Day 10 Task: Log Analyzer and Report Generator

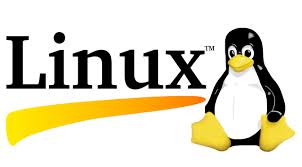
Challenge Title: Log Analyzer and Report Generator
Scenario
As a system administrator, managing a network of servers involves daily tasks such as analyzing log files generated on each server. These log files contain critical system events and error messages. To streamline this process, we will create a Bash script that automates the log file analysis and generates a summary report.
Task Breakdown
Task Overview
Input: The script should take the path to the log file as a command-line argument.
Error Count: Analyze the log file and count the number of error messages.
Critical Events: Search for lines containing the keyword "CRITICAL" and print those lines along with the line number.
Top Error Messages: Identify the top 5 most common error messages and display them along with their occurrence count.
Summary Report: Generate a summary report in a separate text file.
Optional Enhancement: Automatically archive or move processed log files to a designated directory after analysis.
The Script
Here's the complete script named log_
analyzer.sh
:
#!/bin/bash
# Check if the log file path is provided as a command-line argument
if [ -z "$1" ]; then
echo "Usage: $0 <log_file_path>"
exit 1
fi
# Assign the log file path to a variable
LOG_FILE=$1
# Check if the log file exists
if [ ! -f "$LOG_FILE" ]; then
echo "Log file not found!"
exit 1
fi
# Initialize variables
DATE=$(date +"%Y-%m-%d")
REPORT_FILE="summary_report_$DATE.txt"
ERROR_COUNT=0
CRITICAL_EVENTS=()
declare -A ERROR_MESSAGES
# Analyze the log file
while IFS= read -r LINE; do
((TOTAL_LINES++))
# Count error messages
if [[ "$LINE" == *"ERROR"* || "$LINE" == *"Failed"* ]]; then
((ERROR_COUNT++))
ERROR_MESSAGES["$LINE"]=$((ERROR_MESSAGES["$LINE"] + 1))
fi
# Search for critical events
if [[ "$LINE" == *"CRITICAL"* ]]; then
CRITICAL_EVENTS+=("$TOTAL_LINES: $LINE")
fi
done < "$LOG_FILE"
# Identify the top 5 most common error messages
TOP_ERRORS=$(for KEY in "${!ERROR_MESSAGES[@]}"; do echo "${ERROR_MESSAGES[$KEY]} $KEY"; done | sort -nr | head -5)
# Generate the summary report
{
echo "Date of Analysis: $DATE"
echo "Log File Name: $LOG_FILE"
echo "Total Lines Processed: $TOTAL_LINES"
echo "Total Error Count: $ERROR_COUNT"
echo ""
echo "Top 5 Error Messages:"
echo "$TOP_ERRORS"
echo ""
echo "List of Critical Events with Line Numbers:"
for EVENT in "${CRITICAL_EVENTS[@]}"; do
echo "$EVENT"
done
} > "$REPORT_FILE"
# Print report location
echo "Summary report generated: $REPORT_FILE"
# Optional: Archive or move the processed log file
ARCHIVE_DIR="archived_logs"
mkdir -p "$ARCHIVE_DIR"
mv "$LOG_FILE" "$ARCHIVE_DIR"
echo "Log file archived to: $ARCHIVE_DIR/$(basename "$LOG_FILE")"
Step-by-Step Explanation
Check for Command-line Argument: The script checks if a log file path is provided as an argument. If not, it displays usage instructions and exits.
Assign Log File Path to Variable: The provided log file path is assigned to the variable
LOG_FILE
.Check if Log File Exists: The script verifies if the specified log file exists.
Initialize Variables: Initialize necessary variables for date, report file, error count, critical events, and error messages.
Analyze the Log File: The script reads the log file line by line, counts error messages, identifies critical events, and tracks the most common error messages.
Identify Top 5 Error Messages: The script sorts and identifies the top 5 most common error messages.
Generate Summary Report: The script generates a summary report with the date of analysis, log file name, total lines processed, total error count, top 5 error messages, and list of critical events.
Print Report Location: The script outputs the location of the generated summary report.
Optional: Archive Processed Log File: The script optionally archives or moves the processed log file to a designated directory.
Conclusion
This script automates the log file analysis process, making it easier to manage and review system events and errors. By generating a detailed summary report and archiving processed log files, it ensures efficient and organized log management.
Happy scripting! ๐
Subscribe to my newsletter
Read articles from Himanshu Palhade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
