then
echo "Usage: $0 <directory_path>"
exit 1
fi
# Assign the directory path to a variable
DIR=$1
# Create a timestamp for the backup folder name
TIMESTAMP=$(date +"%Y-%m-%d_%H-%M-%S")
# Create the backup folder inside the specified directory
BACKUP_DIR="$DIR/backup_$TIMESTAMP"
mkdir -p "$BACKUP_DIR"
# Copy all files from the specified directory into the backup folder
cp -r "$DIR"/* "$BACKUP_DIR"
# Output the backup creation message
echo "Backup created: $BACKUP_DIR"
# Implement the rotation mechanism to keep only the last 3 backups
cd "$DIR"
BACKUPS=($(ls -d backup_* | sort))
# Check if there are more than 3 backups
if [ ${#BACKUPS[@]} -gt 3 ]; then
# Calculate the number of backups to remove
REMOVE_COUNT=$((${#BACKUPS[@]} - 3))
# Remove the oldest backups
for ((i=0; i<REMOVE_COUNT; i++)); do
rm -rf "${BACKUPS[$i]}"
echo "Removed old backup: ${BACKUPS[$i]}"
done
fi
Step-by-Step Explanation
Check for Command-line Argument: The script checks if a directory path is provided as an argument. If not, it displays usage instructions and exits.
Assign Directory Path to Variable: The provided directory path is assigned to the variable
DIR
.Create Timestamp: A timestamp is generated for naming the backup folder using the
date
command.Create Backup Folder: The script creates a backup folder inside the specified directory.
Copy Files to Backup Folder: All files from the specified directory are copied into the backup folder.
Output Backup Creation Message: The script outputs a message indicating the backup creation.
Implement Rotation Mechanism: The script navigates to the specified directory and lists all backup folders. If there are more than 3 backups, it calculates the number of backups to remove and deletes the oldest backups.
Example Screenshots
Execution and Output:
Directory Contents After Execution:
Conclusion
This script helps in automating the backup process and ensures that only the last 3 backups are retained. It's a simple yet effective way to manage backups in a directory. Happy scripting! ๐
Subscribe to my newsletter
Read articles from Himanshu Palhade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
