Shell Scripting Challenge #Day-11 : Error Handling in Shell Scripting

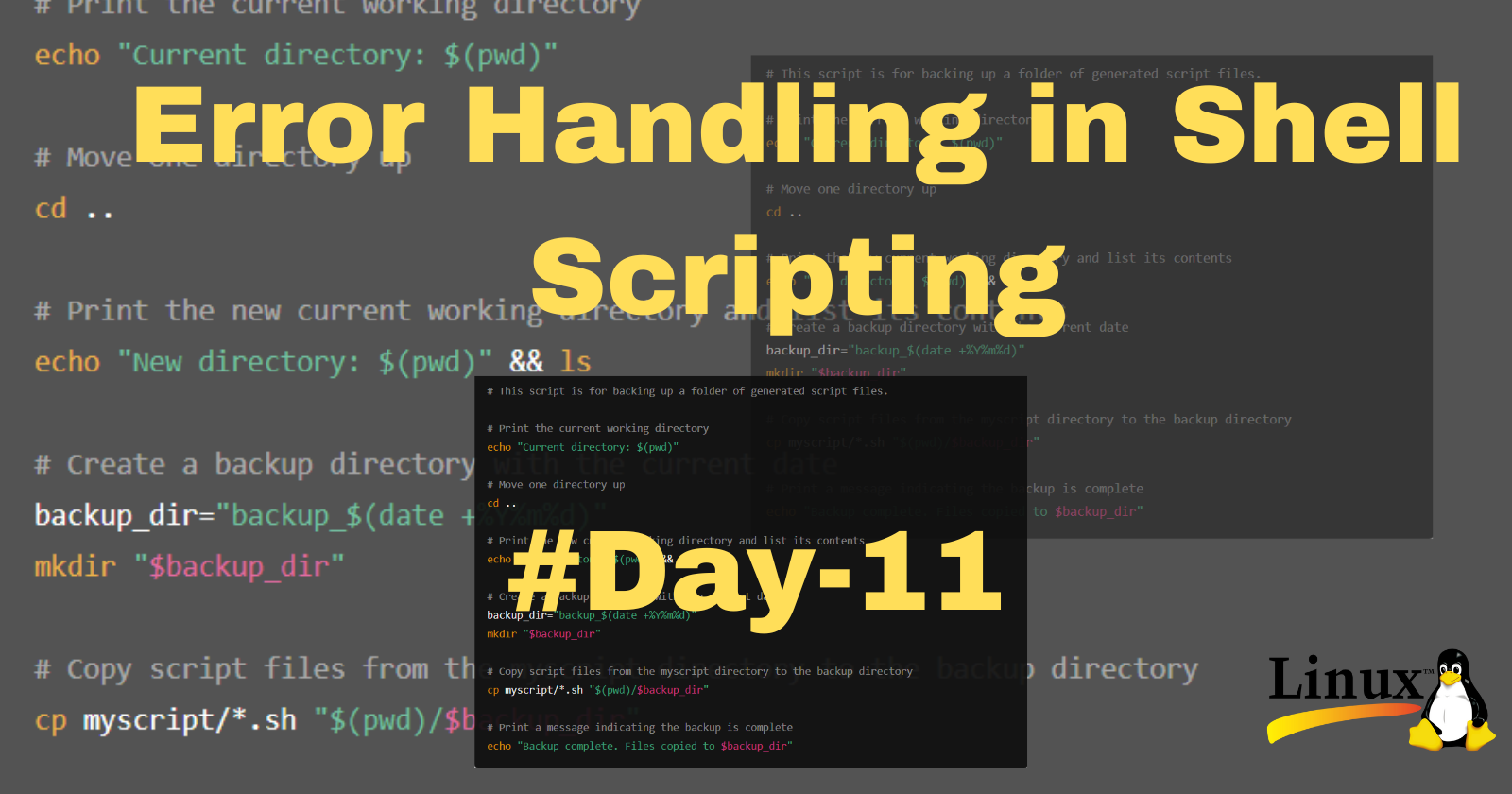
Understanding how to handle errors in shell scripts is crucial for creating robust and reliable scripts. Today, you'll learn how to use various techniques to handle errors effectively in your bash scripts.
Topics to Cover
Understanding Exit Status: Every command returns an exit status (0 for success and non-zero for failure). Learn how to check and use exit statuses.
Using
if
Statements for Error Checking: Learn how to useif
statements to handle errors.Using
trap
for Cleanup: Understand how to use thetrap
command to handle unexpected errors and perform cleanup.Redirecting Errors: Learn how to redirect errors to a file or
/dev/null
.Creating Custom Error Messages: Understand how to create meaningful error messages for debugging and user information.
Tasks
Task 1: Checking Exit Status
- Write a script that attempts to create a directory and checks if the command was successful. If not, print an error message.
#!/bin/bash
mkdir /tmp/mydir
if [ $? -ne 0 ]; then
echo "Failed to create directory /tmp/mydir"
fi
Result:
Task 2: Using if
Statements for Error Checking
- Modify the script from Task 1 to include more commands (e.g., creating a file inside the directory) and use
if
statements to handle errors at each step.
#!/bin/bash
mkdir /tmp/mydir1
if [ $? -ne 0 ]; then
echo "Failed to create directory /tmp/mydir"
else
echo "Directory Created Successfully."
fi
Result:
Task 3: Using trap
for Cleanup
- Write a script that creates a temporary file and sets a
trap
to delete the file if the script exits unexpectedly.
#!/bin/bash
tempfile=$(mktemp)
trap "rm -f $tempfile" EXIT
echo "This is a temporary file." > $tempfile
cat $tempfile
# Simulate an error
exit 1
Result:
Task 4: Redirecting Errors
- Write a script that tries to read a non-existent file and redirects the error message to a file called
error.log
.
In general, here are some common redirection operators in bash:
>
: Redirects standard output (file descriptor 1).2>
: Redirects standard error (file descriptor 2).&>
: Redirects both standard output and standard error to the same file.
#!/bin/bash
cat non_existent_file.txt 2> error.log
Result:
Task 5: Creating Custom Error Messages
- Modify one of the previous scripts to include custom error messages that provide more context about what went wrong.
#!/bin/bash
mkdir /tmp/mydir
if [ $? -ne 0 ]; then
echo "Error: Directory /tmp/mydir could not be created. Check if you have the necessary permissions."
fi
Result:
Conclusion
Mastering error handling in shell scripting is essential for developing robust and reliable scripts. By understanding exit statuses, using if
statements for error checking, employing trap
for cleanup, redirecting errors, and creating custom error messages, you can significantly enhance the stability and maintainability of your scripts. These techniques not only help in debugging but also ensure that your scripts can handle unexpected situations gracefully. Happy scripting!
Thanks for reading. Happy Learning !!
Connect and Follow Me On Socials :
Like👍 | Share📲 | Comment💭
Subscribe to my newsletter
Read articles from Nikunj Vaishnav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nikunj Vaishnav
Nikunj Vaishnav
👋 Hi there! I'm Nikunj Vaishnav, a passionate QA engineer Cloud, and DevOps. I thrive on exploring new technologies and sharing my journey through code. From designing cloud infrastructures to ensuring software quality, I'm deeply involved in CI/CD pipelines, automated testing, and containerization with Docker. I'm always eager to grow in the ever-evolving fields of Software Testing, Cloud and DevOps. My goal is to simplify complex concepts, offer practical tips on automation and testing, and inspire others in the tech community. Let's connect, learn, and build high-quality software together! 📝 Check out my blog for tutorials and insights on cloud infrastructure, QA best practices, and DevOps. Feel free to reach out – I’m always open to discussions, collaborations, and feedback!