Learn Recoil in 10 Minutes ✨
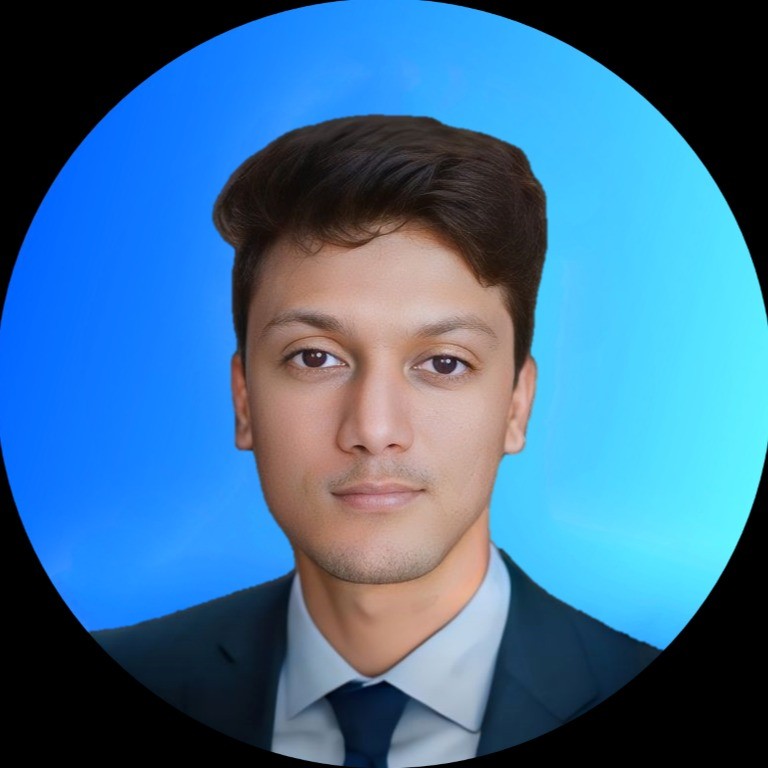
What is Recoil?
Recoil is a state management library for React that allows you to create shared state that is easily accessible and maintainable. It provides a way to manage both local and global state in a React application.
Key Concepts
Atoms:
- Atoms are units of state. They can be read from and written to from any component. Components that read the value of an atom will implicitly subscribe to that atom, so any updates will cause the component to re-render.
Selectors:
- Selectors are pure functions that transform the state. They can be used to derive state from atoms or other selectors. They are useful for computing derived state and ensuring that only the minimal number of components re-render.
Installation
To get started with Recoil, you need to install it in your project. You can do this using npm
or yarn
:
npm install recoil
# or
yarn add recoil
Basic Usage
Here is a basic example to demonstrate how to use Recoil in a React application:
Setting up RecoilRoot:
- Wrap your application in a
RecoilRoot
component to provide the Recoil state to your app.
- Wrap your application in a
import React from 'react';
import ReactDOM from 'react-dom';
import { RecoilRoot } from 'recoil';
import App from './App';
ReactDOM.render(
<RecoilRoot>
<App />
</RecoilRoot>,
document.getElementById('root')
);
Creating Atoms:
- Define an atom using the
atom
function.
- Define an atom using the
import { atom } from 'recoil';
export const textState = atom({
key: 'textState', // unique ID (with respect to other atoms/selectors)
default: '', // default value (aka initial value)
});
- Using Atoms in Components:
- Use the
useRecoilState
hook to read and write the value of an atom.
import React from 'react';
import { useRecoilState } from 'recoil';
import { textState } from './state';
function TextInput() {
const [text, setText] = useRecoilState(textState);
const onChange = (event) => {
setText(event.target.value);
};
return (
<div>
<input type="text" value={text} onChange={onChange} />
<br />
Echo: {text}
</div>
);
}
export default TextInput;
- Creating Selectors:
- Define a selector using the
selector
function to derive state.
import { selector } from 'recoil';
import { textState } from './state';
export const charCountState = selector({
key: 'charCountState',
get: ({ get }) => {
const text = get(textState);
return text.length;
},
});
- Using Selectors in Components:
- Use the
useRecoilValue
hook to read the value of a selector.
import React from 'react';
import { useRecoilValue } from 'recoil';
import { charCountState } from './state';
function CharacterCount() {
const count = useRecoilValue(charCountState);
return <>Character Count: {count}</>;
}
export default CharacterCount;
Putting It All Together
Here's how you can combine everything:
import React from 'react';
import { RecoilRoot } from 'recoil';
import TextInput from './TextInput';
import CharacterCount from './CharacterCount';
function App() {
return (
<RecoilRoot>
<TextInput />
<CharacterCount />
</RecoilRoot>
);
}
export default App;
This is a basic introduction to Recoil. The library has more features and capabilities, such as asynchronous selectors, handling complex state dependencies, and more. You can find more detailed information and advanced usage in the Recoil documentation.
Subscribe to my newsletter
Read articles from Aayush Bharti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
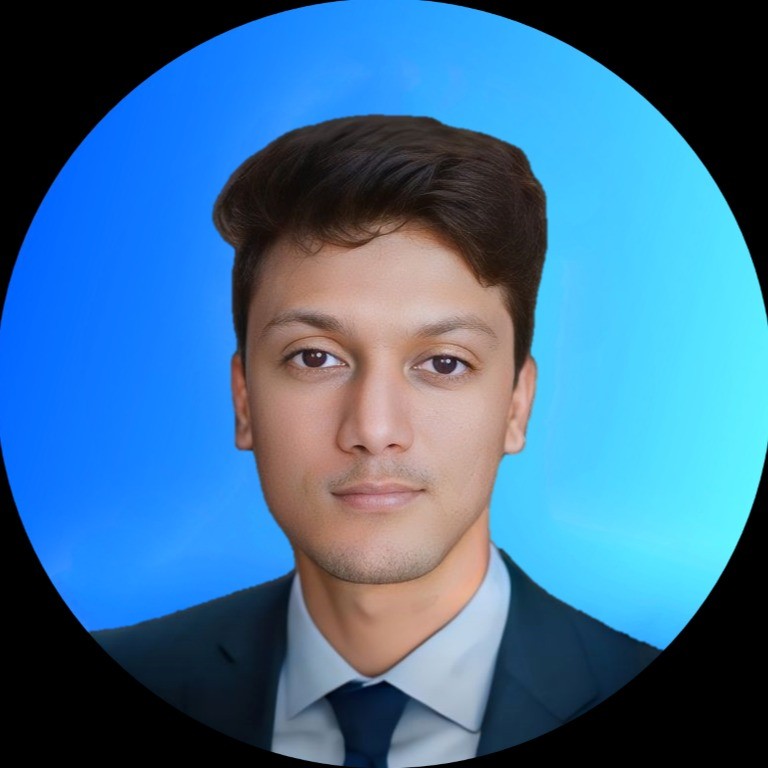
Aayush Bharti
Aayush Bharti
I'm a Next.js developer with a passion for building dynamic web experiences. I'm proficient in TypeScript and Tailwind CSS, and I love using Framer Motion to create eye-catching animations. Currently, I'm expanding my skillset by exploring Next.js's full-stack potential with Express.js and diving into databases like MongoDB and PostgreSQL. I'm always open to exciting opportunities and collaborations – feel free to reach out!