Unraveling the Power of MongoDB Pipelines: Exploring a Real-World Use Case
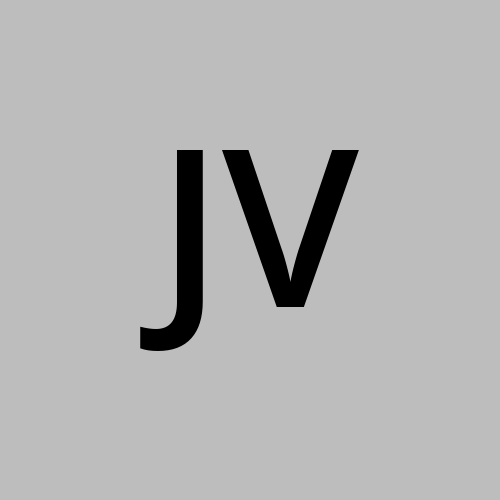
Introduction
In this article, we will explore the code snippet provided and break down the key concepts used in it. The code snippet is a part of a function called getUserChannelProfile
that retrieves a user's channel profile information from a MongoDB database using the Mongoose library. Let's dive into the details of the code and explain the various MongoDB aggregation pipeline stages and operators used.
const getUserChannelProfile = asyncHandler(async(req,res)=>{
// ...
})
The
getUserChannelProfile
function is an asynchronous function that takesreq
(request) andres
(response) objects as parameters. It uses theasyncHandler
middleware to handle any errors that may occur during the execution of the function.
Pipeline
The MongoDB aggregation pipeline is a sequence of stages that process and transform data. Each stage takes the output of the previous stage as input and performs a specific operation on the data. The pipeline is defined as an array of objects, where each object represents a stage in the pipeline.
In the provided code snippet, the aggregation pipeline consists of several stages:
$match
$lookup
(two instances)$addFields
$project
$match
Operator
The $match
stage filters the input documents to only those that match the specified condition. It is often used as the first stage in the pipeline to reduce the number of documents that need to be processed by subsequent stages.
{
$match: {
username: username?.toLowerCase(),
},
},
In this case, the
$match
stage filters the documents based on theusername
field. It converts theusername
parameter to lowercase using the?.toLowerCase()
syntax to ensure case-insensitive matching.
$lookup
Operator
The $lookup
stage performs a left outer join between two collections. It takes documents from the current collection and joins them with documents from a specified collection based on matching fields.
{
$lookup: {
from: "subscriptions",
localField: "_id",
foreignField: "channel",
as: "subscribers",
},
},
This
$lookup
stage joins the current collection (assumed to beusers
) with thesubscriptions
collection. It matches the_id
field of the current collection with thechannel
field in thesubscriptions
collection. The joined documents are stored in thesubscribers
field.
{
$lookup: {
from: "subscriptions",
localField: "_id",
foreignField: "subscriber",
as: "subscribedTo",
},
},
The second
$lookup
stage also joins the current collection with thesubscriptions
collection, but this time it matches the_id
field with thesubscriber
field in thesubscriptions
collection. The joined documents are stored in thesubscribedTo
field.
$addFields
Operator
The $addFields
stage adds new fields to the output documents or renames existing fields. It allows you to perform calculations and transformations on the data.
{
$addFields: {
subscribersCount: {
$size: "$subscribers",
},
channelsSubscribedTo: {
$size: "$subscribedTo",
},
isSubscribedTo: {
$cond: {
if: { $in: [req.user?._id, "$subscribedTo.subscriber"] },
then: true,
else: false,
},
},
},
},
In this stage:
The
subscribersCount
field is added, which calculates the size (number of elements) of thesubscribers
array using the$size
operator.The
channelsSubscribedTo
field is added, which calculates the size of thesubscribedTo
array using the$size
operator.The
isSubscribedTo
field is added, which checks if thereq.user?._id
(the current user's ID) exists in thesubscribedTo.subscriber
array using the$in
operator. If the user is found, it sets the value totrue
, otherwisefalse
.
$project
Operator
The $project
stage specifies the fields to include in the output documents. It allows you to include, exclude, or rename fields.
{
$project: {
fullName: 1,
username: 1,
subscribersCount:1,
channelsSubscribedTo:1,
avatar: 1,
coverImage: 1,
isSubscribedTo: 1,
},
},
In this stage, the following fields are included in the output documents:
fullName
username
subscribersCount
channelsSubscribedTo
avatar
coverImage
isSubscribedTo
The 1
value indicates that these fields should be included, while any omitted fields will be excluded from the output.
After the aggregation pipeline is executed, the function checks if the channel
array is empty. If it is, it throws an error with a 404 status code indicating that the channel was not found.
Finally, the function returns a JSON response with a 200 status code, containing the user's channel profile information.
Conclusion
In this article, we explored the code snippet and explained the key concepts used in it, such as the MongoDB aggregation pipeline, $match
, $lookup
, $addFields
, and $project
operators. We discussed how these operators work together to retrieve and transform data from the database to obtain the desired user channel profile information.
By breaking down the code and explaining the underlying theory and examples, we hope this article helps you better understand the usage of MongoDB aggregation pipeline stages and operators in a real-world scenario.
Subscribe to my newsletter
Read articles from Jaykishan Varma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
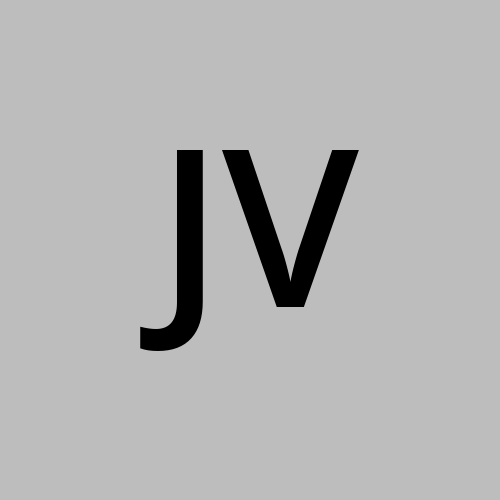