Python List Slicing Explained for Beginners
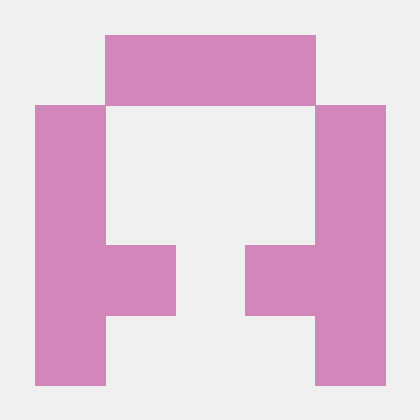
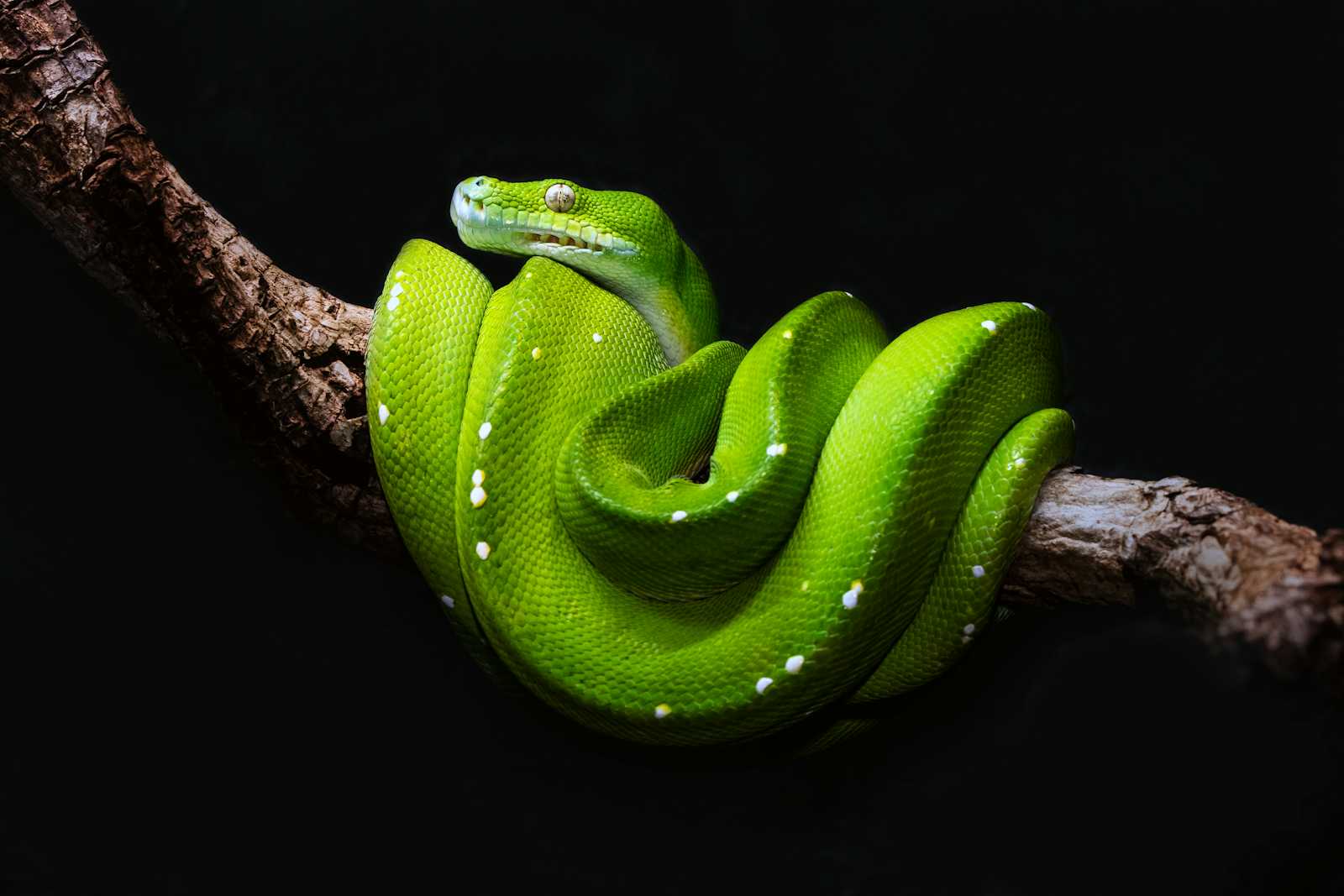
What is slicing
As we know that in Lists we use [] to return an item at that particular index
list = ["python","java","javascript"]
print(list[0]) #It prints the returned item of the index in the list
But if you want return list of items of a particular range from the List then slicing comes in handy.
Definition - Slice operation returns a new list of items that you have requested,this also means that it returns a shallow copy of the list.
You might be wondering what is a shallow copy, but don't worry I will be covering it in detail in this article itself, just read on.
Basic syntax of slicing is as follows -
list = ["python","java","javascript"]
print(list[starting_index:end_index])
In the above syntax starting_index represents the index of the first item in the list that you want and the end_index represents index of the last item of the list(excluding the item at the end_index*)* that you want to return.
list = ["python","java","javascript"]
new_list = list[0:2] #returns a new list - ["python","java"]
print(new_list) #prints the new_list
In the above code slicing is used to obtain python and java items of the list.
you can leave the starting and ending indexes vacant, so that all the items from the beginning and the end can be included.
list = ["python","java","javascript"]
print(list[1:]) #It prints - ["java","javascript"]
print(list[:2] #It prints - ["python","java"]
print(list[:]) #it prints - ["python","java","javascript"]
You can also use negative indexes to return the items from back of the list, -1 represents the last element of the list from back.
list = ["python","java","javascript"]
print(list[-1:-3]) #It prints - nothing
It does not prints anything because in slicing first index is always smaller or equal to the second index (-3<-1) and the correct syntax is-print(list[-3:-1])
How does Slicing works?
When you use slicing to return a new list of item , it is actually returning a shallow copy of the list .To deeply understand Slicing you first have to understand how simple assignment of list to a variable works in Python.
As we know Lists are mutable, so if a list object is assigned to a new variable ,it does not create a new object instead, the new variable points or reference towards the same object location that the list is pointing, this happens because - " The memory location or address of the object is passed to the new variable not the actual value inside the object at the time of assigning ". Due to this process any modification done to the new variable will also reflect back to the original object.Here is a code example for this explanation-
color = ["red","green","blue"] #color is a list of colors colur1 = color #colur1 and color both are pointing towards the same object loacation colur1.append("vgh") #modification to colur also modifies color print(color1,colur) #output similar-["red","green","blue","vgh] print(colur1 is color) #output-true "It checks if both variable are referencing to the same object location."
Now that we have understood that in python when we assign a list to a variable it never makes a copy of the object instead reference to the object memory location or address, but here slicing is an exception as it creates a new object which is a shallow copy(further we will understand that why it is known as shallow copy not copy itself) of the original object.
Slicing and its shallow copy
When a slice operation is used it creates an entire new list and returns a shallow copy of the original list, which means that it only contains the value of the original object not the actual memory location or address of the object, so in this case when you modify the shallow copy it will not reflect back to the original object because both variables are pointing to different object memory locations or addresses.
color = ["red","green","blue"] #color is a list of colors
colur1 = color[:] #colur1 conains the shallow copy of color and also colur1 and color both are pointing towards different object loacations.
colur1[2]="dsf" #colur1 is modified but color remains unchanged
print(color1,colur) #output-["red","green","blue"] ["red","green","dsf"]
Slicing of 2D Lists
In a simple list, elements inside the [ ] are known as items, and in a 2D lists or nested lists, the outer lists is called items and the inner elements are called objects. for example -
numbers = [34,43,32] #here are three items.
numbers = [[23,32,44][33,44,22]] #here are 2 items and 3 objects in each item
The reason why we say Slicing creates a Shallow Copy, not a Deep Copy
First we should understand what is a Deep Copy.
**Deep Copy -**A deep copy creates a copy of the complete list including both items and objects.
**Shallow Copy-**A shallow copy only creates a copy of the items of the list ,and references the memory location of the objects.
What this means is that shallow copy cannot make a complete copy of the list unlike deep copy. To further understand this we will take some examples.
numbers = [[3,5,7],[7,4,3]] #creation of a nested list.
num2 = numbers[:] #assigning of list to a variable through slicing.
num2.append([23,43,4]) #modifications are done in an item of num2 variable.
print(num2,numbers) #output -[[3, 5, 7], [7, 4, 3], [23, 43, 4]]
# -[[3, 5, 7], [7, 4, 3]]
As you can see in the above example, when items of the list are modified, the slice operation makes a copy of the item as seen in the previous examples.It works similar to a simple list, but what will happen if modifications are done to objects of the list?, let's see-
numbers = [[3,5,7],[7,4,3]] #creation of a nested list.
num2 = numbers[:] #assigning of list to a variable through slicing.
num2[0][2] = 100 #modification of objects in lists are done like this.
print(num2,numbers) #output-[[3,100,7],[7,4,3]]
# -[[3,100,7],[7,4,3]]
As you can see this does not create a copy and acts differently from previous example as the changes in objects of list are reflected back to the original object of the original list because it shares the same reference memory location or address.
in short ,in slice operation , when you try to modify an item it's changes does not reflect back to the original list as both their memory locations or addresses are different, but when you try to modify an objects in a list, the changes are reflected back to the original list because the memory location or addresses of both the objects of the lists are same and the the assignment is done through reference not copy.
Subscribe to my newsletter
Read articles from Karan grewal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
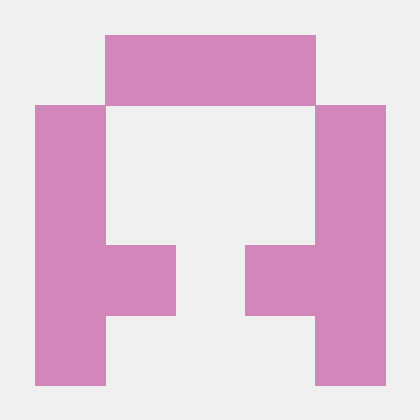
Karan grewal
Karan grewal
I am a computer science student trying to learn new technologies ๐จโ๐ป