Comprehensive Guide to Setting Up Clean URL Routing with Apache and PHP
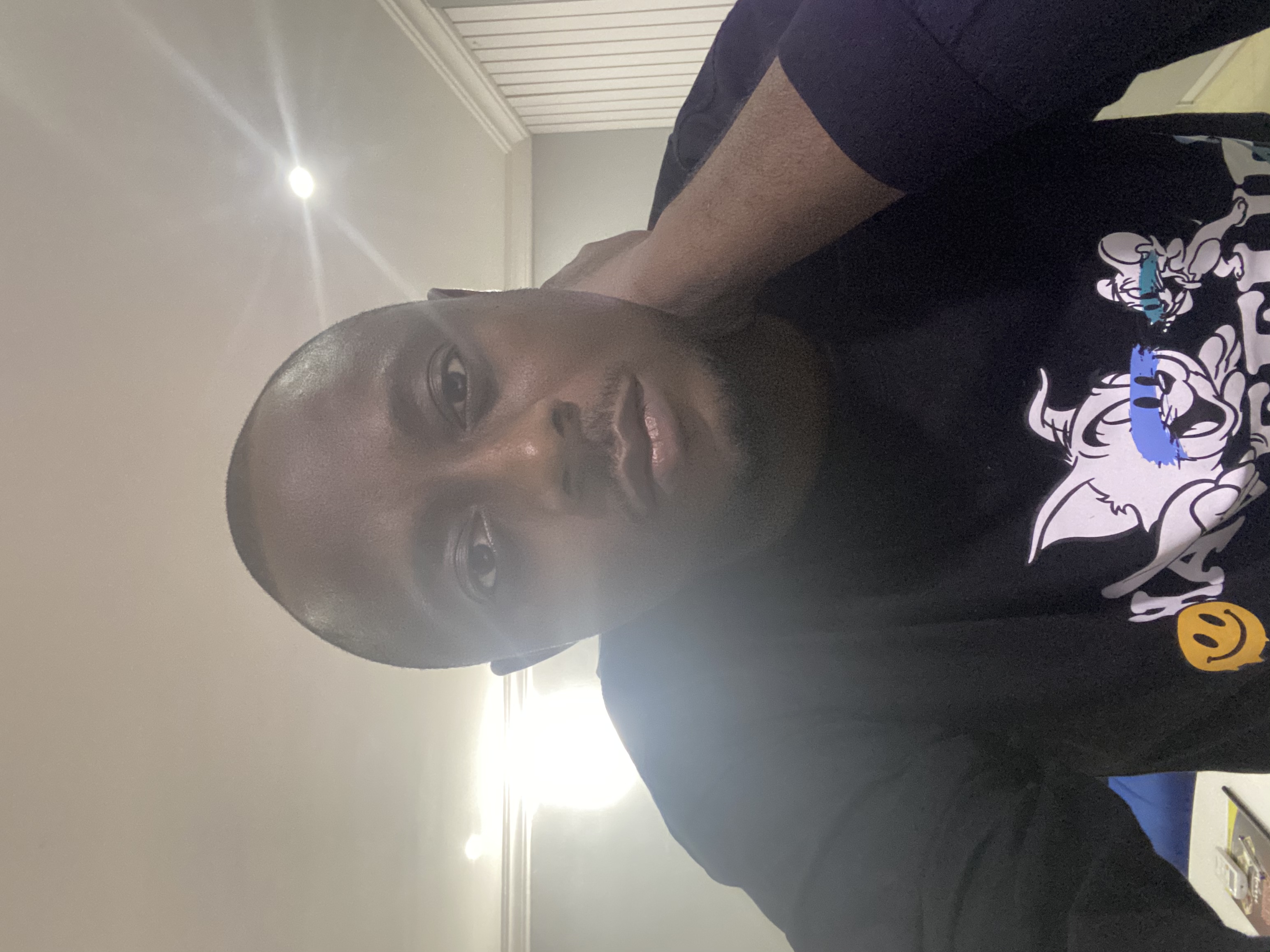
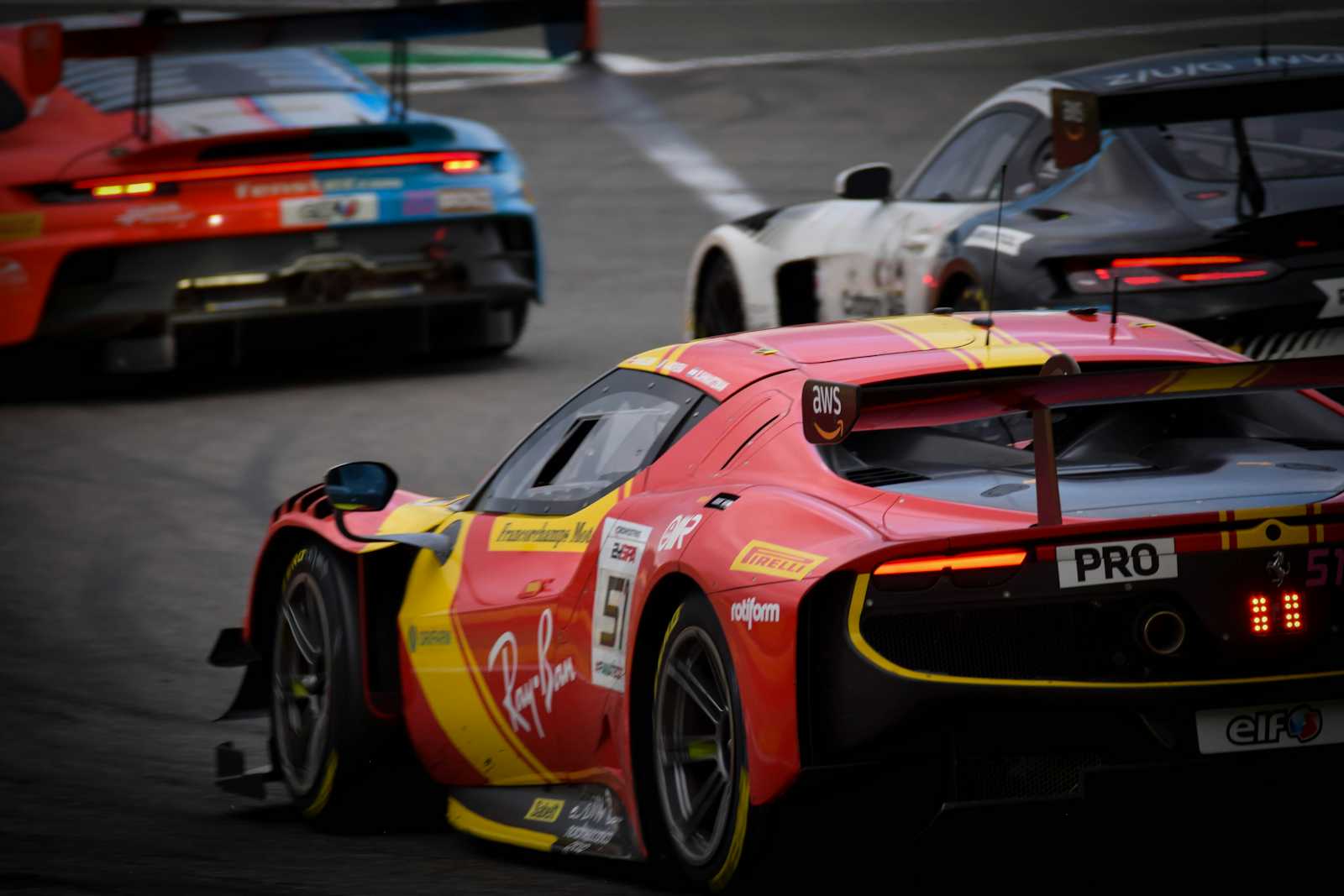
Introduction
Clean URLs enhance the readability and SEO-friendliness of web addresses, making them easier for users to remember and share. This guide will walk you through setting up clean URL routing using Apache's .htaccess
and PHP. By the end, you'll have a robust system where direct access to PHP files is restricted, and only clean URLs are accessible.
Prerequisites
A working Apache server
Basic knowledge of PHP
Access to your server's
.htaccess
file
Step-by-Step Process
1. Initial Setup
Before diving into the configuration, ensure your server allows the use of .htaccess
files. This is typically enabled by the AllowOverride All
directive in your Apache configuration.
2. Create Your Directory Structure
For this guide, let's assume the following directory structure:
/yourproject
├── /auth
│ ├── login.php
│ └── signup.php
├── /pages
│ ├── home.php
│ ├── about.php
│ ├── contact.php
│ ├── 404.php
├── /Learn
│ └── hide-url.php
└── index.php
3. Configure .htaccess
Create or update your .htaccess
file in the root directory (/yourproject
) with the following content:
RewriteEngine On
# Deny direct access to any PHP files
RewriteCond %{THE_REQUEST} \s/[^?]*\.php [NC]
RewriteCond %{REQUEST_URI} !^/index.php [NC]
RewriteRule ^ - [R=404,L]
# Route all other requests to index.php
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^(.*)$ index.php [QSA,L]
Explanation:
RewriteEngine On: Enables the rewriting engine.
Deny Direct Access to PHP Files:
RewriteCond %{THE_REQUEST} \s/[^?]*\.php [NC] RewriteCond %{REQUEST_URI} !^/index.php [NC] RewriteRule ^ - [R=404,L]
The first condition checks if the request includes a direct PHP file access.
The second condition ensures that
index.php
itself can still be accessed.If both conditions are met, it returns a 404 error, denying direct access to PHP files.
Route All Other Requests to
index.php
:RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule ^(.*)$ index.php [QSA,L]
- Routes all requests, except those for existing files or directories, to
index.php
.
- Routes all requests, except those for existing files or directories, to
4. Update index.php
for Routing
In your index.php
, add the following PHP code to handle routing based on clean URLs:
<?php
// List of available routes
$routes = [
'' => 'pages/home.php',
'about' => 'pages/about.php',
'contact' => 'pages/contact.php',
'login' => 'auth/login.php',
'signup' => 'auth/signup.php',
'submit-form' => 'Learn/hide-url.php',
];
// Get the request URI and trim any leading/trailing slashes
$request = trim($_SERVER['REQUEST_URI'], '/');
// Remove query string from request
$request = explode('?', $request, 2)[0];
// Check if the requested route exists in the routes array
if (array_key_exists($request, $routes)) {
// Include the corresponding page
include $routes[$request];
} else {
// Include the 404 page
include 'pages/404.php';
}
?>
Explanation:
List of Routes: Defines the clean URL routes and their corresponding PHP files.
Processing the Request:
Trims leading/trailing slashes from the request URI.
Removes any query string from the request.
Route Matching:
Checks if the requested route exists in the
$routes
array.Includes the corresponding PHP file if a match is found.
Includes the
404.php
file if no match is found.
Testing Your Setup
With the configuration in place, here’s how your site should behave:
Accessing Clean URLs:
http://yourdomain.com/about
should loadpages/about.php
.http://yourdomain.com/contact
should loadpages/contact.php
.
Direct Access to PHP Files:
http://yourdomain.com/pages/about.php
should result in a 404 error.http://yourdomain.com/auth/login.php
should result in a 404 error.http://yourdomain.com/Learn/hide-url.php
should result in a 404 error.
Conclusion
By following this guide, you have successfully set up clean URL routing using Apache's .htaccess
and PHP. This configuration enhances the readability and security of your URLs by ensuring that only clean URLs are accessible and direct access to PHP files is restricted.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
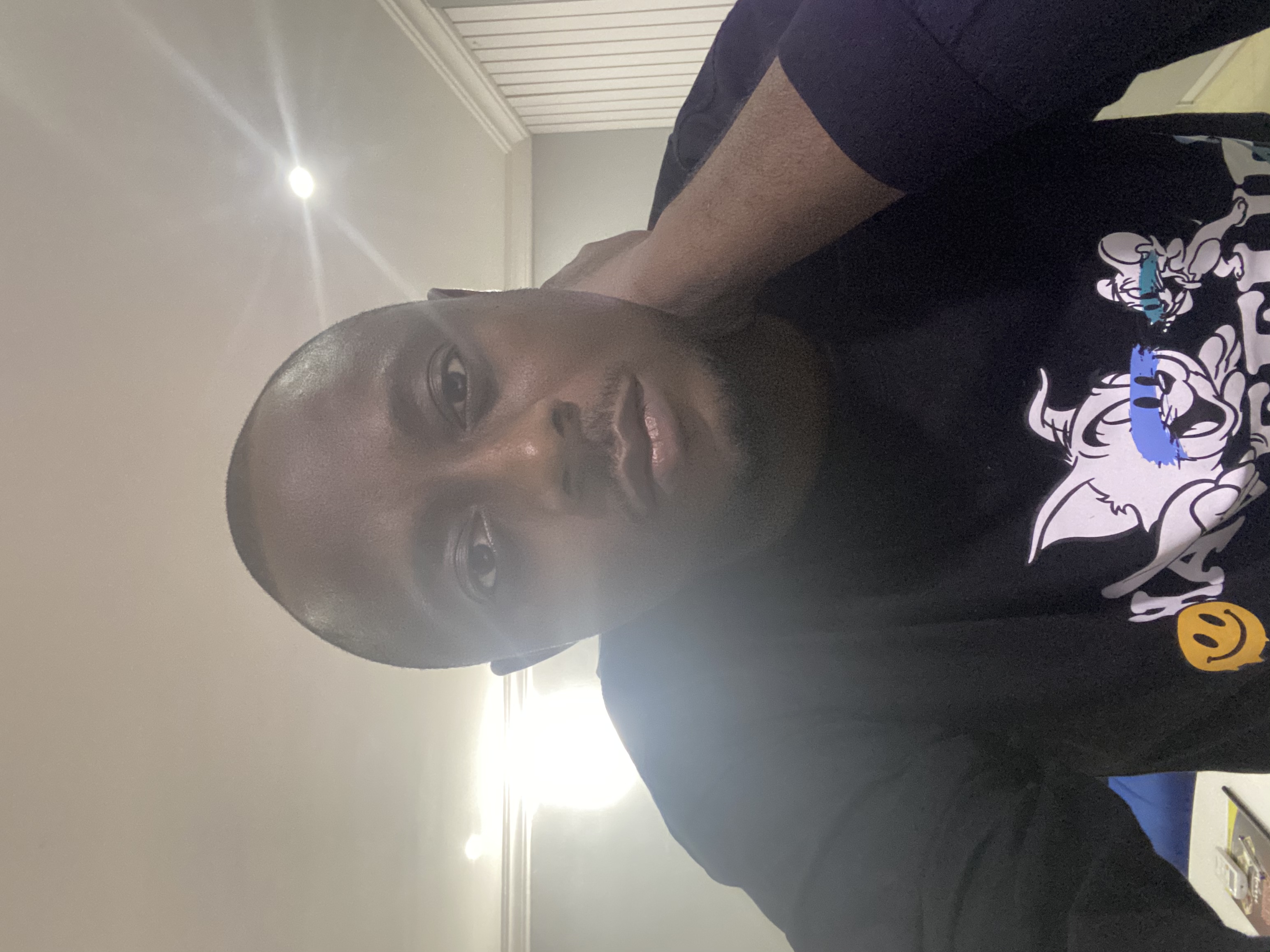
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.