Valid Phone Number - codewars
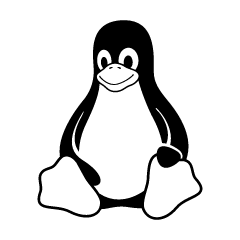
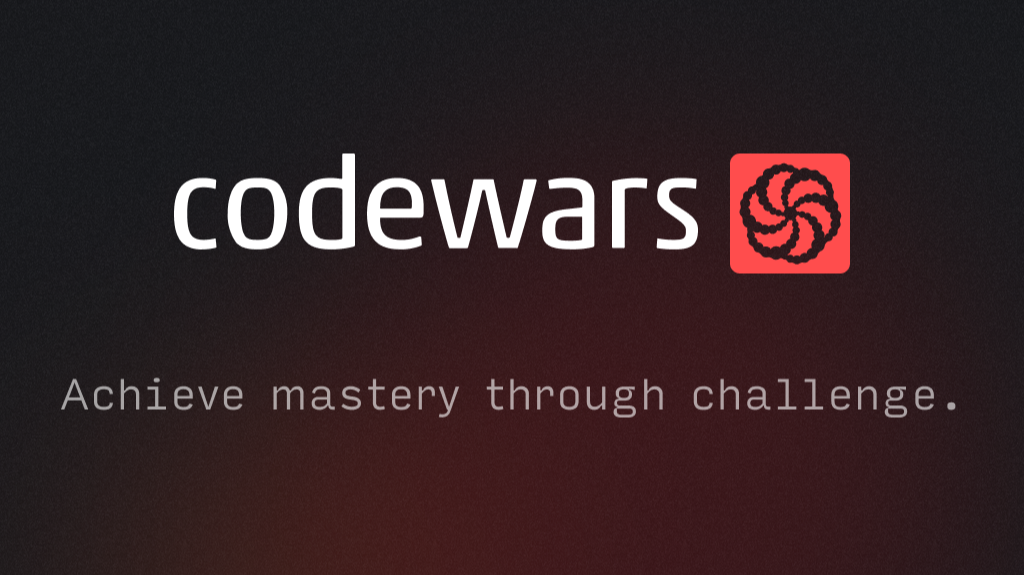
This problem is currently rated as a 6 kyu*(difficulty ratings on codewars range from 8 (easiest) to 1 (hardest))* problem at codewars, this is it’s description :
“Write a function that accepts a string, and returns true if it is in the form of a phone number.
Assume that any integer from 0–9 in any of the spots will produce a valid phone number.Only worry about the following format:
(123) 456–7890 (don’t forget the space after the close parentheses)”
My thoughts after reading that were along the lines of “I could just use regex for that.” (regex stands for regular expressions which are used for searching through text) so that is how I went about it. The problem could be solved in a singular line, however for readability I have made my solution a bit longer. The solution is below:
import re
valid_phone_number = lambda n: not not re.search(r"^\(\d{3}\) \d{3}-\d{4}$",n)
So first we start by importing the package re so we can use regex, then we define a lambda function as “valid_phone_number” so the kata*(problem) can run our code. The function will take one argument **n: string* which stands for number, then it will search through the provided string and see if anything in it is a valid phone number. It checks if it is a valid phone number using regex :
r"^\(\d{3}\) \d{3}-\d{4}$"
The r in front of the string makes it a raw string which means symbols such as \n*(newline) or \t(tab)* are interpreted as normal characters, for ex :
We then specify that we want it to check for the start of the string/line using ^
After we check for the 3 numbers inside of parenthesis at the start :
\(\d{3}\)
We put \ behind characters like ([{}]) when using regex if we want to look for those characters instead of using them normally. We then use \d to specify that we want to search for an integer, you could also use [0–9]. We put {3} next to \d to specify we are looking for 3 numbers.
\d{3}-\d{4}
(There is a space at the start just incase you can't see it)
When looking for a specific character you can type it unless as mentioned above it is used for things in regex in which you would need to put a backspace before it. So, we are looking for a space, 3 numbers, a dash, then 4 more numbers. Now if we put that all together we are looking for a phone number.
Now looking back at the function we see after we call re.search we check if there is any output by putting not in front of it. You can put not before any values/variables to check if it does not exist/ is not true. Example usage of not :
exampleBool: bool = False
exampleEmptyString: str = ""
exampleInteger: int = 0
exampleList: list = []
exampleDict: dict = {}
def example(value: any) -> bool:
return not value
example(exampleBool)
example(exampleEmptyString)
example(exampleInteger)
example(exampleList)
example(exampleDict)
We put a second not because we are flipping the output from False -> True and vice versa. You could also just wrap it in bool() instead, however I think not not sounds funny.
And this is how I solved ‘Valid Phone Numbers’ on codewars. Hopefully you learnt something and if not, thanks for reading!
— 0xsweat 7/10/2024
My socials :
Subscribe to my newsletter
Read articles from 0xsweat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
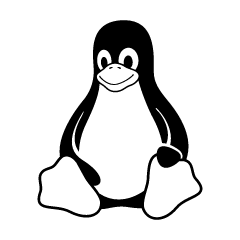
0xsweat
0xsweat
I am a machine that converts hours awake into problems solved at an exponential rate.