Understanding `call()`, `apply()`, and `bind()` Methods in JavaScript

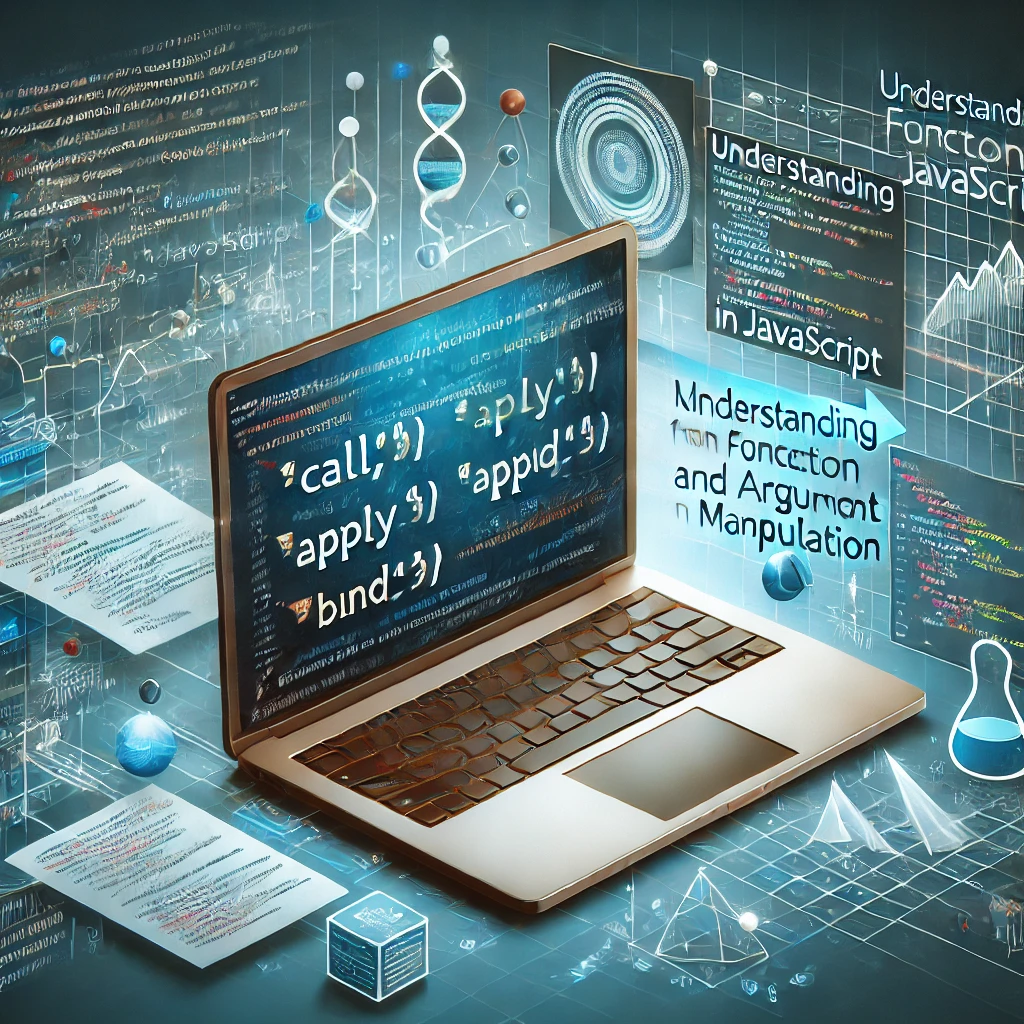
JavaScript provides several methods to control the this
context in functions, object methods, and event handlers. The most common methods are call()
, apply
, and bind()
. In this blog, we'll explore these methods with practical examples.
call()
Method
The call()
method invokes a function with a specified this
value and individual arguments.
Example: Using call()
for Computations
You can use call()
to invoke Math functions with a specific context. For example, you can find the maximum value in an array:
const numbers = [10, 20, 30, 40, 50];
const max = Math.max.call(null, ...numbers);
console.log(max); // 50
In this example, Math.max.call
(null, ...numbers)
passes the elements of numbers
as individual arguments to Math.max
.
Example: Borrowing Methods
You can use call()
to borrow methods from other objects.
const person = {
fullName: function() {
return `${this.firstName} ${this.lastName}`;
}
};
const person1 = {
firstName: "Alice",
lastName: "Johnson"
};
console.log(person.fullName.call(person1)); // Alice Johnson
Here, call()
sets this
to person1
, allowing the fullName
method to access person1
's properties.
apply()
Method
The apply()
method is similar to call()
, but it takes arguments as an array.
Example: Using Math Functions
You can use apply()
to pass an array of arguments to a function like Math.max
.
const numbers = [10, 5, 20, 15];
const max = Math.max.apply(null, numbers);
console.log(max); // 20
In this example, Math.max.apply(null, numbers)
passes the elements of numbers
as individual arguments to Math.max
.
Example: Using Built-in Methods
You can use apply()
to borrow array methods for array-like objects.
function list() {
return Array.prototype.slice.apply(arguments);
}
console.log(list('a', 'b', 'c')); // ['a', 'b', 'c']
Here, Array.prototype.slice.apply(arguments)
converts the arguments
object into an array.
bind()
Method
The bind()
method creates a new function that, when called, has its this
keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
Example: Partial Application
You can use bind()
for partial application, creating a function with preset initial arguments.
function multiply(a, b) {
return a * b;
}
const double = multiply.bind(null, 2);
console.log(double(5)); // 10
const triple = multiply.bind(null, 3);
console.log(triple(5)); // 15
In this example, double
is a version of multiply
with a
preset to 2
, and triple
has a
preset to 3
.
Example: Event Handling in Classes
bind()
is often used to ensure the correct this
context in event handlers, especially within classes.
class Button {
constructor(label) {
this.label = label;
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
console.log(`Button ${this.label} clicked`);
}
render() {
const button = document.createElement('button');
button.innerText = this.label;
button.addEventListener('click', this.handleClick);
document.body.appendChild(button);
}
}
const button1 = new Button('Submit');
button1.render();
Here, this.handleClick.bind(this)
ensures that this
refers to the Button instance when handleClick
is called.
Using bind()
to Create Specialized Functions
bind()
can also be used to create specialized functions with preset arguments, useful for scenarios like region-specific calculations.
Example: Calculating Sales Tax
Suppose you have a function calculateSalesTax(taxRate, price)
that calculates the sales tax for a given price. You can use bind()
to create specialized versions of this function for different tax rates.
function calculateSalesTax(taxRate, price) {
return price * taxRate;
}
const salesTaxCanada = calculateSalesTax.bind(null, 0.05);
const salesTaxUS = calculateSalesTax.bind(null, 0.07);
const salesTaxUK = calculateSalesTax.bind(null, 0.2);
console.log(salesTaxCanada(100)); // 5
console.log(salesTaxUS(100)); // 7
console.log(salesTaxUK(100)); // 20
In this example, salesTaxCanada
, salesTaxUS
, and salesTaxUK
are specialized versions of the calculateSalesTax
function with the tax rate preset for Canada, the US, and the UK, respectively.
Conclusion
Understanding how to use call()
, apply()
, and bind()
methods in JavaScript is crucial for controlling the this
context and manipulating function arguments. These methods provide powerful tools for creating flexible and reusable code.
call()
: Use to invoke a function with a specificthis
value and individual arguments.apply()
: Use to invoke a function with a specificthis
value and arguments provided as an array.bind()
: Use to create a new function with a specificthis
value and preset arguments.
These methods are not only essential for controlling the this
context but also for various practical use cases such as computations and creating specialized function versions.
Subscribe to my newsletter
Read articles from Engr. Animashaun Fisayo Michael directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Engr. Animashaun Fisayo Michael
Engr. Animashaun Fisayo Michael
Frontend Developer | Javascript programmer | Registered Mechanical Engineer (MNSE, COREN) | Facilities Management Technologies Developer