Symbol Example
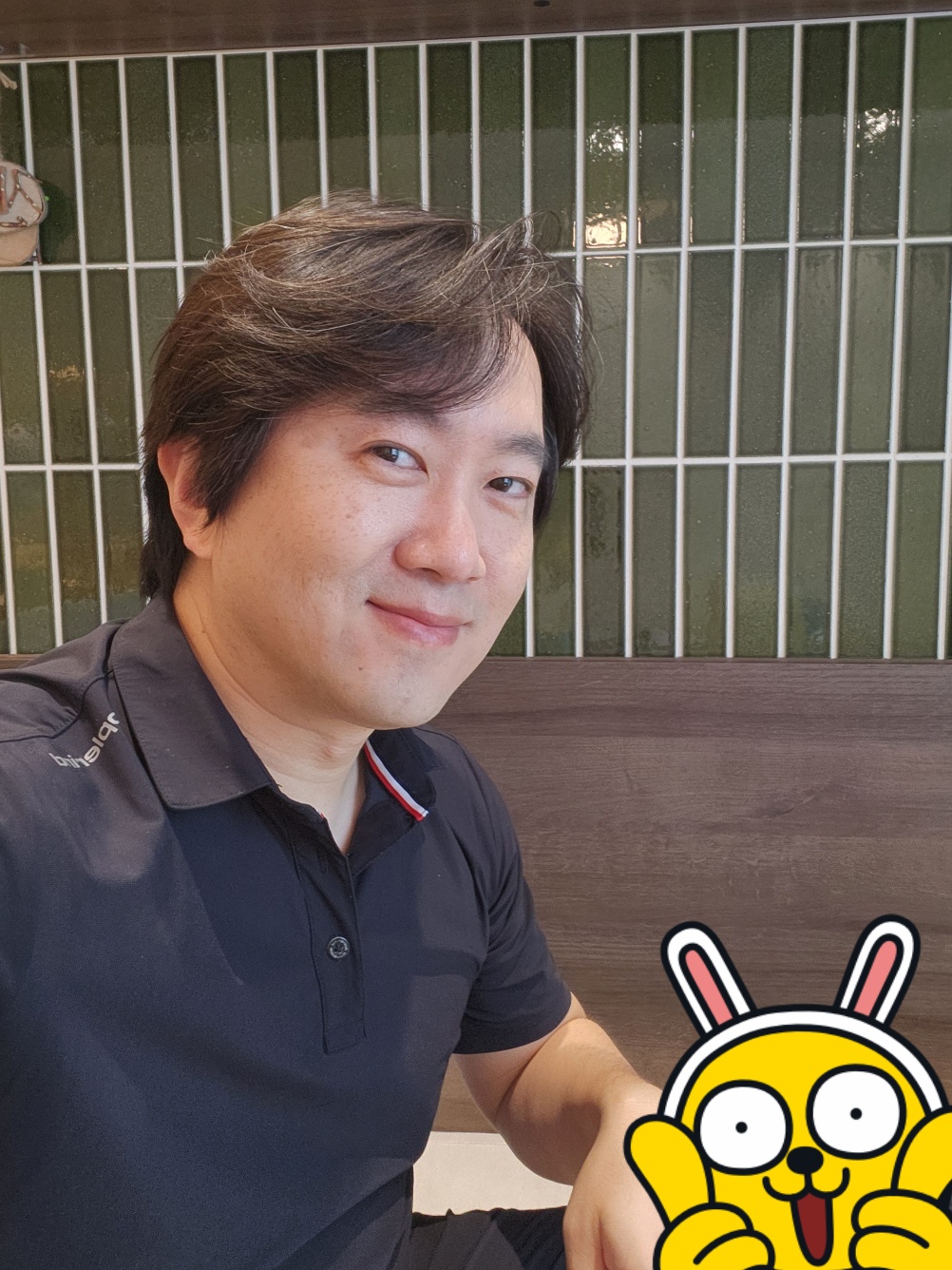
- Symbol.iterator:
const iterableObject = {
data: [1, 2, 3],
[Symbol.iterator]() {
let index = 0;
return {
next: () => {
if (index < this.data.length) {
return { value: this.data[index++], done: false };
} else {
return { done: true };
}
}
};
}
};
for (let value of iterableObject) {
console.log(value); // 1, 2, 3
}
- Symbol.match:
const matcherObject = {
[Symbol.match]: (string) => string.includes("hello")
};
console.log("Say hello".match(matcherObject)); // true
- Symbol.hasInstance:
class MyClass {
static [Symbol.hasInstance](instance) {
return typeof instance === "string";
}
}
console.log("hello" instanceof MyClass); // true
- Symbol.isConcatSpreadable:
const nonSpreadableArray = [1, 2, 3];
nonSpreadableArray[Symbol.isConcatSpreadable] = false;
console.log([].concat(nonSpreadableArray)); // [[1, 2, 3]]
- Symbol.species:
class MyArray extends Array {
static get [Symbol.species]() {
return Array;
}
}
const instance = new MyArray(1, 2, 3);
const mapped = instance.map(x => x * x);
console.log(mapped instanceof MyArray); // false
console.log(mapped instanceof Array); // true
- Symbol.toPrimitive:
const objectWithToPrimitive = {
[Symbol.toPrimitive](hint) {
switch (hint) {
case 'string':
return 'Hello';
case 'number':
return 42;
default:
return 'default';
}
}
};
console.log(+objectWithToPrimitive); // 42
console.log(`${objectWithToPrimitive}`); // 'Hello'
console.log(objectWithToPrimitive + ''); // 'default'
이러한 예제들은 각 심볼의 기본적인 사용 방법을 보여줍니다. 실제 애플리케이션에서는 더 복잡한 시나리오와 함께 이러한 심볼들을 사용할 수 있습니다.
심볼의 간단한 사용 예
class MyClass {
get [Symbol.toStringTag]() {
return "MyClassTag";
}
}
let instance = new MyClass();
console.log(instance.toString()); // [object MyClassTag]
TypeScript에서도 @@toStringTag
를 사용하는 것은 JavaScript와 크게 다르지 않습니다. 그러나 TypeScript의 타입 체크 기능을 활용하여 타입 안정성을 더욱 강화할 수 있습니다.
다음은 TypeScript에서 @@toStringTag
를 사용하는 예시입니다:
- 기본적인 사용:
const myArray: any[] = [];
console.log(myArray.toString()); // [object Array]
@@toStringTag
사용하여 변경:
const myCustomArray: any[] = [];
myCustomArray[Symbol.toStringTag] = "SpecialArray";
console.log(myCustomArray.toString()); // [object SpecialArray]
- 클래스 내에서
@@toStringTag
사용:
class MyClass {
get [Symbol.toStringTag](): string {
return "MyClassTag";
}
}
const instance = new MyClass();
console.log(instance.toString()); // [object MyClassTag]
- 인터페이스와 함께 사용:
interface CustomToString {
[Symbol.toStringTag]: string;
}
class AnotherClass implements CustomToString {
[Symbol.toStringTag] = "AnotherClassTag";
}
const anotherInstance = new AnotherClass();
console.log(anotherInstance.toString()); // [object AnotherClassTag]
여기서는 CustomToString
인터페이스를 정의하여 @@toStringTag
속성을 포함시켰습니다. 그런 다음 AnotherClass
에서 이 인터페이스를 구현하여 해당 클래스의 인스턴스에 대한 toString()
반환 값을 사용자 정의했습니다.
이렇게 TypeScript에서도 @@toStringTag
를 사용하여 객체의 toString()
반환 값을 사용자 정의할 수 있습니다.
간단한 사용 예
TypeScript에서도 @@toStringTag
를 사용하는 것은 JavaScript와 크게 다르지 않습니다. 그러나 TypeScript의 타입 체크 기능을 활용하여 타입 안정성을 더욱 강화할 수 있습니다.
다음은 TypeScript에서 @@toStringTag
를 사용하는 예시입니다:
- 기본적인 사용:
const myArray: any[] = [];
console.log(myArray.toString()); // [object Array]
@@toStringTag
사용하여 변경:
const myCustomArray: any[] = [];
myCustomArray[Symbol.toStringTag] = "SpecialArray";
console.log(myCustomArray.toString()); // [object SpecialArray]
- 클래스 내에서
@@toStringTag
사용:
class MyClass {
get [Symbol.toStringTag](): string {
return "MyClassTag";
}
}
const instance = new MyClass();
console.log(instance.toString()); // [object MyClassTag]
- 인터페이스와 함께 사용:
interface CustomToString {
[Symbol.toStringTag]: string;
}
class AnotherClass implements CustomToString {
[Symbol.toStringTag] = "AnotherClassTag";
}
const anotherInstance = new AnotherClass();
console.log(anotherInstance.toString()); // [object AnotherClassTag]
여기서는 CustomToString
인터페이스를 정의하여 @@toStringTag
속성을 포함시켰습니다. 그런 다음 AnotherClass
에서 이 인터페이스를 구현하여 해당 클래스의 인스턴스에 대한 toString()
반환 값을 사용자 정의했습니다.
이렇게 TypeScript에서도 @@toStringTag
를 사용하여 객체의 toString()
반환 값을 사용자 정의할 수 있습니다.
Subscribe to my newsletter
Read articles from webisme directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
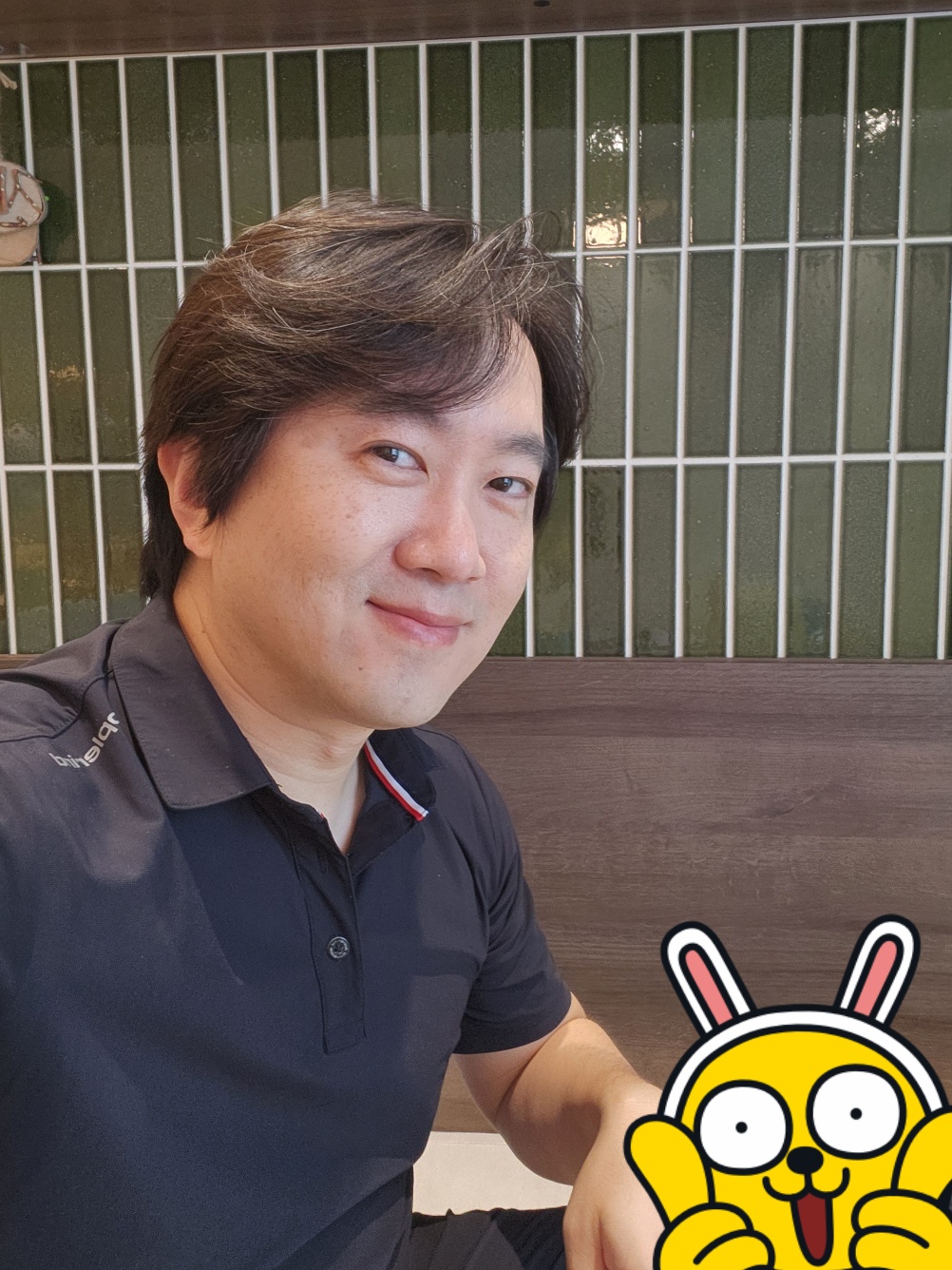