Method Overriding and Hiding in C#
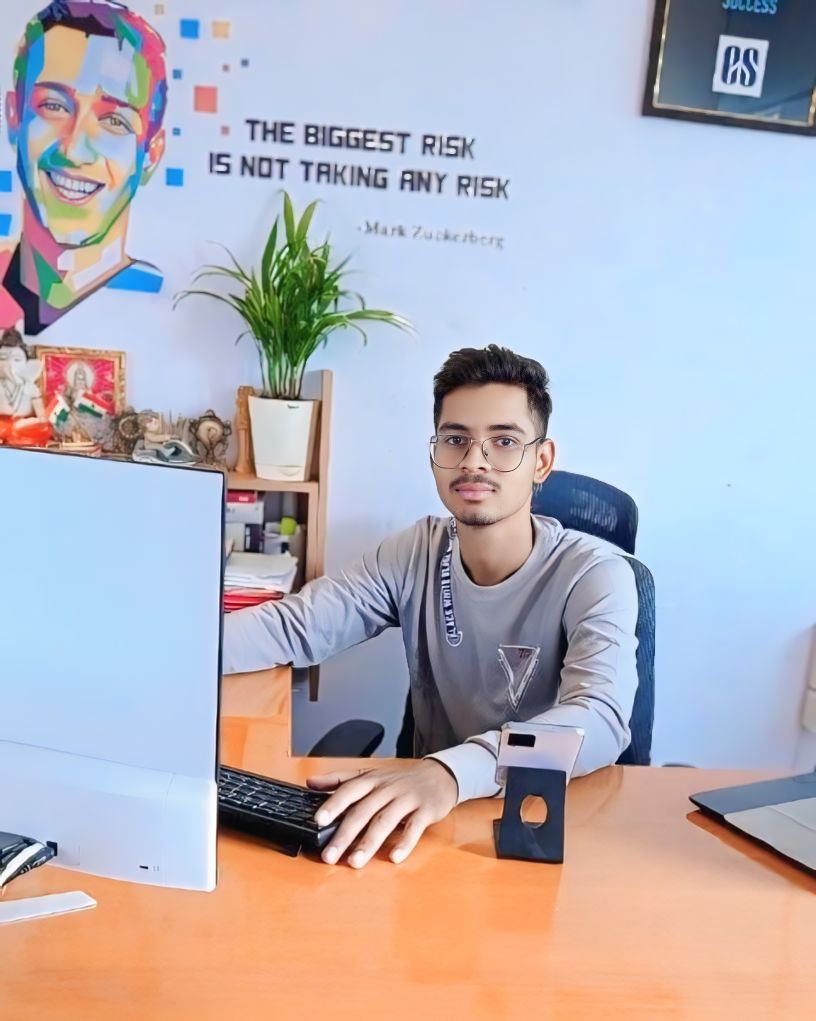
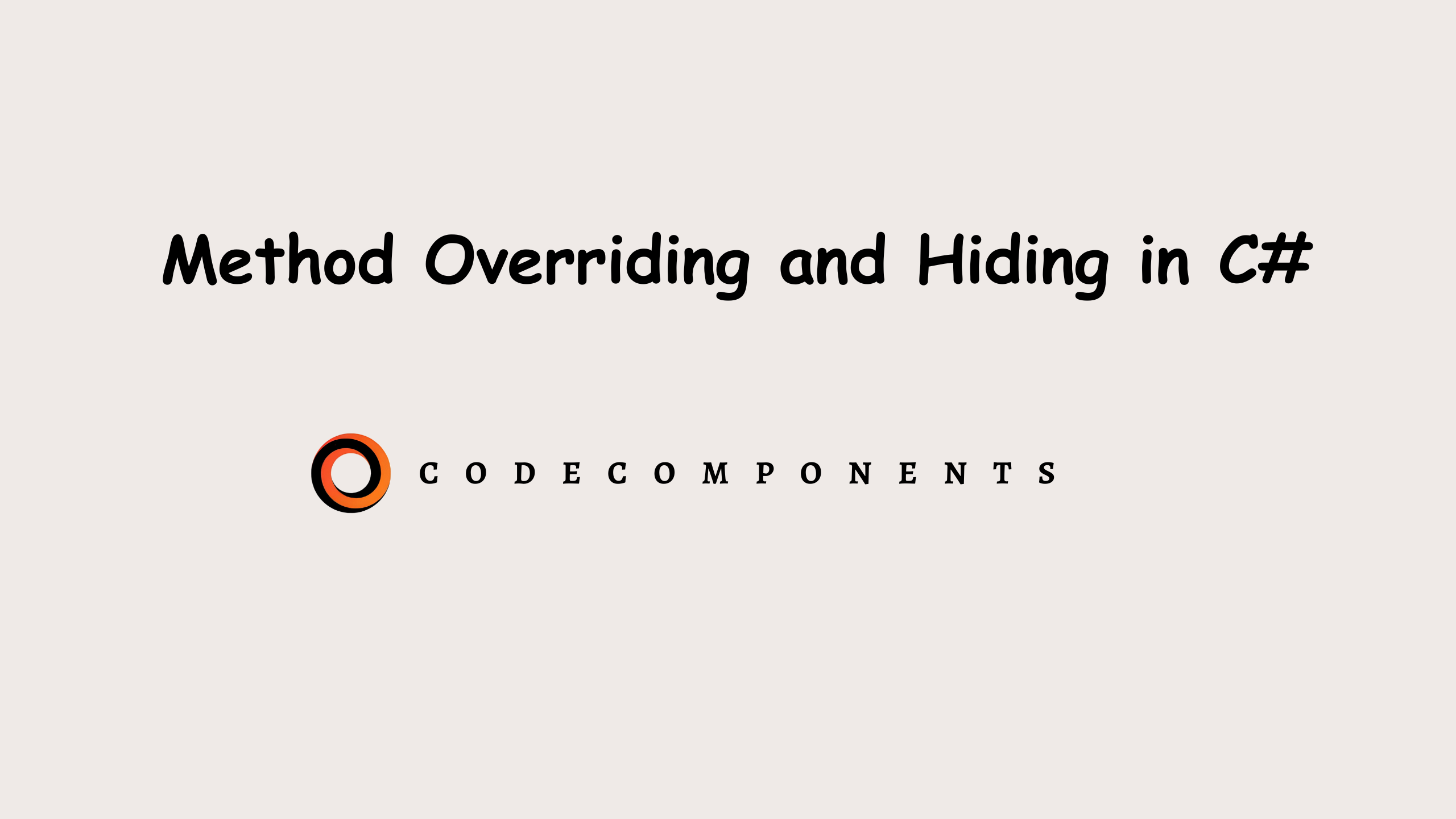
In C#, method overriding and method hiding are mechanisms used to alter or extend the behavior of methods defined in base classes when creating derived classes. These concepts are fundamental to object-oriented programming and provide flexibility and extensibility in class hierarchies. Let's delve into each concept:
Method Overriding
Method overriding refers to the ability of a subclass to provide a specific implementation of a method that is already defined in its superclass. Here's how it works:
Base Class (Parent Class): The class that defines a method as
virtual
indicates that this method can be overridden by derived classes.public class Animal { public virtual void MakeSound() { Console.WriteLine("Animal makes a sound"); } }
Derived Class (Child Class): To override a method from the base class, the derived class uses the
override
keyword.public class Dog : Animal { public override void MakeSound() { Console.WriteLine("Dog barks"); } }
Usage: Method overriding allows a derived class to provide its own specific implementation of a method defined in a base class. This is crucial for implementing polymorphism, where the same method call on different objects can yield different behavior based on the actual type of the object.
csharpCopy codeAnimal myAnimal = new Dog(); myAnimal.MakeSound(); // Output: Dog barks
Virtual Methods
Virtual Method: A method in a base class marked with the
virtual
keyword can be overridden in a derived class using theoverride
keyword. This allows the derived class to provide its own implementation of the method.csharpCopy code// Base class public class Animal { // Virtual method in the base class public virtual void MakeSound() { Console.WriteLine("Animal makes a sound"); } }
Method Overriding
Override: When you use the
override
keyword in the derived class, you explicitly indicate that you are providing a new implementation for a method defined in the base class. This allows for polymorphic behavior, where the method called is determined by the actual type of the object.csharpCopy code// Derived class public class Dog : Animal { // Override the base class method public override void MakeSound() { Console.WriteLine("Dog barks"); } }
Example:
csharpCopy codeAnimal myDog = new Dog(); myDog.MakeSound(); // Output: Dog barks
Here,
myDog
is of typeAnimal
, but since it refers to an instance ofDog
, the overriddenMakeSound
method inDog
is called.
Method Hiding
Method hiding is the concept where a derived class introduces a method with the same name and signature as a method in its base class. This effectively hides the base class method for instances of the derived class and is achieved using the new
keyword.
Base Class:
public class Animal { public void MakeSound() { Console.WriteLine("Animal makes a sound"); } }
Derived Class:
public class Dog : Animal { public new void MakeSound() { Console.WriteLine("Dog barks"); } }
Usage: Method hiding is useful when you want to provide a different implementation of a method in a derived class that has the same signature as a method in the base class. It does not support polymorphism; the method to be called is determined by the compile-time type of the reference.
Animal myAnimal = new Dog(); myAnimal.MakeSound(); // Output: Animal makes a sound (compile-time type is Animal) Dog actualDog = new Dog(); actualDog.MakeSound(); // Output: Dog barks (compile-time type is Dog)
Virtual Keyword
Purpose: The
virtual
keyword is used to declare that a method, property, or indexer in a base class can be overridden in derived classes. It enables polymorphism by allowing objects of derived classes to be treated as objects of their base class.Usage:
Applied to methods:
public virtual void MethodName() { ... }
Applied to properties and indexers:
public virtual int PropertyName { get; set; }
Override vs New:
override
: Used in derived classes to provide a new implementation of a virtual member inherited from a base class.new
: Used to hide a member (method, property, indexer) of the same name and signature from a base class.
Why Use Method Overriding and Hiding?
Flexibility and Extensibility: These mechanisms allow you to customize the behavior of methods inherited from base classes without modifying the base class itself. This promotes code reuse and modularity.
Polymorphism: Method overriding enables polymorphic behavior, where you can treat objects of derived classes as objects of their base class type, yet call methods specific to the derived class.
Specialization: Method hiding allows derived classes to provide specialized implementations of methods, tailored to their specific needs or requirements.
Conclusion
Using virtual
and override
allows you to leverage polymorphism in object-oriented programming, enabling dynamic dispatch of method calls based on the actual type of the object. new
allows for method hiding, where a derived class provides a new implementation unrelated to the base class method with the same name. Understanding these keywords is crucial for designing and implementing class hierarchies that exhibit behavior specific to derived classes while retaining flexibility and code reuse through inheritance.
Subscribe to my newsletter
Read articles from Mritunjay Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
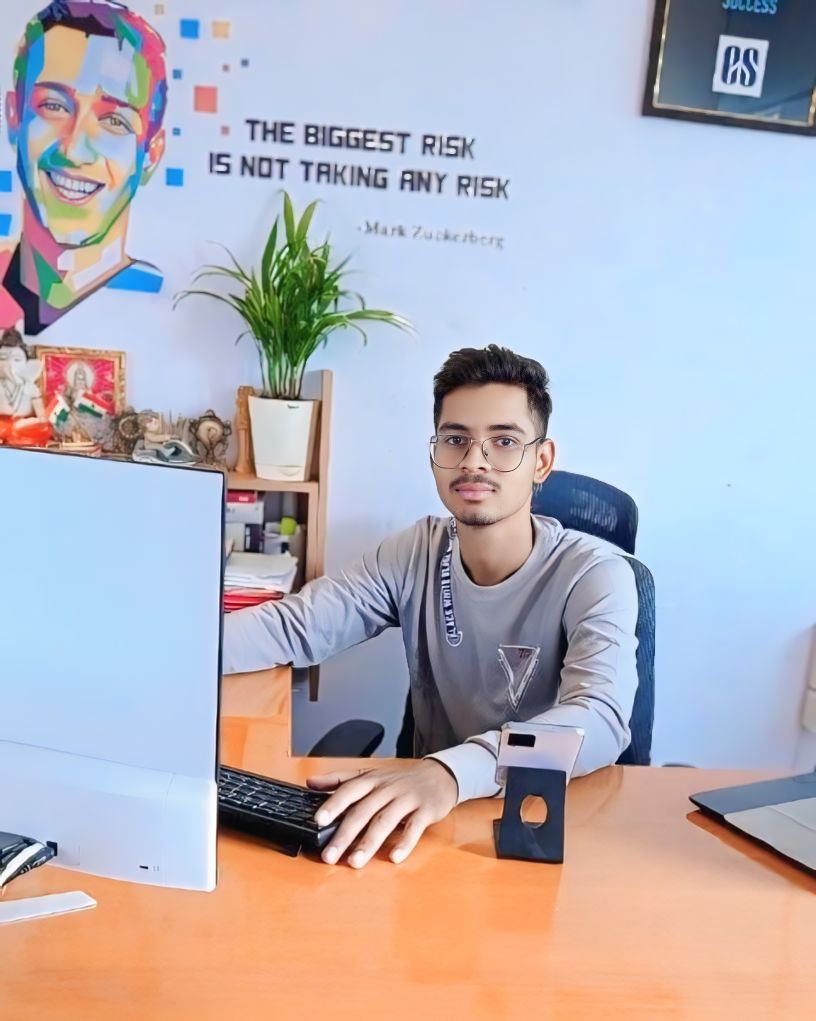