Reverse Substrings Between Each Pair of Parentheses
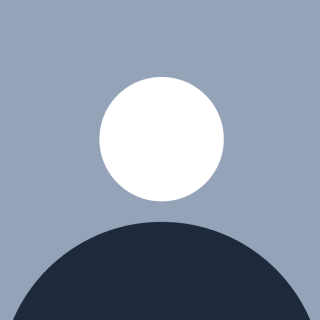
1 min read
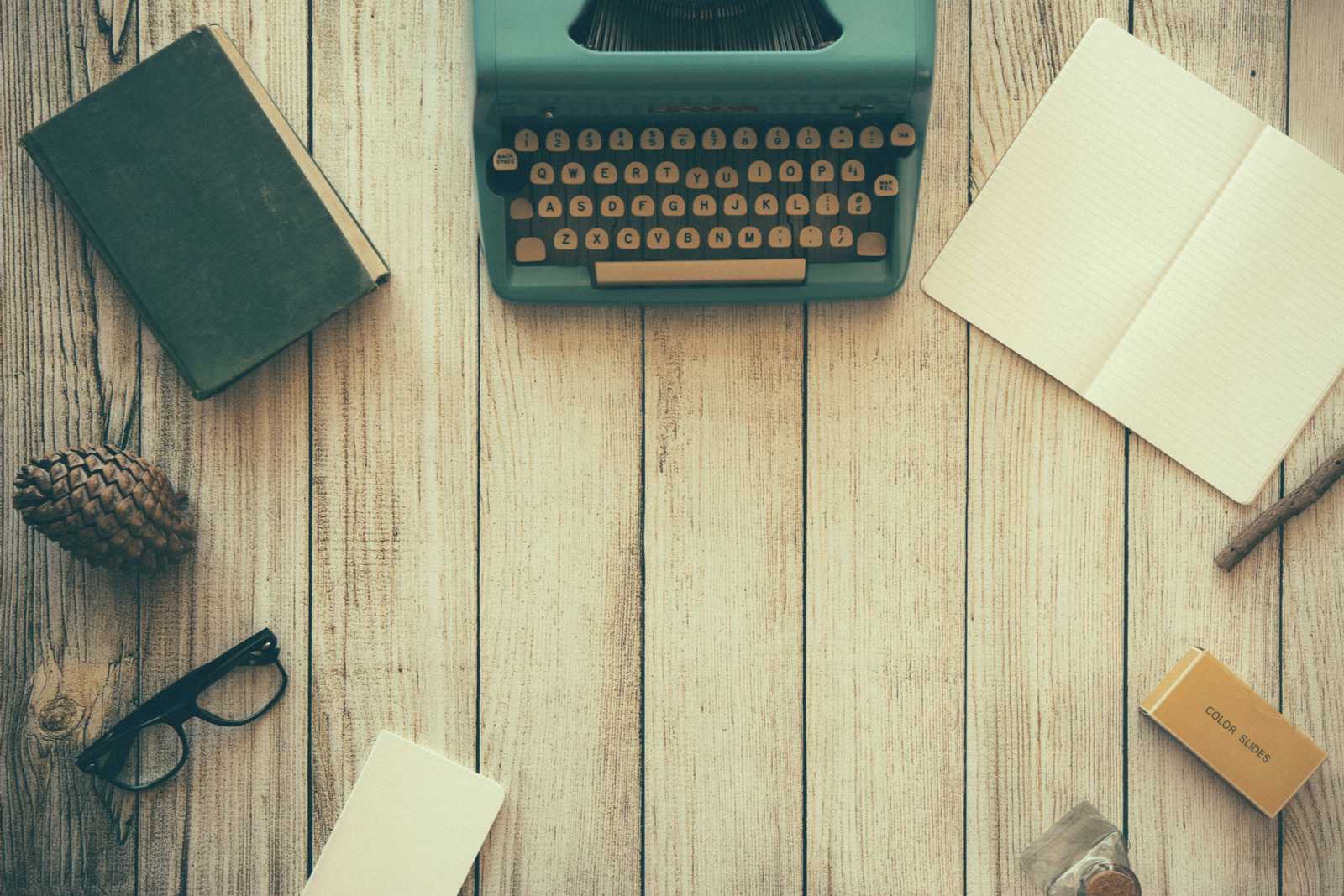
Problem Link
Approach
First we will store the characters other than parenthesis because we don't want parenthesis in our answer.
As soon as we encounter an opening bracket we will store it's index In the vector
As all the parenthesis are guaranteed to be balanced we will keep on iterating until we find a closing bracket
As soon as we encounter the closing parenthesis, we will reverse until the index of the last opening parenthesis and keep doing it until we are done with our iteration.
Code
class Solution {
public:
string reverseParentheses(string s) {
string ans = "" ; // initially the ans is empty string
vector<int> index; // to store the index of the parenthesis
for(int i = 0;i<s.length();i++){
if(s[i]=='('){
index.push_back(ans.size());
}
else if(s[i]==')'){
int start = index.back();
index.pop_back();
reverse(ans.begin()+start, ans.end());
}else{
ans = ans+s[i];
}
}
return ans;
}
};
All the best for your DSA journey! Let me know your feedback so that we can improve this article together.
Ujjwal Sharma
0
Subscribe to my newsletter
Read articles from Ujjwal Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by