[TWIL] Week of June 23, 2024
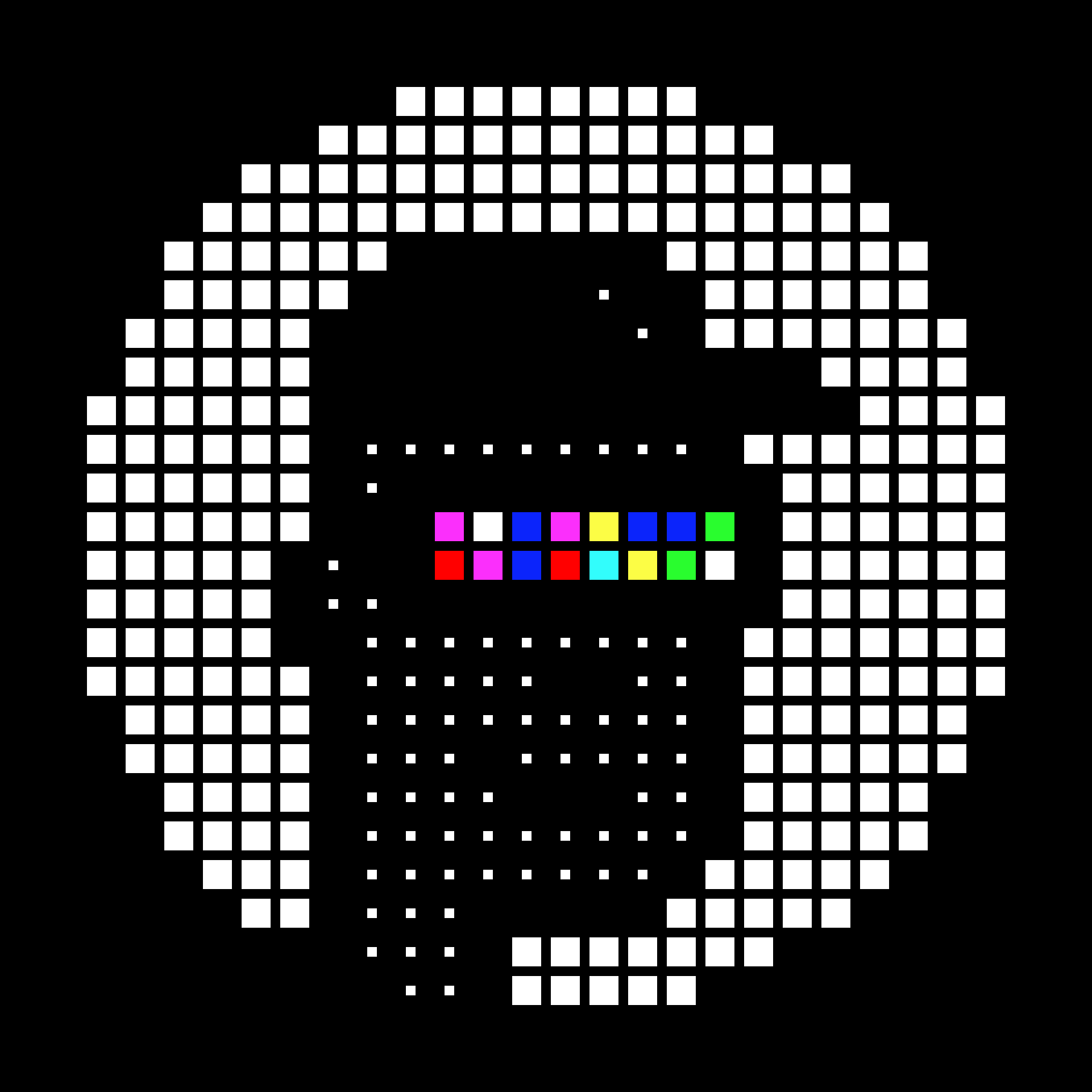
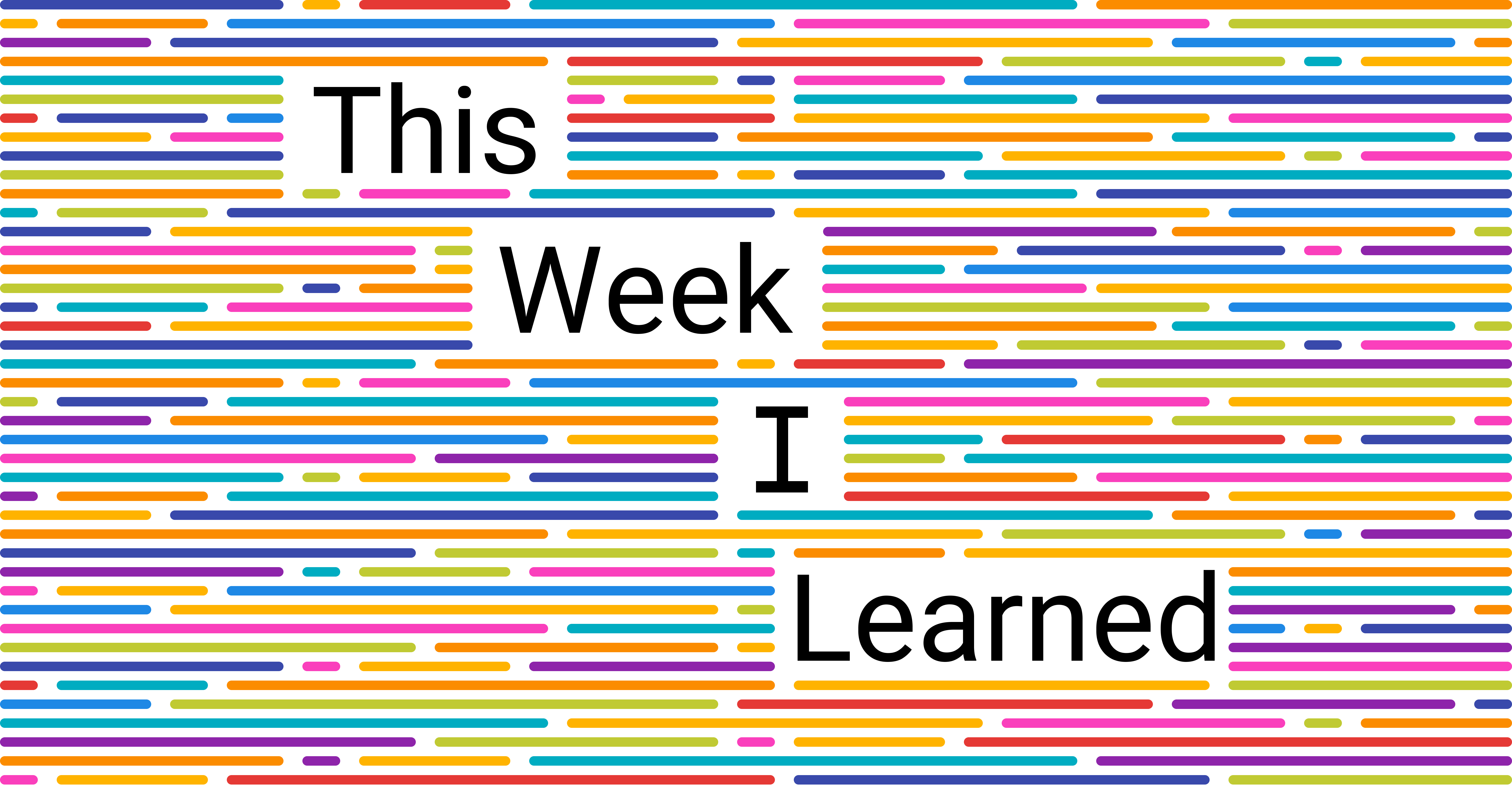
This week marked the final phase of the Onchain Summer Buildathon, where I concentrated on refactoring code and introducing a new feature for artist and visual artist collaborations. Here are some key takeaways from my work:
Alchemy Webhook Integration
Previously, artists on our web app followed these steps to mint a music NFT:
Click the "Release" button.
The button handler mints an NFT for the artist, approves the NFT marketplace smart contract to transfer the NFT, and lists the NFT on the marketplace.
The artist waits for all transactions to be validated.
Once validated, MongoDB is updated with relevant transaction details, such as the transaction hash.
This process was problematic because some artists would leave the page before transaction validation was complete. To address this issue, we needed a way to trigger a database update automatically in the background once the transaction was validated.
Fortunately, our web app uses Alchemy as the primary node provider. I discovered that Alchemy supports webhooks for various on-chain activities, such as address activity and NFT metadata updates. I opted for the NFT activity webhook, which sends transaction data to an API I created whenever an NFT transfer occurs.
I set up a webhook via the Alchemy web app so that once an NFT is minted, the transaction data is sent to the API. The API then extracts the necessary information from the request and updates the database. As a result, the new user flow is as follows:
Click the "Release" button.
The button handler mints an NFT for the artist, approves the NFT marketplace smart contract to transfer the NFT, and lists the NFT on the marketplace.
That's it! Artists no longer need to wait for transaction validation. An optimistic update is performed on the client side, while the Alchemy webhook handles transaction validation and database updates in the background.
There are other types of Alchemy webhooks available, so be sure to check out their official documentation for more details.
Solidity Custom Error
Starting from version 0.8.4, Solidity supports custom errors, which can be thrown using the revert
keyword. Here's an example:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
contract Test {
error NotOwner(address notOwner);
address owner;
constructor() {
owner = msg.sender;
}
function checkAndGetOwner(
address addr
)
public
view
returns (address)
{
if (owner != addr) {
revert NotOwner(addr);
}
return owner;
}
}
The benefits of using a custom error are twofold: it is more gas-efficient and allows for the creation of dynamic error messages.
To test this code, you can use the revertedWithCustomError
method from Chai.
import { ethers } from "hardhat";
import { expect } from "chai";
describe("Test", () => {
it("should throw error", async () => {
const [deployer, account] = await ethers.getSigners();
const test = await ethers.deployContract("Test");
await expect(test.checkAndGetOwner(account.address))
.to.be.revertedWithCustomError(test, "NotOwner")
.withArgs(account.address);
});
});
That's a wrap for this week! We've successfully completed and submitted our Onchain Summer Buildathon application. In the coming week, our focus will shift to refactoring a substantial amount of code. Stay tuned for more updates and happy hacking! ☕️
Subscribe to my newsletter
Read articles from Sean Kim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
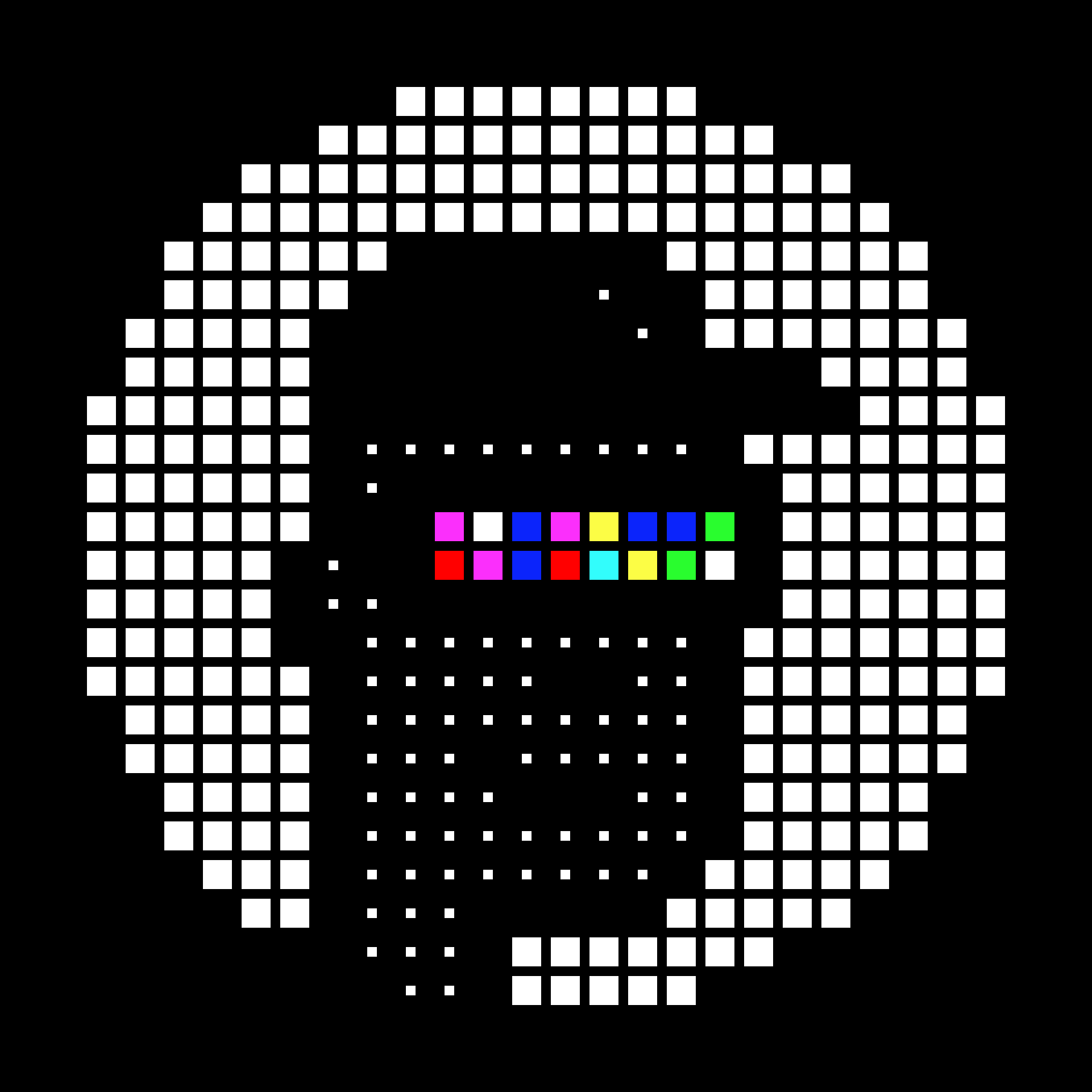
Sean Kim
Sean Kim
Engineer @ ___ | Ex-Ourkive | Ex-Meta