Linear Search Algorithm Explained: Simple Step-by-Step Guide
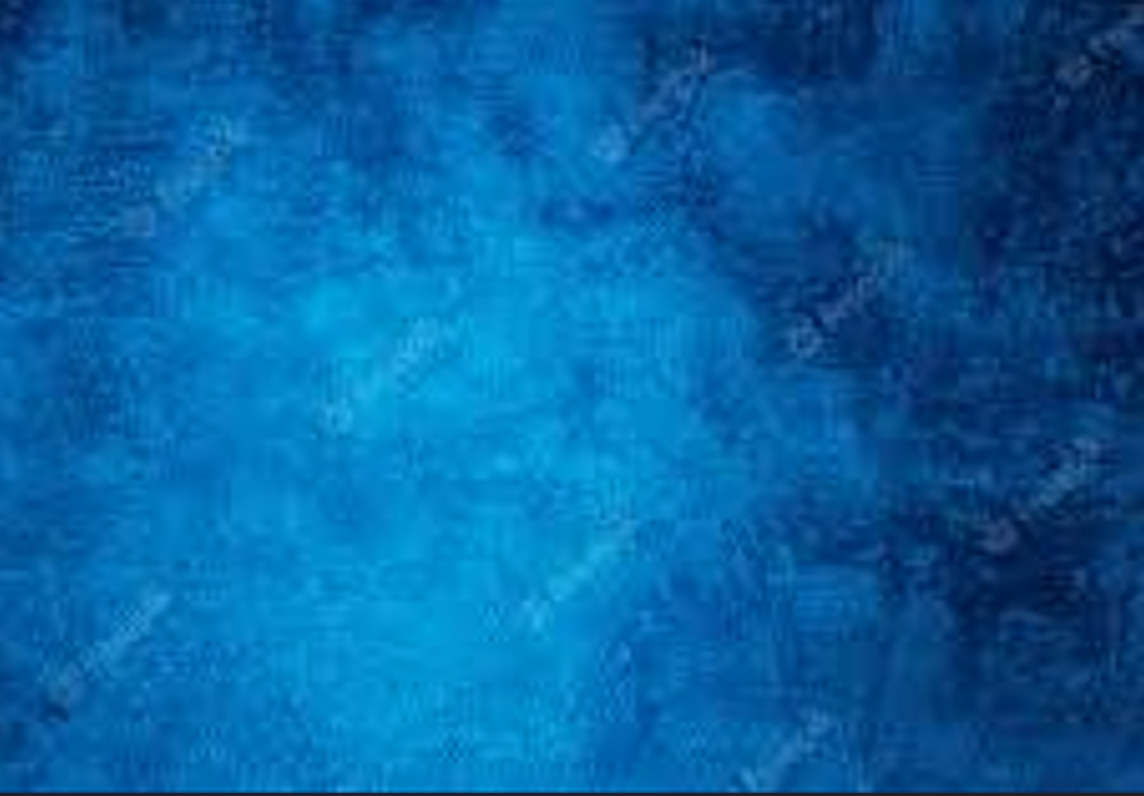
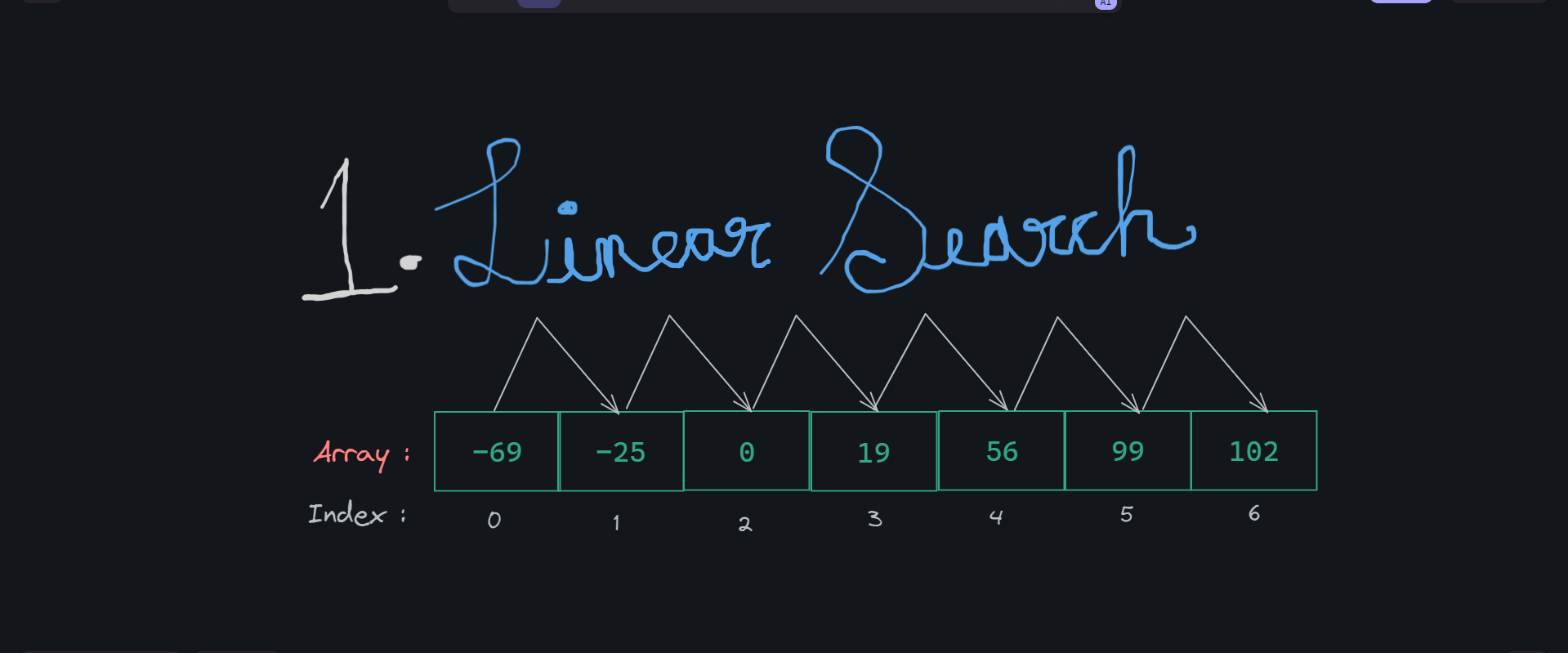
What is linear search algorithm ?
It is an algorithm used to find an element in an array. This array can be of any type (int, char, etc.).
How linear search works ?
It works by checking each element of the list one by one until the desired element is found or the list ends. This sequential checking of all the elements in the array takes time.
This is why linear search is less efficient as a search algorithm.
Algorithm overview:
Start at the beginning: Begin with the first element of the list.
Check each element: Compare the current element with the target value.
Move to the next: If the current element is not the target, move to the next element.
Repeat: Continue this process until the target value is found or the end of the list is reached.
Return result: If the target is found, return its position (index) in the list. If the target is not found, return a value indicating its absence (often -1).
Code:
public class LinearSearch {
public static void main(String[] args) throws Exception {
int[] arr = { 23, 456, 336, 267, 440 };
int n = 33;
System.out.println(search(arr, n));
}
static int search(int[] arr, int n) {
if (arr.length == 0) {
return -999;
}
for (int element : arr) {
if (element == n) {
// System.out.println("found " + element);
return 1;
}
}
return 0;
}
}
Code Explanation:
Class Definition: The
LinearSearch
class is defined, containing themain
method and thesearch
method.Main Method:
The
main
method serves as the entry point for the program.An integer array
arr
is initialized with the values{23, 456, 336, 267, 440}
.The integer
n
is set to33
, which is the target value to search for in the array.The
search
method is called witharr
andn
as arguments, and the result is printed to the console.
Search Method:
Input Check: The method first checks if the array is empty (
arr.length == 0
). If it is, it returns-999
to indicate an invalid search in an empty array.Linear Search Loop: A
for-each
loop iterates through each element in the array.For each element, it checks if it equals the target value
n
.If a match is found, it returns
1
to indicate the target value was found.
Not Found: If the loop completes without finding the target value, the method returns
0
to indicate the target value was not found in the array.
1
if the target value is found, 0
if it is not found, and -999
if the array is empty.Subscribe to my newsletter
Read articles from Shaikh Faris directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
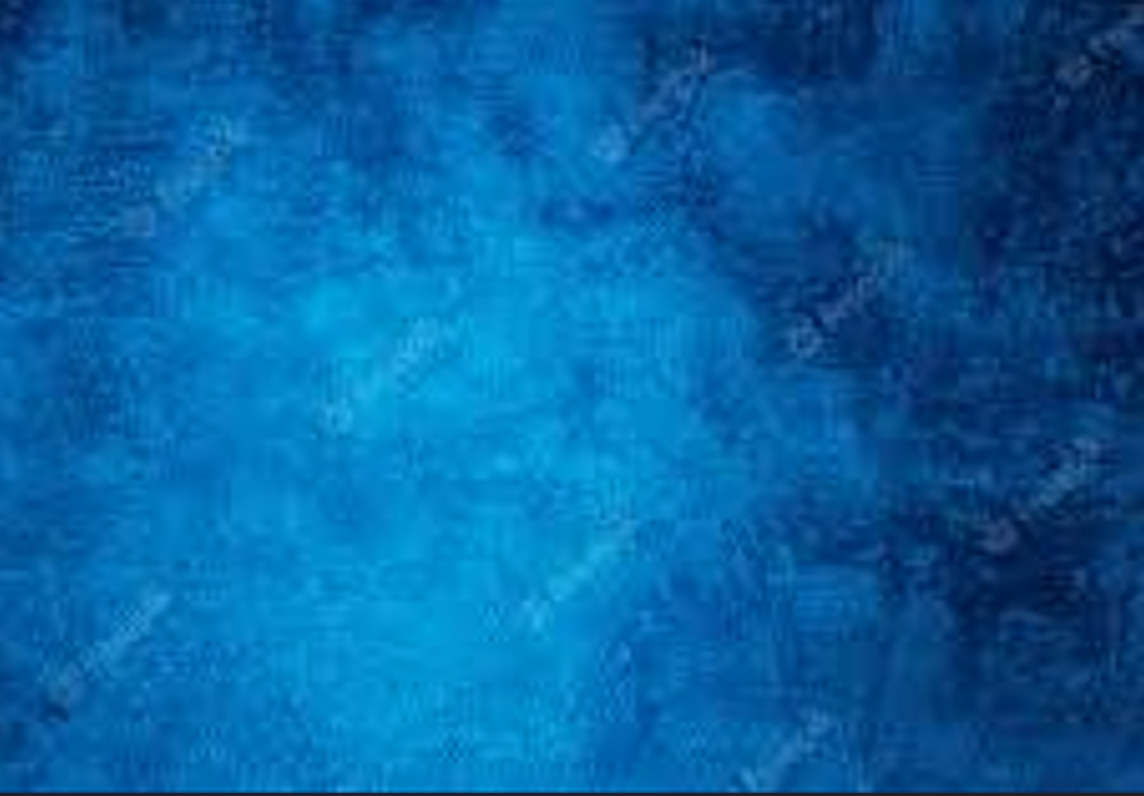