Escaping Callback Hell: A Developer's Guide
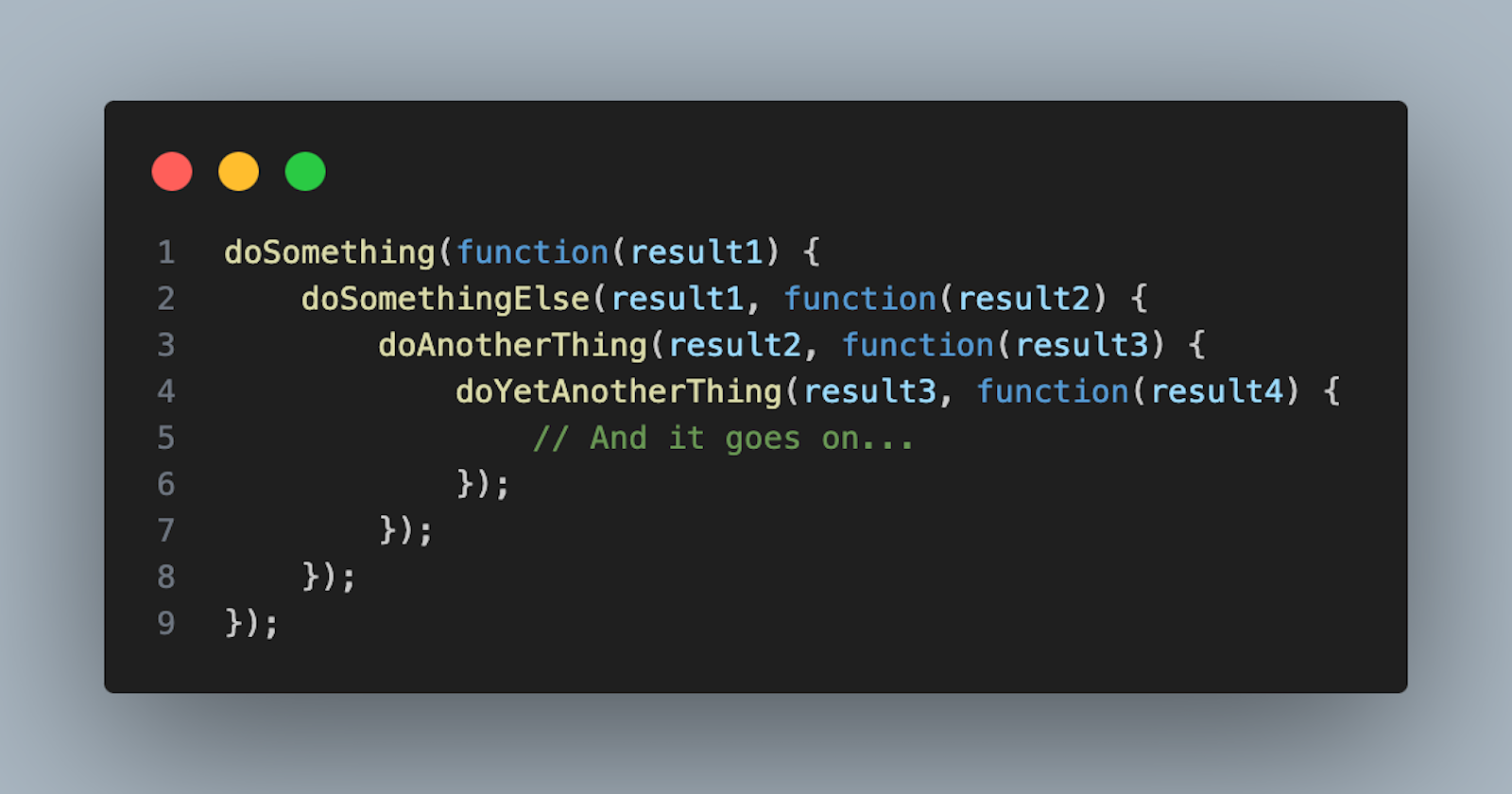
As a developer, you might have found yourself tangled in the messy, complicated world of nested callbacks, commonly referred to as "Callback Hell." This issue arises when you have multiple asynchronous operations that depend on each other, leading to deeply nested and hard-to-read code. But fear not! There are ways to escape this hell and write cleaner, more maintainable code. In this blog, we'll explore the causes of Callback Hell, share some real-life examples, and discuss effective strategies to escape it.
What is Callback Hell?
Callback Hell, also known as "Pyramid of Doom," occurs when you use multiple nested callbacks in JavaScript, leading to code that is difficult to read and maintain. Here's a classic example:
doSomething(function(result1) {
doSomethingElse(result1, function(result2) {
doAnotherThing(result2, function(result3) {
doYetAnotherThing(result3, function(result4) {
// And it goes on...
});
});
});
});
In this example, each callback relies on the result of the previous one, creating a pyramid-like structure that can quickly become unmanageable.
Real-Life Example: Making a Sandwich
Imagine you're making a sandwich. You can't add the toppings until you've put on the condiments, and you can't put on the condiments until you've sliced the bread. Each step depends on the completion of the previous step, much like nested callbacks in code.
sliceBread(function(slicedBread) {
addCondiments(slicedBread, function(condimentsAdded) {
addToppings(condimentsAdded, function(toppingsAdded) {
serveSandwich(toppingsAdded, function(sandwichServed) {
console.log("Sandwich is ready to eat!");
});
});
});
});
How to Escape Callback Hell
1. Use Named Functions
Instead of using anonymous functions, you can create named functions. This approach not only makes the code more readable but also easier to debug.
function sliceBread(callback) {
// slicing bread
callback(slicedBread);
}
function addCondiments(slicedBread, callback) {
// adding condiments
callback(condimentsAdded);
}
function addToppings(condimentsAdded, callback) {
// adding toppings
callback(toppingsAdded);
}
function serveSandwich(toppingsAdded, callback) {
console.log("Sandwich is ready to eat!");
}
sliceBread(function(slicedBread) {
addCondiments(slicedBread, function(condimentsAdded) {
addToppings(condimentsAdded, function(toppingsAdded) {
serveSandwich(toppingsAdded);
});
});
});
2. Promises
Promises are a modern alternative to callbacks that help you avoid deep nesting. They allow you to chain asynchronous operations and handle errors more gracefully.
sliceBread()
.then(addCondiments)
.then(addToppings)
.then(serveSandwich)
.catch(error => {
console.error("Something went wrong:", error);
});
Real-Life Example: Promises for Making a Sandwich
function sliceBread() {
return new Promise((resolve, reject) => {
// slicing bread
resolve(slicedBread);
});
}
function addCondiments(slicedBread) {
return new Promise((resolve, reject) => {
// adding condiments
resolve(condimentsAdded);
});
}
function addToppings(condimentsAdded) {
return new Promise((resolve, reject) => {
// adding toppings
resolve(toppingsAdded);
});
}
function serveSandwich(toppingsAdded) {
return new Promise((resolve, reject) => {
console.log("Sandwich is ready to eat!");
resolve();
});
}
sliceBread()
.then(addCondiments)
.then(addToppings)
.then(serveSandwich)
.catch(error => {
console.error("Something went wrong:", error);
});
3. Async/Await
Async/Await is syntactic sugar over promises, making asynchronous code look and behave more like synchronous code. It greatly improves readability and error handling.
async function makeSandwich() {
try {
const slicedBread = await sliceBread();
const condimentsAdded = await addCondiments(slicedBread);
const toppingsAdded = await addToppings(condimentsAdded);
await serveSandwich(toppingsAdded);
console.log("Sandwich is ready to eat!");
} catch (error) {
console.error("Something went wrong:", error);
}
}
makeSandwich();
Conclusion
Callback Hell can turn your code into an unmanageable mess, but by using named functions, Promises, or Async/Await, you can escape this chaos and write cleaner, more maintainable code. Each of these methods has its own benefits and use cases, so choose the one that best fits your project.
Remember, writing readable and maintainable code is crucial for long-term success in software development. So, don't let Callback Hell trap you—use these strategies to keep your code clean and efficient.
Liked reading?
Let me know in the comments section, and do like my blog & subscribe to my newsletter.
Subscribe to my newsletter
Read articles from Animesh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Animesh Kumar
Animesh Kumar
I’m a software developer who loves problem-solving, data structures, algorithms, and competitive coding. I’ve a keen interest in product development. I’m passionate about AI, ML, and Python. I love exploring new ideas and enjoy innovating with advanced tech. I am eager to learn and contribute effectively to teams.