Python Basics: Lists, Indexing, List Methods, and Tuples
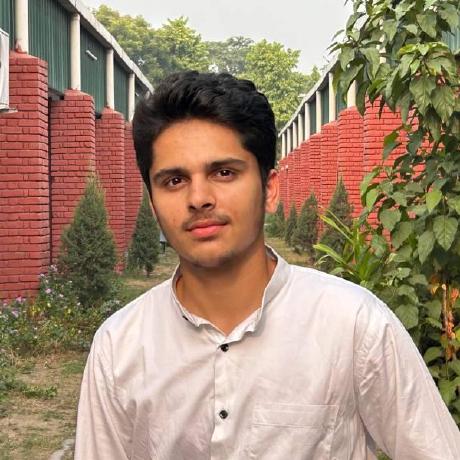
Lists
- What is a List?
A list in Python is a collection of items that are ordered and changeable. Lists allow duplicate members.
- Creating a List
# Creating a list of fruits
fruits = ["apple", "banana", "cherry"]
Indexing in Lists
Indexing allows you to access individual elements in a list. Python uses zero-based indexing.
# Accessing the first element
print(fruits[0]) # Output: apple
# Accessing the last element
print(fruits[-1]) # Output: cherry
List Methods
Python provides several methods to work with lists. Here are some common ones:
append() - Adds an element to the end of the list.
fruits.append("orange") print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
remove() - Removes the first item with the specified value.
fruits.remove("banana") print(fruits) # Output: ['apple', 'cherry', 'orange']
pop() - Removes the element at the specified position (default is the last item).
fruits.pop()
print(fruits) # Output: ['apple', 'cherry'
Tuples
What is a Tuple?
A tuple is a collection of items that are ordered and unchangeable (immutable). Tuples allow duplicate members.
Creating a Tuple
# Creating a tuple of vegetables vegetables = ("carrot", "broccoli", "spinach")
Indexing in Tuples
Just like lists, tuples also use zero-based indexing.
# Accessing the first element print(vegetables[0]) # Output: carrot # Accessing the last element print(vegetables[-1]) # Output: spinach
Tuple Methods
Tuples have fewer methods compared to lists due to their immutable nature. Here are two useful methods:
count() - Returns the number of times a specified value occurs in a tuple
count_carrot = vegetables.count("carrot") print(count_carrot) # Output: 1
index() - Searches the tuple for a specified value and returns the position of where it was found
index_broccoli = vegetables.index("broccoli")
print(index_broccoli) # Output: 1
Conclusion
Understanding lists and tuples is fundamental to becoming proficient in Python. Lists are flexible and allow for dynamic modification, making them perfect for collections of items that may change. Tuples, on the other hand, provide a way to store immutable collections, ensuring the data remains constant throughout the program.
Happy Coding!
Subscribe to my newsletter
Read articles from Prince Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
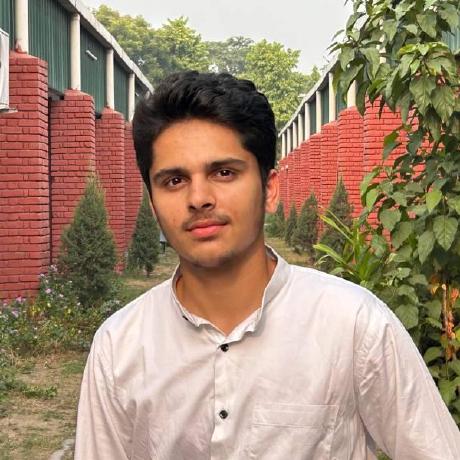