How to Optimize Web Apps Using Lazy Loading and Code Splitting
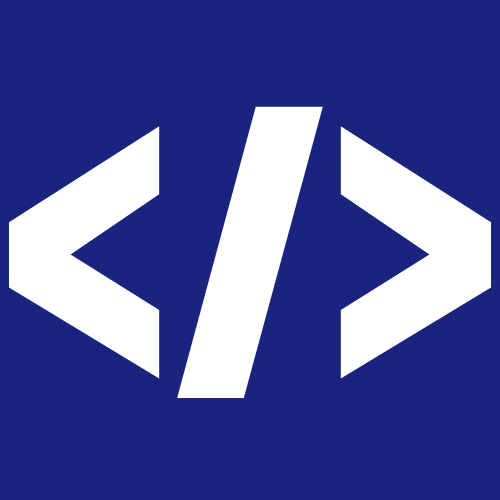
Table of contents
- Understanding Lazy Loading
- FAQs about Lazy Loading and Code Splitting
- What is Lazy Loading?
- How does Lazy Loading improve performance?
- How can I implement Lazy Loading in React?
- What is Code Splitting?
- How does Code Splitting enhance web application performance?
- How can I implement Code Splitting in React?
- Can Lazy Loading and Code Splitting be used together?
- What are the benefits of combining Lazy Loading and Code Splitting?
- What are some real-world use cases for Lazy Loading and Code Splitting?
- How do these techniques affect SEO?
- What tools and libraries support Lazy Loading and Code Splitting?
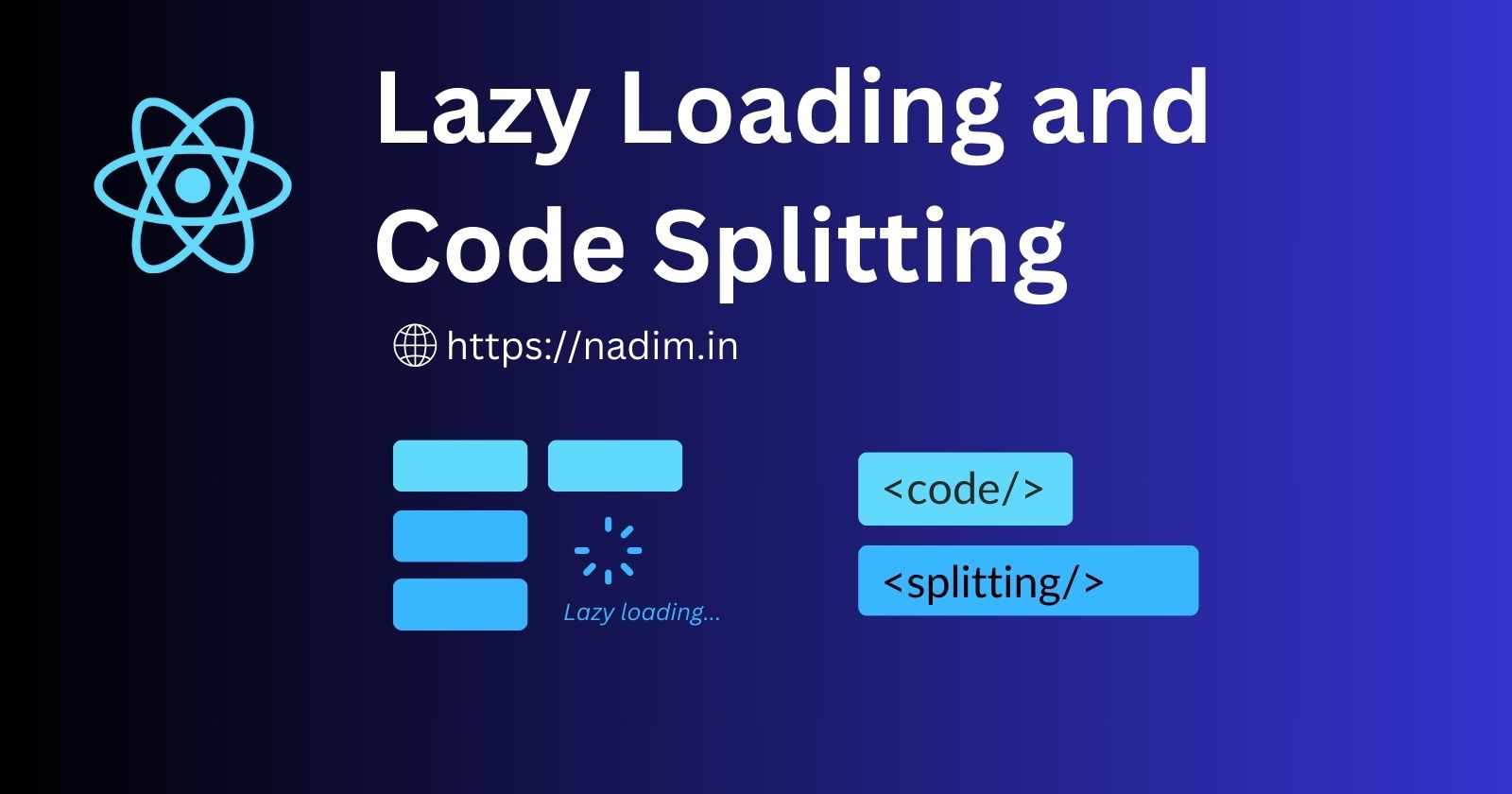
In the world of web development, performance is key. Users expect fast, responsive applications that load quickly and provide a seamless experience. Two powerful techniques to achieve these goals are lazy loading and code splitting. These strategies are essential for optimizing web applications, especially those built with modern JavaScript frameworks like React.
Understanding Lazy Loading
Lazy loading is a design pattern that defers the loading of non-critical resources until they are needed. Instead of loading everything at once, which can slow down the initial page load, lazy loading ensures that only the essential parts of the application are loaded first. Additional resources are fetched as they become necessary, improving overall performance and user experience.
Key Benefits of Lazy Loading:
Improved Performance: By loading only the necessary components initially, the initial load time is reduced, leading to a faster, more responsive application.
Reduced Bandwidth Usage: Only the required resources are loaded, saving bandwidth and reducing data usage.
Enhanced User Experience: Users can interact with the essential parts of the application more quickly, while other parts load in the background.
Implementing Lazy Loading in React
In React, lazy loading can be implemented using React.lazy
and Suspense
. Here's an example:
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
In this example, the LazyComponent
is loaded only when it is needed. The Suspense
component is used to display a fallback (e.g., a loading spinner) while the component is being loaded.
Understanding Code Splitting
Code splitting is a technique to split your code into various bundles that can be loaded on demand or in parallel. This approach reduces the size of the initial bundle, speeding up the initial load time of your application.
Key Benefits of Code Splitting:
Optimized Load Time: By splitting code into smaller bundles, the browser loads only the necessary code for the current page, reducing the initial load time.
Improved Performance: It prevents loading the entire application codebase at once, which can significantly enhance performance, especially for large applications.
Enhanced User Experience: Users can start interacting with the application faster, as the critical parts load quicker.
Implementing Code Splitting in React
In React, code splitting can be achieved using dynamic import()
statements. Here's an example:
import React, { Component } from 'react';
class App extends Component {
state = {
Component: null
};
loadComponent = () => {
import('./MyComponent').then(Component => {
this.setState({ Component: Component.default });
});
};
render() {
const { Component } = this.state;
return (
<div>
<button onClick={this.loadComponent}>Load Component</button>
{Component && <Component />}
</div>
);
}
}
export default App;
In this example, the MyComponent
is loaded only when the button is clicked, demonstrating code splitting in action.
Combining Lazy Loading and Code Splitting
These techniques are often used together to optimize performance and user experience. Lazy loading components that are code-split ensures that the initial bundle is small and additional code is loaded only when necessary.
Real-World Use Cases and Benefits
Use Cases:
Large Applications: In applications with numerous routes and components, lazy loading and code splitting can significantly improve performance by loading only the required components for the current route.
Media-Rich Content: For applications with heavy media content (images, videos), lazy loading ensures that these resources are loaded only when needed, reducing the initial load time.
Third-Party Libraries: Large third-party libraries can be loaded on demand, reducing the initial bundle size.
Benefits:
Faster Initial Load: Only essential code is loaded initially, leading to faster page loads.
Improved Performance: Loading code on demand reduces memory usage and enhances application responsiveness.
Better User Experience: Users can access and interact with the core functionalities of the application quickly while additional features load in the background.
Conclusion
Lazy loading and code splitting are powerful techniques for optimizing web applications. By deferring the loading of non-critical resources and splitting code into smaller bundles, developers can create more efficient, performant, and user-friendly applications. These strategies not only improve performance but also enhance the overall user experience, making them essential tools in the modern web development toolkit.
FAQs about Lazy Loading and Code Splitting
What is Lazy Loading?
Lazy loading is a design pattern that defers the loading of non-critical resources until they are needed. This helps in reducing the initial load time of a web application by loading only the essential components first.
How does Lazy Loading improve performance?
By loading only the necessary resources initially, lazy loading reduces the initial load time, conserves bandwidth, and allows users to interact with the essential parts of the application more quickly.
How can I implement Lazy Loading in React?
In React, lazy loading can be implemented using React.lazy
and Suspense
. Here's a simple example:
codeimport React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
What is Code Splitting?
Code splitting is a technique to divide your code into smaller bundles that can be loaded on demand or in parallel. This approach optimizes the load time and performance of web applications by loading only the necessary code for the current page.
How does Code Splitting enhance web application performance?
By splitting the code into smaller bundles, code splitting ensures that the browser only loads the code required for the current view, reducing the initial load time and improving overall performance.
How can I implement Code Splitting in React?
In React, code splitting can be achieved using dynamic import()
statements. Here’s an example:
import React, { Component } from 'react';
class App extends Component {
state = {
Component: null
};
loadComponent = () => {
import('./MyComponent').then(Component => {
this.setState({ Component: Component.default });
});
};
render() {
const { Component } = this.state;
return (
<div>
<button onClick={this.loadComponent}>Load Component</button>
{Component && <Component />}
</div>
);
}
}
export default App;
Can Lazy Loading and Code Splitting be used together?
Yes, these techniques are often used together to optimize the performance and user experience of web applications. Lazy loading components that are code-split ensures that the initial bundle is small and additional code is loaded only when necessary.
What are the benefits of combining Lazy Loading and Code Splitting?
Faster Initial Load: Only essential code is loaded initially, leading to faster page loads.
Improved Performance: Loading code on demand reduces memory usage and enhances application responsiveness.
Better User Experience: Users can access and interact with the core functionalities of the application quickly while additional features load in the background.
What are some real-world use cases for Lazy Loading and Code Splitting?
Large Applications: Improves performance by loading only the required components for the current route.
Media-Rich Content: Loads heavy media content (images, videos) only when needed, reducing the initial load time.
Third-Party Libraries: Loads large third-party libraries on demand, reducing the initial bundle size.
How do these techniques affect SEO?
For client-side rendered applications, lazy loading can sometimes delay the loading of content, which may affect SEO if not handled properly. Using server-side rendering (SSR) or static site generation (SSG) with frameworks like Next.js can mitigate these issues by ensuring that content is available to search engines.
What tools and libraries support Lazy Loading and Code Splitting?
React: Supports lazy loading and code splitting natively with
React.lazy
,Suspense
, and dynamicimport()
statements.Webpack: A popular module bundler that supports code splitting and lazy loading.
Next.js: A React framework that provides built-in support for server-side rendering, static site generation, and code splitting.
By understanding and implementing lazy loading and code splitting, developers can create web applications that are not only efficient and performant but also deliver a superior user experience.
Subscribe to my newsletter
Read articles from Nadim Anwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
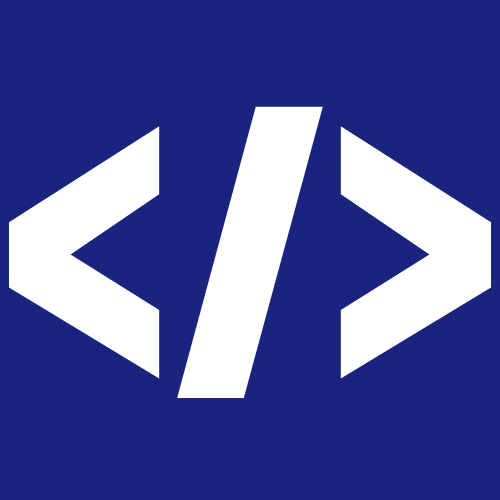
Nadim Anwar
Nadim Anwar
As an experienced front-end developer, I have expertise in building scalable and responsive web applications using modern technologies like ReactJS, Next.js, and TailwindCSS. With a solid understanding of JavaScript and a strong design sense, I excel at turning concepts into visually appealing and practical user interfaces. I am committed to continuously enhancing my skills and staying abreast of the latest industry trends to provide cutting-edge solutions.