Printing a Reverse Triangle shape

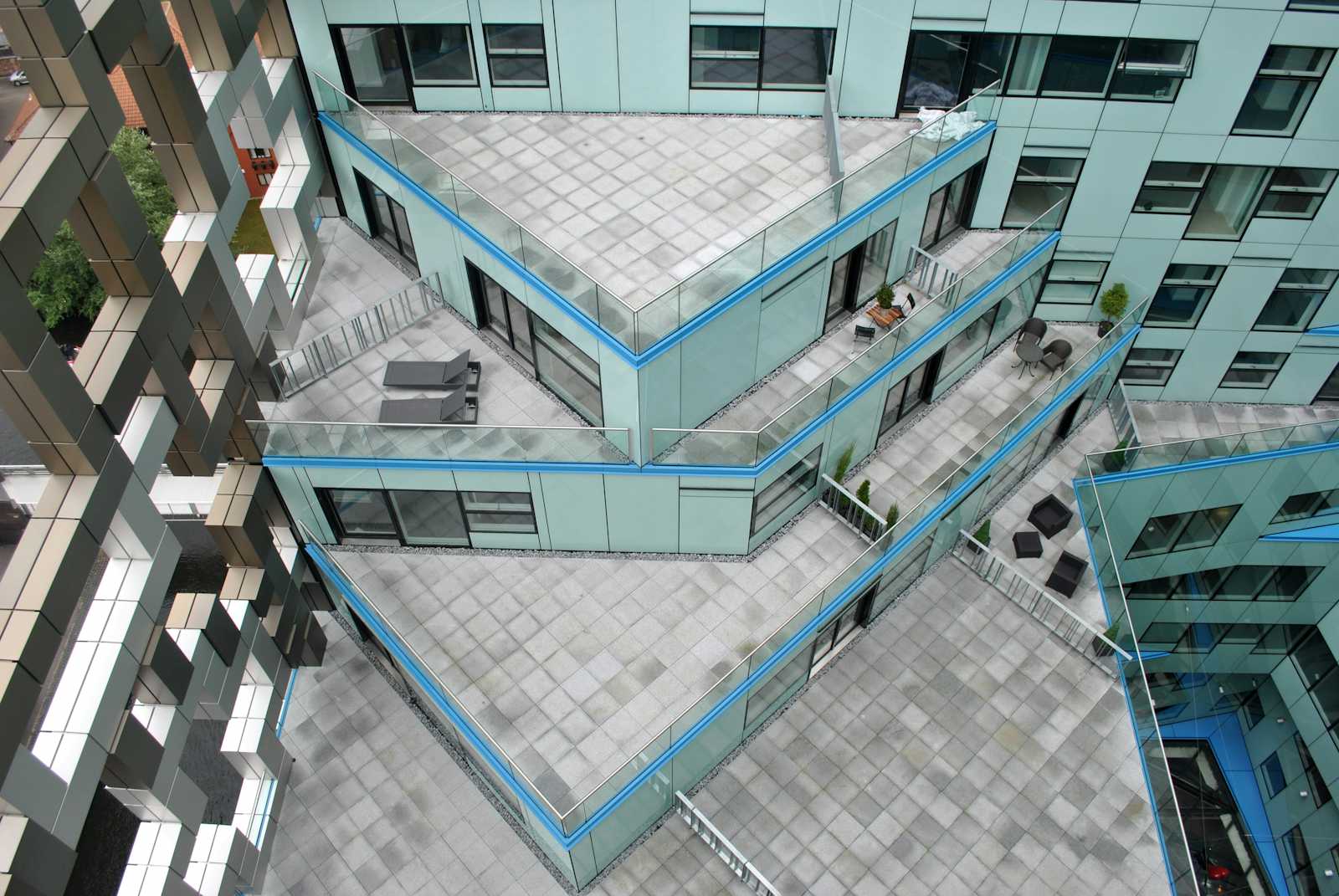
Introduction
I've already written a post, "Printing a Pyramid shape", showing how to print a triangle with the apex at the top.
In this post, we will be printing the shape of reverse-triangle ๐ป (apex at the bottom and base at the top), using COBOL.
Approach
The shape will be spread out across 10 lines.
PERFORM loop and DISPLAY statements in COBOL will be used to generate the shape.
Reverse Triangle shape in COBOL
Here ๐ is the COBOL code to print the shape.
IDENTIFICATION DIVISION.
PROGRAM-ID. REVERSE-TRIANGLE.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-OUT PIC X(80) VALUE SPACES.
01 WS-N PIC S9(3) VALUE 0.
01 WS-LINE-POS PIC 9(2) VALUE 1.
PROCEDURE DIVISION.
PERFORM VARYING WS-N FROM 19 BY -2 UNTIL WS-N < 1
INITIALIZE WS-OUT
MOVE ALL '*' TO WS-OUT(WS-LINE-POS:WS-N)
COMPUTE WS-LINE-POS = WS-LINE-POS + 1
DISPLAY WS-OUT
END-PERFORM.
STOP RUN.
How the code works?
Here is a detailed explanation of the code from the WORKING-STORAGE section.
We have the following data-items defined in the WORKING-STORAGE section:
WS-OUT is an 80-character alphanumeric data-item which is used to display the output. It is initialized with spaces.
WS-N is a signed integer data-item initialized to 0. This will be used to control the number of asterisks printed in each line.
WS-LINE-POS is an integer data-item initialized to 1. It will control the starting position for asterisks in each line.
Next, we have the statements to be executed in the PROCEDURE DIVISION.
PERFORM VARYING WS-N FROM 19 BY -2 UNTIL WS-N < 1: This loop starts with WS-N at 19 and decrements it by 2 in each iteration until WS-N is less than 1. I've hardcoded 19 because we need to ensure that we're picking up an odd number to display the base/apex of the triangle with asterisks so as to form a symmetrical shape. To print the shape as symmetrical as possible and in 10 lines, 19 is the best number to start with. In the post that I wrote to print a triangle with the apex at the top, WS-N started from 1 and went on until 19 with an increment of 2 in each iteration. There are totally 10 loop iterations to print both the shapes (triangle and reverse-triangle) in 10 lines.
INITIALIZE WS-OUT: This resets WS-OUT to spaces at the start of each loop iteration.
MOVE ALL '*' TO WS-OUT(WS-LINE-POS:WS-N): This moves a number of asterisks equal to the value of WS-N into WS-OUT, starting at position WS-LINE-POS.
COMPUTE WS-LINE-POS = WS-LINE-POS + 1: This increments WS-LINE-POS by 1, moving the starting position of asterisks to the right for the next line.
DISPLAY WS-OUT: This outputs the current line stored in WS-OUT.
Output
Here ๐ is the output after running the code.
*******************
*****************
***************
*************
***********
*********
*******
*****
***
*
By clicking ๐ here, you can execute the code for yourself on JDoodle.
Conclusion
That's the 5th shape in the series, "Printing shapes using COBOL". There's something interesting to come. See you in the next post.
Thx!
๐
Subscribe to my newsletter
Read articles from Srinivasan JV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Srinivasan JV
Srinivasan JV
I have been a mainframe developer for the past 10 years. As part of work obligations, I am currently in pursuit of learning Java. You'll find me writing more about the mainframe.