Automated Testing with Selenium: A Comprehensive Guide
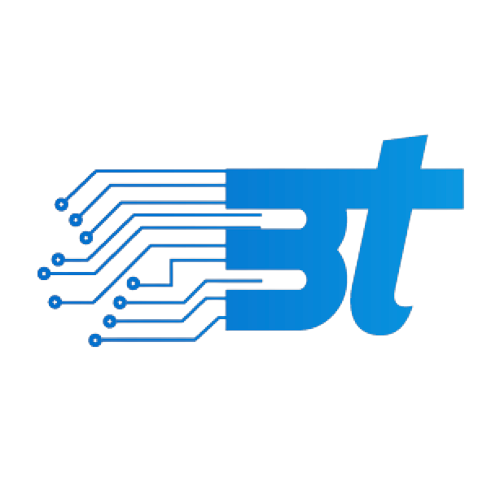
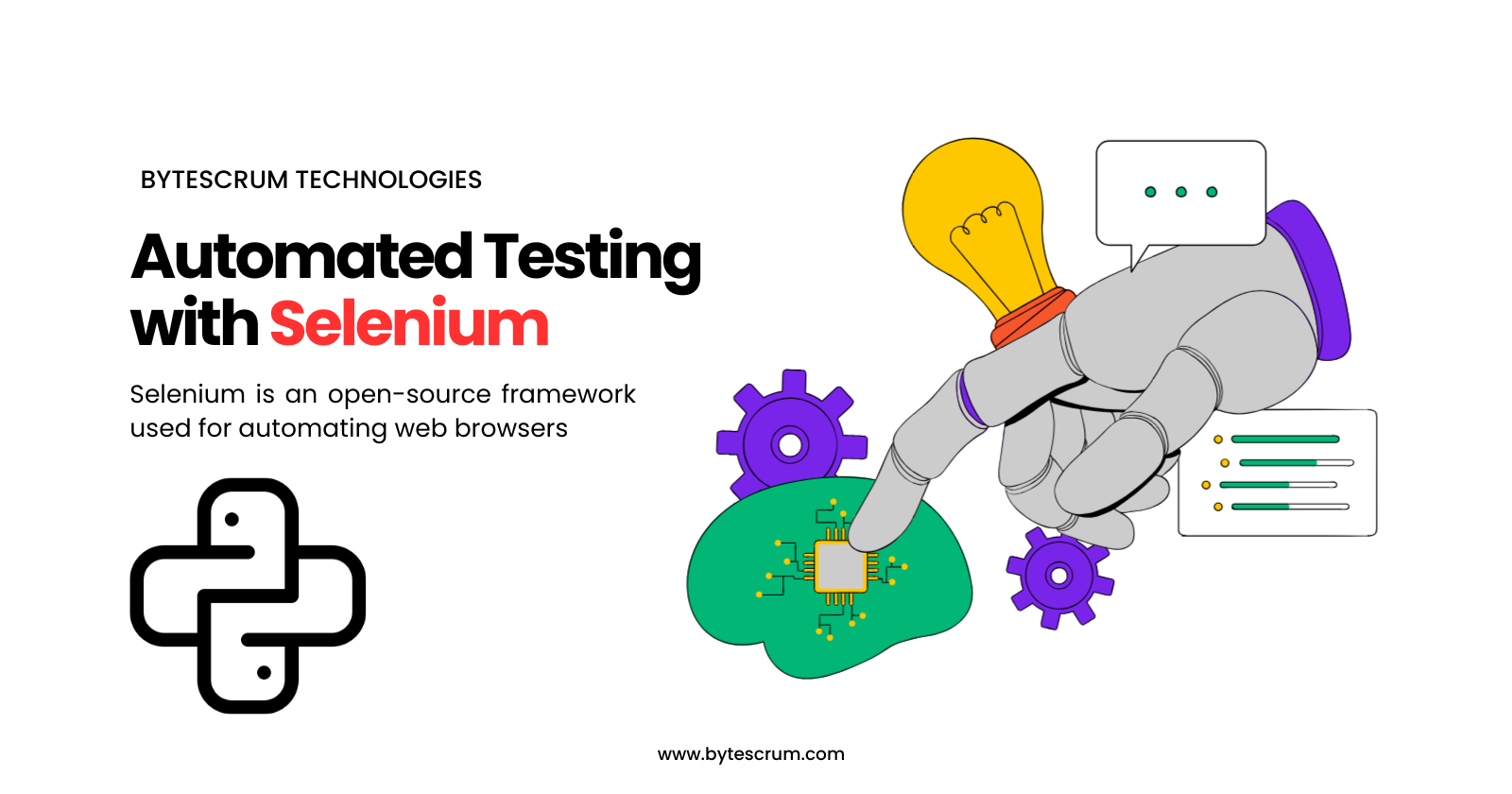
In the fast-paced world of software development, ensuring the reliability and performance of web applications is crucial. Automated testing is a key practice that helps developers and testers maintain high-quality standards without the need for repetitive manual testing. One of the most popular tools for automated web testing is Selenium. This blog will guide you through the essentials of Selenium, its setup, and how to create your first automated test.
What is Selenium?
Selenium is an open-source framework used for automating web browsers. It provides a suite of tools and libraries that allow you to automate web browser interactions, making it possible to simulate user actions like clicking, typing, and navigating through web pages. Selenium supports multiple programming languages, including Python, Java, C#, and Ruby, making it versatile for different development environments.
Why Use Selenium?
Cross-Browser Testing: Selenium supports multiple browsers such as Chrome, Firefox, Safari, and Edge, enabling you to test your web application across different browsers.
Parallel Execution: Selenium can run multiple tests in parallel, speeding up the testing process.
Integration with CI/CD: Selenium integrates seamlessly with Continuous Integration and Continuous Deployment (CI/CD) tools, allowing for automated testing as part of the development pipeline.
Flexibility: Selenium WebDriver provides a programming interface to interact with web elements, offering great flexibility in creating custom test cases.
Setting Up Selenium
Before we dive into writing tests, let’s set up Selenium in a Python environment.
Prerequisites
Python installed on your machine. You can download it from python.org.
pip, the Python package installer.
Installation
Install Selenium:
Open a terminal or command prompt and run:
pip install selenium
Download WebDriver:
Depending on the browser you want to use, download the corresponding WebDriver:
Chrome: ChromeDriver
Firefox: GeckoDriver
Edge: EdgeDriver
Make sure to add the WebDriver executable to your system’s PATH.
Writing Your First Test
Let’s create a simple test that opens a web page, searches for a term, and verifies the results.
Create a new Python script (e.g.,
test_google_search.py
):from selenium import webdriver from selenium.webdriver.common.keys import Keys import time # Set up the WebDriver driver = webdriver.Chrome() # Use the appropriate WebDriver for your browser # Open the web page driver.get("https://www.google.com") # Find the search box search_box = driver.find_element("name", "q") # Enter search term search_term = "Selenium automated testing" search_box.send_keys(search_term) # Simulate hitting the Enter key search_box.send_keys(Keys.RETURN) # Wait for the results to load time.sleep(3) # Check the title of the page assert "Selenium automated testing" in driver.title # Close the browser driver.quit()
Run the script:
python test_google_search.py
Advanced Usage
Locating Elements
Selenium provides multiple ways to locate web elements:
By ID:
element = driver.find_element("id", "element_id")
By Name:
element = driver.find_element("name", "element_name")
By XPath:
element = driver.find_element("xpath", "//tag[@attribute='value']")
By CSS Selector:
element = driver.find_element("css selector", "css_selector")
Handling Dynamic Content
Selenium can handle dynamic content by waiting for elements to appear or become interactable:
Implicit Wait:
driver.implicitly_wait(10) # Waits for up to 10 seconds for elements to appear
Explicit Wait:
from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC element = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.ID, "element_id")) )
Taking Screenshots
Selenium can take screenshots of the current browser window:
driver.save_screenshot('screenshot.png')
Integrating with Testing Frameworks
For more structured testing, integrate Selenium with testing frameworks like unittest
, pytest
, or nose
. Here’s an example using unittest
:
import unittest
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
class GoogleSearchTest(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
def test_search(self):
driver = self.driver
driver.get("https://www.google.com")
search_box = driver.find_element("name", "q")
search_box.send_keys("Selenium automated testing")
search_box.send_keys(Keys.RETURN)
self.assertIn("Selenium automated testing", driver.title)
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
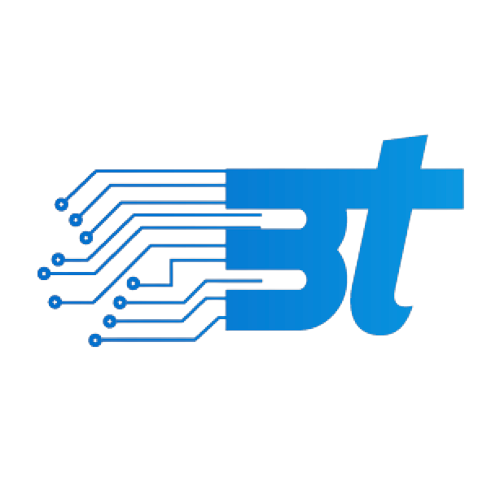
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.