Hashing and Salting of passwords in Node.js
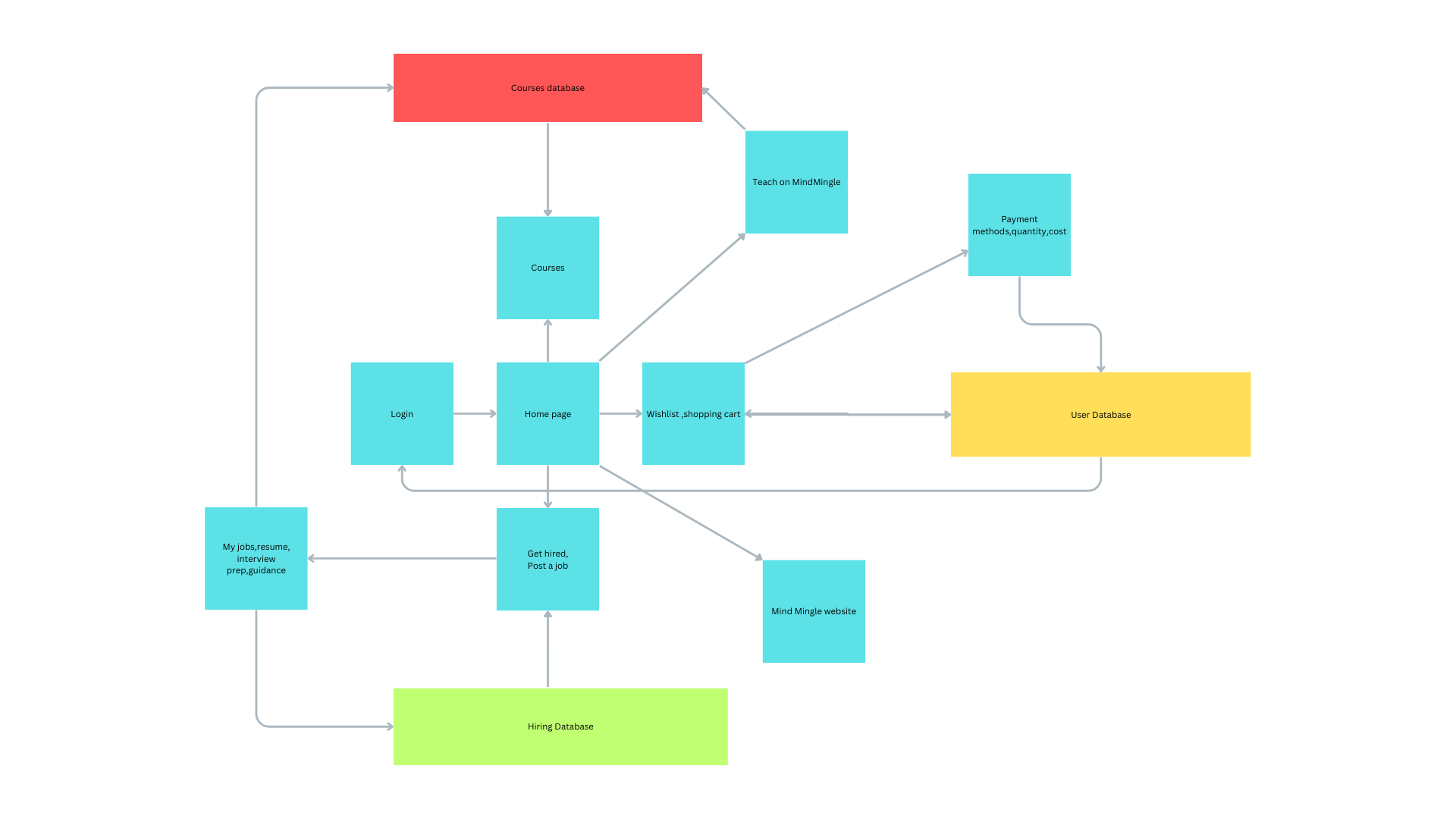
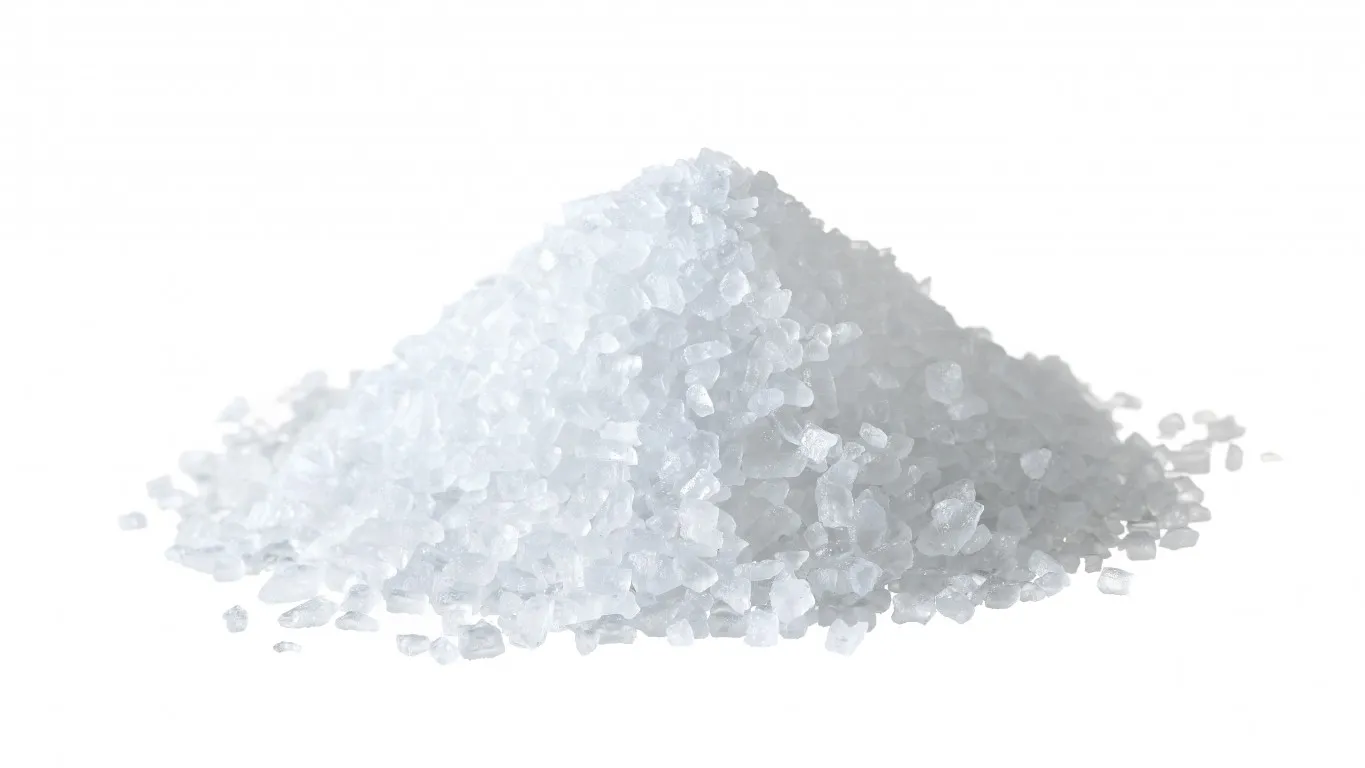
In web applications, securing user passwords is crucial. Hashing and salting are two methods that greatly improve password security by making it harder for hackers to access user credentials.
What is Hashing?
Hashing turns plain-text passwords into a fixed-length string of characters using a special function. This process is irreversible, meaning you can't get the original password from the hash.
Why Just Hashing Isn't Enough?
Hashed passwords can still be vulnerable to attacks that use precomputed lists of hashes to guess passwords quickly. To counter this, we use salting.
What is Salting?
Salting involves adding a random value (salt) to each password before hashing it. This randomness ensures that even if two users have the same password, their hashed passwords look different.
How to Implement Hashing and Salting in Node.js
Setting Up
First, make sure you have Node.js installed. Then, install the bcrypt
library:
bashCopy codenpm install bcrypt
Creating a Hashing Function
Here's how you can create a function to hash passwords using bcrypt:
javascriptCopy codeconst bcrypt = require('bcrypt');
const saltRounds = 10;
async function hashPassword(password) {
try {
const salt = await bcrypt.genSalt(saltRounds);
const hash = await bcrypt.hash(password, salt);
return hash;
} catch (error) {
throw new Error('Hashing failed', error);
}
}
Adding Salting
To add salting, generate a salt and use it with bcrypt.hash()
:
javascriptCopy codeasync function hashPasswordWithSalt(password) {
try {
const salt = await bcrypt.genSalt(saltRounds);
const hash = await bcrypt.hash(password, salt);
return hash;
} catch (error) {
throw new Error('Hashing failed', error);
}
}
Storing Passwords
When storing hashed and salted passwords in your database, make sure the field can store the generated hash safely.
Conclusion
By hashing and salting passwords with bcrypt in your Node.js applications, you greatly enhance security against password attacks. These simple steps help protect user accounts from unauthorized access and ensure compliance with security standards.
Further Reading
OWASP Password Storage Guide
Subscribe to my newsletter
Read articles from Rahul Boney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
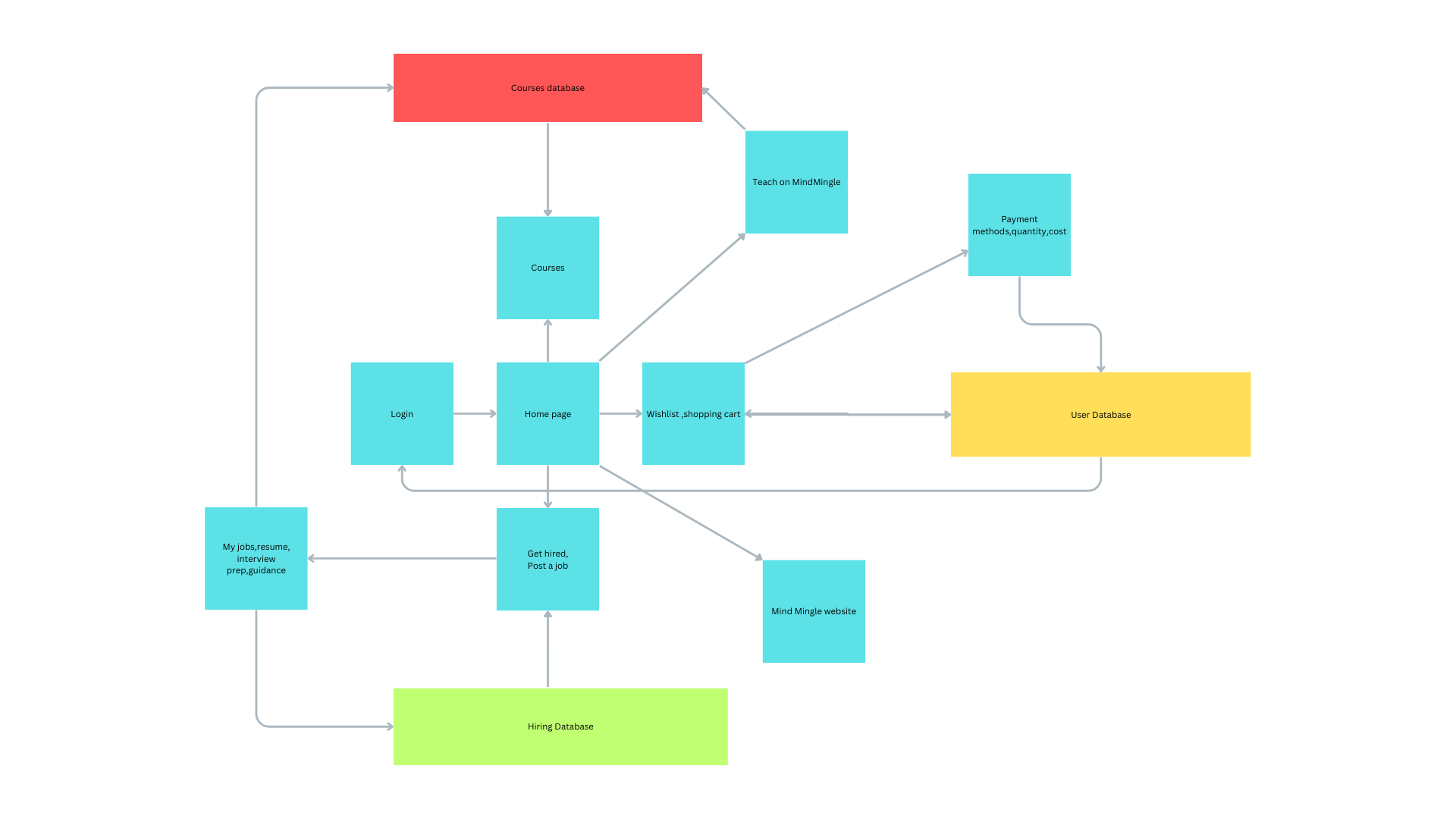
Rahul Boney
Rahul Boney
Hey, I'm Rahul Boney, really into Computer Science and Engineering. I love working on backend development, exploring machine learning, and diving into AI. I am always excited about learning and building new things.