Introduction to React Redux

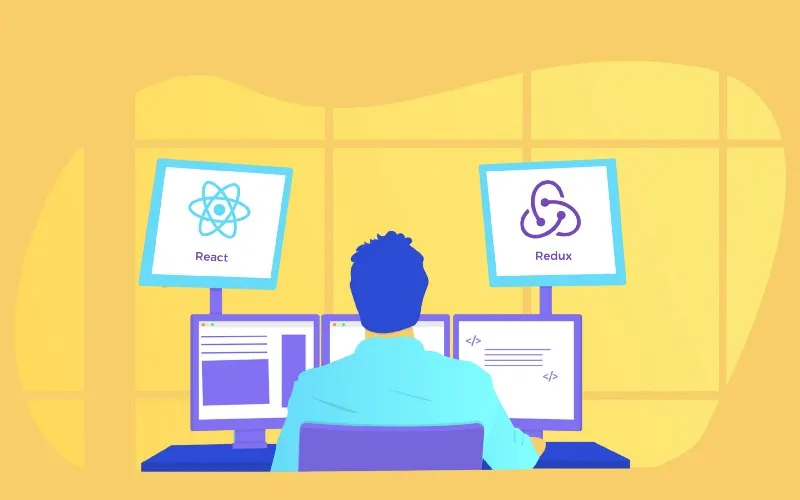
Redux is a popular library for managing application state. When combined with React, it simplifies state management by providing a central store that all components can access. Let's explore why and how to use Redux with React.
Why Use Redux?
Predictable State: Redux makes the state predictable by ensuring that state changes occur in a consistent manner through actions and reducers.
Centralized State: All application state is stored in a single place, making it easier to manage and debug.
Easier Debugging: Redux tools allow for time-travel debugging, making it easier to track state changes and identify issues.
Scalability: Redux helps in managing state for larger applications by providing a clear structure.
Unique Features of Redux
Single Source of Truth: The entire application state is stored in a single store.
State is Read-Only: The only way to change the state is by dispatching actions.
Pure Reducers: Reducers are pure functions that take the previous state and an action, and return the next state.
How to Use Redux with React
Step 1: Install Redux and React-Redux
First, install the required packages:
bashCopy codenpm install redux react-redux
Step 2: Create a Redux Store
Create a file named store.js
:
javascriptCopy codeimport { createStore } from 'redux';
// Define initial state
const initialState = {
count: 0
};
// Create a reducer
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
case 'DECREMENT':
return { ...state, count: state.count - 1 };
default:
return state;
}
};
// Create a store
const store = createStore(counterReducer);
export default store;
Step 3: Provide the Store to Your React App
Wrap your app with the Provider
component in index.js
:
javascriptCopy codeimport React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import App from './App';
import store from './store';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Step 4: Connect Components to the Redux Store
Use the useSelector
and useDispatch
hooks to access the state and dispatch actions. Update App.js
:
javascriptCopy codeimport React from 'react';
import { useSelector, useDispatch } from 'react-redux';
function App() {
// Access state
const count = useSelector(state => state.count);
// Dispatch actions
const dispatch = useDispatch();
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => dispatch({ type: 'INCREMENT' })}>Increment</button>
<button onClick={() => dispatch({ type: 'DECREMENT' })}>Decrement</button>
</div>
);
}
export default App;
Conclusion
Redux is a powerful tool for managing state in React applications, especially as they grow in complexity. By centralizing the state, providing predictability, and offering powerful debugging tools, Redux helps maintain the integrity and reliability of your application's state.
In summary:
Why Use Redux: For predictable, centralized state management.
Unique Features: Single source of truth, state is read-only, pure reducers.
How to Use:
Install Redux and React-Redux.
Create a store and define reducers.
Provide the store to your React app.
Connect components to the Redux store using hooks.
Subscribe to my newsletter
Read articles from Rohan Shrivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rohan Shrivastava
Rohan Shrivastava
Hi, I'm Rohan, a B.Tech graduate in Computer Science (Batch 2022) with expertise in web development (HTML, CSS, JavaScript, Bootstrap, PHP, XAMPP). My journey expanded with certifications and intensive training at Infosys, covering DBMS, Java, SQL, Ansible, and networking. I've successfully delivered projects, including a dynamic e-commerce site and an Inventory Management System using Java. My proactive approach is reflected in certifications and contributions to open-source projects on GitHub. Recognized for excellence at Infosys, I bring a blend of technical proficiency and adaptability. Eager to leverage my skills and contribute to innovative projects, I'm excited about exploring new opportunities for hands-on experiences. Let's connect and explore how my skills align with your organization's goals.