Ultimate Guide to Kubernetes YAML Files: Detailed Breakdown & Use Cases

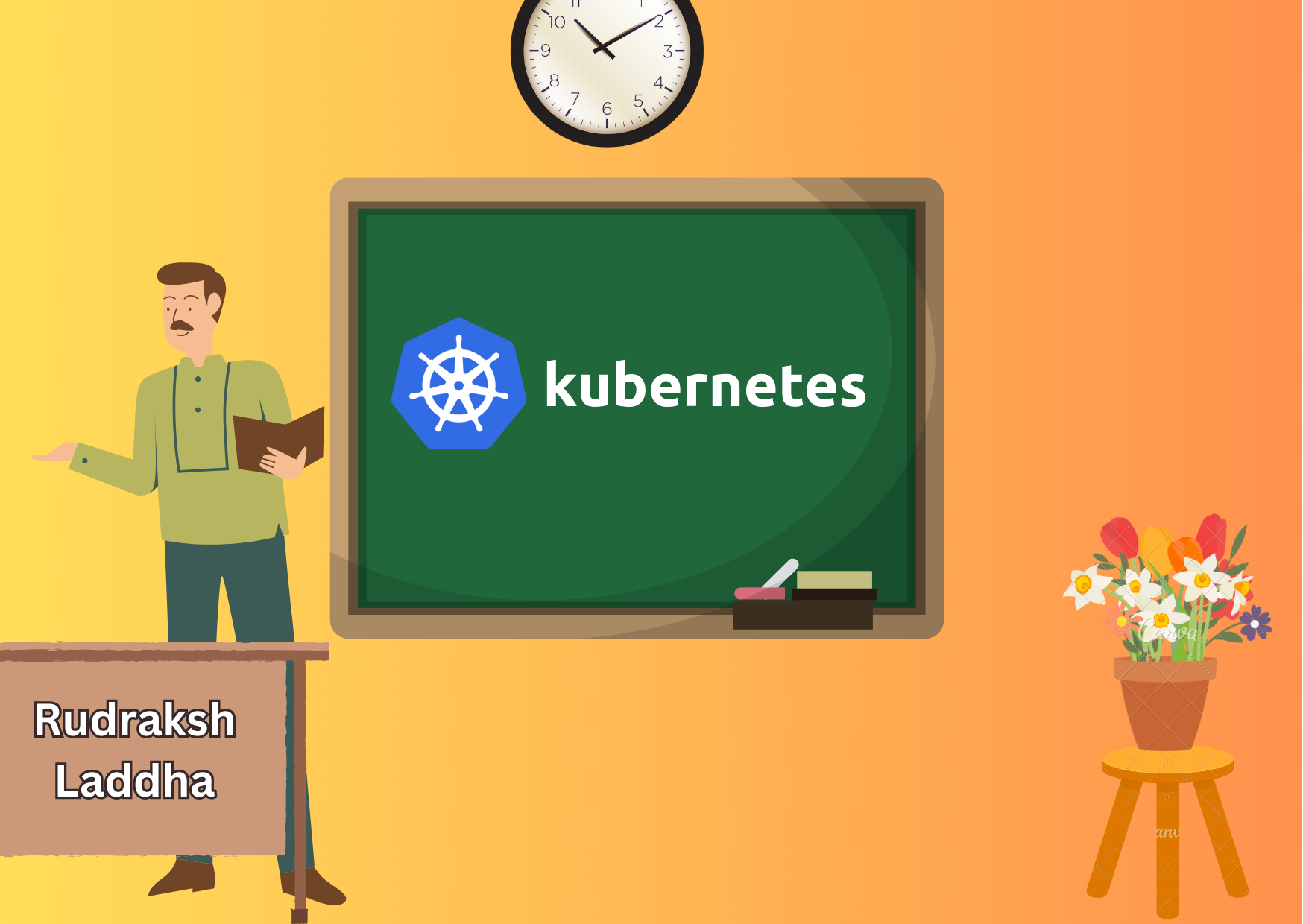
Kubernetes YAML files are the cornerstone of configuring and managing applications within a Kubernetes cluster. This article will break down the components of several common Kubernetes YAML files, explaining each term and its use case. By the end, you will have a solid understanding of how to read and write these files to effectively manage your Kubernetes resources.
Basic Structure of a Kubernetes YAML File
A Kubernetes YAML file is a plain text file that defines the desired state of a Kubernetes object. These files are essential for managing applications and services within a Kubernetes cluster. Let’s dive into various types of Kubernetes YAML files and understand each part in detail.
Deployment YAML Example
A Deployment manages a set of replicated Pods, ensuring that a specified number of them are running at all times.
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deployment
labels:
app: my-app
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-container
image: my-image:latest
ports:
- containerPort: 80
Explanation of Each Part
apiVersion: apps/v1
Description: Specifies the API version of the Kubernetes object. The
apps/v1
indicates that the Deployment object is using versionv1
of theapps
API group.Use Case: Ensures compatibility with the Kubernetes API server.
kind: Deployment
Description: Defines the type of Kubernetes object being created. In this case, it is a
Deployment
.Use Case: Tells Kubernetes to create a Deployment resource, which manages a set of replicated Pods.
metadata:
name: my-deployment
Description: Specifies the name of the Deployment.
Use Case: Provides a unique identifier for the Deployment within the namespace.
labels:
app: my-app
Description: Defines labels for the Deployment.
Use Case: Helps in organizing and selecting resources based on these labels.
spec:
replicas: 3
Description: Defines the number of Pod replicas to be maintained.
Use Case: Ensures that the desired number of Pod instances are running at all times.
selector:
matchLabels:
app: my-app
Description: Specifies the label selector for identifying Pods managed by the Deployment.
Use Case: Ensures the Deployment manages Pods with the specified labels.
template:
metadata:
labels:
app: my-app
Description: Defines labels for the Pods created by the Deployment.
Use Case: Ensures that Pods have the correct labels to match the selector.
spec:
containers:
- name: my-container
Description: Specifies the name of the container within the Pod.
Use Case: Identifies the container in logs and other outputs.
image: my-image:latest
Description: Defines the container image to be used.
Use Case: Specifies which application version to deploy in the container.
ports:
- containerPort: 80
Description: Exposes the specified port within the container.
Use Case: Makes the application accessible on the given port.
Service YAML Example
A Service exposes an application running on a set of Pods as a network service.
apiVersion: v1
kind: Service
metadata:
name: my-service
spec:
selector:
app: my-app
ports:
- protocol: TCP
port: 80
targetPort: 80
Explanation of Each Part
apiVersion: v1
Description: Specifies the API version of the Kubernetes object.
v1
is used for core resources like Services.Use Case: Ensures compatibility with the Kubernetes API server.
kind: Service
Description: Defines the type of Kubernetes object being created, which in this case is a Service.
Use Case: Tells Kubernetes to create a Service resource to expose an application running on a set of Pods.
metadata:
name: my-service
Description: Specifies the name of the Service.
Use Case: Provides a unique identifier for the Service within the namespace.
spec:
selector:
app: my-app
Description: Defines the label selector to identify the Pods targeted by this Service.
Use Case: Ensures the Service directs traffic to the correct set of Pods.
ports:
- protocol: TCP
Description: Specifies the protocol used by the Service port.
Use Case: Defines the protocol (e.g., TCP, UDP) for the Service.
port: 80
Description: Defines the port that the Service exposes.
Use Case: The port through which external clients can access the Service.
targetPort: 80
Description: Specifies the port on the container to which the Service should forward traffic.
Use Case: Directs traffic to the specified container port.
ConfigMap YAML Example
A ConfigMap is used to store non-confidential data in key-value pairs.
apiVersion: v1
kind: ConfigMap
metadata:
name: my-config
data:
key1: value1
key2: value2
Explanation of Each Part
apiVersion: v1
Description: Specifies the API version of the Kubernetes object.
v1
is used for core resources like ConfigMaps.Use Case: Ensures compatibility with the Kubernetes API server.
kind: ConfigMap
Description: Defines the type of Kubernetes object being created, which in this case is a ConfigMap.
Use Case: Tells Kubernetes to create a ConfigMap resource for storing non-confidential configuration data.
metadata:
name: my-config
Description: Specifies the name of the ConfigMap.
Use Case: Provides a unique identifier for the ConfigMap within the namespace.
data:
key1: value1
key2: value2
Description: Defines key-value pairs of configuration data.
Use Case: Stores configuration data that can be consumed by Pods.
Secret YAML Example
A Secret is used to store sensitive data, such as passwords and API keys, in a secure manner.
apiVersion: v1
kind: Secret
metadata:
name: my-secret
type: Opaque
data:
key1: dmFsdWUx
key2: dmFsdWUy
Explanation of Each Part
apiVersion: v1
Description: Specifies the API version of the Kubernetes object.
v1
is used for core resources like Secrets.Use Case: Ensures compatibility with the Kubernetes API server.
kind: Secret
Description: Defines the type of Kubernetes object being created, which in this case is a Secret.
Use Case: Tells Kubernetes to create a Secret resource for storing sensitive data.
metadata:
name: my-secret
Description: Specifies the name of the Secret.
Use Case: Provides a unique identifier for the Secret within the namespace.
type: Opaque
Description: Defines the type of the Secret.
Opaque
indicates a generic secret.Use Case: Specifies the type of data the Secret holds.
data:
key1: dmFsdWUx
key2: dmFsdWUy
Description: Defines base64-encoded key-value pairs of sensitive data.
Use Case: Stores sensitive data that can be consumed by Pods.
Ingress YAML Example
An Ingress manages external access to services in a Kubernetes cluster, typically HTTP.
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: my-ingress
spec:
rules:
- host: my-app.example.com
http:
paths:
- path: /
pathType: Prefix
backend:
service:
name: my-service
port:
number: 80
Explanation of Each Part
apiVersion: networking.k8s.io/v1
Description: Specifies the API version of the Kubernetes object.
networking.k8s.io/v1
is used for networking resources like Ingress.Use Case: Ensures compatibility with the Kubernetes API server.
kind: Ingress
- Description: Defines the type of Kubernetes object being created, which in this case is an
Ingress.
- Use Case: Tells Kubernetes to create an Ingress resource for managing external access to services.
metadata:
name: my-ingress
Description: Specifies the name of the Ingress.
Use Case: Provides a unique identifier for the Ingress within the namespace.
spec:
rules:
- host: my-app.example.com
Description: Defines the hostname for routing HTTP traffic.
Use Case: Specifies the domain name for the application.
http:
paths:
- path: /
Description: Defines the path for routing HTTP traffic.
Use Case: Specifies the URL path for the application.
pathType: Prefix
Description: Specifies the type of path matching.
Use Case: Ensures traffic is routed based on URL prefix.
backend:
service:
name: my-service
Description: Defines the backend service to route traffic to.
Use Case: Specifies the service handling the traffic.
port:
number: 80
Description: Defines the port on the service to route traffic to.
Use Case: Specifies the service port handling the traffic.
Conclusion
Understanding Kubernetes YAML files is crucial for managing resources within a Kubernetes cluster. By breaking down each component of common YAML files like Deployments, Services, ConfigMaps, Secrets, and Ingresses, you can effectively configure and manage your applications. With this knowledge, you are well-equipped to write and understand Kubernetes YAML files, ensuring your applications run smoothly within a Kubernetes environment.
If you find this article helpful, please consider sponsoring me for more in-depth articles and contributions. Your support helps me create even better content! 🙌
Subscribe to my newsletter
Read articles from Rudraksh Laddha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rudraksh Laddha
Rudraksh Laddha
DevOps Engineer || Technical Writer || Content Creator || | Adventurer chasing dreams, capturing life's kaleidoscope. || 🎓UCET '24 || Dm for Collabs📥||