EJS vs. HTML: How Dynamic and Static Content Differ
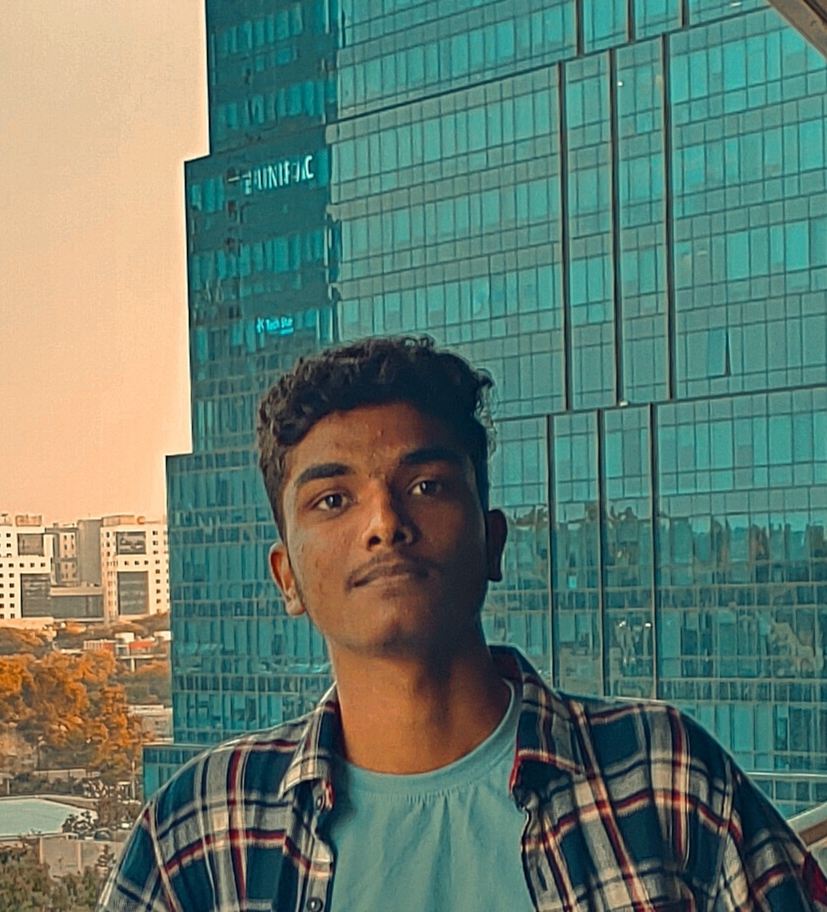
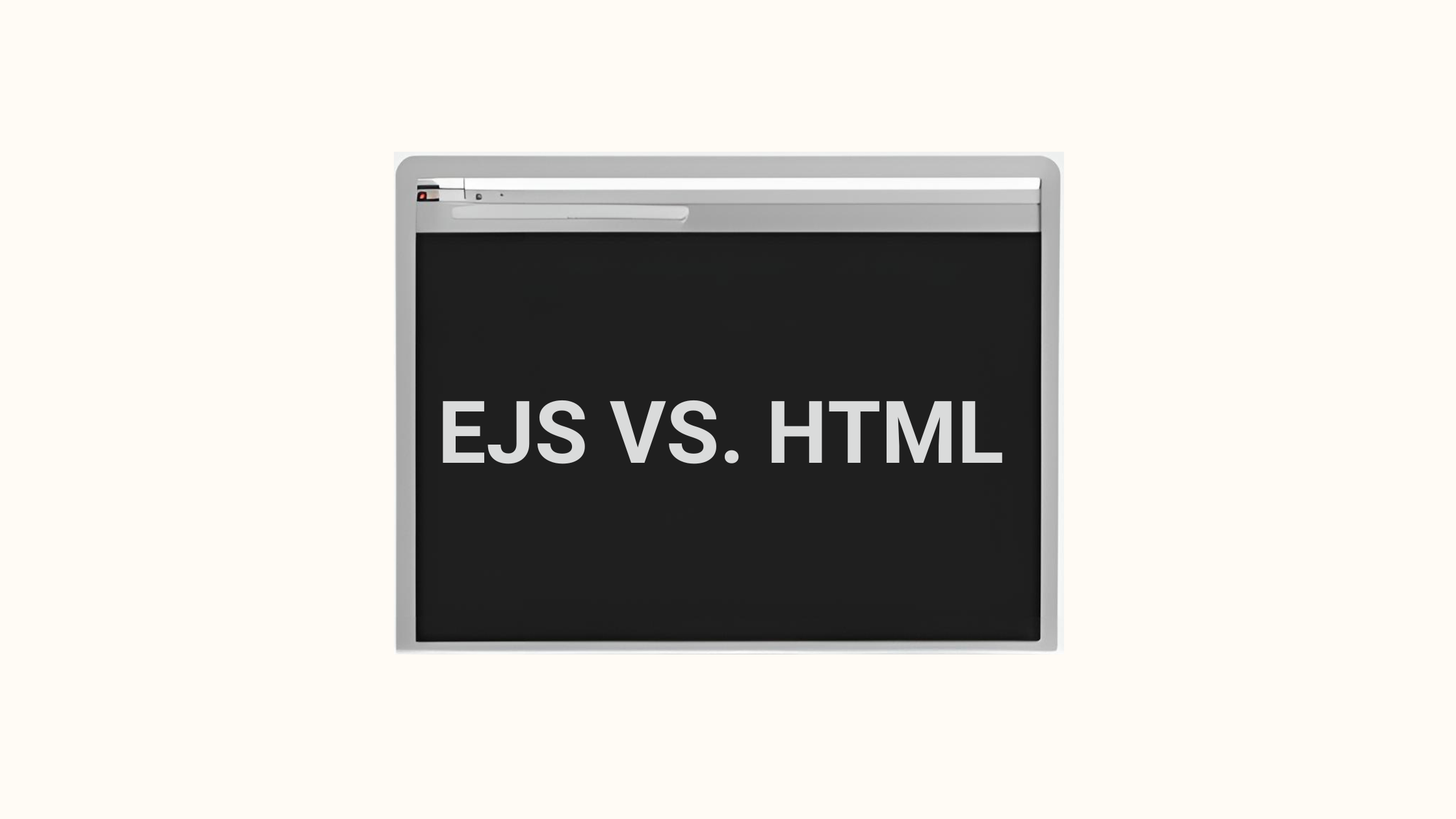
Selecting the appropriate tools and technologies in web development is crucial for your project's success. EJS (Embedded JavaScript) and plain HTML are two popular choices for rendering web pages. Although both serve the purpose of creating web pages, they have distinct features and applications. Let's explore what distinguishes EJS from HTML and when you might choose one over the other.
What is HTML?
HTML (HyperText Markup Language) is the foundational markup language used to create web pages. It provides the structure of a webpage by defining elements such as headings, paragraphs, links, images, and forms. HTML is static, meaning that once a page is loaded, its content does not change unless a new request is made to the server or JavaScript modifies the DOM.
Sample HTML Code
Here’s a simple example of an HTML page:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sample HTML Page</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a static HTML page.</p>
</body>
</html>
What is EJS?
EJS (Embedded JavaScript) is a templating language that allows you to embed JavaScript code directly within your HTML. It is often used in server-side applications to generate dynamic web pages. With EJS, you can create reusable templates and dynamically insert data into your HTML, making it a powerful tool for web developers.
Sample EJS Code
Here’s a simple example of an EJS template:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sample EJS Page</title>
</head>
<body>
<h1>Welcome to <%= title %></h1>
<p>This is a dynamic EJS page.</p>
<ul>
<% users.forEach(function(user){ %>
<li><%= user.name %></li>
<% }); %>
</ul>
</body>
</html>
Key Differences Between EJS and HTML
Static vs. Dynamic Content
HTML: Produces static content that remains unchanged unless modified by JavaScript or reloaded from the server.
EJS: Produces dynamic content by embedding JavaScript code within the HTML, allowing you to insert data and logic directly into your templates.
Data Binding
HTML: Lacks built-in support for data binding. You need to use JavaScript or a framework like React or Angular to dynamically update the content.
EJS: Offers built-in support for data binding. You can easily pass data from your server to your EJS templates and render it dynamically.
Server-Side Rendering
HTML: Typically used for client-side rendering, where the browser processes the HTML, CSS, and JavaScript to display the page.
EJS: Primarily used for server-side rendering. The server processes the EJS templates, generates the HTML with embedded data, and sends the final HTML to the client.
Logic and Control Structures
HTML: Does not support logic or control structures. You need to use JavaScript to handle conditions, loops, and other logic.
EJS: Supports JavaScript logic and control structures directly within the templates. You can use conditionals, loops, and functions to control the rendering of your HTML.
Benefits of Using EJS
Separation of Concerns
EJS allows you to separate your HTML structure from your application logic. By embedding JavaScript code within your templates, you can keep your HTML clean and maintainable while handling dynamic data and logic in a more organized way.
Reusability
EJS promotes reusability by allowing you to create partials and layouts. Partials are reusable snippets of code that you can include in multiple templates, while layouts provide a consistent structure for your pages. This helps reduce redundancy and makes your codebase easier to manage.
Ease of Use
For developers familiar with JavaScript, EJS is easy to learn and use. Its syntax is similar to HTML, with additional JavaScript capabilities, making it a smooth transition for those already comfortable with front-end development.
Integration with Node.js
EJS is a popular choice for Node.js applications. It integrates seamlessly with Express, a widely used Node.js web framework, allowing you to build powerful server-side applications with minimal setup.
When to Use HTML
Static Websites: For simple static websites that do not require dynamic content, plain HTML is sufficient.
Client-Side Applications: When building client-side applications with frameworks like React, Angular, or Vue, plain HTML is often used alongside these frameworks for templating.
When to Use EJS
Server-Side Applications: When building server-side applications with Node.js and Express, EJS is an excellent choice for rendering dynamic web pages.
Dynamic Content: When your application requires dynamic content that changes based on user input or server-side data, EJS simplifies the process of generating and updating your HTML.
Conclusion
Both EJS and HTML have their place in web development, each serving different needs and use cases. While HTML is the foundation of web pages, EJS enhances its capabilities by enabling dynamic content and server-side rendering. By understanding the differences and benefits of each, you can make informed decisions about which technology to use in your projects, ultimately leading to more efficient and maintainable code.
Subscribe to my newsletter
Read articles from Gondi Bharadhwaj Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
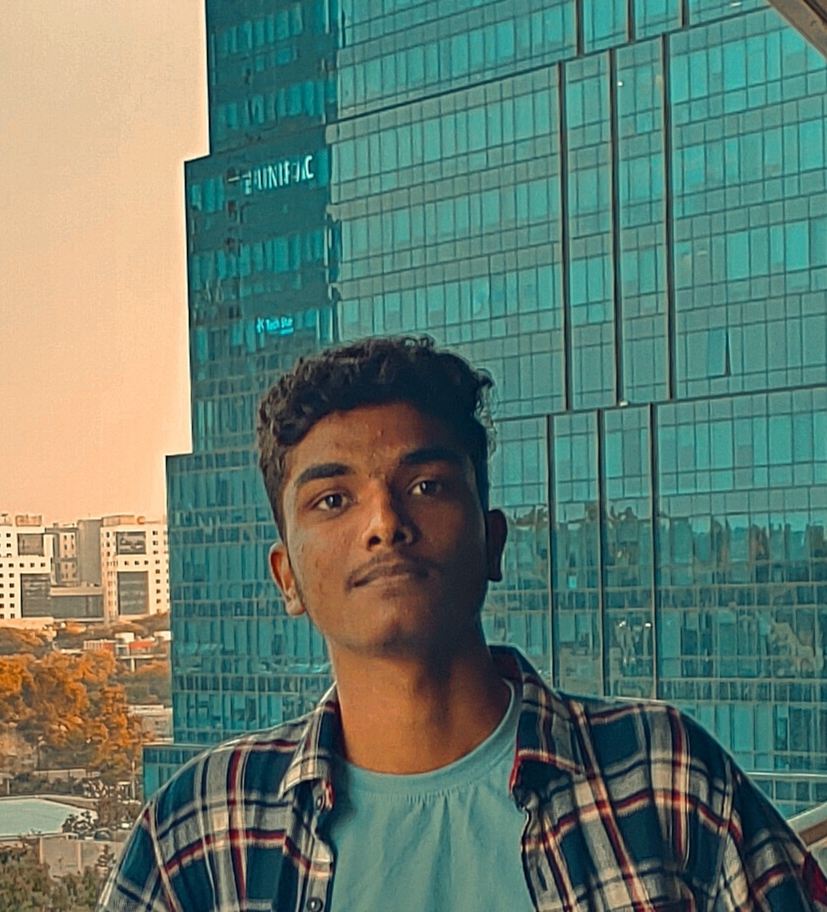
Gondi Bharadhwaj Reddy
Gondi Bharadhwaj Reddy
I am a dedicated undergraduate student passionate about exploring and learning new things. My interests span across various fields, from technology and web development. Currently focused on projects that enhance learning experiences and make meaningful contributions to communities.