Why you should be using the HTML data-* attribute
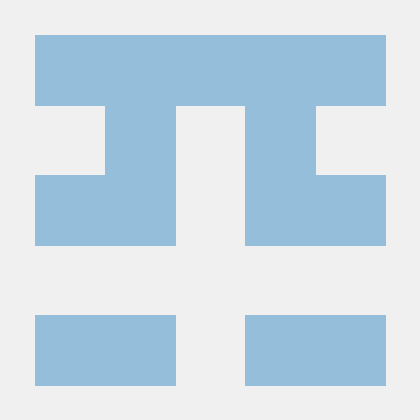
What is it?
HTML tags are the main components that define webpage elements. HTML attributes describe tags with additional information. This information may have many purposes, including:
Identifying tags, including uniquely
Providing users (and sometimes search engines) with helpful information
Controlling the element's behavior
Specifying the element's design
Tracking custom data
But the tracking custom data functionality often gets ignored. Enter the data-* attribute. The HTML data-* attribute is a customizable tool for storing non-sensitive, localized page or application data. As an example, data-id and data-animal-type below are both data-* attributes that describe the image.
<img data-id="1" data-animal-type="lion" src=".assets/.../.jpg">
How do you use it?
On the HTML side, the data-* attribute is a global attribute that takes at least one character after the prefix "data-". Do not use any uppercase letters; instead use dashes if you need to be more descriptive. Other than that, the attribute value can be any string.
As this attribute can be particularly useful for JavaScript actions, let's focus on how JavaScript can access it. On the JavaScript side, it can be accessed using the "dataset" property as well as the "getAttribute", "setAttribute", and "removeAttribute" methods.
const image = document.querySelector('img');
const imageId = image.dataset.id;
const imageAnimalType = image.dataset['animal-type'];
//or
const imageId = image.getAttribute('data-id');
const imageAnimalType = image.getAttribute('data-animal-type');
When should you use it?
The data-* attribute is very helpful when you want to store custom data to be accessible on demand, especially when your JavaScript scope does not have the access it requires. The data-* attribute can be used to:
Store additional information that is needed for JavaScript usage
Improve the UX by enabling dynamic content loading
Collect analytical data on user interactions
Retrieve elements for automated testing frameworks
(Easily style with CSS with greater detail and readability)
Imagine an example with a task list application allowing tasks to be added and their states changed. We can store the task ID and status with the data-* attribute to ensure accessibility regardless of the scope (in this case function scope) in which they are required. When a new task is added, the addTask function below is called, creating a list item element and the data-id and data-completed attributes. By attaching these attributes to each task, tasks always have their associated status information. This is especially useful because this information can be used to manage task status dynamically without having to make AJAX calls or server-side database queries.
function addTask(content) {
const taskId = //unique id
const taskItem = document.createElement('li');
taskItem.setAttribute('data-id', taskId);
taskItem.setAttribute('data-completed', 'false');
taskItem.innerHTML = `
<span class="task-content">${content}</span>
<button class="toggle-complete">Complete</button>
`;
todoList.appendChild(taskItem);
}
function toggleTaskComplete(taskItem) {
const isCompleted = taskItem.dataset.completed === 'true';
taskItem.dataset.completed = isCompleted ? 'false' : 'true';
taskItem.classList.toggle('completed', !isCompleted);
}
The toggleTaskComplete function above is called when the Complete button is clicked. The function reads the current status directly from the data-* attribute and quickly toggles the status. Whereas scope issues can arise from managing statuses via JavaScript variables or objects, including the statuses directly in HTML attributes simplifies our JavaScript code. This reduces the potential for bugs and makes the code easier to understand, especially as additional functionality is added that may need access to the same information in other contexts.
What should you be careful of?
Since HTML attributes are accessible to anyone who can inspect a webpage's code, the data-* attribute should not be used when handling sensitive or private information. Malicious automated attacks can access the data and expose it or use it to further infiltrate the application.
On the flip side, if you want data-* attribute information to assist with SEO, think again, as search crawlers typically do not index this data.
Why do so few people take advantage of its power?
Despite data-*'s benefits, many developers do not harness its potential because it is not widely taught. In the course of learning a language, it is a more common practice to refactor code to avoid scope issues and so the implementation of data-* falls slightly outside of common styles of coding. Due to its esoteric nature, data-* is perceived as being complex even though it is straightforward and can simplify code.
Summary & Conclusion
When creating applications and structures that require interactivity, it may be very beneficial to consider using the data-* attribute. The data-* attribute can enable responsive interactions like item state changes without having to manage complex calls or scope issues across different contexts. It can also be helpful for styling elements with CSS in an extensible, legible manner. Do not use data-* with sensitive data. When used properly, the data-* can significantly simplify code, reduce issues, and increase scalability.
Subscribe to my newsletter
Read articles from Jason Malefakis directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
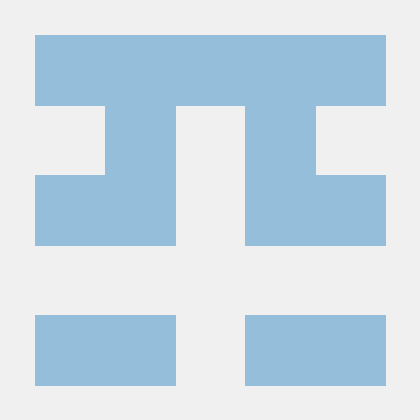