Moving Zeros To The End - codewars
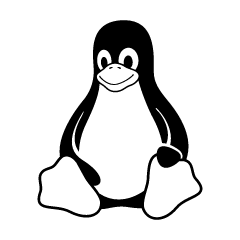
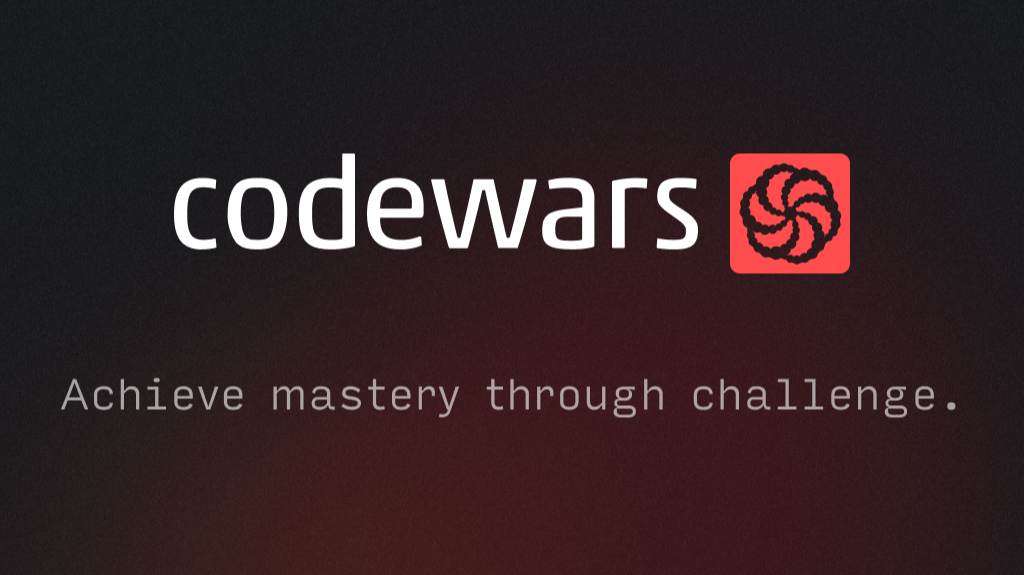
This problem is currently rated as a 5kyu*(ratings on codewars range from 8 (easiest) to 1 (hardest))* problem on codewars, this is it’s description :
“Write an algorithm that takes an array and moves all of the zeros to the end, preserving the order of the other elements.”
After reading that I thought “how is this a 5 kyu this is incredibly easy”. Well codewars is an old website and this problem was published back in Oct 12, 2013… which was ages ago and explains why it’s difficulty is still 5 kyu instead of being readjusted to 7 or even 8 kyu.
I have solved this problem quite a few times, 3 different ways in python, 1 time in C and C++ respectively. So I will be going over 2 of my python solutions, then my C, and C++ solution. My C++ solution is quite old from when I was first learning C++ but can be used as an example of what not to do.
Starting with my most readable python solution :
def move_zeros(lst: list) -> list:
for _ in range(lst.count(0)):
lst.remove(0)
lst.append(0)
return lst
So we start with a for loop that iterates through the amount of zeros in the list. I put an underscore instead of assigning a variable because we are only going to remove a zero, and append a zero for every zero in the list. The reason I did it this way is because when we first take the count it isn’t going to be affected by the new zeros we add to the end, therefore those zeros will remain unaffected by calling list.remove. After that we return the list.
We will now visit the less readable python solution:
move_zeros = lambda lst: [int(y) for y in ''.join(str(x) for x in lst).replace("0","") + "0"*lst.count(0)]
We start by defining a lambda function named ‘move_zeroes’ that takes an argument lst: list which is our list. We will break it down into sections and then go by step to step.
''.join(str(x) for x in lst)
First we convert the integer list to a string by iterating through it and converting them all to a string. Next we turn the list into a string by using string.join and making it join to an empty string.
''.join(str(x) for x in lst).replace("0","")
After that we get rid of all the zeros by using string.replace and replacing each zero with nothing.
''.join(str(x) for x in lst).replace("0","") + "0"*lst.count(0)
We will continue by appending as many zeroes as there were inside of the original list. By multiplying “0” by the amount of zeroes in the original list.
[int(y) for y in ''.join(str(x) for x in lst).replace("0","") + "0"*lst.count(0)]
Finally we will iterate through that then convert it back to a list full of integers with list comprehension. Once that is done it is returned, and now the solution is finished.
We will now look at my C solution :
#include <stddef.h>
void move_zeros(size_t len, int arr[len])
{
int p = 0;
for (size_t i = 0;i < len; i++){
if (arr[i] != 0){
arr[p] = arr[i];
if (i > p){
arr[i] = 0;
}
p++;
}
}
}
We start by including the header file stddef.h so we can use size_t (data type for an unsigned integer which is commonly used for storing the size of an array) . We can then initialize an integer at 0 named p which will be used to store which index we will move each non zero integer to. Next we iterate through the array and check if each integer is equal to zero, if so we move it to the index denoted by p. If the index we are at is higher than what p is we will start adding in zeros, so if we are done with non zero integers we can start appending zeros.
Now we will look at my lengthy C++ solution :
#include <vector>
std::vector<int> move_zeroes(const std::vector<int>& input) {
std::vector<int> newV = {};
int zeroCount = 0;
for (auto & i : input){
if (i == 0){
zeroCount ++;
} else {
newV.push_back(i);
}
}
for (int i = 0; i < zeroCount; i++){
newV.push_back(0);
}
return newV;
}
We start by including vector, we initialize a new vector with the name newV which will be our new vector. We also initialize an integer named zeroCount to 0 which will be our amount of zeros. We continue by iterating through the input vector, and each iteration we check if the element is equal to zero, if it is we increment our zero counter but if it isn’t we append it to our new vector. After we are done iterating through that we will iterate through our zero count to append zeroes. We finish off by returning our new vector.
In conclusion that is how I solved ‘Moving Zeroes To The End’ on codewars, hopefully this helps you improve in the future!
— 0xsweat 7/11/2024
My socials :
Subscribe to my newsletter
Read articles from 0xsweat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
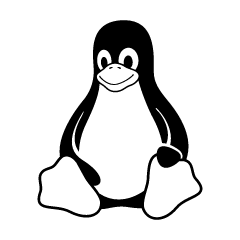
0xsweat
0xsweat
I am a machine that converts hours awake into problems solved at an exponential rate.